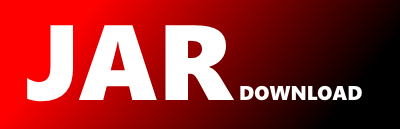
xworker.swt.actions.StyledTextActions Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2007-2013 See AUTHORS file.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package xworker.swt.actions;
import java.io.IOException;
import java.io.InputStream;
import ognl.OgnlException;
import org.eclipse.swt.custom.StyledText;
import org.xmeta.ActionContext;
import org.xmeta.Thing;
import xworker.swt.custom.StyledTextInputStreamRunnable;
import xworker.swt.util.VerifyEventToInputStream;
public class StyledTextActions {
/**
* 绑定InputStream到StyledText。
*
* @param actionContext
* @return
* @throws OgnlException
*/
public static Object bindInputStream(ActionContext actionContext) throws OgnlException{
Thing self = (Thing) actionContext.get("self");
StyledText text = (StyledText) self.doAction("getStyledText", actionContext);
InputStream inputStream = (InputStream) self.doAction("getInputStream", actionContext);
boolean autoScroolToBottom = self.getBoolean("autoScroolToBottom");
if(text != null && inputStream != null){
String charset = self.getStringBlankAsNull("charset");
if(charset == null){
charset = "utf-8";
}
StyledTextInputStreamRunnable run = new StyledTextInputStreamRunnable(text, inputStream, autoScroolToBottom, charset);
if(self.getBoolean("createThread")){
new Thread(run).start();
}else{
run.run();
}
return run;
}else{
return null;
}
}
public static void keyInputToInputStream(ActionContext actionContext) throws IOException{
Thing self = (Thing) actionContext.get("self");
StyledText text = (StyledText) self.doAction("getStyledText", actionContext);
VerifyEventToInputStream listener = new VerifyEventToInputStream(self.getBoolean("eventDoit"));
text.addVerifyKeyListener(listener);
String inputStreamVarName = self.getStringBlankAsNull("inputStreamVarName");
if(inputStreamVarName != null){
actionContext.getScope(0).put(inputStreamVarName, listener.getInputStream());
}
actionContext.getScope(0).put(self.getMetadata().getName(), listener);
}
public static void append(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
StyledText styledText = (StyledText) self.doAction("getStyledText", actionContext);
String text = (String) self.doAction("getText", actionContext);
if(styledText != null && text != null){
styledText.append(text);
if(self.getBoolean("autoScroolToBottom")){
styledText.setCaretOffset(styledText.getText().length());
styledText.showSelection();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy