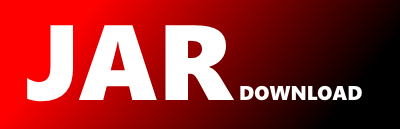
de.itemis.xtend.auto.gwt.UiBinderParser Maven / Gradle / Ivy
The newest version!
/**
* Copyright (c) 2015 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*/
package de.itemis.xtend.auto.gwt;
import com.google.common.base.Objects;
import com.google.common.collect.Iterables;
import com.google.gwt.dom.client.AnchorElement;
import com.google.gwt.dom.client.AreaElement;
import com.google.gwt.dom.client.AudioElement;
import com.google.gwt.dom.client.BRElement;
import com.google.gwt.dom.client.BaseElement;
import com.google.gwt.dom.client.BodyElement;
import com.google.gwt.dom.client.ButtonElement;
import com.google.gwt.dom.client.CanvasElement;
import com.google.gwt.dom.client.DListElement;
import com.google.gwt.dom.client.DivElement;
import com.google.gwt.dom.client.FieldSetElement;
import com.google.gwt.dom.client.FormElement;
import com.google.gwt.dom.client.FrameElement;
import com.google.gwt.dom.client.FrameSetElement;
import com.google.gwt.dom.client.HRElement;
import com.google.gwt.dom.client.HeadElement;
import com.google.gwt.dom.client.HeadingElement;
import com.google.gwt.dom.client.IFrameElement;
import com.google.gwt.dom.client.ImageElement;
import com.google.gwt.dom.client.InputElement;
import com.google.gwt.dom.client.LIElement;
import com.google.gwt.dom.client.LabelElement;
import com.google.gwt.dom.client.LegendElement;
import com.google.gwt.dom.client.LinkElement;
import com.google.gwt.dom.client.MapElement;
import com.google.gwt.dom.client.MetaElement;
import com.google.gwt.dom.client.ModElement;
import com.google.gwt.dom.client.OListElement;
import com.google.gwt.dom.client.ObjectElement;
import com.google.gwt.dom.client.OptGroupElement;
import com.google.gwt.dom.client.OptionElement;
import com.google.gwt.dom.client.ParagraphElement;
import com.google.gwt.dom.client.ParamElement;
import com.google.gwt.dom.client.PreElement;
import com.google.gwt.dom.client.QuoteElement;
import com.google.gwt.dom.client.ScriptElement;
import com.google.gwt.dom.client.SelectElement;
import com.google.gwt.dom.client.SourceElement;
import com.google.gwt.dom.client.SpanElement;
import com.google.gwt.dom.client.StyleElement;
import com.google.gwt.dom.client.TableCaptionElement;
import com.google.gwt.dom.client.TableCellElement;
import com.google.gwt.dom.client.TableColElement;
import com.google.gwt.dom.client.TableElement;
import com.google.gwt.dom.client.TableRowElement;
import com.google.gwt.dom.client.TableSectionElement;
import com.google.gwt.dom.client.TagName;
import com.google.gwt.dom.client.TextAreaElement;
import com.google.gwt.dom.client.TitleElement;
import com.google.gwt.dom.client.UListElement;
import com.google.gwt.dom.client.VideoElement;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.eclipse.xtend.lib.annotations.AccessorType;
import org.eclipse.xtend.lib.annotations.Accessors;
import org.eclipse.xtend.lib.macro.declaration.AnnotationReference;
import org.eclipse.xtend.lib.macro.declaration.Type;
import org.eclipse.xtend.lib.macro.declaration.TypeDeclaration;
import org.eclipse.xtend.lib.macro.services.TypeLookup;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Conversions;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.ListExtensions;
import org.eclipse.xtext.xbase.lib.Pure;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
@SuppressWarnings("all")
public class UiBinderParser {
private final static String PACKAGE_URI_SCHEME = "urn:import:";
private final static String BINDER_URI = "urn:ui:com.google.gwt.uibinder";
@Accessors(AccessorType.PUBLIC_GETTER)
private String gwtPrefix;
@Accessors(AccessorType.PUBLIC_GETTER)
private Map fields = CollectionLiterals.newHashMap();
private final Document document;
@Extension
private final TypeLookup typeLookup;
public UiBinderParser(final InputStream input, final TypeLookup typeLookup) throws Exception {
this.typeLookup = typeLookup;
final DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
factory.setNamespaceAware(true);
DocumentBuilder _newDocumentBuilder = factory.newDocumentBuilder();
Document _parse = _newDocumentBuilder.parse(input);
this.document = _parse;
}
public UiBinderParser parse() {
UiBinderParser _xblockexpression = null;
{
this.parse(this.document);
_xblockexpression = this;
}
return _xblockexpression;
}
protected void parse(final Document doc) {
final Element documentElement = doc.getDocumentElement();
String _lookupPrefix = documentElement.lookupPrefix(UiBinderParser.BINDER_URI);
this.gwtPrefix = _lookupPrefix;
this.parse(documentElement);
}
protected void parse(final Node it) {
if ((it instanceof Element)) {
this.collectData(((Element)it));
}
NodeList _childNodes = it.getChildNodes();
int max = _childNodes.getLength();
for (int i = 0; (i < max); i++) {
NodeList _childNodes_1 = it.getChildNodes();
Node _item = _childNodes_1.item(i);
this.parse(_item);
}
}
protected void collectData(final Element it) {
final String fieldName = this.getFieldName(it);
boolean _notEquals = (!Objects.equal(fieldName, null));
if (_notEquals) {
Type _fieldType = this.getFieldType(it);
this.fields.put(fieldName, _fieldType);
}
}
protected String getFieldName(@Extension final Element it) {
String _xblockexpression = null;
{
String _uiFieldAttributeName = this.getUiFieldAttributeName();
boolean _hasAttribute = it.hasAttribute(_uiFieldAttributeName);
boolean _not = (!_hasAttribute);
if (_not) {
return null;
}
String _uiFieldAttributeName_1 = this.getUiFieldAttributeName();
String _attribute = it.getAttribute(_uiFieldAttributeName_1);
_xblockexpression = _attribute.trim();
}
return _xblockexpression;
}
protected Type getFieldType(final Element it) {
Type _xblockexpression = null;
{
final String tagName = it.getLocalName();
boolean _isImportedElement = this.isImportedElement(it);
boolean _not = (!_isImportedElement);
if (_not) {
return this.findDomElementTypeForTag(tagName);
}
final String ns = it.getNamespaceURI();
int _length = UiBinderParser.PACKAGE_URI_SCHEME.length();
String _substring = ns.substring(_length);
String _plus = (_substring + ".");
final String qualifiedName = (_plus + tagName);
_xblockexpression = this.typeLookup.findTypeGlobally(qualifiedName);
}
return _xblockexpression;
}
protected Type findDomElementTypeForTag(final String tagName) {
Type _xblockexpression = null;
{
final Type tagNameType = this.typeLookup.findTypeGlobally(TagName.class);
Iterable _elementSubTypes = this.getElementSubTypes();
for (final TypeDeclaration elementSubType : _elementSubTypes) {
{
final AnnotationReference annotation = elementSubType.findAnnotation(tagNameType);
boolean _and = false;
boolean _notEquals = (!Objects.equal(annotation, null));
if (!_notEquals) {
_and = false;
} else {
String[] _stringArrayValue = annotation.getStringArrayValue("value");
boolean _contains = ((List)Conversions.doWrapArray(_stringArrayValue)).contains(tagName);
_and = _contains;
}
if (_and) {
return elementSubType;
}
}
}
_xblockexpression = this.typeLookup.findTypeGlobally(com.google.gwt.dom.client.Element.class);
}
return _xblockexpression;
}
protected Iterable getElementSubTypes() {
final ArrayList> _cacheKey = CollectionLiterals.newArrayList();
final Iterable _result;
synchronized (_createCache_getElementSubTypes) {
if (_createCache_getElementSubTypes.containsKey(_cacheKey)) {
return _createCache_getElementSubTypes.get(_cacheKey);
}
final Function1, Type> _function = new Function1, Type>() {
@Override
public Type apply(final Class extends com.google.gwt.dom.client.Element> it) {
return UiBinderParser.this.typeLookup.findTypeGlobally(it);
}
};
List _map = ListExtensions.map(Collections.>unmodifiableList(CollectionLiterals.>newArrayList(AnchorElement.class, AreaElement.class, BaseElement.class, BodyElement.class, BRElement.class, ButtonElement.class, CanvasElement.class, DivElement.class, DListElement.class, FieldSetElement.class, FormElement.class, FrameElement.class, FrameSetElement.class, HeadElement.class, HeadingElement.class, HRElement.class, IFrameElement.class, ImageElement.class, InputElement.class, LabelElement.class, LegendElement.class, LIElement.class, LinkElement.class, MapElement.class, AudioElement.class, VideoElement.class, MetaElement.class, ModElement.class, ObjectElement.class, OListElement.class, OptGroupElement.class, OptionElement.class, ParagraphElement.class, ParamElement.class, PreElement.class, QuoteElement.class, ScriptElement.class, SelectElement.class, SourceElement.class, SpanElement.class, StyleElement.class, TableCaptionElement.class, TableCellElement.class, TableColElement.class, TableElement.class, TableRowElement.class, TableSectionElement.class, TextAreaElement.class, TitleElement.class, UListElement.class)), _function);
_result = Iterables.filter(_map, TypeDeclaration.class);
_createCache_getElementSubTypes.put(_cacheKey, _result);
}
_init_getElementSubTypes(_result);
return _result;
}
private final HashMap, Iterable> _createCache_getElementSubTypes = CollectionLiterals.newHashMap();
private void _init_getElementSubTypes(final Iterable it) {
}
protected boolean isImportedElement(final Element it) {
boolean _xblockexpression = false;
{
final String uri = it.getNamespaceURI();
boolean _and = false;
boolean _notEquals = (!Objects.equal(uri, null));
if (!_notEquals) {
_and = false;
} else {
boolean _startsWith = uri.startsWith(UiBinderParser.PACKAGE_URI_SCHEME);
_and = _startsWith;
}
_xblockexpression = _and;
}
return _xblockexpression;
}
protected String getUiFieldAttributeName() {
final ArrayList> _cacheKey = CollectionLiterals.newArrayList();
final String _result;
synchronized (_createCache_getUiFieldAttributeName) {
if (_createCache_getUiFieldAttributeName.containsKey(_cacheKey)) {
return _createCache_getUiFieldAttributeName.get(_cacheKey);
}
StringConcatenation _builder = new StringConcatenation();
_builder.append(this.gwtPrefix, "");
_builder.append(":field");
_result = _builder.toString();
_createCache_getUiFieldAttributeName.put(_cacheKey, _result);
}
_init_getUiFieldAttributeName(_result);
return _result;
}
private final HashMap, String> _createCache_getUiFieldAttributeName = CollectionLiterals.newHashMap();
private void _init_getUiFieldAttributeName(final String it) {
}
@Pure
public String getGwtPrefix() {
return this.gwtPrefix;
}
@Pure
public Map getFields() {
return this.fields;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy