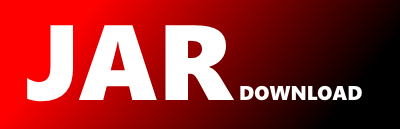
de.itemis.xtend.auto.gwt.WithUiBindingProcessor Maven / Gradle / Ivy
The newest version!
/**
* Copyright (c) 2015 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*/
package de.itemis.xtend.auto.gwt;
import com.google.common.base.Objects;
import com.google.common.collect.Iterables;
import com.google.gwt.uibinder.client.UiBinder;
import com.google.gwt.uibinder.client.UiField;
import com.google.gwt.user.client.ui.Widget;
import de.itemis.xtend.auto.gwt.UiBinderParser;
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.io.PrintStream;
import java.util.Collections;
import java.util.Map;
import java.util.Set;
import org.eclipse.xtend.lib.macro.AbstractClassProcessor;
import org.eclipse.xtend.lib.macro.RegisterGlobalsContext;
import org.eclipse.xtend.lib.macro.TransformationContext;
import org.eclipse.xtend.lib.macro.declaration.AnnotationReference;
import org.eclipse.xtend.lib.macro.declaration.ClassDeclaration;
import org.eclipse.xtend.lib.macro.declaration.CompilationUnit;
import org.eclipse.xtend.lib.macro.declaration.MutableClassDeclaration;
import org.eclipse.xtend.lib.macro.declaration.MutableFieldDeclaration;
import org.eclipse.xtend.lib.macro.declaration.MutableInterfaceDeclaration;
import org.eclipse.xtend.lib.macro.declaration.Type;
import org.eclipse.xtend.lib.macro.declaration.TypeReference;
import org.eclipse.xtend.lib.macro.declaration.Visibility;
import org.eclipse.xtend.lib.macro.file.Path;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtend2.lib.StringConcatenationClient;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
@SuppressWarnings("all")
public class WithUiBindingProcessor extends AbstractClassProcessor {
@Override
public void doRegisterGlobals(final ClassDeclaration it, @Extension final RegisterGlobalsContext context) {
String _uiBinderInterface = this.uiBinderInterface(it);
context.registerInterface(_uiBinderInterface);
}
@Override
public void doTransform(final MutableClassDeclaration it, @Extension final TransformationContext context) {
String _uiBinderInterface = this.uiBinderInterface(it);
final MutableInterfaceDeclaration uiBinderInterfaceType = context.findInterface(_uiBinderInterface);
Iterable extends TypeReference> _extendedInterfaces = uiBinderInterfaceType.getExtendedInterfaces();
TypeReference _newTypeReference = context.newTypeReference(Widget.class);
TypeReference _newTypeReference_1 = context.newTypeReference(it);
TypeReference _newTypeReference_2 = context.newTypeReference(UiBinder.class, _newTypeReference, _newTypeReference_1);
Iterable _plus = Iterables.concat(_extendedInterfaces,
Collections.unmodifiableList(CollectionLiterals.newArrayList(_newTypeReference_2)));
uiBinderInterfaceType.setExtendedInterfaces(_plus);
final Procedure1 _function = new Procedure1() {
@Override
public void apply(final MutableFieldDeclaration it) {
it.setStatic(true);
it.setFinal(true);
TypeReference _newTypeReference = context.newTypeReference(uiBinderInterfaceType);
it.setType(_newTypeReference);
StringConcatenationClient _client = new StringConcatenationClient() {
@Override
protected void appendTo(StringConcatenationClient.TargetStringConcatenation _builder) {
_builder.append("com.google.gwt.core.client.GWT.create(");
String _simpleName = uiBinderInterfaceType.getSimpleName();
_builder.append(_simpleName, "");
_builder.append(".class)");
}
};
it.setInitializer(_client);
}
};
it.addField("UI_BINDER", _function);
final UiBinderParser parser = this.createUiBinderParser(it, context);
boolean _equals = Objects.equal(parser, null);
if (_equals) {
return;
}
UiBinderParser _parse = parser.parse();
final Map fields = _parse.getFields();
Set> _entrySet = fields.entrySet();
for (final Map.Entry entry : _entrySet) {
String _key = entry.getKey();
final Procedure1 _function_1 = new Procedure1() {
@Override
public void apply(final MutableFieldDeclaration it) {
Type _value = entry.getValue();
TypeReference _newTypeReference = context.newTypeReference(_value);
it.setType(_newTypeReference);
it.setVisibility(Visibility.PROTECTED);
AnnotationReference _newAnnotationReference = context.newAnnotationReference(UiField.class);
it.addAnnotation(_newAnnotationReference);
}
};
it.addField(_key, _function_1);
}
}
protected UiBinderParser createUiBinderParser(final MutableClassDeclaration it, @Extension final TransformationContext context) {
UiBinderParser _xtrycatchfinallyexpression = null;
try {
Path _uiXmlPath = this.uiXmlPath(it);
InputStream _contentsAsStream = context.getContentsAsStream(_uiXmlPath);
_xtrycatchfinallyexpression = new UiBinderParser(_contentsAsStream, context);
} catch (final Throwable _t) {
if (_t instanceof Exception) {
final Exception io = (Exception)_t;
Object _xblockexpression = null;
{
StringConcatenation _builder = new StringConcatenation();
_builder.append("Error loading file \'");
Path _uiXmlPath_1 = this.uiXmlPath(it);
_builder.append(_uiXmlPath_1, "");
_builder.append("\' : [");
String _message = io.getMessage();
_builder.append(_message, "");
_builder.append("]");
context.addError(it, _builder.toString());
final ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
PrintStream _printStream = new PrintStream(byteArrayOutputStream);
io.printStackTrace(_printStream);
String _string = byteArrayOutputStream.toString();
context.addError(it, _string);
_xblockexpression = null;
}
_xtrycatchfinallyexpression = ((UiBinderParser)_xblockexpression);
} else {
throw Exceptions.sneakyThrow(_t);
}
}
return _xtrycatchfinallyexpression;
}
protected String uiBinderInterface(final ClassDeclaration it) {
String _qualifiedName = it.getQualifiedName();
String _plus = (_qualifiedName + ".");
String _simpleName = it.getSimpleName();
String _plus_1 = (_plus + _simpleName);
return (_plus_1 + "UiBinder");
}
protected Path uiXmlPath(final MutableClassDeclaration it) {
CompilationUnit _compilationUnit = it.getCompilationUnit();
Path _filePath = _compilationUnit.getFilePath();
Path _parent = _filePath.getParent();
StringConcatenation _builder = new StringConcatenation();
String _simpleName = it.getSimpleName();
_builder.append(_simpleName, "");
_builder.append(".ui.xml");
return _parent.append(_builder.toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy