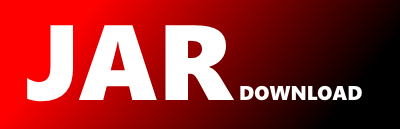
org.xson.web.cache.SoftCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xco-web Show documentation
Show all versions of xco-web Show documentation
xco-web is an easy to use control layer framework, is part of the SOA system, using xml language to describe the controller.
The newest version!
package org.xson.web.cache;
import java.lang.ref.ReferenceQueue;
import java.lang.ref.SoftReference;
import java.util.LinkedList;
public class SoftCache extends AbstractCache {
private final LinkedList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy