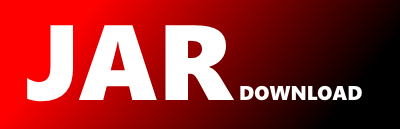
xworker.app.userflow.UserFlowManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xworker_app Show documentation
Show all versions of xworker_app Show documentation
XWorker app model liberary.
The newest version!
package xworker.app.userflow;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xmeta.ActionContext;
import org.xmeta.Thing;
import xworker.dataObject.DataObject;
public class UserFlowManager {
private final static Logger logger = LoggerFactory.getLogger(UserFlowManager.class);
private final static List listeners = new ArrayList<>();
public static int startFlow(Thing flow, ActionContext actionContext) throws SQLException {
int count = 0;
for(Thing task : flow.getChilds()){
if(task.getBoolean("autoStart")){
if (startTask(task, actionContext)){
count++;
}
}
}
return count;
}
public static boolean startTask(Thing task, ActionContext actionContext) throws SQLException {
return UserFlowActions.startTask(task, false, actionContext);
}
public static boolean startTask(Thing task, boolean forceStart, ActionContext actionContext) throws SQLException {
return UserFlowActions.startTask(task, forceStart, actionContext);
}
public static void finish(Thing task , ActionContext actionContext) throws SQLException {
UserFlowActions.finish(task, actionContext);
}
public static void finishAndNotify(Thing task , ActionContext actionContext) throws SQLException {
NewTaskNotifier.clear();
try {
UserFlowActions.finish(task, actionContext);
} finally {
if(NewTaskNotifier.hasTasks()) {
NewTaskNotifier.showDialog();
}
}
}
public static void addListener(UserFlowListener listener){
if(!listeners.contains(listener)){
listeners.add(listener);
}
}
public static void removeListener(UserFlowListener listener){
listeners.remove(listener);
}
public static void fireTaskStarted(Thing thing, DataObject task){
for(UserFlowListener l :listeners){
try{
l.started(thing, task);
}catch(Exception e){
logger.error("handle fireTskStarted error, task=" + task, e);
}
}
}
public static void fireTaskFinished(Thing thing, DataObject task){
for(UserFlowListener l :listeners){
try{
l.finished(thing, task);
}catch(Exception e){
logger.error("handle fireTaskFinished error, task=" + task, e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy