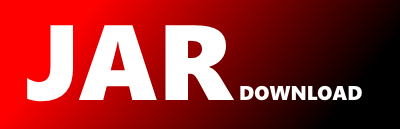
xworker.swt.actions.FileActions Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2007-2013 See AUTHORS file.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package xworker.swt.actions;
import ognl.Ognl;
import ognl.OgnlException;
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.FileDialog;
import org.eclipse.swt.widgets.Shell;
import org.xmeta.ActionContext;
import org.xmeta.ActionException;
import org.xmeta.Thing;
import org.xmeta.util.UtilAction;
import org.xmeta.util.UtilString;
public class FileActions {
/**
* 选择文件当做字符串。
*
* @param actionContext
* @return
*/
public static Object selectFileAsString(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
//打开文件对话框
FileDialog fileDialog = createFileDialog(actionContext);
String file = fileDialog.open();
//保存变量
String varName = self.getStringBlankAsNull("varName");
UtilAction.putVarByActioScope(self, varName, file, actionContext);
return file;
}
/**
* 创建文件对话框。
*
* @param actionContext
* @return
*/
public static FileDialog createFileDialog(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
//样式
int style = SWT.NONE;
if("SAVE".equals(self.getString("style"))){
style = style | SWT.SAVE;
}else{
style = style | SWT.OPEN;
}
if(self.getBoolean("MULTI")){
style = style | SWT.MULTI;
}
//创建FileDialog
Shell parent = null;
String parentShell = self.getStringBlankAsNull("parentShell");
if(parentShell != null){
try {
parent = (Shell) Ognl.getValue(parentShell, actionContext);
} catch (OgnlException e) {
throw new ActionException("create file dialog error", e);
}
}else{
parent = (Shell) actionContext.get("parent");
}
FileDialog fileDialog = new FileDialog(parent, style);
//其他属性
String fileName = self.getStringBlankAsNull("fileName");
if(fileName != null){
fileDialog.setFileName(fileName);
}
String filterExtensions = self.getStringBlankAsNull("filterExtensions");
if(filterExtensions != null){
fileDialog.setFilterExtensions(UtilString.getStringArray(filterExtensions));
}
String filterNames = self.getStringBlankAsNull("filterNames");
if(filterNames != null){
fileDialog.setFilterNames(UtilString.getStringArray(filterNames));
}
String filterPath = self.getStringBlankAsNull("filterPath");
if(filterPath != null){
fileDialog.setFilterPath(filterPath);
}
int filterIndex = self.getInt("filterIndex", 0);
fileDialog.setFilterIndex(filterIndex);
boolean overwrite = self.getBoolean("overwrite");
fileDialog.setOverwrite(overwrite);
return fileDialog;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy