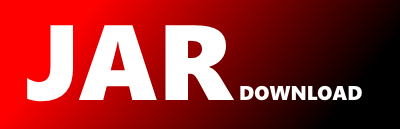
xworker.dataObject.DataObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xworker_dataobject Show documentation
Show all versions of xworker_dataobject Show documentation
DataObject is a data mapping model.
/*******************************************************************************
* Copyright 2007-2013 See AUTHORS file.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package xworker.dataObject;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.xmeta.ActionContext;
import org.xmeta.ActionException;
import org.xmeta.Bindings;
import org.xmeta.Thing;
import org.xmeta.World;
import org.xmeta.util.UtilData;
import xworker.dataObject.cache.DataObjectCache;
import xworker.dataObject.utils.DataObjectUtil;
/**
* 数据对象,用于保存数据的数据对象,数据对象之间的关系类似关系数据库。
*
* 数据对象是一个Map,用于存放key-value键值对,MetaData保存了关键字、属性定义和脏字段信息等。
*
* 数据对象的定义是事物,数据对象的行为是由事物实现的,可以有自己的行为。
*
* 数据对象如果已初始化setInited(true),那么会记录脏字段信息,否则不会记录。
*
* 数据对象的标志(flag)表示数据的存储情况,FLAG_STORED=1表示已存储,FLAG_NEW新数据,FLAG_DELETED已删除。
* 数据对象的标志设置为FLAG_STORED和FLAG_DELETED并不表示数据在真实的存储中的状态,但会影响当前数据对象的操作,
* 当数据对象commit()时,如果是新的或已删除,那么会调用create或delete方法。
*
* @author zhangyuxiang
*
*/
public class DataObject extends HashMap {
private static final long serialVersionUID = 1L;
/** 标志 - 已保存 */
public static final int FLAG_STORED = 1;
/** 标志 - 新数据 */
public static final int FLAG_NEW = 2;
/** 标志 - 已删除 */
public static final int FLAG_DELETED = 3;
/**
* 元数据。
*/
private DataObjectMetadata metadata = null;
private int flag = FLAG_STORED;
/** 和SWT控件setData和getData一样,用于保存第三方的数据 */
private Map datas = new HashMap();
/**
* 无描述者的构造函数,这样构造出来的数据对象只能当作临时数据(Map)用。
*/
public DataObject() {
}
/**
* 使用描述者的路径构造一个数据对象。
*
* @param descriptorPath
*/
public DataObject(String descriptorPath) {
Thing descriptor = World.getInstance().getThing(descriptorPath);
if(descriptor == null){
throw new ActionException("Descriptor is null, path=" + descriptorPath);
}
metadata = new DataObjectMetadata(descriptor, this);
}
/**
* 构造函数。
*
* @param descriptor 描述者
*/
public DataObject(Thing descriptor) {
metadata = new DataObjectMetadata(descriptor, this);
}
@Override
public Object put(String key, Object value) {
return put(key, value, true);
}
public Object set(String key, Object value) {
return put(key, value, true);
}
public String getString(String key){
return UtilData.getString(get(key), null);
}
public byte getByte(String key){
return UtilData.getByte(get(key), (byte) 0);
}
public short getShort(String key){
return UtilData.getShort(get(key), (short) 0);
}
public int getInt(String key){
return UtilData.getInt(get(key), 0);
}
public long getLong(String key){
return UtilData.getLong(get(key), 0);
}
public boolean getBoolean(String key){
return UtilData.getBoolean(get(key), false);
}
public byte[] getBytes(String key){
return UtilData.getBytes(get(key), null);
}
public Date getDate(String key){
return UtilData.getDate(get(key), null);
}
public double getDouble(String key){
return UtilData.getDouble(get(key), 0);
}
public float getFloat(String key){
return UtilData.getFloat(get(key), 0);
}
public BigDecimal getBigDecimal(String key){
return UtilData.getBigDecimal(get(key), null);
}
public BigInteger getBigInteger(String key){
return UtilData.getBigInteger(get(key), null);
}
/**
* 设置数据对象的键值。
*
* @param key 键
* @param value 值
* @param fireEvent 是否触发事件
* @return 结果
*/
public Object put(String key, Object value, boolean fireEvent) {
if (key == null) {
return null;
}
if (fireEvent && metadata != null) {
metadata.beforeFieldChanged(key, value);
}
Thing definition = metadata.getDefinition(key);
if (definition != null && "attribute".equals(definition.getThingName())) {
return super.put(key, DataObjectUtil.getValue(value, definition));
} else {
return super.put(key, value);
}
}
@Override
public void putAll(Map extends String, ? extends Object> m) {
for (String key : m.keySet()) {
this.put(key, m.get(key));
}
}
/**
* 设置是否已初始化,如果未初始化,那么字段变动时不会记录到脏数据中。
*
* @param inited
*/
public void setInited(boolean inited) {
metadata.setInited(inited);
}
/**
* 返回是否已初始化。
*
* @return 是否已初始化
*/
public boolean isInited() {
return metadata.isInited();
}
/**
* 返回是否是脏数据。
*
* @return 如果是脏数据返回true,否则返回false
*/
public boolean isDirty() {
return metadata.isDirty() || flag == DataObject.FLAG_DELETED
|| flag == DataObject.FLAG_NEW;
}
/**
* 返回元数据。
*
* @return 元数据
*/
public DataObjectMetadata getMetadata() {
return metadata;
}
/**
* 返回主键和主键的值。
*
* @return 主键和对应的值
*/
public Object[][] getKeyAndDatas() {
return metadata.getKeyAndDatas();
}
/**
* 初始化默认值,并设置状态为已初始化。
*
* @param actionContext 变量上下文
*/
public void init(ActionContext actionContext) {
setInited(true);
doAction("init", actionContext);
}
/**
* 查询数据,是DataObjectUtil的一种快捷方式。
*
* @param conditions 条件集合
* @param actionContext 变量上下文
* @return 查询结果
*/
public List query(Map conditions, ActionContext actionContext){
return DataObjectUtil.query(getMetadata().getDescriptor().getMetadata().getPath(), conditions, actionContext);
}
@Override
public Object get(Object key) {
if (!isInited()) {
load(null);
}
Object obj = super.get(key);
if (obj instanceof DataObject) {
DataObject dobj = (DataObject) obj;
if (!dobj.isInited()) {
dobj.load(null);
}
} else if (obj instanceof DataObjectList) {
DataObjectList dobjlist = (DataObjectList) obj;
if (!dobjlist.isInited()) {
dobjlist.load(null);
}
}
return obj;
}
/**
* 装载数据,并设置状态为已初始化。
*
* 如果数据存在返回本事物,否则返回null或者抛出异常。
*
* @param actionContext 变量上下文
*/
public DataObject load(ActionContext actionContext) {
setInited(true);
return (DataObject) doAction("load", actionContext);
}
/**
* 查询数据。
*
* @param actionContext 变量上下文
* @param condition 条件
* @param queryDatas 查询条件
*/
public Object query(ActionContext actionContext, Object condition,
Object queryDatas) {
if (actionContext == null) {
actionContext = new ActionContext();
}
try {
Bindings bindings = actionContext.push(null);
bindings.put("condition", condition);
bindings.put("datas", queryDatas);
return doAction("query", actionContext);
} finally {
actionContext.pop();
}
}
/**
* 提交变更。
*
* @param actionContext 变量上下文
*/
public void commit(ActionContext actionContext) {
switch (flag) {
case DataObject.FLAG_DELETED:
delete(actionContext);
break;
case DataObject.FLAG_NEW:
create(actionContext);
break;
case DataObject.FLAG_STORED:
if (isDirty()) {
update(actionContext);
}
break;
}
}
/**
* 创建如果成功返回创建后的数据对象,否则返回null。
*
* @return 结果
*/
public DataObject create(ActionContext actionContext) {
return (DataObject) doAction("create", actionContext);
}
/**
* 创建或更新。
*/
public void createOrUpdate(ActionContext actionContext) {
DataObject loadData = this.load(actionContext);
if(loadData != null){
this.update(actionContext);
}else{
this.create(actionContext);
}
}
public DataObject createOrUpdate(List keys, ActionContext actionContext) {
Map conditions = new HashMap();
for(String key : keys){
conditions.put(key, get(key));
}
DataObject loadData = null;
List datas = DataObjectUtil.query(getMetadata().getDescriptor().getMetadata().getPath(), conditions, actionContext);
if(datas != null && datas.size() > 0){
loadData = datas.get(0);
}
if(loadData != null){
loadData.update(actionContext);
return loadData;
}else{
return this.create(actionContext);
}
}
/**
* 通过keys执行的字段名作为查询条件判断记录是否存在,如果存在则不创建,否则执行创建。
*
* @param keys 关键字列表
* @param actionContext 变量上下文
*
* @return 如果新建了数据对象,或返回第一个已经有了数据对象
*/
public DataObject createIfNotExists(List keys, ActionContext actionContext){
Map conditions = new HashMap();
for(String key : keys){
conditions.put(key, get(key));
}
List datas = DataObjectUtil.query(getMetadata().getDescriptor().getMetadata().getPath(), conditions, actionContext);
if(datas == null || datas.size() <= 0){
return this.create(actionContext);
}else{
return datas.get(0);
}
}
/**
* 更新。
*
* @return 已更新的数量
*/
public int update(ActionContext actionContext) {
Object obj = doAction("update", actionContext);
//清空脏数据和重置主键
metadata.updateKeyAndDatas();
metadata.cleanDirty();
return (int) UtilData.getLong(obj, 0);
}
/**
* 删除。
*
* @return 已删除的数量
*/
public int delete(ActionContext actionContext) {
Object obj = doAction("delete", actionContext);
return (int) UtilData.getLong(obj, 0);
}
/**
* 执行动作。
*
* @param name 动作名
* @return 结果
*/
public Object doAction(String name) {
return doAction(name, null, null);
}
/**
* 执行动作。
*
* @param name 动作名
* @param actionContext 变量上下文
* @return 结果
*/
public Object doAction(String name, ActionContext actionContext) {
return doAction(name, actionContext, null);
}
/**
* 执行动作。
*
* @param name 动作名
* @param actionContext 变量上下文
* @param params 参数
* @return 结果
*/
public Object doAction(String name, ActionContext actionContext,
Map params) {
Thing thing = metadata.getDescriptor();
if (actionContext == null) {
actionContext = new ActionContext();
}
Bindings bindings = actionContext.push(null);
try {
bindings.setCaller(this, name);
bindings.put("theData", this);
return thing.doAction(name, actionContext, params);
} finally {
actionContext.pop();
}
}
/**
* 获取数据的标志,是新的还是旧的还是已删除的。
*
* @return 标志
*/
public int getFlag() {
return flag;
}
/**
* 设置数据的标志,是新的还是旧的还是已删除的。
*/
public void setFlag(int flag) {
this.flag = flag;
}
/**
* 设置第三方数据,运行时调用者需要的数据。
*
* @param key 键
* @param value 值
*/
public void setData(String key, Object value) {
datas.put(key, value);
}
/**
* 获取第三方数据,运行时调用者需要的数据。
*
* @param key 键
* @return 值
*/
public Object getData(String key) {
return datas.get(key);
}
/**
* 开始线程缓存。 try{ beginThreadCache(); }finally{ finishThreadCache(); }
*/
public static void beginThreadCache() {
DataObjectCache.begin();
}
/**
* 结束线程缓存。和beginThreadCache()匹配。
*/
public static void finishThreadCache() {
DataObjectCache.finish();
}
/**
* 数据对象装载后的初始化 ,初始化一对多的关系和装载事件。
*/
public void fireOnLoaded(ActionContext actionContext){
Thing descriptor = getMetadata().getDescriptor();
//初始化多个属性列表
List things = getMetadata().getThings();
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy