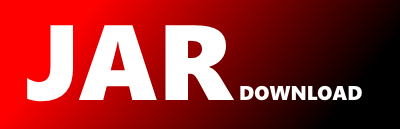
org.xlightweb.MultipartFormDataPart Maven / Gradle / Ivy
/*
* Copyright (c) xlightweb.org, 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xlightweb.org/
*/
package org.xlightweb;
import java.io.IOException;
import java.nio.BufferUnderflowException;
import java.nio.ByteBuffer;
import org.xsocket.Execution;
/**
* A part
*
* @author [email protected]
*/
final class MultipartFormDataPart extends AbstractHeader implements IPart {
private final IPartReadListener partReadListener;
private final String boundary;
private final NonBlockingBodyDataSource partBodyDataSource;
public MultipartFormDataPart(IPartReadListener partReadListener, String boundary, String[] headerlines, NonBlockingBodyDataSource messageBodyDataSource) throws IOException {
this.partReadListener = partReadListener;
this.boundary = boundary;
String encoding = AbstractHeader.DEFAULT_ENCODING;
addHeaderlines(headerlines);
partBodyDataSource = new NonBlockingBodyDataSource(encoding);
MultipartFileDataHandler dh = new MultipartFileDataHandler();
messageBodyDataSource.setDataHandler(dh);
dh.onData(messageBodyDataSource);
}
public String getName() {
return getDispositionParam("name");
}
public String getOriginalFilename() {
return getDispositionParam("filename");
}
public void close() throws IOException {
partBodyDataSource.close();
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder(super.toString());
sb.append("\r\n" + partBodyDataSource.toString());
return sb.toString();
}
public BlockingBodyDataSource getBlockingBody() throws IOException {
return new BlockingBodyDataSource(partBodyDataSource);
}
public NonBlockingBodyDataSource getNonBlockingBody() throws IOException {
return partBodyDataSource;
}
public boolean hasBody() {
return true;
}
static interface IPartReadListener {
void onPartRead(MultipartFormDataPart part);
void onRequestRead();
void onException(IOException ioe);
}
public void destroy() {
partBodyDataSource.destroy();
}
@Execution(Execution.NONTHREADED)
private final class MultipartFileDataHandler implements IBodyDataHandler {
private final String intermediateBoundary = "--" + boundary + "\r\n";
private final String endBoundary = "\r\n--" + boundary + "--\r\n";
public boolean onData(NonBlockingBodyDataSource bodyDataSource) throws BufferUnderflowException {
try {
if (partBodyDataSource.isComplete()) {
return true;
}
int idx = bodyDataSource.indexOf(intermediateBoundary);
if (idx != -1) {
ByteBuffer[] data = bodyDataSource.readByteBufferByLength(idx);
partBodyDataSource.append(data);
partBodyDataSource.setComplete(true);
partReadListener.onPartRead(MultipartFormDataPart.this);
} else {
idx = bodyDataSource.indexOf(endBoundary);
if (idx != -1) {
ByteBuffer[] data = bodyDataSource.readByteBufferByLength(idx);
partBodyDataSource.append(data);
// remove end boundary
bodyDataSource.readByteBufferByLength(endBoundary.length());
partBodyDataSource.setComplete(true);
partReadListener.onPartRead(MultipartFormDataPart.this);
partReadListener.onRequestRead();
}
}
} catch (IOException ioe) {
ioe.printStackTrace();
}
return true;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy