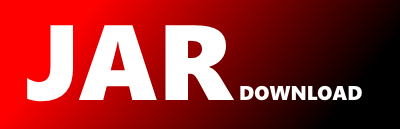
org.xwiki.displayer.internal.DefaultHTMLDisplayerManager Maven / Gradle / Ivy
/*
* See the NOTICE file distributed with this work for additional
* information regarding copyright ownership.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.xwiki.displayer.internal;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.Map;
import javax.inject.Inject;
import javax.inject.Named;
import javax.inject.Provider;
import javax.inject.Singleton;
import org.xwiki.component.annotation.Component;
import org.xwiki.component.manager.ComponentLookupException;
import org.xwiki.component.manager.ComponentManager;
import org.xwiki.component.util.DefaultParameterizedType;
import org.xwiki.displayer.HTMLDisplayer;
import org.xwiki.displayer.HTMLDisplayerException;
import org.xwiki.displayer.HTMLDisplayerManager;
/**
* Default implementation for {@link org.xwiki.displayer.HTMLDisplayerManager}.
*
* @version $Id: 97f138f0ca6b5a831ca69d1d43b2df30cacec6c1 $
* @since 10.11RC1
*/
@Component
@Singleton
public class DefaultHTMLDisplayerManager implements HTMLDisplayerManager
{
/**
* Use to find the proper {@link org.xwiki.displayer.HTMLDisplayer} component for the provided target type.
*/
@Inject
@Named("context")
private Provider componentManagerProvider;
@Override
public HTMLDisplayer getHTMLDisplayer(Type targetType) throws HTMLDisplayerException
{
return this.getHTMLDisplayer(targetType, null);
}
/**
* {@inheritDoc}
*
* Example: if the target type is A<B<C>>
with {@code hint} hint, the following lookups
* will be made until a {@link HTMLDisplayer} component is found:
*
* - Component:
HTMLDisplayer<A<B<C>>>
; Role: {@code hint}
* - Component:
HTMLDisplayer<A<B>>
; Role: {@code hint}
* - Component:
HTMLDisplayer<A>
; Role: {@code hint}
* - Component: {@code HTMLDisplayer}; Role: {@code hint}
*
- Component: {@code HTMLDisplayer}; Role: default
*
*/
@Override
public HTMLDisplayer getHTMLDisplayer(Type targetType, String roleHint) throws HTMLDisplayerException
{
try {
HTMLDisplayer component;
ComponentManager componentManager = this.componentManagerProvider.get();
Type type = targetType;
Type displayerType = new DefaultParameterizedType(null, HTMLDisplayer.class, type);
while (!componentManager.hasComponent(displayerType, roleHint) && type instanceof ParameterizedType) {
type = ((ParameterizedType) type).getRawType();
displayerType = new DefaultParameterizedType(null, HTMLDisplayer.class, type);
}
if (!componentManager.hasComponent(displayerType, roleHint)) {
displayerType = new DefaultParameterizedType(null, HTMLDisplayer.class, Object.class);
}
if (componentManager.hasComponent(displayerType, roleHint)) {
component = componentManager.getInstance(displayerType, roleHint);
} else {
component = componentManager.getInstance(displayerType);
}
return component;
} catch (ComponentLookupException e) {
throw new HTMLDisplayerException("Failed to initialized the HTML displayer for target type [" + targetType
+ "] and role [" + String.valueOf(roleHint) + "]", e);
}
}
@Override
public String display(Type targetType, T value) throws HTMLDisplayerException
{
Type type = getType(targetType, value);
return getHTMLDisplayer(type).display(type, value);
}
@Override
public String display(Type targetType, T value, Map parameters) throws HTMLDisplayerException
{
Type type = getType(targetType, value);
return getHTMLDisplayer(type).display(type, value, parameters);
}
@Override
public String display(Type targetType, T value, Map parameters, String mode)
throws HTMLDisplayerException
{
Type type = getType(targetType, value);
return getHTMLDisplayer(type).display(type, value, parameters, mode);
}
private Type getType(Type targetType, T value)
{
if (targetType == null && value != null) {
return value.getClass();
} else {
return targetType;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy