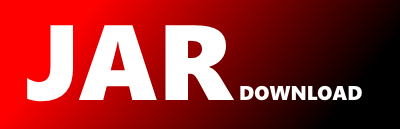
xworker.swt.actions.ControlSetActions Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2007-2013 See AUTHORS file.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package xworker.swt.actions;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.eclipse.swt.widgets.Control;
import org.xmeta.ActionContext;
import org.xmeta.Thing;
import org.xmeta.World;
import xworker.swt.design.Designer;
public class ControlSetActions {
public static Map> run(ActionContext actionContext){
Map> controls = new HashMap>();
Map pathNameMap = new HashMap();
Thing self = (Thing) actionContext.get("self");
if(self.getString("ref") != null){
Thing ref = World.getInstance().getThing(self.getString("ref"));
if(ref != null){
self = ref;
}
}
//父控件列表
List parents = new ArrayList();
if(self.getString("parentConrols") != null){
for(String p : self.getString("parentConrols").split("[,]")){
p = p.trim();
if("".equals(p)){
continue;
}else{
parents.add(p);
}
}
}
//查找范围和要查找的控件
String scope = self.getString("scope");
List controlPathList = new ArrayList();
for(Thing child : self.getChilds()){
controlPathList.add(child.getString("thingPath"));
}
//初步获取指定控件的所有列表,可能包含于多个shell
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy