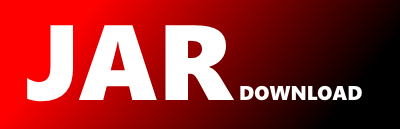
xworker.util.ThingUtils Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2007-2014 See AUTHORS file.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package xworker.util;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import javax.xml.parsers.ParserConfigurationException;
import org.xmeta.ActionContext;
import org.xmeta.ActionException;
import org.xmeta.Thing;
import org.xmeta.World;
import org.xmeta.codes.XmlCoder;
import org.xmeta.util.UtilMap;
import org.xml.sax.SAXException;
import xworker.dataObject.DataObject;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.fasterxml.jackson.databind.node.ObjectNode;
public class ThingUtils {
/**
* 根据注册类型(registType)查找注册到被注册事物(registorThing)下的事物。
*
* 可以输入关键字查找注册的事物。
*
* @param registorThing
* @param registType
* @param keywords
* @param actionContext
* @return
*/
@SuppressWarnings("unchecked")
public static List searchRegistThings(Thing registorThing, String registType, List keywords, ActionContext actionContext){
if(registorThing == null){
return Collections.emptyList();
}
List thingList = new ArrayList();
if("child".equals(registType) && !"xworker.lang.util.ThingIndex".equals(registorThing.getMetadata().getPath())){
//如果是子节点,优先添加事物自身的以及继承的子节点,
for(Thing child : registorThing.getChilds()){
thingList.add(child);
}
for(Thing ext : registorThing.getExtends()){
for(Thing child : ext.getChilds()){
thingList.add(child);
}
}
}
String keyStr = "";
for(int i=0; i 0){
keyStr = keyStr + ",";
}
keyStr = keyStr + keywords.get(i);
}
World world = World.getInstance();
Thing registByThing = world.getThing("xworker.ide.db.dbindex.app.dataObject.RegistsByThing");
List results = (List) registByThing.doAction("query", actionContext, UtilMap.toMap(
new Object[]{"thing", registorThing, "keywords", keyStr, "registType", registType, "noDescriptor", true}));
for(DataObject obj : results){
Thing thing = world.getThing((String) obj.get("path"));
if(thing != null){
if(thing.getBoolean("th_registDisabled")){
continue;
}
//用户自定义分组
thing.getMetadata().setUserGroup(obj.getString("userGroup"));
//是否注册是子事物,即把自己注册到某一个事物,其实是要注册自己的所有子事物到被注册的事物下
if(thing.getBoolean("th_registMyChilds")){
for(Thing child : thing.getChilds()){
if(child.getBoolean("th_registDisabled")){
continue;
}
thingList.add(child);
}
}else{
thingList.add(thing);
}
}
}
return thingList;
}
/**
* 获取显示事物Description的URL地址。
*
* @param thing
* @return
*/
public static String getThingDescUrl(Thing thing){
return GlobalConfig.getThingDescUrl(thing);
}
/**
* 分析XML的结构获取描述者。
*
* @param xml
* @return
* @throws ParserConfigurationException
* @throws SAXException
* @throws IOException
*/
public static Thing parseXMLStrucutre(String xml) throws ParserConfigurationException, SAXException, IOException{
Thing thing = createEmptyThing();
thing.parseXML(xml);
return parseDescriptor(thing);
}
/**
* 分析JSON的结构获取描述者。
*
* @param json
* @return
* @throws IOException
* @throws JsonMappingException
* @throws JsonParseException
*/
public static Thing parseJSONStructure(String json) throws JsonParseException, JsonMappingException, IOException{
Thing thing = parseJson(json);
return parseDescriptor(thing);
}
public static Thing parseDescriptor(Thing thing){
if(thing.getDescriptors().size() > 1){
return thing.getDescriptor().detach();
}else{
Thing desc = new Thing("xworker.lang.MetaDescriptor3");
String nodeName = thing.getStringBlankAsNull(XmlCoder.NODE_NAME);
if(nodeName != null){
desc.put("name", nodeName);
}else{
desc.put("name", "thing");
}
if(thing.getBoolean("json_isArray")){
desc.put("json_isArray", "true");
}
for(String key : thing.getAttributes().keySet()){
if(key.equals(XmlCoder.NODE_NAME)){
continue;
}
if("json_isArray".equals(key)){
continue;
}
Thing attr = new Thing("xworker.lang.MetaDescriptor3/@attribute");
attr.put("name", key);
desc.addChild(attr);
}
for(Thing child : thing.getChilds()){
desc.addChild(parseDescriptor(child));
}
return desc;
}
}
/**
* 把事物转化成JSON字符串。
*
* @param thing
* @return
*/
public static String toJson(Thing thing){
ObjectMapper mapper = new ObjectMapper();
JsonNode node = toJsonNode(thing, mapper);
return node.toString();
}
public static JsonNode toJsonNode(Thing thing, ObjectMapper mapper){
if(thing.getBoolean("json_isArray")){
ArrayNode node = mapper.createArrayNode();
for(Thing child : thing.getChilds()){
node.add(toJsonNode(child, mapper));
}
return node;
}else{
ObjectNode node = mapper.createObjectNode();
List attributes = thing.getAttributesDescriptors();
for(Thing attr : attributes){
if(attr.getBoolean("notXmlAttribute") == false){
String name = attr.getMetadata().getName();
Object value = thing.get(name);
if(value != null){
String type = attr.getString("string");
if(type == null || "".equals(type) || "string".equals(type)){
node.put(name, thing.getString(name));
}else if("bigDecimal".equals(type)){
node.put(name, thing.getBigDecimal(name));
}else if("bigInteger".equals(type)){
node.put(name, thing.getBigDecimal(name));
}else if("boolean".equals(type)){
node.put(name, thing.getBoolean(name));
}else if("byte".equals(type)){
node.put(name, thing.getByte(name));
}else if("char".equals(type)){
node.put(name, thing.getChar(name));
}else if("date".equals(type)){
node.put(name, thing.getString(name));
}else if("double".equals(type)){
node.put(name, thing.getDouble(name));
}else if("float".equals(type)){
node.put(name, thing.getFloat(name));
}else if("int".equals(type)){
node.put(name, thing.getInt(name));
}else if("long".equals(type)){
node.put(name, thing.getLong(name));
}else if("short".equals(type)){
node.put(name, thing.getShort(name));
}else{
throw new ActionException("Not support type '" + type + "'");
}
}
}
}
for(Thing child : thing.getChilds()){
node.put(child.getThingName(), toJsonNode(child, mapper));
}
return node;
}
}
/**
* 把一个JSON字符串转化为一个事物。
*
* @param jsonString
* @return
* @throws IOException
* @throws JsonMappingException
* @throws JsonParseException
*/
@SuppressWarnings("unchecked")
public static Thing parseJson(String jsonString) throws JsonParseException, JsonMappingException, IOException{
ObjectMapper mapper = new ObjectMapper();
jsonString = jsonString.trim();
Thing thing = createEmptyThing();
ByteArrayInputStream in = new ByteArrayInputStream(jsonString.getBytes());
if(jsonString.startsWith("{")){
Map values = mapper.readValue(in, Map.class);
parseJson(thing, values);
}else{
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy