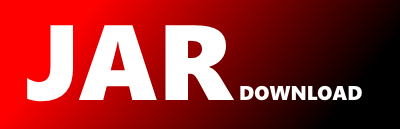
xworker.app.task.TaskCalendar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xworker_app Show documentation
Show all versions of xworker_app Show documentation
XWorker app model liberary.
The newest version!
package xworker.app.task;
import org.eclipse.swt.internal.C;
import org.xmeta.Action;
import org.xmeta.ActionContext;
import org.xmeta.Thing;
import org.xmeta.World;
import xworker.dataObject.DataObject;
import xworker.dataObject.DataObjectEntity;
import xworker.lang.executor.Executor;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.List;
public class TaskCalendar extends DataObjectEntity {
private static final String TAG = TaskCalendar.class.getName();
public static final int ONCE = 0;
public static final int EVERY_DAY = 1;
public static final int EVERY_WEEK = 2;
public static final int EVERY_MONTH = 3;
public static final int EVERY_YEAR = 4;
public TaskCalendar(){
super(new DataObject("xworker.app.task.dataobjects.TaskCalandar"));
}
public TaskCalendar(DataObject data){
super(data);
}
@Override
public TaskCalendar createInstance() {
return new TaskCalendar();
}
public void setId(Long value){
this.put("id", value);
}
public Long getId(){
return this.getLong("id");
}
public void setName(String value){
this.put("name", value);
}
public String getName(){
return this.getString("name");
}
public void setThingPath(String value){
this.put("thingPath", value);
}
public String getThingPath(){
return this.getString("thingPath");
}
public void setStartDate(Date value){
this.put("startDate", value);
}
public Date getStartDate(){
return this.getDate("startDate");
}
public void setEndDate(Date value){
this.put("endDate", value);
}
public Date getEndDate(){
return this.getDate("endDate");
}
public void setStartTime(Date value){
this.put("startTime", value);
}
public Date getStartTime(){
return this.getDate("startTime");
}
public void setEndTime(Date value){
this.put("endTime", value);
}
public Date getEndTime(){
return this.getDate("endTime");
}
public void setPeriodType(Integer value){
this.put("periodType", value);
}
public Integer getPeriodType(){
return this.getInt("periodType");
}
public void setReminderMinute(String value){
this.put("reminderMinute", value);
}
public String getReminderMinute(){
return this.getString("reminderMinute");
}
public void setActive(Boolean value){
this.put("active", value);
}
public Boolean getActive(){
return this.getBoolean("active");
}
public void setDescription(String value){
this.put("description", value);
}
public String getDescription(){
return this.getString("description");
}
public void setCreateDate(Date value){
this.put("createDate", value);
}
public void setPriority(String value){
this.put("priority", value);
}
public String getPriority(){
return this.getString("priority");
}
public Date getCreateDate(){
return this.getDate("createDate");
}
public void setLastRemindDate(Date value){
this.put("lastRemindDate", value);
}
public Date getLastRemindDate(){
return this.getDate("lastRemindDate");
}
public void setLastActiveTime(Date value){
this.put("lastActiveTime", value);
}
public Date getLastActiveTime(){
return this.getDate("lastActiveTime");
}
public boolean isInDate(Date date){
Date startDate = getStartDate();
if(startDate == null){
//需要有开始日期
return false;
}
if(date.getTime() < startDate.getTime()){
//还未到开始
return false;
}
Date endDate = getEndDate();
if(endDate != null && date.getTime() > endDate.getTime()){
//超过了截至日期
return false;
}
int periodType = getPeriodType();
GregorianCalendar dateCalendar = new GregorianCalendar();
dateCalendar.setTime(date);
GregorianCalendar startCalendar = new GregorianCalendar();
startCalendar.setTime(startDate);
Date lastActiveDate = getLastActiveTime();
if(periodType == EVERY_YEAR){
//每年,即每年的指定月-日
return !isSameDay(lastActiveDate, date) && dateCalendar.get(Calendar.MONTH) == startCalendar.get(Calendar.MONTH) &&
dateCalendar.get(Calendar.DAY_OF_MONTH) == startCalendar.get(Calendar.DAY_OF_MONTH);
} else if(periodType == EVERY_MONTH){
//每月
return !isSameDay(lastActiveDate, date) && dateCalendar.get(Calendar.DAY_OF_MONTH) == startCalendar.get(Calendar.DAY_OF_MONTH);
} else if(periodType == EVERY_WEEK){
//每周
return !isSameDay(lastActiveDate, date) && dateCalendar.get(Calendar.DAY_OF_WEEK) == startCalendar.get(Calendar.DAY_OF_WEEK);
} else if(periodType == EVERY_DAY){
//每天
return !isSameDay(lastActiveDate, date);
} else {
//只执行一次
return lastActiveDate == null;
}
}
public boolean isSameDay(Date lastActiveDate, Date date){
if(lastActiveDate == null){
return true;
}
GregorianCalendar dateCalendar = new GregorianCalendar();
dateCalendar.setTime(date);
GregorianCalendar lastCalendar = new GregorianCalendar();
lastCalendar.setTime(lastActiveDate);
return dateCalendar.get(Calendar.YEAR) == lastCalendar.get(Calendar.YEAR) &&
dateCalendar.get(Calendar.MONTH) == lastCalendar.get(Calendar.MONTH) &&
dateCalendar.get(Calendar.DAY_OF_MONTH) == lastCalendar.get(Calendar.DAY_OF_MONTH);
}
//是否在提醒时间内。提醒的最小间隔是按每5分钟一次算的
public boolean isInRemind(Date date) throws ParseException {
SimpleDateFormat sf = new SimpleDateFormat("yyyy-MM-dd");
Date startDate = getStartDate();
Date startTime = getStartTime();
if(startDate == null){
startDate = sf.parse(sf.format(new Date()));
}
startDate = new Date(startDate.getTime() + startTime.getTime());
String reminderMinute = getReminderMinute();
if(reminderMinute != null){
for(String ms : reminderMinute.split("[,]")){
ms = ms.trim();
if(ms.isEmpty()){
continue;
}
int m = Integer.parseInt(ms);
if(m > 0 && isRemindDate(startDate, m, date) && !isLastRemindDate(date)){
//符合提醒时间范围
Date lastRemindDate = new Date(startDate.getTime() + m * 60000L);
setLastRemindDate(lastRemindDate);
return true;
}
}
}
return false;
}
boolean isLastRemindDate(Date date){
Date lastRemindDate = getLastRemindDate();
if(lastRemindDate == null){
return false;
}
//5分钟内都算
return date.getTime() >= lastRemindDate.getTime() && date.getTime() <= lastRemindDate.getTime() + 300000;
}
boolean isRemindDate(Date startDate, int minute, Date date){
Date start = new Date(startDate.getTime() + minute * 60000L);
return date.getTime() >= start.getTime() && date.getTime() < start.getTime() + 300000;
}
//返回是否在起始的时间范围内
public boolean isInTime(Date date) throws ParseException {
Date startTime = getStartTime();
Date endTime = getEndTime();
//通过格式化去掉年月日等
SimpleDateFormat sf = new SimpleDateFormat("HH:mm:ss");
if(startTime == null){
return false;
}
startTime = sf.parse(sf.format(startTime));
date = sf.parse(sf.format(date));
if(endTime != null){
endTime = sf.parse(sf.format(endTime));
}
if(date.getTime() < startTime.getTime()){
return false;
}
return endTime == null || date.getTime() < endTime.getTime();
}
public boolean isActive(Date date) throws ParseException {
if(!this.getActive()){
//未激活
return false;
}
if(isInRemind(date)){
//是否到了提醒时间
return true;
} else if(isInDate(date) && isInTime(date)){
//是否到了运行时间
setLastActiveTime(date);
return true;
} else {
return false;
}
}
public void execute(ActionContext actionContext){
//更新数据库
this.update(actionContext);
Action action = World.getInstance().getAction(getThingPath());
if(action != null){
//执行指定的动作
action.run(actionContext);
} else {
//发送通知
Thing htmlReq = new Thing("xworker.lang.executor.RequestIndex/@Html");
htmlReq.set("label", getName());
htmlReq.set("html", getDescription());
htmlReq.set("priority", getPriority());
htmlReq.set("noCancelButton", "true");
htmlReq.set("requestId", "XWorkerAPP:TaskCalendar:" + getId());
Date endTime = getEndTime();
if(endTime != null){
GregorianCalendar dateCalendar = new GregorianCalendar();
GregorianCalendar endCalendar = new GregorianCalendar();
endCalendar.setTime(endTime);
htmlReq.set("timeout", getMinuteOfDay(endCalendar) - getMinuteOfDay(dateCalendar));
}
htmlReq.doAction("run", actionContext);
}
}
public int getMinuteOfDay(GregorianCalendar calendar){
int hour = calendar.get(Calendar.HOUR_OF_DAY);
int minute = calendar.get(Calendar.MINUTE);
return hour * 60 + minute;
}
/**
* 日期任务定时器,执行顶确定好了的日期的任务。
*/
@SuppressWarnings({"unchecked" })
public static void run(ActionContext actionContext) {
//从数据库中读取要执行的任务
Thing dbThing = World.getInstance().getThing("xworker.app.task.dataobjects.TaskCalandar");
List datas = (List) dbThing.doAction("query", actionContext);
//遍历执行任务
Date date = new Date();
for(DataObject data : datas){
try {
TaskCalendar taskCalendar = new TaskCalendar(data);
if (taskCalendar.isActive(date)) {
taskCalendar.execute(actionContext);
}
} catch (Exception e){
Executor.warn(TAG, "Execute calendar task error, id=" + data.get("id"), e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy