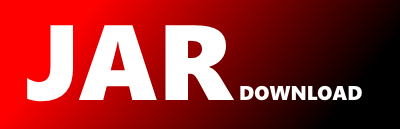
org.xsocket.connection.http.Request Maven / Gradle / Ivy
/*
* Copyright (c) xsocket.org, 2006 - 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xsocket.org/
*/
package org.xsocket.connection.http;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.InetSocketAddress;
import java.nio.channels.ReadableByteChannel;
import java.util.Map;
import java.util.Set;
/**
* http request representation
*
* @author [email protected]
*/
public class Request extends AbstractMessage {
private RequestHeader requestHeader = null;
/**
* constructor
*
* @param requestHeader the request header
*/
public Request(RequestHeader requestHeader) {
this.requestHeader = requestHeader;
}
/**
* constructor
*
* @param requestHeader the request header
* @param bodyDataSource the body data source
*/
public Request(RequestHeader requestHeader, NonBlockingBodyDataSource bodyDataSource) {
this.requestHeader = requestHeader;
setBodyDataSource(bodyDataSource);
}
/**
* constructor
*
* @param method the method (GET, POST, ...)
* @param uri the uri
* @param contentType the content type
* @param body the body
*/
public Request(String method, String uri, String contentType, String body) {
requestHeader = new RequestHeader(method, uri, contentType);
try {
setBodyDataSource(body, requestHeader.getCharacterEncoding());
} catch (UnsupportedEncodingException use) {
throw new RuntimeException(use.toString());
}
}
/**
* constructor
*
* @param method the method (GET, POST, ...)
* @param uri the uri
* @param contentType the content type
* @param body the body
* @throws IOException if an exception occurs by reading the body
*/
public Request(String method, String uri, String contentType, ReadableByteChannel body) throws IOException {
if (contentType != null) {
requestHeader = new RequestHeader(method, uri, contentType);
} else {
requestHeader = new RequestHeader(method, uri);
}
NonBlockingBodyDataSource dataSource = new NonBlockingBodyDataSource(body, requestHeader.getCharacterEncoding());
if (dataSource.available() > 0) {
setBodyDataSource(dataSource);
}
}
/**
* returns the request header
*
* @return the request header
*/
public RequestHeader getRequestHeader() {
return requestHeader;
}
/**
* set the method
*
* @param method the method
*/
public void setMethod(String method) {
requestHeader.setMethod(method);
}
/**
* returns the method
*
* @return the method
*/
public String getMethod() {
return requestHeader.getMethod();
}
/**
* returns the connection header
*
* @return the connection header
*/
public String getConnection() {
return requestHeader.getConnection();
}
/**
* returns the keep alive header
*
* @return the keep alive header
*/
public String getKeepAlive() {
return requestHeader.getKeepAlive();
}
/**
* returns the scheme
*
* @return the scheme
*/
public String getScheme() {
return requestHeader.getScheme();
}
/**
* sets the scheme
*
* @param scheme the scheme
*/
public void setScheme(String scheme) {
requestHeader.setScheme(scheme);
}
/**
* returns the protocol
*
* @return the protocol
*/
public String getProtocol() {
return requestHeader.getProtocol();
}
/**
* returns the host header
*
* @return the host header
*/
public String getHost() {
return requestHeader.getHost();
}
/**
* sets the host header
*
* @param host the host header
*/
public void setHost(String host) {
requestHeader.setHost(host);
}
/**
* sets the remote address (updates the uri)
*
* @param address the remote address
*/
public void setRemoteSocketAddress(InetSocketAddress address) {
requestHeader.setRemoteSocketAddress(address);
}
/**
* returns the remote host
*
* @return the remote host
*/
public String getRemoteHost() {
return requestHeader.getRemoteHost();
}
/**
* returns the remote port
*
* @return the remote port
*/
public int getRemotePort() {
return requestHeader.getRemotePort();
}
/**
* returns the request uri
*
* @return the request uri
*/
public String getRequestURI() {
return requestHeader.getRequestURI();
}
/**
* returns the full request uri
*
* @return the full request uri
*/
public String getFullRequestURI() {
return requestHeader.getFullRequestURI();
}
/**
* returns the query string
*
* @return the query string
*/
public String getQueryString() {
return requestHeader.getQueryString();
}
/**
* returns true if is secured
*
* @return true, if is secured
*/
public boolean isSecure() {
return requestHeader.isSecure();
}
/**
* returns the parameter map
*
* @return the parameter map
*/
@SuppressWarnings("unchecked")
public Map getParameterMap() {
return requestHeader.getParameterMap();
}
/**
* returns the parameter name set
*
* @return the parameter name set
*/
public Set getParameterNameSet() {
return requestHeader.getParameterNameSet();
}
/**
* gets a parameter
*
* @param name the parameter name
* @return the parameter value or null
*/
public String getParameter(String name) {
return requestHeader.getParameter(name);
}
/**
* sets a parameter
*
* @param parameterName the parameter name
* @param parameterValue the parameter value
*/
public void setParameter(String parameterName, String parameterValue) {
requestHeader.setParameter(parameterName, parameterValue);
}
/**
* {@inheritDoc}
*/
@Override
AbstractMessageHeader getMessageHeader() {
return getRequestHeader();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy