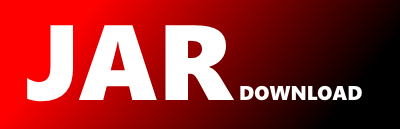
org.xsocket.connection.http.client.ClientUtils Maven / Gradle / Ivy
/*
* Copyright (c) xsocket.org, 2006 - 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xsocket.org/
*/
package org.xsocket.connection.http.client;
import java.io.IOException;
import java.lang.reflect.Method;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.xsocket.Execution;
import org.xsocket.connection.http.HttpUtils;
import org.xsocket.connection.http.IBodyHandler;
import org.xsocket.connection.http.IHttpConnectHandler;
import org.xsocket.connection.http.IHttpConnection;
import org.xsocket.connection.http.IHttpConnectionTimeoutHandler;
import org.xsocket.connection.http.IHttpDisconnectHandler;
import org.xsocket.connection.http.IHttpHandler;
import org.xsocket.connection.http.InvokeOn;
import org.xsocket.connection.http.NonBlockingBodyDataSource;
import org.xsocket.connection.http.Response;
import org.xsocket.connection.http.client.IResponseHandler;
import org.xsocket.connection.http.server.IRequestHandler;
/**
* A utility class
*
* @author [email protected]
*/
final class ClientUtils {
private static final Logger LOG = Logger.getLogger(ClientUtils.class.getName());
@SuppressWarnings("unchecked")
private static final Map bodyExecutionModeCache = HttpUtils.newMapCache(25);
@SuppressWarnings("unchecked")
private static final Map responseHandlerInfoCache = HttpUtils.newMapCache(25);
static final ResponseHandlerInfo RESPONSE_HANDLER_INFO_NONTHREADED_HEADER_RECEIVED = new ResponseHandlerInfo(InvokeOn.Mode.HEADER_RECEIVED, Execution.Mode.NONTHREADED);
static final ResponseHandlerInfo RESPONSE_HANDLER_INFO_NONTHREADED_MESSAGE_RECEIVED = new ResponseHandlerInfo(InvokeOn.Mode.MESSAGE_RECEIVED, Execution.Mode.NONTHREADED);
@SuppressWarnings("unchecked")
private static final Map httpHandlerInfoCache = HttpUtils.newMapCache(25);
public static final HttpHandlerInfo EMPTY_HTTP_HANDLER_INFO = new HttpHandlerInfo(null);
static Execution.Mode getExecutionMode(IBodyHandler bodyHandler) {
Execution.Mode mode = bodyExecutionModeCache.get(bodyHandler.getClass());
if (mode == null) {
mode = IBodyHandler.DEFAULT_EXECUTION_MODE;
Execution execution = bodyHandler.getClass().getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
try {
Method meth = bodyHandler.getClass().getMethod("onData", new Class[] { NonBlockingBodyDataSource.class });
execution = meth.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
} catch (NoSuchMethodException nsme) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("shouldn't occure because body handler has to have such a method " + nsme.toString());
}
}
}
return mode;
}
@SuppressWarnings("unchecked")
static ResponseHandlerInfo getResponseHandlerInfo(IResponseHandler responseHandler) throws IOException {
if (responseHandler == null) {
throw new IOException("response handler has to be set");
}
ResponseHandlerInfo responseHandlerInfo = responseHandlerInfoCache.get(responseHandler.getClass());
if (responseHandlerInfo == null) {
responseHandlerInfo = new ResponseHandlerInfo((Class) responseHandler.getClass());
responseHandlerInfoCache.put(responseHandler.getClass(), responseHandlerInfo);
}
return responseHandlerInfo;
}
@SuppressWarnings("unchecked")
static HttpHandlerInfo getHttpHandlerInfo(IHttpHandler httpHandler) {
if (httpHandler == null) {
return EMPTY_HTTP_HANDLER_INFO;
}
HttpHandlerInfo httpHandlerInfo = httpHandlerInfoCache.get(httpHandler.getClass());
if (httpHandlerInfo == null) {
httpHandlerInfo = new HttpHandlerInfo((Class) httpHandler.getClass());
httpHandlerInfoCache.put(httpHandler.getClass(), httpHandlerInfo);
}
return httpHandlerInfo;
}
static final class ResponseHandlerInfo {
private boolean isResponseHandler = false;
private boolean isResponseTimeoutHandler = false;
private InvokeOn.Mode invokationMode = null;
private Execution.Mode onResponseExecutionMode = null;
private Execution.Mode onResponseTimeoutExecutionMode = null;
public ResponseHandlerInfo(Class responseHandlerClass) {
Execution.Mode em = introspectClassLevelExecutionMode(responseHandlerClass);
onResponseExecutionMode = em;
onResponseTimeoutExecutionMode = em;
if (IResponseHandler.class.isAssignableFrom(responseHandlerClass)) {
isResponseHandler = true;
onResponseExecutionMode = introspectOnResponseExecutionMode(responseHandlerClass, onResponseExecutionMode);
}
if (IResponseTimeoutHandler.class.isAssignableFrom(responseHandlerClass)) {
isResponseTimeoutHandler = true;
onResponseTimeoutExecutionMode = introspectOnResponseTimeoutExecutionMode(responseHandlerClass, onResponseTimeoutExecutionMode);
}
invokationMode = introspectInvokationMode(responseHandlerClass);
}
ResponseHandlerInfo(InvokeOn.Mode invokationMode, Execution.Mode executionMode) {
isResponseHandler = true;
isResponseTimeoutHandler = true;
this.invokationMode = invokationMode;
this.onResponseExecutionMode = executionMode;
this.onResponseTimeoutExecutionMode = executionMode;
}
static Execution.Mode introspectClassLevelExecutionMode(Class responseHandlerClass) {
Execution.Mode mode = IResponseHandler.DEFAULT_EXECUTION_MODE;
Execution execution = responseHandlerClass.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
return mode;
}
static Execution.Mode introspectOnResponseExecutionMode(Class responseHandlerClass, Execution.Mode defaultMode) {
Execution.Mode mode = defaultMode;
try {
Method meth = responseHandlerClass.getMethod("onResponse", new Class[] { Response.class });
Execution execution = meth.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
} catch (NoSuchMethodException nsme) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("shouldn't occure because body handler has to have such a method " + nsme.toString());
}
}
return mode;
}
static Execution.Mode introspectOnResponseTimeoutExecutionMode(Class responseHandlerClass, Execution.Mode defaultMode) {
Execution.Mode mode = defaultMode;
try {
Method meth = responseHandlerClass.getMethod("onResponseTimeout", new Class[] { });
Execution execution = meth.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
} catch (NoSuchMethodException nsme) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("shouldn't occure because body handler has to have such a method " + nsme.toString());
}
}
return mode;
}
static InvokeOn.Mode introspectInvokationMode(Class responseHandlerClass) {
InvokeOn.Mode mode = IResponseHandler.DEFAULT_INVOKE_ON_MODE;
InvokeOn invokeOn = responseHandlerClass.getAnnotation(InvokeOn.class);
if (invokeOn != null) {
mode = invokeOn.value();
}
try {
Method meth = responseHandlerClass.getMethod("onResponse", new Class[] { Response.class });
invokeOn = meth.getAnnotation(InvokeOn.class);
if (invokeOn != null) {
mode = invokeOn.value();
}
} catch (NoSuchMethodException nsme) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("shouldn't occure because response handler has to have such a method " + nsme.toString());
}
}
return mode;
}
public boolean isResponseHandler() {
return isResponseHandler;
}
public boolean isResponseTimeuotHandler() {
return isResponseTimeoutHandler;
}
public InvokeOn.Mode getInvokationMode() {
return invokationMode;
}
public Execution.Mode getOnResponseExecutionMode() {
return onResponseExecutionMode;
}
public Execution.Mode getOnResponseTimeoutExecutionMode() {
return onResponseTimeoutExecutionMode;
}
}
static final class HttpHandlerInfo {
private boolean isConnectHandler = false;
private boolean isConnectHandlerMultithreaded = true;
private boolean isDisconnectHandler = false;
private boolean isDisconnectHandlerMultithreaded = true;
private boolean isConnectionTimoutHandler = false;
private boolean isConnectionTimoutHandlerMultithreaded = true;
@SuppressWarnings("unchecked")
public HttpHandlerInfo(Class clazz) {
if (clazz == null) {
return;
}
if (IHttpConnectHandler.class.isAssignableFrom(clazz)) {
isConnectHandler = true;
isConnectHandlerMultithreaded = (introspectOnConnectExecutionMode(clazz) == Execution.Mode.MULTITHREADED);
}
if (IHttpDisconnectHandler.class.isAssignableFrom(clazz)) {
isDisconnectHandler = true;
isDisconnectHandlerMultithreaded = (introspectOnDisconnectExecutionMode(clazz) == Execution.Mode.MULTITHREADED);
}
if (IHttpConnectionTimeoutHandler.class.isAssignableFrom(clazz)) {
isConnectionTimoutHandler = true;
isConnectionTimoutHandlerMultithreaded = (introspectOnConnectionTimeoutExecutionMode(clazz) == Execution.Mode.MULTITHREADED);
}
}
static Execution.Mode introspectOnConnectExecutionMode(Class serverHandlerClass) {
Execution.Mode mode = IRequestHandler.DEFAULT_EXECUTION_MODE;
Execution execution = serverHandlerClass.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
try {
Method meth = serverHandlerClass.getMethod("onConnect", new Class[] { IHttpConnection.class });
execution = meth.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
} catch (NoSuchMethodException nsme) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("shouldn't occure because body handler has to have such a method " + nsme.toString());
}
}
return mode;
}
static Execution.Mode introspectOnDisconnectExecutionMode(Class serverHandlerClass) {
Execution.Mode mode = IRequestHandler.DEFAULT_EXECUTION_MODE;
Execution execution = serverHandlerClass.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
try {
Method meth = serverHandlerClass.getMethod("onDisconnect", new Class[] { IHttpConnection.class });
execution = meth.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
} catch (NoSuchMethodException nsme) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("shouldn't occure because body handler has to have such a method " + nsme.toString());
}
}
return mode;
}
static Execution.Mode introspectOnIdleTimeoutExecutionMode(Class serverHandlerClass) {
Execution.Mode mode = IRequestHandler.DEFAULT_EXECUTION_MODE;
Execution execution = serverHandlerClass.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
try {
Method meth = serverHandlerClass.getMethod("onIdleTimeout", new Class[] { IHttpConnection.class });
execution = meth.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
} catch (NoSuchMethodException nsme) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("shouldn't occure because body handler has to have such a method " + nsme.toString());
}
}
return mode;
}
static Execution.Mode introspectOnConnectionTimeoutExecutionMode(Class serverHandlerClass) {
Execution.Mode mode = IRequestHandler.DEFAULT_EXECUTION_MODE;
Execution execution = serverHandlerClass.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
try {
Method meth = serverHandlerClass.getMethod("onConnectionTimeout", new Class[] { IHttpConnection.class });
execution = meth.getAnnotation(Execution.class);
if (execution != null) {
mode = execution.value();
}
} catch (NoSuchMethodException nsme) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("shouldn't occure because body handler has to have such a method " + nsme.toString());
}
}
return mode;
}
public boolean isConnectHandler() {
return isConnectHandler;
}
public boolean isConnectHandlerMultithreaded() {
return isConnectHandlerMultithreaded;
}
public boolean isDisconnectHandler() {
return isDisconnectHandler;
}
public boolean isDisconnectHandlerMultithreaded() {
return isDisconnectHandlerMultithreaded;
}
public boolean isConnectionTimeoutHandler() {
return isConnectionTimoutHandler;
}
public boolean isConnectionTimeoutHandlerMultithreaded() {
return isConnectionTimoutHandlerMultithreaded;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy