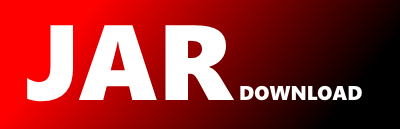
xworker.swt.actions.DisplayActions Maven / Gradle / Ivy
package xworker.swt.actions;
import java.util.HashMap;
import java.util.Map;
import ognl.Ognl;
import ognl.OgnlException;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Widget;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xmeta.ActionContext;
import org.xmeta.Thing;
import xworker.swt.design.Designer;
import xworker.task.Task;
public class DisplayActions {
private static Logger logger = LoggerFactory.getLogger(DisplayActions.class);
public static void exec(ActionContext actionContext) throws OgnlException{
Thing self = (Thing) actionContext.get("self");
//获取display
String widgetForDisplay = self.getStringBlankAsNull("widgetForDisplay");
Display display = null;
if(widgetForDisplay != null){
try{
Widget widget = (Widget) Ognl.getValue(widgetForDisplay, actionContext);//actionContext.get(widgetForDisplay);
if(widget != null && !widget.isDisposed()){
display = widget.getDisplay();
}
}catch(Exception e){
display = Designer.getExplorerDisplay();
}
}else{
display = Display.getCurrent();
}
if(display == null){
if(self.getBoolean("cancelTaskOnWidgetDisposed")){
try{
Task task = (Task) actionContext.get("task");
if(task != null){
task.cancel(false);
}
}catch(Exception e){
logger.warn("DisplayExex end task error, path=" + self.getMetadata().getPath(), e);
}
}
self.doAction("onDisplayError", actionContext);
return;
}
String type = self.getString("type");
String variables = self.getStringBlankAsNull("variables");
Map vars = null;
if(variables != null){
vars = new HashMap();
for(String var : variables.split("[,]")){
vars.put(var, actionContext.get(var));
}
}
Exec exec = new Exec();
exec.variables = vars;
exec.actionContext = actionContext;
exec.thing = self;
if("async".equals(type)){
display.asyncExec(exec);
}else{
display.syncExec(exec);
}
}
static class Exec implements Runnable{
Map variables = null;
ActionContext actionContext = null;
Thing thing = null;
@Override
public void run() {
try{
if(variables != null){
thing.doAction("doAction", actionContext, variables);
}else{
thing.doAction("doAction", actionContext);
}
}catch(Exception e){
logger.error("DisplayExec: execute error happened, thing=" + thing.getMetadata().getPath(), e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy