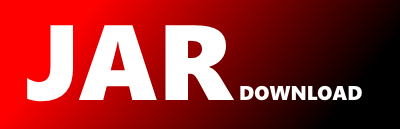
xworker.swt.actions.FileDialogActions Maven / Gradle / Ivy
package xworker.swt.actions;
import ognl.OgnlException;
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.FileDialog;
import org.eclipse.swt.widgets.Shell;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xmeta.ActionContext;
import org.xmeta.Thing;
import org.xmeta.util.UtilData;
import org.xmeta.util.UtilMap;
import org.xmeta.util.UtilString;
public class FileDialogActions {
private static Logger logger = LoggerFactory.getLogger(FileDialogActions.class);
public static Object run(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
Shell shell = (Shell) self.doAction("getShell", actionContext);
int style = SWT.OPEN;
String s = self.getStringBlankAsNull("style");
if("SAVE".equals(s)){
style = SWT.SAVE;
}else if("MULTI".equals(s)){
style = SWT.MULTI;
}
FileDialog dialog = new FileDialog(shell, style);
String fileName = (String) self.doAction("getFileName", actionContext);
String text = (String) self.doAction("getText", actionContext);
String[] filterExtensions = (String[]) self.doAction("getFilterExtensions", actionContext);
Integer filterIndex = (Integer) self.doAction("getFilterIndex", actionContext);
String[] filterNames = (String[]) self.doAction("getFilterNames", actionContext);
String filterPath = (String) self.doAction("getFilterPath", actionContext);
Boolean overwrite = (Boolean) self.doAction("getOverwrite", actionContext);
if(fileName != null){
dialog.setFileName(fileName);
}
if(filterExtensions != null){
dialog.setFilterExtensions(filterExtensions);
}
if(filterIndex != null){
dialog.setFilterIndex(filterIndex);
}
if(filterNames != null){
dialog.setFilterNames(filterNames);
}
if(filterPath != null){
dialog.setFilterPath(filterPath);
}
if(overwrite != null){
dialog.setOverwrite(overwrite);
}
if(text != null){
dialog.setText(text);
}
String f = dialog.open();
return self.doAction("open", actionContext, UtilMap.toMap("fileName", f));
}
public static Object open(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
logger.info(self.getMetadata().getPath() + " open:" + actionContext.get("fileName"));
return actionContext.get("fileName");
}
public static Object getShell(ActionContext actionContext) throws OgnlException{
Thing self = (Thing) actionContext.get("self");
Object obj = UtilData.getData(self, "shell", actionContext);
if(obj instanceof String){
return actionContext.get((String) obj);
}else{
return obj;
}
}
public static Object getFileName(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
return UtilString.getString(self, "fileName", actionContext);
}
public static Object getText(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
return UtilString.getString(self, "text", actionContext);
}
public static Object getFilterExtensions(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
String filterExtensions = self.getStringBlankAsNull("filterExtensions");
if(filterExtensions != null){
return filterExtensions.split("[,]");
}else{
return null;
}
}
public static Object getFilterIndex(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
if(self.getStringBlankAsNull("filterIndex") != null){
return self.getInt("filterIndex");
}else{
return null;
}
}
public static Object getFilterNames(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
String filterExtensions = self.getStringBlankAsNull("filterNames");
if(filterExtensions != null){
return filterExtensions.split("[,]");
}else{
return null;
}
}
public static Object getFilterPath(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
return UtilString.getString(self, "filterPath", actionContext);
}
public static Object getOverwrite(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
return self.getBoolean("overwrite");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy