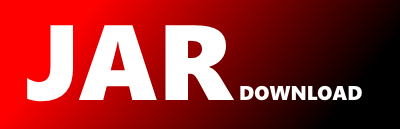
org.xsocket.connection.http.HttpRequest Maven / Gradle / Ivy
/*
* Copyright (c) xsocket.org, 2006 - 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xsocket.org/
*/
package org.xsocket.connection.http;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.MalformedURLException;
import java.net.URL;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.channels.ReadableByteChannel;
import java.util.Map;
import java.util.Set;
/**
* http request representation
*
* @author [email protected]
*/
public class HttpRequest extends AbstractHttpMessage {
private HttpRequestHeader requestHeader = null;
private String requestHandlerPath = "";
private String contextPath = "";
/**
* constructor
*
* @param requestHeader the request header
*/
public HttpRequest(HttpRequestHeader requestHeader) {
this.requestHeader = requestHeader;
}
/**
* constructor
*
* @param requestHeader the request header
* @param bodyDataSource the body data source
*/
public HttpRequest(HttpRequestHeader requestHeader, NonBlockingBodyDataSource bodyDataSource) {
this.requestHeader = requestHeader;
setBodyDataSource(bodyDataSource);
}
/**
* constructor
*
* @param method the method (GET, POST, ...)
* @param url the url
* @param contentType the content type
* @param body the body
*/
public HttpRequest(String method, String url, String contentType, String body) throws IOException, MalformedURLException {
requestHeader = new HttpRequestHeader(method, url, contentType);
try {
setBodyDataSource(body, requestHeader.getCharacterEncoding());
} catch (UnsupportedEncodingException use) {
throw new RuntimeException(use.toString());
}
}
/**
* constructor
*
* @param method the method (GET, POST, ...)
* @param url the url
* @param contentType the content type
* @param body the body
*/
public HttpRequest(String method, String url, String contentType, byte[] body) throws IOException, MalformedURLException {
requestHeader = new HttpRequestHeader(method, url, contentType);
try {
setBodyDataSource(new ByteBuffer[] { ByteBuffer.wrap(body) }, requestHeader.getCharacterEncoding());
} catch (UnsupportedEncodingException use) {
throw new RuntimeException(use.toString());
}
}
/**
* constructor
*
* @param method the method (GET, POST, ...)
* @param url the url
* @param contentType the content type
* @param body the body
*/
public HttpRequest(String method, String url, String contentType, ByteBuffer[] body) throws IOException, MalformedURLException {
requestHeader = new HttpRequestHeader(method, url, contentType);
try {
setBodyDataSource(body, requestHeader.getCharacterEncoding());
} catch (UnsupportedEncodingException use) {
throw new RuntimeException(use.toString());
}
}
/**
* constructor
*
* @param method the method (GET, POST, ...)
* @param url the url
*/
public HttpRequest(String method, String url) throws MalformedURLException {
requestHeader = new HttpRequestHeader(method, url);
}
/**
* constructor
*
* @param method the method (GET, POST, ...)
* @param url the url
* @param contentType the content type
* @param body the body
* @throws IOException if an exception occurs by reading the body
*/
public HttpRequest(String method, String url, String contentType, FileChannel body) throws IOException, MalformedURLException {
if (contentType != null) {
requestHeader = new HttpRequestHeader(method, url, contentType);
} else {
requestHeader = new HttpRequestHeader(method, url);
}
requestHeader.setContentLength((int) body.size());
setBodyDataSource(body);
}
/**
* constructor
*
* @param method the method (GET, POST, ...)
* @param url the url
* @param contentType the content type
* @param body the body
* @throws IOException if an exception occurs by reading the body
*/
public HttpRequest(String method, String url, String contentType, ReadableByteChannel body) throws IOException, MalformedURLException{
if (contentType != null) {
requestHeader = new HttpRequestHeader(method, url, contentType);
} else {
requestHeader = new HttpRequestHeader(method, url);
}
setBodyDataSource(body);
}
/**
* constructor
*
* @param method the method (GET, POST, ...)
* @param url the url
* @param contentType the content type
* @param contentLength the content length
* @param body the body
* @throws IOException if an exception occurs by reading the body
*/
public HttpRequest(String method, String url, String contentType, int contentLength, ReadableByteChannel body) throws IOException, MalformedURLException {
if (contentType != null) {
requestHeader = new HttpRequestHeader(method, url, contentType);
} else {
requestHeader = new HttpRequestHeader(method, url);
}
requestHeader.setContentLength(contentLength);
setBodyDataSource(body);
}
/**
* returns the request header
*
* @return the request header
*/
public HttpRequestHeader getRequestHeader() {
return requestHeader;
}
public String getRequestHandlerPath() {
return requestHandlerPath;
}
public void setRequestHandlerPath(String requestHandlerPath) {
this.requestHandlerPath = requestHandlerPath;
}
public String getContextPath() {
return contextPath;
}
public void setContextPath(String contextPath) {
this.contextPath = contextPath;
}
/**
* Sets the name of the HTTP method
*
* @param method a String specifying the name of the method
*/
public void setMethod(String method) {
requestHeader.setMethod(method);
}
/**
* Returns the name of the HTTP method with
* which this request was made, for example, GET, POST, or PUT.
*
* @return a String specifying the name of the method
*/
public String getMethod() {
return requestHeader.getMethod();
}
/**
* returns the scheme
*
* @return the scheme
*/
public String getScheme() {
return requestHeader.getScheme();
}
/**
* sets the scheme
*
* @param scheme the scheme
*/
public void setScheme(String scheme) {
requestHeader.setScheme(scheme);
}
/**
* Returns the host name of the server to which the request was sent.
* It is the value of the part before ":" in the Host header value,
* if any, or the resolved server name, or the server IP address.
*
* @return the server name
*/
public String getServerName() {
return requestHeader.getServerName();
}
/**
* Returns the port number to which the request was sent. It is the
* value of the part after ":" in the Host header value, if any,
* or the server port where the client connection was accepted on.
*
* @return the server port
*/
public int getServerPort() {
return requestHeader.getServerPort();
}
/**
* Returns the name and version of the protocol the request
* uses in the form protocol/majorVersion.minorVersion, for example, HTTP/1.1.
*
* @return a String containing the protocol name and version number
*/
public String getProtocol() {
return requestHeader.getProtocol();
}
/**
* returns the target request url
*
* @return the target request url
*/
public URL getTargetURL() {
return requestHeader.getTargetURL();
}
/**
* set the target request url
*
* @param url the target request url
*/
public void setTargetURL(URL url) {
requestHeader.setTargetURL(url);
}
/**
* updates the target url
*
* @param host the host
* @param port the port
*/
public void updateTargetURL(String host, int port) {
requestHeader.updateTargetURL(host, port);
}
/**
* Returns the fully qualified name of the client
* or the last proxy that sent the request. If the
* engine cannot or chooses not to resolve the hostname
* (to improve performance), this method returns the
* dotted-string form of the IP address.
*
* @return a String containing the fully qualified name of the client
*/
public String getRemoteHost() {
return requestHeader.getRemoteHost();
}
/**
* Returns the Internet Protocol (IP) source
* port of the client or last proxy that sent the request.
*
* @return an integer specifying the port number
*/
public int getRemotePort() {
return requestHeader.getRemotePort();
}
/**
* Returns the part of this request's URL from the protocol
* name up to the query string in the first line of the HTTP request.
*
* @return a String containing the part of the URL from the protocol
* name up to the query string
*/
public String getRequestURI() {
return requestHeader.getRequestURI();
}
/**
* Returns the query string that is contained in the request URL
* after the path. This method returns null
* if the URL does not have a query string.
*
* @return a String containing the query string or null if the URL contains no query string.
*/
public String getQueryString() {
return requestHeader.getQueryString();
}
/**
* Returns a boolean indicating whether this request
* was made using a secure channel, such as HTTPS.
*
* @return a boolean indicating if the request was made using a secure channel
*/
public boolean isSecure() {
return requestHeader.isSecure();
}
/**
* returns the parameter map
*
* @return the parameter map
*/
@SuppressWarnings("unchecked")
public Map getParameterMap() {
return requestHeader.getParameterMap();
}
/**
* returns the parameter name set
*
* @return the parameter name set
*/
public Set getParameterNameSet() {
return requestHeader.getParameterNameSet();
}
/**
* Returns the value of a request parameter as a String, or null
* if the parameter does not exist. Request parameters are extra
* information sent with the request.
*
* @param name a String specifying the name of the parameter
* @return a String representing the single value of the parameter
*/
public String getParameter(String name) {
return requestHeader.getParameter(name);
}
/**
* sets a parameter
*
* @param parameterName the parameter name
* @param parameterValue the parameter value
*/
public void setParameter(String parameterName, String parameterValue) {
requestHeader.setParameter(parameterName, parameterValue);
}
/**
* {@inheritDoc}
*/
@Override
AbstractMessageHeader getMessageHeader() {
return getRequestHeader();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy