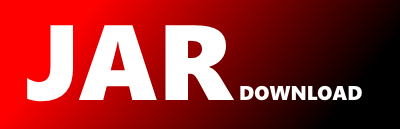
org.xsocket.connection.http.server.HttpServer Maven / Gradle / Ivy
/*
* Copyright (c) xsocket.org, 2006 - 2008. All rights reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Please refer to the LGPL license at: http://www.gnu.org/copyleft/lesser.txt
* The latest copy of this software may be found on http://www.xsocket.org/
*/
package org.xsocket.connection.http.server;
import java.io.IOException;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.UnknownHostException;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Logger;
import javax.net.ssl.SSLContext;
import org.xsocket.connection.Server;
import org.xsocket.connection.IConnection.FlushMode;
import org.xsocket.connection.http.IHttpHandler;
/**
* Http server class. The http server extends the {@link Server} class to be capable to accept
* http request handlers in a direct way. Internally the http server uses the {@link HttpProtocolAdapter}
*
* Example:
*
* IRequestHandler hdl = new MyRequestHandler();
*
* IServer server = new HttpServer(hdl);
*
* server.run();
* // or ConnectionUtils.start(server); (blocks until the server has been started)
* ...
*
*
*
*
*
* @author [email protected]
*/
public class HttpServer extends Server {
private static final Logger LOG = Logger.getLogger(HttpServer.class.getName());
private IHttpHandler handler = null;
/**
* constructor
*
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, ILifeCycle)
* @throws IOException If some other I/O error occurs
* @throws UnknownHostException if the local host cannot determined
*/
public HttpServer(IHttpHandler handler) throws UnknownHostException, IOException {
this(new InetSocketAddress(InetAddress.getByName(DEFAULT_HOST_ADDRESS), 0), new HashMap(), handler, null, false);
}
/**
* constructor
*
*
* @param options the socket options
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IDataHandler, IHttpTimeoutHandler, IHandlerLifeCycleListener)
* @throws IOException If some other I/O error occurs
* @throws UnknownHostException if the local host cannot determined
*/
public HttpServer(Map options, IHttpHandler handler) throws UnknownHostException, IOException {
this(InetAddress.getByName(DEFAULT_HOST_ADDRESS), 0, options, handler, null, false);
}
/**
* constructor
*
*
* @param port the local port
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IHandlerLifeCycleListener)
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port, IHttpHandler handler) throws UnknownHostException, IOException {
this(new InetSocketAddress(InetAddress.getByName(DEFAULT_HOST_ADDRESS), port), new HashMap(), handler, null, false);
}
/**
* constructor
*
*
* @param port the local port
* @param options the acceptor socket options
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IHandlerLifeCycleListener)
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port, Map options, IHttpHandler handler) throws UnknownHostException, IOException {
this(InetAddress.getByName(DEFAULT_HOST_ADDRESS), port, options, handler, null, false);
}
/**
* constructor
*
*
* @param address the local address
* @param port the local port
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IHandlerLifeCycleListener)
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(InetAddress address, int port, IHttpHandler handler) throws UnknownHostException, IOException {
this(address, port, new HashMap(), handler, null, false);
}
/**
* constructor
*
*
* @param ipAddress the local ip address
* @param port the local port
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IHandlerLifeCycleListener)
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(String ipAddress, int port, IHttpHandler handler) throws UnknownHostException, IOException {
this(InetAddress.getByName(ipAddress), port, new HashMap(), handler, null, false);
}
/**
* constructor
*
*
* @param ipAddress the local ip address
* @param port the local port
* @param options the socket options
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IHandlerLifeCycleListener)
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(String ipAddress, int port, Map options, IHttpHandler handler) throws UnknownHostException, IOException {
this(InetAddress.getByName(ipAddress), port, options, handler, null, false);
}
/**
* constructor
*
*
* @param port local port
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IHandlerLifeCycleListener)
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port, IHttpHandler handler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(InetAddress.getByName(DEFAULT_HOST_ADDRESS), port, new HashMap(), handler, sslContext, sslOn);
}
/**
* constructor
*
* @param port local port
* @param options the acceptor socket options
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IHandlerLifeCycleListener)
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(int port,Map options, IHttpHandler handler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(InetAddress.getByName(DEFAULT_HOST_ADDRESS), port, options, handler, sslContext, sslOn);
}
/**
* constructor
*
* @param ipAddress local ip address
* @param port local port
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IConnectionScoped, IHandlerLifeCycleListener)
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(String ipAddress, int port, IHttpHandler handler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(InetAddress.getByName(ipAddress), port, new HashMap(), handler, sslContext, sslOn);
}
/**
* constructor
*
*
* @param ipAddress local ip address
* @param port local port
* @param options the acceptor socket options
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IConnectionScoped, IHandlerLifeCycleListener)
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(String ipAddress, int port, Map options, IHttpHandler handler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(InetAddress.getByName(ipAddress), port, options, handler, sslContext, sslOn);
}
/**
* constructor
*
*
* @param address local address
* @param port local port
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IConnectionScoped, IHandlerLifeCycleListener)
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(InetAddress address, int port, IHttpHandler handler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(address, port, new HashMap(), handler, sslContext, sslOn);
}
/**
* constructor
*
*
* @param address local address
* @param port local port
* @param options the socket options
* @param handler the handler to use (supported: IHttpConnectHandler, IHttpDisconnectHandler, IServerHandler, IHttpTimeoutHandler, IConnectionScoped, IHandlerLifeCycleListener)
* @param sslOn true, is SSL should be activated
* @param sslContext the ssl context to use
* @throws UnknownHostException if the local host cannot determined
* @throws IOException If some other I/O error occurs
*/
public HttpServer(InetAddress address, int port, Map options, IHttpHandler handler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
this(new InetSocketAddress(address, port), options, handler, sslContext, sslOn);
}
private HttpServer(InetSocketAddress address, Map options, IHttpHandler appHandler, SSLContext sslContext, boolean sslOn) throws UnknownHostException, IOException {
super(address, options, new HttpProtocolAdapter(appHandler), sslContext, sslOn, 0);
this.handler = appHandler;
}
/**
* sets the message receive timeout
*
* @param receivetimeout the message receive timeout
*/
public void setRequestTimeoutSec(int receivetimeout) {
((HttpProtocolAdapter) super.getHandler()).setRequestTimeoutSec(receivetimeout);
}
/**
* returns the message receive timeout
*
* @return the message receive timeout
*/
public int getRequestTimeoutSec() {
return ((HttpProtocolAdapter) super.getHandler()).getRequestTimeoutSec();
}
/**
* set is if the server-side connection will closed, if an error message is sent
*
* @param isCloseOnSendingError if the connection will closed, if an error message is sent
*/
public void setCloseOnSendingError(boolean isCloseOnSendingError) {
((HttpProtocolAdapter) super.getHandler()).setCloseOnSendingError(isCloseOnSendingError);
}
/**
* returns if the server-side connection will closed, if an error message will be sent
* @return true, if the connection will closed by sending an error message
*/
public boolean isCloseOnSendingError() {
return ((HttpProtocolAdapter) super.getHandler()).isCloseOnSendingError();
}
/**
* set the max transactions per connection. Setting this filed causes that
* a keep-alive response header will be added
*
* @param maxTransactions the max transactions
*/
public void setMaxTransactions(int maxTransactions) {
((HttpProtocolAdapter) getHandler()).setMaxTransactions(maxTransactions);
}
/**
* this method is not supported
*/
@Override
public void setWriteTransferRate(int bytesPerSecond) throws IOException {
throw new UnsupportedOperationException("setWriteTransferRate is not supported");
}
/**
* returns the http handler
*
* @return http handler
*/
public IHttpHandler getHttpHandler() {
return handler;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy