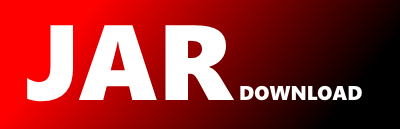
org.xrpl.xrpl4j.wallet.ImmutableWallet Maven / Gradle / Ivy
package org.xrpl.xrpl4j.wallet;
import com.google.common.base.MoreObjects;
import com.google.common.primitives.Booleans;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import org.xrpl.xrpl4j.model.transactions.Address;
import org.xrpl.xrpl4j.model.transactions.XAddress;
/**
* Immutable implementation of {@link Wallet}.
*
* Use the builder to create immutable instances:
* {@code ImmutableWallet.builder()}.
*/
@Generated(from = "Wallet", generator = "Immutables")
@SuppressWarnings({"all", "deprecation"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableWallet implements Wallet {
private final @Nullable String privateKey;
private final String publicKey;
private final Address classicAddress;
private final XAddress xAddress;
private final boolean isTest;
private ImmutableWallet(
@Nullable String privateKey,
String publicKey,
Address classicAddress,
XAddress xAddress,
boolean isTest) {
this.privateKey = privateKey;
this.publicKey = publicKey;
this.classicAddress = classicAddress;
this.xAddress = xAddress;
this.isTest = isTest;
}
/**
* The private key of the wallet, encoded in hexadecimal.
* @return An optionally present {@link String} containing a private key.
* @deprecated This method will be removed in a future version. Consider storing private keys in an associated
* instance of TransactionSigner.
*/
@Deprecated
@Override
public Optional privateKey() {
return Optional.ofNullable(privateKey);
}
/**
* The public key of the wallet, encoded in hexadecimal.
* @return A {@link String} containing a public key.
*/
@Override
public String publicKey() {
return publicKey;
}
/**
* The XRPL address of this wallet, in the Classic Address form.
* @return The classic {@link Address} of this wallet.
*/
@Override
public Address classicAddress() {
return classicAddress;
}
/**
* The XRPL address of this wallet, in the X-Address form.
* @return An {@link XAddress} containing the X-Address of this wallet.
*/
@Override
public XAddress xAddress() {
return xAddress;
}
/**
* Whether or not this wallet is on XRPL testnet or mainnet.
* @return A boolean indicating if this is a testnet or mainnet wallet.
*/
@Override
public boolean isTest() {
return isTest;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Wallet#privateKey() privateKey} attribute.
* @param value The value for privateKey
* @return A modified copy of {@code this} object
*/
@Deprecated
public final ImmutableWallet withPrivateKey(String value) {
@Nullable String newValue = Objects.requireNonNull(value, "privateKey");
if (Objects.equals(this.privateKey, newValue)) return this;
return new ImmutableWallet(newValue, this.publicKey, this.classicAddress, this.xAddress, this.isTest);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Wallet#privateKey() privateKey} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for privateKey
* @return A modified copy of {@code this} object
*/
@Deprecated
public final ImmutableWallet withPrivateKey(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.privateKey, value)) return this;
return new ImmutableWallet(value, this.publicKey, this.classicAddress, this.xAddress, this.isTest);
}
/**
* Copy the current immutable object by setting a value for the {@link Wallet#publicKey() publicKey} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for publicKey
* @return A modified copy of the {@code this} object
*/
public final ImmutableWallet withPublicKey(String value) {
String newValue = Objects.requireNonNull(value, "publicKey");
if (this.publicKey.equals(newValue)) return this;
return new ImmutableWallet(this.privateKey, newValue, this.classicAddress, this.xAddress, this.isTest);
}
/**
* Copy the current immutable object by setting a value for the {@link Wallet#classicAddress() classicAddress} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for classicAddress
* @return A modified copy of the {@code this} object
*/
public final ImmutableWallet withClassicAddress(Address value) {
if (this.classicAddress == value) return this;
Address newValue = Objects.requireNonNull(value, "classicAddress");
return new ImmutableWallet(this.privateKey, this.publicKey, newValue, this.xAddress, this.isTest);
}
/**
* Copy the current immutable object by setting a value for the {@link Wallet#xAddress() xAddress} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for xAddress
* @return A modified copy of the {@code this} object
*/
public final ImmutableWallet withXAddress(XAddress value) {
if (this.xAddress == value) return this;
XAddress newValue = Objects.requireNonNull(value, "xAddress");
return new ImmutableWallet(this.privateKey, this.publicKey, this.classicAddress, newValue, this.isTest);
}
/**
* Copy the current immutable object by setting a value for the {@link Wallet#isTest() isTest} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isTest
* @return A modified copy of the {@code this} object
*/
public final ImmutableWallet withIsTest(boolean value) {
if (this.isTest == value) return this;
return new ImmutableWallet(this.privateKey, this.publicKey, this.classicAddress, this.xAddress, value);
}
/**
* This instance is equal to all instances of {@code ImmutableWallet} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableWallet
&& equalTo((ImmutableWallet) another);
}
private boolean equalTo(ImmutableWallet another) {
return Objects.equals(privateKey, another.privateKey)
&& publicKey.equals(another.publicKey)
&& classicAddress.equals(another.classicAddress)
&& xAddress.equals(another.xAddress)
&& isTest == another.isTest;
}
/**
* Computes a hash code from attributes: {@code privateKey}, {@code publicKey}, {@code classicAddress}, {@code xAddress}, {@code isTest}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Objects.hashCode(privateKey);
h += (h << 5) + publicKey.hashCode();
h += (h << 5) + classicAddress.hashCode();
h += (h << 5) + xAddress.hashCode();
h += (h << 5) + Booleans.hashCode(isTest);
return h;
}
/**
* Prints the immutable value {@code Wallet} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Wallet")
.omitNullValues()
.add("privateKey", privateKey)
.add("publicKey", publicKey)
.add("classicAddress", classicAddress)
.add("xAddress", xAddress)
.add("isTest", isTest)
.toString();
}
/**
* Creates an immutable copy of a {@link Wallet} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Wallet instance
*/
public static ImmutableWallet copyOf(Wallet instance) {
if (instance instanceof ImmutableWallet) {
return (ImmutableWallet) instance;
}
return ImmutableWallet.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableWallet ImmutableWallet}.
*
* ImmutableWallet.builder()
* .privateKey(String) // optional {@link Wallet#privateKey() privateKey}
* .publicKey(String) // required {@link Wallet#publicKey() publicKey}
* .classicAddress(org.xrpl.xrpl4j.model.transactions.Address) // required {@link Wallet#classicAddress() classicAddress}
* .xAddress(org.xrpl.xrpl4j.model.transactions.XAddress) // required {@link Wallet#xAddress() xAddress}
* .isTest(boolean) // required {@link Wallet#isTest() isTest}
* .build();
*
* @return A new ImmutableWallet builder
*/
public static ImmutableWallet.Builder builder() {
return new ImmutableWallet.Builder();
}
/**
* Builds instances of type {@link ImmutableWallet ImmutableWallet}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "Wallet", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_PUBLIC_KEY = 0x1L;
private static final long INIT_BIT_CLASSIC_ADDRESS = 0x2L;
private static final long INIT_BIT_X_ADDRESS = 0x4L;
private static final long INIT_BIT_IS_TEST = 0x8L;
private long initBits = 0xfL;
private @Nullable String privateKey;
private @Nullable String publicKey;
private @Nullable Address classicAddress;
private @Nullable XAddress xAddress;
private boolean isTest;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Wallet} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Wallet instance) {
Objects.requireNonNull(instance, "instance");
Optional privateKeyOptional = instance.privateKey();
if (privateKeyOptional.isPresent()) {
privateKey(privateKeyOptional);
}
publicKey(instance.publicKey());
classicAddress(instance.classicAddress());
xAddress(instance.xAddress());
isTest(instance.isTest());
return this;
}
/**
* Initializes the optional value {@link Wallet#privateKey() privateKey} to privateKey.
* @param privateKey The value for privateKey
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
@Deprecated
public final Builder privateKey(String privateKey) {
this.privateKey = Objects.requireNonNull(privateKey, "privateKey");
return this;
}
/**
* Initializes the optional value {@link Wallet#privateKey() privateKey} to privateKey.
* @param privateKey The value for privateKey
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@Deprecated
public final Builder privateKey(Optional privateKey) {
this.privateKey = privateKey.orElse(null);
return this;
}
/**
* Initializes the value for the {@link Wallet#publicKey() publicKey} attribute.
* @param publicKey The value for publicKey
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder publicKey(String publicKey) {
this.publicKey = Objects.requireNonNull(publicKey, "publicKey");
initBits &= ~INIT_BIT_PUBLIC_KEY;
return this;
}
/**
* Initializes the value for the {@link Wallet#classicAddress() classicAddress} attribute.
* @param classicAddress The value for classicAddress
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder classicAddress(Address classicAddress) {
this.classicAddress = Objects.requireNonNull(classicAddress, "classicAddress");
initBits &= ~INIT_BIT_CLASSIC_ADDRESS;
return this;
}
/**
* Initializes the value for the {@link Wallet#xAddress() xAddress} attribute.
* @param xAddress The value for xAddress
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder xAddress(XAddress xAddress) {
this.xAddress = Objects.requireNonNull(xAddress, "xAddress");
initBits &= ~INIT_BIT_X_ADDRESS;
return this;
}
/**
* Initializes the value for the {@link Wallet#isTest() isTest} attribute.
* @param isTest The value for isTest
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder isTest(boolean isTest) {
this.isTest = isTest;
initBits &= ~INIT_BIT_IS_TEST;
return this;
}
/**
* Builds a new {@link ImmutableWallet ImmutableWallet}.
* @return An immutable instance of Wallet
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableWallet build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableWallet(privateKey, publicKey, classicAddress, xAddress, isTest);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_PUBLIC_KEY) != 0) attributes.add("publicKey");
if ((initBits & INIT_BIT_CLASSIC_ADDRESS) != 0) attributes.add("classicAddress");
if ((initBits & INIT_BIT_X_ADDRESS) != 0) attributes.add("xAddress");
if ((initBits & INIT_BIT_IS_TEST) != 0) attributes.add("isTest");
return "Cannot build Wallet, some of required attributes are not set " + attributes;
}
}
}