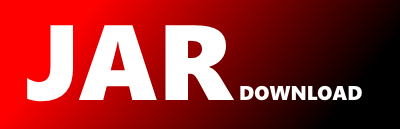
org.xmlcml.cml.inchi.InChIToStructure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jumbo-inchi Show documentation
Show all versions of jumbo-inchi Show documentation
A container for JUMBO's InChI functionality
The newest version!
/**
* Copyright 2011 Peter Murray-Rust et. al.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xmlcml.cml.inchi;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import net.sf.jniinchi.INCHI_BOND_TYPE;
import net.sf.jniinchi.INCHI_RET;
import net.sf.jniinchi.JniInchiAtom;
import net.sf.jniinchi.JniInchiBond;
import net.sf.jniinchi.JniInchiException;
import net.sf.jniinchi.JniInchiInputInchi;
import net.sf.jniinchi.JniInchiOutputStructure;
import net.sf.jniinchi.JniInchiWrapper;
import org.xmlcml.cml.base.CMLConstants;
import org.xmlcml.cml.element.CMLAtom;
import org.xmlcml.cml.element.CMLBond;
import org.xmlcml.cml.element.CMLMolecule;
import org.xmlcml.molutil.ChemicalElement.AS;
/**
* This class generates a CMLMolecule from an InChI string. It places
* calls to a JNI wrapper for the InChI C++ library.
*
*
The generated IAtomContainer will have all 2D and 3D coordinates set to 0.0,
* but may have atom parities set. Double bond and allene stereochemistry are
* not currently recorded.
*
*
Example usage
*
* // Generate factory - if native code does not load
* InChIGeneratorFactory factory = new InChIGeneratorFactory();
* // Get InChIToStructure
* InChIToStructure intostruct = factory.getInChIToStructure(inchi);
*
* INCHI_RET intostruct = gen.getReturnStatus();
* if (ret == INCHI_RET.WARNING) {
* // Structure generated, but with warning message
* System.out.println("InChI warning: " + intostruct.getMessage());
* } else if (ret != INCHI_RET.OKAY) {
* // Structure generation failed
* throw new CMLxception("Structure generation failed failed: " + ret.toString()
* + " [" + intostruct.getMessage() + S_RSQUARE);
* }
*
* CMLMolecule molecule = intostruct.getMolecule();
*
* @author Sam Adams
*/
public class InChIToStructure implements CMLConstants {
protected JniInchiInputInchi input;
protected JniInchiOutputStructure output;
protected CMLMolecule molecule;
/**
* Constructor. Generates CMLMolecule from InChI.
* @param inchi
* @throws RuntimeException
*/
public InChIToStructure(String inchi) {
try {
input = new JniInchiInputInchi(inchi, S_EMPTY);
} catch (JniInchiException jie) {
throw new RuntimeException("Failed to convert InChI to molecule: " + jie.getMessage());
}
generateCMLMoleculeFromInchi();
}
/**
* Constructor. Generates CMLMolecule from InChI.
* @param inchi
* @param options
* @throws RuntimeException
*/
public InChIToStructure(String inchi, String options) {
try {
input = new JniInchiInputInchi(inchi, options);
} catch (JniInchiException jie) {
throw new RuntimeException("Failed to convert InChI to molecule: " + jie.getMessage());
}
generateCMLMoleculeFromInchi();
}
/**
* Constructor. Generates CMLMolecule from InChI.
* @param inchi
* @param options
* @throws RuntimeException
*/
public InChIToStructure(String inchi, List options) {
try {
input = new JniInchiInputInchi(inchi, options);
} catch (JniInchiException jie) {
throw new RuntimeException("Failed to convert InChI to molecule: " + jie.getMessage());
}
generateCMLMoleculeFromInchi();
}
protected void generateCMLMoleculeFromInchi() {
try {
output = JniInchiWrapper.getStructureFromInchi(input);
} catch (JniInchiException jie) {
throw new RuntimeException("Failed to convert InChI to molecule: " + jie.getMessage());
}
molecule = new CMLMolecule();
Map inchiCmlAtomMap = new HashMap();
for (int i = 0; i < output.getNumAtoms(); i ++) {
JniInchiAtom iAt = output.getAtom(i);
CMLAtom cAt = new CMLAtom();
inchiCmlAtomMap.put(iAt, cAt);
cAt.setId("a" + i);
cAt.setElementType(iAt.getElementType());
// Ignore coordinates - all zero
int charge = iAt.getCharge();
if (charge != 0) {
cAt.setFormalCharge(charge);
}
int numH = iAt.getImplicitH();
if (numH != 0) {
cAt.setHydrogenCount(numH);
}
molecule.addAtom(cAt);
}
for (int i = 0; i < output.getNumBonds(); i ++) {
JniInchiBond iBo = output.getBond(i);
CMLAtom atO = inchiCmlAtomMap.get(iBo.getOriginAtom());
CMLAtom atT = inchiCmlAtomMap.get(iBo.getTargetAtom());
CMLBond cBo = new CMLBond(atO, atT);
INCHI_BOND_TYPE type = iBo.getBondType();
if (type == INCHI_BOND_TYPE.SINGLE) {
cBo.setOrder(CMLBond.SINGLE_S);
} else if (type == INCHI_BOND_TYPE.DOUBLE) {
cBo.setOrder(CMLBond.DOUBLE_D);
} else if (type == INCHI_BOND_TYPE.TRIPLE) {
cBo.setOrder(CMLBond.TRIPLE_T);
} else if (type == INCHI_BOND_TYPE.ALTERN) {
cBo.setOrder(CMLBond.AROMATIC);
} else {
throw new RuntimeException("Unknown bond type: " + type);
}
// TODO: bond sterochemistry
molecule.addBond(cBo);
}
// Add explict hydrogens to hydrogen counts
for (int i = 0; i < molecule.getAtomCount(); i ++) {
CMLAtom at = molecule.getAtom(i);
if (at.getHydrogenCountAttribute() != null) {
List ligandList = at.getLigandAtoms();
int hLigands = 0;
for (int j = 0; j < ligandList.size(); j ++) {
if (AS.H.equals(ligandList.get(j).getElementType())) {
hLigands ++;
}
}
if (hLigands > 0) {
int numH = at.getHydrogenCount() + hLigands;
at.setHydrogenCount(numH);
}
}
}
}
/**
* Returns generated molecule.
* @return CMLMolecule
*/
public CMLMolecule getMolecule() {
return(molecule);
}
/**
* Gets return status from InChI process. OKAY and WARNING indicate
* InChI has been generated, in all other cases InChI generation
* has failed.
* @return status
*/
public INCHI_RET getReturnStatus() {
return(output.getReturnStatus());
}
/**
* Gets generated (error/warning) messages.
* @return message
*/
public String getMessage() {
return(output.getMessage());
}
/**
* Gets generated log.
* @return log
*/
public String getLog() {
return(output.getLog());
}
/**
* Returns warning flags, see INCHIDIFF in inchicmp.h.
*
*
[x][y]:
*
x=0 => Reconnected if present in InChI otherwise Disconnected/Normal
*
x=1 => Disconnected layer if Reconnected layer is present
*
y=1 => Main layer or Mobile-H
*
y=0 => Fixed-H layer
* @return flags
*/
public long[][] getWarningFlags() {
return(output.getWarningFlags());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy