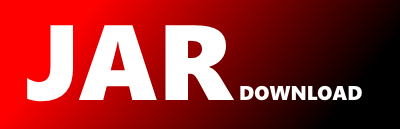
org.xsocket.connection.http.server.HttpProtocolAdapter Maven / Gradle / Ivy
/*
* Copyright (c) xsocket.org, 2006 - 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xsocket.org/
*/
package org.xsocket.connection.http.server;
import java.io.IOException;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.xsocket.Execution;
import org.xsocket.ILifeCycle;
import org.xsocket.Resource;
import org.xsocket.connection.IConnectHandler;
import org.xsocket.connection.IConnectionScoped;
import org.xsocket.connection.IHandlerAdapter;
import org.xsocket.connection.INonBlockingConnection;
import org.xsocket.connection.IServer;
import org.xsocket.connection.http.IHttpConnectHandler;
import org.xsocket.connection.http.IHttpConnectionTimeoutHandler;
import org.xsocket.connection.http.IHttpDisconnectHandler;
import org.xsocket.connection.http.IHttpHandler;
import org.xsocket.connection.http.server.ServerUtils.HttpHandlerInfo;
import org.xsocket.connection.http.server.ServerUtils.ServerHandlerInfo;
/**
* The http protocol adapter. The protocol adapter implements the {@link IConnectHandler} interface, and
* "maps" each incoming connection into a {@link HttpServerConnection}.
* HttpProtcol adapter is required, if the {@link HttpServer} will not be used.
*
* Example:
*
*
* // establishing a http server based on a mutliplexed tcp connection
* // for multiplexex connections see http://xsocket.sourceforge.net/multiplexed/tutorial/V2/TutorialMultiplexed.htm
*
* IRequestHandler hdl = new MyRequestHandler();
* IConnectHandler httpAdapter = new HttpProtocolAdapter(hdl);
*
*
* IServer mutliplexedHttpServer = new Server(port, new MultiplexedProtocolAdapter(httpAdapter)));
* ConnectionUtils.start(mutliplexedHttpServer);
* ...
*
*
*
* @author grro
*/
@Execution(Execution.Mode.NONTHREADED)
public final class HttpProtocolAdapter implements IConnectHandler, ILifeCycle, IHandlerAdapter {
private static final Logger LOG = Logger.getLogger(HttpProtocolAdapter.class.getName());
@Resource
private IServer server = null;
private IHttpHandler handler = null;
private ServerHandlerInfo serverHandlerInfo = null;
private HttpHandlerInfo httpHandlerInfo = null;
private boolean isConnectionScoped = false;
private boolean isLifeCycle = false;
private int requestTimeoutMillis = Integer.MAX_VALUE;
private boolean isCloseOnSendingError = false;
/**
* constructor
*
* @param handler the handler (supported: {@link IRequestHandler}, {@link IHttpConnectHandler}, {@link IHttpDisconnectHandler}, {@link IHttpConnectionTimeoutHandler}, {@link ILifeCycle})
*/
public HttpProtocolAdapter(IHttpHandler handler) {
this.handler = handler;
this.serverHandlerInfo = ServerUtils.getServerHandlerInfo(handler);
this.httpHandlerInfo = ServerUtils.getHttpHandlerInfo(handler);
this.isConnectionScoped = (handler instanceof IConnectionScoped);
this.isLifeCycle = (handler instanceof ILifeCycle);
}
/*
public void setRequestTimeoutMillis(int requestTimeoutMillis) {
this.requestTimeoutMillis = requestTimeoutMillis;
}
public int getRequestTimeoutMillis() {
return requestTimeoutMillis;
}
*/
/**
* set is if the server-side connection will closed, if an error message is sent
*
* @param isCloseOnSendingError if the connection will closed, if an error message is sent
*/
public void setCloseOnSendingError(boolean isCloseOnSendingError) {
this.isCloseOnSendingError = isCloseOnSendingError;
}
/**
* returns if the server-side connection will closed, if an error message will be sent
* @return true, if the connection will closed by sending an error message
*/
public boolean isCloseOnSendingError() {
return isCloseOnSendingError;
}
/**
* {@inheritDoc}
*/
public Object getAdaptee() {
return handler;
}
/**
* {@inheritDoc}
*/
public void onInit() {
server.setStartUpLogMessage(server.getStartUpLogMessage() + "; http " + ServerUtils.getVersionInfo());
ServerUtils.injectServerField(handler, server);
ServerUtils.injectProtocolAdapter(handler, this);
if (isLifeCycle) {
((ILifeCycle) handler).onInit();
}
}
/**
* {@inheritDoc}
*/
public void onDestroy() {
if (isLifeCycle) {
try {
((ILifeCycle) handler).onDestroy();
} catch (IOException ioe) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("exception occured by destroying " + handler + " " + ioe.toString());
}
}
}
}
/**
* {@inheritDoc}
*/
public boolean onConnect(INonBlockingConnection connection) throws IOException {
if (isConnectionScoped) {
try {
IRequestHandler handlerCopy = (IRequestHandler) ((IConnectionScoped) handler).clone();
new HttpServerConnection(connection, serverHandlerInfo, httpHandlerInfo, handlerCopy, requestTimeoutMillis, isCloseOnSendingError);
} catch (CloneNotSupportedException clne) {
throw new RuntimeException("couldn't clone handler " + handler + " " + clne.toString());
}
} else {
new HttpServerConnection(connection, serverHandlerInfo, httpHandlerInfo, handler, requestTimeoutMillis, isCloseOnSendingError);
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy