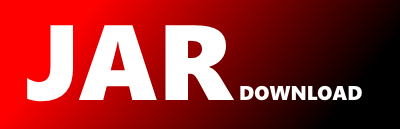
org.xsocket.connection.http.PlainBodyDataSink Maven / Gradle / Ivy
/*
* Copyright (c) xsocket.org, 2006 - 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xsocket.org/
*/
package org.xsocket.connection.http;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.xsocket.ClosedException;
import org.xsocket.MaxWriteSizeExceededException;
import org.xsocket.connection.INonBlockingConnection;
import org.xsocket.connection.IConnection.FlushMode;
/**
*
* plain implementation of a body data sink
*
* @author [email protected]
*/
final class PlainBodyDataSink extends BodyDataSink {
private static final Logger LOG = Logger.getLogger(PlainBodyDataSink.class.getName());
private AbstractHttpConnection httpConnection = null;
private INonBlockingConnection tcpConnection = null;
private AbstractMessageHeader header = null;
private boolean isHeaderWritten = false;
private boolean isCloseHttpConnectionAfterWritten = false;
private int contentLength = 0;
private int written = 0;
/**
* constructor
*
* @param httpConnection the http connection
* @param header the message header
* @param encoding the encoding to use
* @throws IOException if the content length has not be set
*/
public PlainBodyDataSink(AbstractHttpConnection httpConnection, AbstractMessageHeader header, String encoding) throws IOException {
this.httpConnection = httpConnection;
this.tcpConnection = httpConnection.getUnderlyingConnection();
this.header = header;
setEncoding(encoding);
if (header.getContentLength() < 0) {
throw new IOException("Content Length has to be set");
}
contentLength = header.getContentLength();
}
/**
* {@inheritDoc}
*/
void setCloseHttpConnectionAfterWritten(boolean isCloseHttpConnectionAfterWritten) {
this.isCloseHttpConnectionAfterWritten = isCloseHttpConnectionAfterWritten;
}
/**
* {@inheritDoc}
*/
@Override
protected void onWriteDataInserted() throws IOException, ClosedException {
if (isAutoflush()) {
flush();
}
}
/**
* {@inheritDoc}
*/
public void flush() throws IOException {
removeWriteMark();
int dataWritten = 0;
if (!isHeaderWritten) {
isHeaderWritten = true;
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("[" + httpConnection.getId() + "] sending " + header.toString());
}
dataWritten = header.writeTo(tcpConnection);
dataWritten += writeBody();
} else {
dataWritten = writeBody();
}
// flushing
if (dataWritten > 0) {
try {
tcpConnection.setFlushmode(this.getFlushmode());
tcpConnection.flush();
} finally {
tcpConnection.setFlushmode(FlushMode.ASYNC);
}
}
}
private int writeBody() throws IOException {
int dataWritten = 0;
ByteBuffer[] body = drainWriteQueue();
if (body != null) {
dataWritten = (int) tcpConnection.write(body);
written += dataWritten;
}
if (written > contentLength) {
throw new MaxWriteSizeExceededException();
}
return dataWritten;
}
/**
* {@inheritDoc}
*/
@Override
public void close() throws IOException {
flush();
super.close();
if (isCloseHttpConnectionAfterWritten) {
httpConnection.close();
}
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return printWriteBuffer(getEncoding());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy