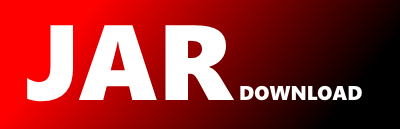
xworker.swt.design.DesignShellActions Maven / Gradle / Ivy
package xworker.swt.design;
import java.util.ArrayList;
import java.util.List;
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.StyledText;
import org.eclipse.swt.graphics.Point;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Control;
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
import org.eclipse.swt.widgets.Tree;
import org.eclipse.swt.widgets.TreeItem;
import org.xmeta.ActionContext;
import org.xmeta.Thing;
import org.xmeta.World;
import org.xmeta.util.UtilMap;
import xworker.swt.ActionContainer;
import xworker.swt.form.TextEditor;
import xworker.swt.util.XWorkerTreeUtil;
import xworker.swt.xworker.CodeAssistor;
/**
* 设计器界面的相关动作。
*
* @author Administrator
*
*/
public class DesignShellActions {
/**
* 控件的设计树。
*
* @param actionContext
*/
public static void designTreeInit(ActionContext actionContext){
//初始化条目
Control control = (Control) actionContext.get("control");
String thingPath = (String) control.getData("_designer_thingPath");
Thing thing = World.getInstance().getThing(thingPath);
if(thing == null){
return;
}
Thing rootThing = thing.getRoot();
Control rootControl = getRootControl(control, rootThing);
Tree tree = (Tree) actionContext.get("tree");
if(rootControl != actionContext.getScope(0).get("rootControl")){
actionContext.getScope(0).put("rootControl", rootControl);
if(tree == null){
return;
}
tree.removeAll();
List- items = new ArrayList
- ();
initItem(rootControl, items, rootThing);
//在树上显示
for(Item item : items){
initItemAtTree(tree, item, actionContext, control);
}
}
Text pathText = (Text) actionContext.get("pathText");
pathText.setText(thingPath);
ActionContainer ac = (ActionContainer) actionContext.get("actions");
if(tree.getItems().length == 0){
ac.doAction("itemUnSelected", actionContext);
}else{
ac.doAction("itemSelected", actionContext);
}
//设置编辑器
ActionContext thingEditor = (ActionContext) actionContext.get("thingEditor");
ActionContainer editorActions = (ActionContainer) thingEditor.get("editorActions");
editorActions.doAction("setThing", UtilMap.toMap("thing", thing));
editorActions.doAction("selectThing", UtilMap.toMap("thing", thing));
//代码辅助
StyledText codeText = (StyledText) actionContext.get("codeText");
TextEditor.attach(codeText);
actionContext.g().put("controlContext", control.getData(Designer.DATA_ACTIONCONTEXT));
CodeAssistor.attach(null, codeText, (ActionContext) control.getData(Designer.DATA_ACTIONCONTEXT));
}
public static void initItemAtTree(Object parentItem, Item item, ActionContext actionContext, Control control){
TreeItem treeItem = null;
if(parentItem instanceof Tree){
treeItem = new TreeItem((Tree) parentItem, SWT.None);
}else{
treeItem = new TreeItem((TreeItem) parentItem, SWT.None);
}
XWorkerTreeUtil.initItem(treeItem, item.thing, actionContext);
treeItem.setData(item);
for(Item childItem : item.child){
initItemAtTree(treeItem, childItem, actionContext, control);
}
treeItem.setExpanded(true);
if(item.control == control){
treeItem.getParent().select(treeItem);
treeItem.getParent().showItem(treeItem);
actionContext.getScope(0).put("item", item);
}
}
/**
* 删除选中的控件,会同时删除相关的事物。
*
* @param control
*/
public static void deleteControl(final Control control){
String thingPath = (String) control.getData("_designer_thingPath");
Thing thing = World.getInstance().getThing(thingPath);
Thing parentThing = thing.getParent();
if(parentThing != null){
parentThing.removeChild(thing);
}else{
thing.remove();
}
control.getDisplay().asyncExec(new Runnable(){
public void run(){
Composite parent = control.getParent();
Composite parentParent = parent.getParent();
control.dispose();
if(parent != null){
if(parentParent != null){
parentParent.layout();
}else{
parent.redraw();
}
}
}
});
}
public static void updateControl(final Item item, final ActionContainer parentActions){
item.control.getDisplay().asyncExec(new Runnable(){
public void run(){
ActionContext actionContext = (ActionContext) item.control.getData("_designer_actionContext");
if(item.control instanceof Shell){
Shell shell = (Shell) item.control.getShell();
Point location = shell.getLocation();
Object parent = shell.getParent();
if(parent == null){
parent = shell.getDisplay();
}
shell.dispose();
actionContext.peek().put("parent", parent);
shell = (Shell) item.thing.doAction("create", actionContext);
item.control = shell;
shell.setLocation(location);
shell.open();
}else{
Composite parent = item.control.getParent();
int index = -1;
int childCount = parent.getChildren().length;
for(int i=0;i
items, Thing rootThing){
String thingPath = (String) control.getData("_designer_thingPath");
//ActionContext actionContext = (ActionContext) control.getData("_designer_actionContext");
Boolean isAttribute = (Boolean) control.getData("_designer_isAttribute");
if(thingPath != null && !isAttribute){
Item item = new Item();
item.thing = World.getInstance().getThing(thingPath);
if(item.thing == null || item.thing.getRoot() != rootThing){
return;
}
item.control = control;
items.add(item);
items = item.child;
}
if(control instanceof Composite){
Composite composite = (Composite) control;
for(Control childControl : composite.getChildren()){
initItem(childControl, items, rootThing);
}
}
}
public static Control getRootControl(Control control, Thing rootThing){
Composite parent = control.getParent();
if(parent != null){// && !(control instanceof Shell)){
String thingPath = (String) parent.getData("_designer_thingPath");
Thing thing = World.getInstance().getThing(thingPath);
if(thing != null && thing.getRoot() == rootThing){
return getRootControl(parent, rootThing);
}
}
return control;
}
static class Item{
public Control control;
public Thing thing;
public List- child = new ArrayList
- ();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy