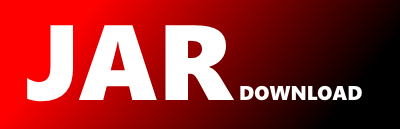
org.xlightweb.server.SessionManager Maven / Gradle / Ivy
/*
* Copyright (c) xlightweb.org, 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xlightweb.org/
*/
package org.xlightweb.server;
import java.io.IOException;
import java.lang.ref.WeakReference;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.TimerTask;
import java.util.UUID;
import java.util.Map.Entry;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* In-memory SessionManager implementation.
*
* @author [email protected]
*/
public class SessionManager implements ISessionManager {
public static final Logger LOG = Logger.getLogger(SessionManager.class.getName());
private static final List> INSTANCE_LIST = Collections.synchronizedList(new ArrayList>());
private static final long CLEANING_PERIOD_MILLIS = 5 * 1000;
static {
TimerTask cleaningTask = new TimerTask() {
@Override
public void run() {
for (SessionManager sessionManager : getInstances()) {
sessionManager.clean();
}
}
};
HttpServerConnection.schedule(cleaningTask, CLEANING_PERIOD_MILLIS, CLEANING_PERIOD_MILLIS);
}
private final HashMap sessions = new HashMap();
/**
* constructor
*/
public SessionManager() {
WeakReference sessionManagerRef = new WeakReference(this);
INSTANCE_LIST.add(sessionManagerRef);
}
private static List getInstances() {
List instances = new ArrayList();
boolean isCleanRequired = false;
for (WeakReference sessionManagerRef : INSTANCE_LIST) {
SessionManager sessionManager = sessionManagerRef.get();
if (sessionManager != null) {
instances.add(sessionManager);
} else {
isCleanRequired = true;
}
}
if (isCleanRequired) {
cleanInstances();
}
return instances;
}
private static void cleanInstances() {
List> refsToDelete = new ArrayList>();
for (WeakReference sessionManagerRef : INSTANCE_LIST) {
SessionManager sessionManager = sessionManagerRef.get();
if (sessionManager == null) {
refsToDelete.add(sessionManagerRef);
}
}
for (WeakReference sessionManagerRef : refsToDelete) {
INSTANCE_LIST.remove(sessionManagerRef);
}
}
/**
* {@inheritDoc}
*/
public boolean isEmtpy() {
return sessions.isEmpty();
}
/**
* {@inheritDoc}
*/
public HttpSession getSession(String sessionId) {
synchronized (sessions) {
HttpSession session = sessions.get(sessionId);
if (session != null) {
long currentMillis = System.currentTimeMillis();
session.setLastAccessTime(currentMillis);
if (!session.isValid()) {
sessions.remove(session.getId());
session = null;
}
}
return session;
}
}
/**
* {@inheritDoc}
*/
public void registerSession(HttpSession session) throws IOException {
synchronized (sessions) {
sessions.put(session.getId(), session);
}
}
/**
* {@inheritDoc}
*/
public void saveSession(String sessionId) throws IOException {
}
/**
* {@inheritDoc}
*/
public String newSession(String idPrefix) throws IOException {
String sessionId = idPrefix + "-" + UUID.randomUUID().toString();
registerSession(new HttpSession(sessionId));
return sessionId;
}
/**
* {@inheritDoc}
*/
public void removeSession(String sessionId) {
synchronized (sessions) {
sessions.remove(sessionId);
}
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
public void close() {
HashMap sessionsCopy = null;
synchronized (sessions) {
sessionsCopy = (HashMap) sessions.clone();
}
for (HttpSession httpSession : sessionsCopy.values()) {
httpSession.invalidate();
}
}
@SuppressWarnings("unchecked")
void clean() {
try {
HashMap sessionsCopy = null;
synchronized (sessions) {
sessionsCopy = (HashMap) sessions.clone();
}
for (Entry entry : sessionsCopy.entrySet()) {
if (!entry.getValue().isValid()) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("session " + entry.getValue() + " has been expired. Deleting it");
}
removeSession(entry.getValue().getId());
}
}
} catch (Exception e) {
// eat and log exception
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("error occured by cleaning sessions " + e.toString());
}
}
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder(super.toString());
sb.append(" (");
for (Entry entry: sessions.entrySet()) {
sb.append(entry.getKey() + "-> " + entry.getValue() + " ");
}
sb.append(")");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy