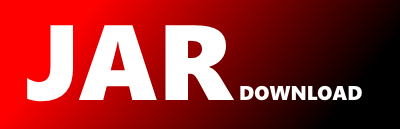
org.xlightweb.client.CookieHandler Maven / Gradle / Ivy
/*
* Copyright (c) xlightweb.org, 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xlightweb.org/
*/
package org.xlightweb.client;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.xlightweb.IHttpExchange;
import org.xlightweb.IHttpRequest;
import org.xlightweb.IHttpRequestHandler;
import org.xlightweb.IHttpResponse;
import org.xlightweb.IHttpResponseHandler;
import org.xsocket.Execution;
/**
* Cookie handler
*
* @author [email protected]
*/
final class CookieHandler implements IHttpRequestHandler {
private static final Logger LOG = Logger.getLogger(CookieHandler.class.getName());
private static final String COOKIE_WARNING_KEY = "org.xlightweb.client.cookieHandler.cookieWarning";
private final CookieManager cookieManager = new CookieManager();
/**
* {@inheritDoc}
*/
@Execution(Execution.NONTHREADED)
public void onRequest(final IHttpExchange exchange) throws IOException {
IHttpResponseHandler respHdl = new IHttpResponseHandler() {
@Execution(Execution.NONTHREADED)
public void onResponse(IHttpResponse response) throws IOException {
Map> responseHeaders = new HashMap>();
for (String headername : response.getHeaderNameSet()) {
responseHeaders.put(headername, response.getHeaderList(headername));
}
try {
cookieManager.put(getRequestURI(exchange), responseHeaders);
} catch (URISyntaxException ue) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("invcalid URI. ignore handling cookies " + ue.toString());
}
}
exchange.send(response);
}
public void onException(IOException ioe) throws IOException {
exchange.sendError(ioe);
}
};
IHttpRequest request = exchange.getRequest();
try {
Map> cookieHeaders = cookieManager.get(getRequestURI(exchange));
for (Entry> entry : cookieHeaders.entrySet()) {
if (!entry.getValue().isEmpty()) {
StringBuilder sb = new StringBuilder();
List cookies = entry.getValue();
for (int i = 0; i < cookies.size(); i++) {
sb.append(cookies.get(i));
if ((i +1) < cookies.size()) {
sb.append("; ");
}
}
if ((request.getHeader("Cookie") != null) && Boolean.parseBoolean(System.getProperty(COOKIE_WARNING_KEY, "true"))) {
LOG.warning("cookie is set manually and auto handle cookie is activate " +
"(hint: deactivate auto handling cookie by calling .setAutoHandleCookies(false) or " +
"suppress this message by setting system property 'org.xlightweb.client.cookieHandler.cookieWarning=false')");
}
request.addHeader(entry.getKey(), sb.toString());
}
}
} catch (URISyntaxException ue) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("invcalid URI. ignore handling cookies " + ue.toString());
}
}
exchange.forward(request, respHdl);
}
private URI getRequestURI(IHttpExchange exchange) throws URISyntaxException {
return exchange.getRequest().getRequestUrl().toURI();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy