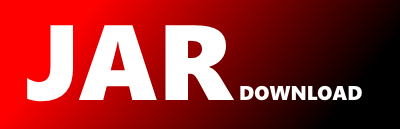
org.xmlactions.pager.actions.HttpAction Maven / Gradle / Ivy
package org.xmlactions.pager.actions;
/**
\page action_code_action Action Code
A code action invokes java code during the construction of the web page on the server. The response, if any will replace the code element on the web page.
Action:code
Elements
Description
param
- optional
One or more param elements may be added to the action. These will be added in order to the method invocation. See \ref action_param
Attributes
Description
call
- required
The full package, class and method name. As an example "org.xmlactions.utils.Class.methodName"
An example of how it looks on the web page
\code
\endcode
When this call is invoked the response from the call replaces the code action element. If the response was "Hello World!!!" then
\code
\endcode
becomes
"Hello World!!!"
The action will also accept parameters. As an example
\code
\endcode
This call will invoke
\code
org.xmlactions.utils.Class.methodName(int i, String s)
\endcode
\see \ref action_param
*/
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang.StringUtils;
import org.apache.commons.lang.Validate;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpEntity;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
import org.xmlactions.action.actions.BaseAction;
import org.xmlactions.action.config.IExecContext;
public class HttpAction extends BaseAction
{
private static Logger log = LoggerFactory.getLogger(HttpAction.class);
private String method; // the http methid to call such as get or port
private String href; // the http address to call without parameters such as http://google.com
private String key; // use this if we want to store the result of the http call to exec
private List params = new ArrayList();
public List getParams()
{
return params;
}
public void setParams(List params)
{
this.params = params;
}
public void setParam(Param param)
{
params.add((Param) param);
}
/**
* gets the last param in the list or null if none found.
*
* @return
*/
public Param getParam()
{
if (params.size() == 0) {
return null;
}
return params.get(params.size() - 1);
}
public void setChild(Param param)
{
params.add(param);
}
public void _setChild(BaseAction param)
{
Validate.isTrue(param instanceof Param, "Parameter must be a " + Param.class.getName());
params.add((Param) param);
}
public String execute(IExecContext execContext) throws Exception
{
String error = "";
validate();
RestTemplate restTemplate = new RestTemplate();
ResponseEntity response = null;
if (getMethod().equalsIgnoreCase("get")) {
String url = execContext.replace(getHref()) + buildParamsForGet(execContext);
try {
if (log.isInfoEnabled()) {
log.info("get:{}", url);
}
response = restTemplate.getForEntity(url, String.class);
} catch (Exception ex) {
error = buildError(ex, url);
}
} else if (getMethod().equalsIgnoreCase("post")) {
String url = execContext.replace(getHref());
try {
Map map = buildParamsForPost(execContext);
HttpEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy