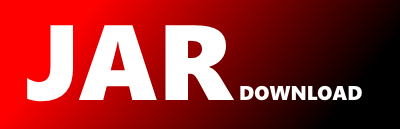
org.xsocket.connection.http.client.PostRequest Maven / Gradle / Ivy
/*
* Copyright (c) xsocket.org, 2006 - 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xsocket.org/
*/
package org.xsocket.connection.http.client;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.URISyntaxException;
import java.net.URLEncoder;
import java.nio.channels.FileChannel;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.xsocket.connection.http.HttpRequest;
import org.xsocket.connection.http.HttpRequestHeader;
/**
* POST request
*
* @author [email protected]
*/
public final class PostRequest extends HttpRequest {
private static final Logger LOG = Logger.getLogger(PostRequest.class.getName());
/**
* constructor
*
* @param uri the uri
*/
public PostRequest(String uri) throws URISyntaxException {
super(new HttpRequestHeader("POST", uri));
}
/**
* constructor
*
* @param uri the uri
* @param contentType the content type
* @param dataSource the body
* @throws IOException if an error occurs by reading the body
*/
public PostRequest(String uri, String contentType, FileChannel dataSource) throws IOException, URISyntaxException {
super("POST", uri, contentType, dataSource);
}
/**
* constructor
*
* @param uri the uri
* @param contentType the content type
* @param body the body
*/
public PostRequest(String uri, String contentType, String body) throws IOException, URISyntaxException {
super("POST", uri, contentType, body);
}
/**
* constructor
*
* @param uri the uri
* @param formParameters the FORM parameter
*/
public PostRequest(String uri, String[] formParameters) throws IOException, URISyntaxException {
super(new HttpRequestHeader("POST", uri));
setContentType("application/x-www-form-urlencoded; charset=utf-8");
StringBuilder sb = new StringBuilder();
for (String param : formParameters) {
try {
String[] kvp = param.split("=");
sb.append(URLEncoder.encode(kvp[0], "UTF-8") + "=" + URLEncoder.encode(kvp[1], "UTF-8") + "&");
} catch (UnsupportedEncodingException usec) {
if (LOG.isLoggable(Level.FINE)) {
LOG.fine("error occured by encoding param " + param + " " + usec.toString());
}
}
}
String body = sb.toString();
if (body.length() > 0) {
body = body.substring(0, body.length() - 1);
}
setBodyDataSource(body);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy