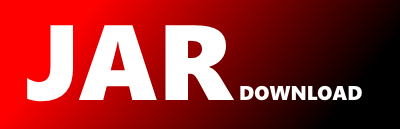
org.xmlactions.action.config.FileExecContext Maven / Gradle / Ivy
package org.xmlactions.action.config;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings("serial")
public class FileExecContext extends ExecContext {
private static final Logger log = LoggerFactory.getLogger(FileExecContext.class);
String fileName;
public FileExecContext(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy