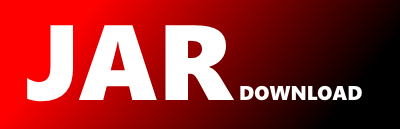
xworker.swt.util.SwtUtils Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2007-2013 See AUTHORS file.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package xworker.swt.util;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.text.NumberFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import ognl.Ognl;
import ognl.OgnlException;
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.CCombo;
import org.eclipse.swt.custom.StyledText;
import org.eclipse.swt.events.DisposeEvent;
import org.eclipse.swt.events.DisposeListener;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.graphics.GC;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.graphics.ImageData;
import org.eclipse.swt.graphics.ImageLoader;
import org.eclipse.swt.graphics.Point;
import org.eclipse.swt.graphics.Rectangle;
import org.eclipse.swt.graphics.Transform;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Combo;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Control;
import org.eclipse.swt.widgets.DateTime;
import org.eclipse.swt.widgets.Dialog;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Monitor;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
import org.eclipse.swt.widgets.Widget;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xmeta.ActionContext;
import org.xmeta.ActionException;
import org.xmeta.Thing;
import org.xmeta.World;
import org.xmeta.util.UtilData;
import xworker.swt.ActionContainer;
import xworker.util.XWorkerUtils;
public class SwtUtils {
private static Logger log = LoggerFactory.getLogger(SwtUtils.class);
private static Map swtKeys = new HashMap();
static{
swtKeys.put("ABORT", SWT.ABORT);
swtKeys.put("Activate", SWT.Activate);
swtKeys.put("ALPHA", SWT.ALPHA);
swtKeys.put("ALT", SWT.ALT);
swtKeys.put("APPLICATION_MODAL", SWT.APPLICATION_MODAL);
swtKeys.put("Arm", SWT.Arm);
swtKeys.put("ARROW", SWT.ARROW);
swtKeys.put("ARROW_DOWN", SWT.ARROW_DOWN);
swtKeys.put("ARROW_LEFT", SWT.ARROW_LEFT);
swtKeys.put("ARROW_RIGHT", SWT.ARROW_RIGHT);
swtKeys.put("ARROW_UP", SWT.ARROW_UP);
swtKeys.put("BACKGROUND", SWT.BACKGROUND);
swtKeys.put("BALLOON", SWT.BALLOON);
swtKeys.put("BAR", SWT.BAR);
swtKeys.put("BEGINNING", SWT.BEGINNING);
swtKeys.put("BITMAP", SWT.BITMAP);
swtKeys.put("BOLD", SWT.BOLD);
swtKeys.put("BORDER", SWT.BORDER);
swtKeys.put("BORDER_DASH", SWT.BORDER_DASH);
swtKeys.put("BORDER_DOT", SWT.BORDER_DOT);
swtKeys.put("BORDER_SOLID", SWT.BORDER_SOLID);
swtKeys.put("BOTTOM", SWT.BOTTOM);
swtKeys.put("BREAK", SWT.BREAK);
swtKeys.put("BS", (int) SWT.BS);
swtKeys.put("BUTTON_MASK", SWT.BUTTON_MASK);
swtKeys.put("BUTTON1", SWT.BUTTON1);
swtKeys.put("BUTTON2", SWT.BUTTON2);
swtKeys.put("BUTTON3", SWT.BUTTON3);
swtKeys.put("BUTTON4", SWT.BUTTON4);
swtKeys.put("BUTTON5", SWT.BUTTON5);
swtKeys.put("CALENDAR", SWT.CALENDAR);
swtKeys.put("CANCEL", SWT.CANCEL);
swtKeys.put("CAP_FLAT", SWT.CAP_FLAT);
swtKeys.put("CAP_ROUND", SWT.CAP_ROUND);
swtKeys.put("CAP_SQUARE", SWT.CAP_SQUARE);
swtKeys.put("CAPS_LOCK", SWT.CAPS_LOCK);
swtKeys.put("CASCADE", SWT.CASCADE);
swtKeys.put("CENTER", SWT.CENTER);
swtKeys.put("CHECK", SWT.CHECK);
swtKeys.put("CLIP_CHILDREN", SWT.CLIP_CHILDREN);
swtKeys.put("CLIP_SIBLINGS", SWT.CLIP_SIBLINGS);
swtKeys.put("Close", SWT.Close);
swtKeys.put("CLOSE", SWT.CLOSE);
swtKeys.put("Collapse", SWT.Collapse);
swtKeys.put("COLOR_BLACK", SWT.COLOR_BLACK);
swtKeys.put("COLOR_BLUE", SWT.COLOR_BLUE);
swtKeys.put("COLOR_CYAN", SWT.COLOR_CYAN);
swtKeys.put("COLOR_DARK_BLUE", SWT.COLOR_DARK_BLUE);
swtKeys.put("COLOR_DARK_CYAN", SWT.COLOR_DARK_CYAN);
swtKeys.put("COLOR_DARK_GRAY", SWT.COLOR_DARK_GRAY);
swtKeys.put("COLOR_DARK_GREEN", SWT.COLOR_DARK_GREEN);
swtKeys.put("COLOR_DARK_MAGENTA", SWT.COLOR_DARK_MAGENTA);
swtKeys.put("COLOR_DARK_RED", SWT.COLOR_DARK_RED);
swtKeys.put("COLOR_DARK_YELLOW", SWT.COLOR_DARK_YELLOW);
swtKeys.put("COLOR_GRAY", SWT.COLOR_GRAY);
swtKeys.put("COLOR_GREEN", SWT.COLOR_GREEN);
swtKeys.put("COLOR_INFO_BACKGROUND", SWT.COLOR_INFO_BACKGROUND);
swtKeys.put("COLOR_INFO_FOREGROUND", SWT.COLOR_INFO_FOREGROUND);
swtKeys.put("COLOR_LIST_BACKGROUND", SWT.COLOR_LIST_BACKGROUND);
swtKeys.put("COLOR_LIST_FOREGROUND", SWT.COLOR_LIST_FOREGROUND);
swtKeys.put("COLOR_LIST_SELECTION", SWT.COLOR_LIST_SELECTION);
swtKeys.put("COLOR_LIST_SELECTION_TEXT", SWT.COLOR_LIST_SELECTION_TEXT);
swtKeys.put("COLOR_MAGENTA", SWT.COLOR_MAGENTA);
swtKeys.put("COLOR_RED", SWT.COLOR_RED);
swtKeys.put("COLOR_TITLE_BACKGROUND", SWT.COLOR_TITLE_BACKGROUND);
swtKeys.put("COLOR_TITLE_BACKGROUND_GRADIENT", SWT.COLOR_TITLE_BACKGROUND_GRADIENT);
swtKeys.put("COLOR_TITLE_FOREGROUND", SWT.COLOR_TITLE_FOREGROUND);
swtKeys.put("COLOR_TITLE_INACTIVE_BACKGROUND", SWT.COLOR_TITLE_INACTIVE_BACKGROUND);
swtKeys.put("COLOR_TITLE_INACTIVE_BACKGROUND_GRADIENT", SWT.COLOR_TITLE_INACTIVE_BACKGROUND_GRADIENT);
swtKeys.put("COLOR_TITLE_INACTIVE_FOREGROUND", SWT.COLOR_TITLE_INACTIVE_FOREGROUND);
swtKeys.put("COLOR_WHITE", SWT.COLOR_WHITE);
swtKeys.put("COLOR_WIDGET_BACKGROUND", SWT.COLOR_WIDGET_BACKGROUND);
swtKeys.put("COLOR_WIDGET_BORDER", SWT.COLOR_WIDGET_BORDER);
swtKeys.put("COLOR_WIDGET_DARK_SHADOW", SWT.COLOR_WIDGET_DARK_SHADOW);
swtKeys.put("COLOR_WIDGET_FOREGROUND", SWT.COLOR_WIDGET_FOREGROUND);
swtKeys.put("COLOR_WIDGET_HIGHLIGHT_SHADOW", SWT.COLOR_WIDGET_HIGHLIGHT_SHADOW);
swtKeys.put("COLOR_WIDGET_LIGHT_SHADOW", SWT.COLOR_WIDGET_LIGHT_SHADOW);
swtKeys.put("COLOR_WIDGET_NORMAL_SHADOW", SWT.COLOR_WIDGET_NORMAL_SHADOW);
swtKeys.put("COLOR_YELLOW", SWT.COLOR_YELLOW);
swtKeys.put("COMMAND", SWT.COMMAND);
swtKeys.put("COMPOSITION_CHANGED", SWT.COMPOSITION_CHANGED);
swtKeys.put("COMPOSITION_OFFSET", SWT.COMPOSITION_OFFSET);
swtKeys.put("COMPOSITION_SELECTION", SWT.COMPOSITION_SELECTION);
swtKeys.put("CONTROL", SWT.CONTROL);
swtKeys.put("CR", (int) SWT.CR);
swtKeys.put("CTRL", SWT.CTRL);
swtKeys.put("CURSOR_APPSTARTING", SWT.CURSOR_APPSTARTING);
swtKeys.put("CURSOR_ARROW", SWT.CURSOR_ARROW);
swtKeys.put("CURSOR_CROSS", SWT.CURSOR_CROSS);
swtKeys.put("CURSOR_HAND", SWT.CURSOR_HAND);
swtKeys.put("CURSOR_HELP", SWT.CURSOR_HELP);
swtKeys.put("CURSOR_IBEAM", SWT.CURSOR_IBEAM);
swtKeys.put("CURSOR_NO", SWT.CURSOR_NO);
swtKeys.put("CURSOR_SIZEALL", SWT.CURSOR_SIZEALL);
swtKeys.put("CURSOR_SIZEE", SWT.CURSOR_SIZEE);
swtKeys.put("CURSOR_SIZEN", SWT.CURSOR_SIZEN);
swtKeys.put("CURSOR_SIZENE", SWT.CURSOR_SIZENE);
swtKeys.put("CURSOR_SIZENESW", SWT.CURSOR_SIZENESW);
swtKeys.put("CURSOR_SIZENS", SWT.CURSOR_SIZENS);
swtKeys.put("CURSOR_SIZENW", SWT.CURSOR_SIZENW);
swtKeys.put("CURSOR_SIZENWSE", SWT.CURSOR_SIZENWSE);
swtKeys.put("CURSOR_SIZES", SWT.CURSOR_SIZES);
swtKeys.put("CURSOR_SIZESE", SWT.CURSOR_SIZESE);
swtKeys.put("CURSOR_SIZESW", SWT.CURSOR_SIZESW);
swtKeys.put("CURSOR_SIZEW", SWT.CURSOR_SIZEW);
swtKeys.put("CURSOR_SIZEWE", SWT.CURSOR_SIZEWE);
swtKeys.put("CURSOR_UPARROW", SWT.CURSOR_UPARROW);
swtKeys.put("CURSOR_WAIT", SWT.CURSOR_WAIT);
swtKeys.put("DATE", SWT.DATE);
swtKeys.put("DBCS", SWT.DBCS);
swtKeys.put("Deactivate", SWT.Deactivate);
swtKeys.put("DEFAULT", SWT.DEFAULT);
swtKeys.put("DefaultSelection", SWT.DefaultSelection);
swtKeys.put("Deiconify", SWT.Deiconify);
swtKeys.put("DEL", (int) SWT.DEL);
swtKeys.put("DELIMITER_SELECTION", SWT.DELIMITER_SELECTION);
swtKeys.put("DIALOG_TRIM", SWT.DIALOG_TRIM);
swtKeys.put("Dispose", SWT.Dispose);
swtKeys.put("DM_FILL_BACKGROUND", SWT.DM_FILL_BACKGROUND);
swtKeys.put("DM_FILL_NONE", SWT.DM_FILL_NONE);
swtKeys.put("DM_FILL_PREVIOUS", SWT.DM_FILL_PREVIOUS);
swtKeys.put("DM_UNSPECIFIED", SWT.DM_UNSPECIFIED);
swtKeys.put("DOUBLE_BUFFERED", SWT.DOUBLE_BUFFERED);
swtKeys.put("DOWN", SWT.DOWN);
swtKeys.put("DRAG", SWT.DRAG);
swtKeys.put("DragDetect", SWT.DragDetect);
swtKeys.put("DRAW_DELIMITER", SWT.DRAW_DELIMITER);
swtKeys.put("DRAW_MNEMONIC", SWT.DRAW_MNEMONIC);
swtKeys.put("DRAW_TAB", SWT.DRAW_TAB);
swtKeys.put("DRAW_TRANSPARENT", SWT.DRAW_TRANSPARENT);
swtKeys.put("DROP_DOWN", SWT.DROP_DOWN);
swtKeys.put("EMBEDDED", SWT.EMBEDDED);
swtKeys.put("END", SWT.END);
swtKeys.put("EraseItem", SWT.EraseItem);
swtKeys.put("ERROR", SWT.ERROR);
swtKeys.put("ERROR_CANNOT_BE_ZERO", SWT.ERROR_CANNOT_BE_ZERO);
swtKeys.put("ERROR_CANNOT_GET_COUNT", SWT.ERROR_CANNOT_GET_COUNT);
swtKeys.put("ERROR_CANNOT_GET_ENABLED", SWT.ERROR_CANNOT_GET_ENABLED);
swtKeys.put("ERROR_CANNOT_GET_ITEM", SWT.ERROR_CANNOT_GET_ITEM);
swtKeys.put("ERROR_CANNOT_GET_ITEM_HEIGHT", SWT.ERROR_CANNOT_GET_ITEM_HEIGHT);
swtKeys.put("ERROR_CANNOT_GET_SELECTION", SWT.ERROR_CANNOT_GET_SELECTION);
swtKeys.put("ERROR_CANNOT_GET_TEXT", SWT.ERROR_CANNOT_GET_TEXT);
swtKeys.put("ERROR_CANNOT_INVERT_MATRIX", SWT.ERROR_CANNOT_INVERT_MATRIX);
swtKeys.put("ERROR_CANNOT_SET_ENABLED", SWT.ERROR_CANNOT_SET_ENABLED);
swtKeys.put("ERROR_CANNOT_SET_MENU", SWT.ERROR_CANNOT_SET_MENU);
swtKeys.put("ERROR_CANNOT_SET_SELECTION", SWT.ERROR_CANNOT_SET_SELECTION);
swtKeys.put("ERROR_CANNOT_SET_TEXT", SWT.ERROR_CANNOT_SET_TEXT);
swtKeys.put("ERROR_DEVICE_DISPOSED", SWT.ERROR_DEVICE_DISPOSED);
swtKeys.put("ERROR_FAILED_EXEC", SWT.ERROR_FAILED_EXEC);
swtKeys.put("ERROR_FAILED_LOAD_LIBRARY", SWT.ERROR_FAILED_LOAD_LIBRARY);
swtKeys.put("ERROR_GRAPHIC_DISPOSED", SWT.ERROR_GRAPHIC_DISPOSED);
swtKeys.put("ERROR_INVALID_ARGUMENT", SWT.ERROR_INVALID_ARGUMENT);
swtKeys.put("ERROR_INVALID_FONT", SWT.ERROR_INVALID_FONT);
swtKeys.put("ERROR_INVALID_IMAGE", SWT.ERROR_INVALID_IMAGE);
swtKeys.put("ERROR_INVALID_PARENT", SWT.ERROR_INVALID_PARENT);
swtKeys.put("ERROR_INVALID_RANGE", SWT.ERROR_INVALID_RANGE);
swtKeys.put("ERROR_INVALID_SUBCLASS", SWT.ERROR_INVALID_SUBCLASS);
swtKeys.put("ERROR_IO", SWT.ERROR_IO);
swtKeys.put("ERROR_ITEM_NOT_ADDED", SWT.ERROR_ITEM_NOT_ADDED);
swtKeys.put("ERROR_ITEM_NOT_REMOVED", SWT.ERROR_ITEM_NOT_REMOVED);
swtKeys.put("ERROR_MENU_NOT_BAR", SWT.ERROR_MENU_NOT_BAR);
swtKeys.put("ERROR_MENU_NOT_DROP_DOWN", SWT.ERROR_MENU_NOT_DROP_DOWN);
swtKeys.put("ERROR_MENU_NOT_POP_UP", SWT.ERROR_MENU_NOT_POP_UP);
swtKeys.put("ERROR_MENUITEM_NOT_CASCADE", SWT.ERROR_MENUITEM_NOT_CASCADE);
swtKeys.put("ERROR_NO_GRAPHICS_LIBRARY", SWT.ERROR_NO_GRAPHICS_LIBRARY);
swtKeys.put("ERROR_NO_HANDLES", SWT.ERROR_NO_HANDLES);
swtKeys.put("ERROR_NO_MORE_CALLBACKS", SWT.ERROR_NO_MORE_CALLBACKS);
swtKeys.put("ERROR_NOT_IMPLEMENTED", SWT.ERROR_NOT_IMPLEMENTED);
swtKeys.put("ERROR_NULL_ARGUMENT", SWT.ERROR_NULL_ARGUMENT);
swtKeys.put("ERROR_THREAD_INVALID_ACCESS", SWT.ERROR_THREAD_INVALID_ACCESS);
swtKeys.put("ERROR_UNSPECIFIED", SWT.ERROR_UNSPECIFIED);
swtKeys.put("ERROR_UNSUPPORTED_DEPTH", SWT.ERROR_UNSUPPORTED_DEPTH);
swtKeys.put("ERROR_UNSUPPORTED_FORMAT", SWT.ERROR_UNSUPPORTED_FORMAT);
swtKeys.put("ERROR_WIDGET_DISPOSED", SWT.ERROR_WIDGET_DISPOSED);
swtKeys.put("ESC", (int) SWT.ESC);
swtKeys.put("Expand", SWT.Expand);
swtKeys.put("F1", SWT.F1);
swtKeys.put("F10", SWT.F10);
swtKeys.put("F11", SWT.F11);
swtKeys.put("F12", SWT.F12);
swtKeys.put("F13", SWT.F13);
swtKeys.put("F14", SWT.F14);
swtKeys.put("F15", SWT.F15);
swtKeys.put("F2", SWT.F2);
swtKeys.put("F3", SWT.F3);
swtKeys.put("F4", SWT.F4);
swtKeys.put("F5", SWT.F5);
swtKeys.put("F6", SWT.F6);
swtKeys.put("F7", SWT.F7);
swtKeys.put("F8", SWT.F8);
swtKeys.put("F9", SWT.F9);
swtKeys.put("FILL", SWT.FILL);
swtKeys.put("FILL_EVEN_ODD", SWT.FILL_EVEN_ODD);
swtKeys.put("FILL_WINDING", SWT.FILL_WINDING);
swtKeys.put("FLAT", SWT.FLAT);
swtKeys.put("FOCUSED", SWT.FOCUSED);
swtKeys.put("FocusIn", SWT.FocusIn);
swtKeys.put("FocusOut", SWT.FocusOut);
swtKeys.put("FOREGROUND", SWT.FOREGROUND);
swtKeys.put("FULL_SELECTION", SWT.FULL_SELECTION);
swtKeys.put("H_SCROLL", SWT.H_SCROLL);
swtKeys.put("HardKeyDown", SWT.HardKeyDown);
swtKeys.put("HardKeyUp", SWT.HardKeyUp);
swtKeys.put("Help", SWT.Help);
swtKeys.put("HELP", SWT.HELP);
swtKeys.put("Hide", SWT.Hide);
swtKeys.put("HIDE_SELECTION", SWT.HIDE_SELECTION);
swtKeys.put("HIGH", SWT.HIGH);
swtKeys.put("HOME", SWT.HOME);
swtKeys.put("HORIZONTAL", SWT.HORIZONTAL);
swtKeys.put("HOT", SWT.HOT);
swtKeys.put("ICON", SWT.ICON);
swtKeys.put("ICON_ERROR", SWT.ICON_ERROR);
swtKeys.put("ICON_INFORMATION", SWT.ICON_INFORMATION);
swtKeys.put("ICON_QUESTION", SWT.ICON_QUESTION);
swtKeys.put("ICON_WARNING", SWT.ICON_WARNING);
swtKeys.put("ICON_WORKING", SWT.ICON_WORKING);
swtKeys.put("Iconify", SWT.Iconify);
swtKeys.put("IGNORE", SWT.IGNORE);
swtKeys.put("IMAGE_BMP", SWT.IMAGE_BMP);
swtKeys.put("IMAGE_BMP_RLE", SWT.IMAGE_BMP_RLE);
swtKeys.put("IMAGE_COPY", SWT.IMAGE_COPY);
swtKeys.put("IMAGE_DISABLE", SWT.IMAGE_DISABLE);
swtKeys.put("IMAGE_GIF", SWT.IMAGE_GIF);
swtKeys.put("IMAGE_GRAY", SWT.IMAGE_GRAY);
swtKeys.put("IMAGE_ICO", SWT.IMAGE_ICO);
swtKeys.put("IMAGE_JPEG", SWT.IMAGE_JPEG);
swtKeys.put("IMAGE_OS2_BMP", SWT.IMAGE_OS2_BMP);
swtKeys.put("IMAGE_PNG", SWT.IMAGE_PNG);
swtKeys.put("IMAGE_TIFF", SWT.IMAGE_TIFF);
swtKeys.put("IMAGE_UNDEFINED", SWT.IMAGE_UNDEFINED);
swtKeys.put("ImeComposition", SWT.ImeComposition);
swtKeys.put("INDETERMINATE", SWT.INDETERMINATE);
swtKeys.put("INHERIT_DEFAULT", SWT.INHERIT_DEFAULT);
swtKeys.put("INHERIT_FORCE", SWT.INHERIT_FORCE);
swtKeys.put("INHERIT_NONE", SWT.INHERIT_NONE);
swtKeys.put("INSERT", SWT.INSERT);
swtKeys.put("ITALIC", SWT.ITALIC);
swtKeys.put("JOIN_BEVEL", SWT.JOIN_BEVEL);
swtKeys.put("JOIN_MITER", SWT.JOIN_MITER);
swtKeys.put("JOIN_ROUND", SWT.JOIN_ROUND);
swtKeys.put("KEY_MASK", SWT.KEY_MASK);
swtKeys.put("KEYCODE_BIT", SWT.KEYCODE_BIT);
swtKeys.put("KeyDown", SWT.KeyDown);
swtKeys.put("KEYPAD_0", SWT.KEYPAD_0);
swtKeys.put("KEYPAD_1", SWT.KEYPAD_1);
swtKeys.put("KEYPAD_2", SWT.KEYPAD_2);
swtKeys.put("KEYPAD_3", SWT.KEYPAD_3);
swtKeys.put("KEYPAD_4", SWT.KEYPAD_4);
swtKeys.put("KEYPAD_5", SWT.KEYPAD_5);
swtKeys.put("KEYPAD_6", SWT.KEYPAD_6);
swtKeys.put("KEYPAD_7", SWT.KEYPAD_7);
swtKeys.put("KEYPAD_8", SWT.KEYPAD_8);
swtKeys.put("KEYPAD_9", SWT.KEYPAD_9);
swtKeys.put("KEYPAD_ADD", SWT.KEYPAD_ADD);
swtKeys.put("KEYPAD_CR", SWT.KEYPAD_CR);
swtKeys.put("KEYPAD_DECIMAL", SWT.KEYPAD_DECIMAL);
swtKeys.put("KEYPAD_DIVIDE", SWT.KEYPAD_DIVIDE);
swtKeys.put("KEYPAD_EQUAL", SWT.KEYPAD_EQUAL);
swtKeys.put("KEYPAD_MULTIPLY", SWT.KEYPAD_MULTIPLY);
swtKeys.put("KEYPAD_SUBTRACT", SWT.KEYPAD_SUBTRACT);
swtKeys.put("KeyUp", SWT.KeyUp);
swtKeys.put("LAST_LINE_SELECTION", SWT.LAST_LINE_SELECTION);
swtKeys.put("LEAD", SWT.LEAD);
swtKeys.put("LEFT", SWT.LEFT);
swtKeys.put("LEFT_TO_RIGHT", SWT.LEFT_TO_RIGHT);
swtKeys.put("LF", (int) SWT.LF);
swtKeys.put("LINE_CUSTOM", SWT.LINE_CUSTOM);
swtKeys.put("LINE_DASH", SWT.LINE_DASH);
swtKeys.put("LINE_DASHDOT", SWT.LINE_DASHDOT);
swtKeys.put("LINE_DASHDOTDOT", SWT.LINE_DASHDOTDOT);
swtKeys.put("LINE_DOT", SWT.LINE_DOT);
swtKeys.put("LINE_SOLID", SWT.LINE_SOLID);
swtKeys.put("LONG", SWT.LONG);
swtKeys.put("LOW", SWT.LOW);
swtKeys.put("MAX", SWT.MAX);
swtKeys.put("MeasureItem", SWT.MeasureItem);
swtKeys.put("MEDIUM", SWT.MEDIUM);
swtKeys.put("MENU", SWT.MENU);
swtKeys.put("MenuDetect", SWT.MenuDetect);
swtKeys.put("MIN", SWT.MIN);
swtKeys.put("MIRRORED", SWT.MIRRORED);
swtKeys.put("MOD1", SWT.MOD1);
swtKeys.put("MOD2", SWT.MOD2);
swtKeys.put("MOD3", SWT.MOD3);
swtKeys.put("MOD4", SWT.MOD4);
swtKeys.put("MODELESS", SWT.MODELESS);
swtKeys.put("MODIFIER_MASK", SWT.MODIFIER_MASK);
swtKeys.put("Modify", SWT.Modify);
swtKeys.put("MouseDoubleClick", SWT.MouseDoubleClick);
swtKeys.put("MouseDown", SWT.MouseDown);
swtKeys.put("MouseEnter", SWT.MouseEnter);
swtKeys.put("MouseExit", SWT.MouseExit);
swtKeys.put("MouseHover", SWT.MouseHover);
swtKeys.put("MouseMove", SWT.MouseMove);
swtKeys.put("MouseUp", SWT.MouseUp);
swtKeys.put("MouseWheel", SWT.MouseWheel);
swtKeys.put("Move", SWT.Move);
swtKeys.put("MOVEMENT_CHAR", SWT.MOVEMENT_CHAR);
swtKeys.put("MOVEMENT_CLUSTER", SWT.MOVEMENT_CLUSTER);
swtKeys.put("MOVEMENT_WORD", SWT.MOVEMENT_WORD);
swtKeys.put("MOVEMENT_WORD_END", SWT.MOVEMENT_WORD_END);
swtKeys.put("MOVEMENT_WORD_START", SWT.MOVEMENT_WORD_START);
swtKeys.put("MOZILLA", SWT.MOZILLA);
swtKeys.put("MULTI", SWT.MULTI);
swtKeys.put("NATIVE", SWT.NATIVE);
swtKeys.put("NO", SWT.NO);
swtKeys.put("NO_BACKGROUND", SWT.NO_BACKGROUND);
swtKeys.put("NO_FOCUS", SWT.NO_FOCUS);
swtKeys.put("NO_MERGE_PAINTS", SWT.NO_MERGE_PAINTS);
swtKeys.put("NO_RADIO_GROUP", SWT.NO_RADIO_GROUP);
swtKeys.put("NO_REDRAW_RESIZE", SWT.NO_REDRAW_RESIZE);
swtKeys.put("NO_SCROLL", SWT.NO_SCROLL);
swtKeys.put("NO_TRIM", SWT.NO_TRIM);
swtKeys.put("None", SWT.None);
swtKeys.put("NONE", SWT.NONE);
swtKeys.put("NORMAL", SWT.NORMAL);
swtKeys.put("NULL", SWT.NULL);
swtKeys.put("NUM_LOCK", SWT.NUM_LOCK);
swtKeys.put("OFF", SWT.OFF);
swtKeys.put("OK", SWT.OK);
swtKeys.put("ON", SWT.ON);
swtKeys.put("ON_TOP", SWT.ON_TOP);
swtKeys.put("OPEN", SWT.OPEN);
swtKeys.put("PAGE_DOWN", SWT.PAGE_DOWN);
swtKeys.put("PAGE_UP", SWT.PAGE_UP);
swtKeys.put("Paint", SWT.Paint);
swtKeys.put("PaintItem", SWT.PaintItem);
swtKeys.put("PASSWORD", SWT.PASSWORD);
swtKeys.put("PATH_CLOSE", SWT.PATH_CLOSE);
swtKeys.put("PATH_CUBIC_TO", SWT.PATH_CUBIC_TO);
swtKeys.put("PATH_LINE_TO", SWT.PATH_LINE_TO);
swtKeys.put("PATH_MOVE_TO", SWT.PATH_MOVE_TO);
swtKeys.put("PATH_QUAD_TO", SWT.PATH_QUAD_TO);
swtKeys.put("PAUSE", SWT.PAUSE);
swtKeys.put("PAUSED", SWT.PAUSED);
swtKeys.put("PHONETIC", SWT.PHONETIC);
swtKeys.put("POP_UP", SWT.POP_UP);
swtKeys.put("PRIMARY_MODAL", SWT.PRIMARY_MODAL);
swtKeys.put("PRINT_SCREEN", SWT.PRINT_SCREEN);
swtKeys.put("PUSH", SWT.PUSH);
swtKeys.put("RADIO", SWT.RADIO);
swtKeys.put("READ_ONLY", SWT.READ_ONLY);
swtKeys.put("Resize", SWT.Resize);
swtKeys.put("RESIZE", SWT.RESIZE);
swtKeys.put("RETRY", SWT.RETRY);
swtKeys.put("RIGHT", SWT.RIGHT);
swtKeys.put("RIGHT_TO_LEFT", SWT.RIGHT_TO_LEFT);
swtKeys.put("ROMAN", SWT.ROMAN);
swtKeys.put("SAVE", SWT.SAVE);
swtKeys.put("SCROLL_LINE", SWT.SCROLL_LINE);
swtKeys.put("SCROLL_LOCK", SWT.SCROLL_LOCK);
swtKeys.put("SCROLL_PAGE", SWT.SCROLL_PAGE);
swtKeys.put("SEARCH", SWT.SEARCH);
swtKeys.put("SELECTED", SWT.SELECTED);
swtKeys.put("Selection", SWT.Selection);
swtKeys.put("SEPARATOR", SWT.SEPARATOR);
swtKeys.put("SetData", SWT.SetData);
swtKeys.put("Settings", SWT.Settings);
swtKeys.put("SHADOW_ETCHED_IN", SWT.SHADOW_ETCHED_IN);
swtKeys.put("SHADOW_ETCHED_OUT", SWT.SHADOW_ETCHED_OUT);
swtKeys.put("SHADOW_IN", SWT.SHADOW_IN);
swtKeys.put("SHADOW_NONE", SWT.SHADOW_NONE);
swtKeys.put("SHADOW_OUT", SWT.SHADOW_OUT);
swtKeys.put("SHELL_TRIM", SWT.SHELL_TRIM);
swtKeys.put("SHIFT", SWT.SHIFT);
swtKeys.put("SHORT", SWT.SHORT);
swtKeys.put("Show", SWT.Show);
swtKeys.put("SIMPLE", SWT.SIMPLE);
swtKeys.put("SINGLE", SWT.SINGLE);
swtKeys.put("SMOOTH", SWT.SMOOTH);
swtKeys.put("SYSTEM_MODAL", SWT.SYSTEM_MODAL);
swtKeys.put("TAB", (int) SWT.TAB);
swtKeys.put("TIME", SWT.TIME);
swtKeys.put("TITLE", SWT.TITLE);
swtKeys.put("TOGGLE", SWT.TOGGLE);
swtKeys.put("TOOL", SWT.TOOL);
swtKeys.put("TOP", SWT.TOP);
swtKeys.put("TRAIL", SWT.TRAIL);
swtKeys.put("TRANSPARENCY_ALPHA", SWT.TRANSPARENCY_ALPHA);
swtKeys.put("TRANSPARENCY_MASK", SWT.TRANSPARENCY_MASK);
swtKeys.put("TRANSPARENCY_NONE", SWT.TRANSPARENCY_NONE);
swtKeys.put("TRANSPARENCY_PIXEL", SWT.TRANSPARENCY_PIXEL);
swtKeys.put("TRANSPARENT", SWT.TRANSPARENT);
swtKeys.put("Traverse", SWT.Traverse);
swtKeys.put("TRAVERSE_ARROW_NEXT", SWT.TRAVERSE_ARROW_NEXT);
swtKeys.put("TRAVERSE_ARROW_PREVIOUS", SWT.TRAVERSE_ARROW_PREVIOUS);
swtKeys.put("TRAVERSE_ESCAPE", SWT.TRAVERSE_ESCAPE);
swtKeys.put("TRAVERSE_MNEMONIC", SWT.TRAVERSE_MNEMONIC);
swtKeys.put("TRAVERSE_NONE", SWT.TRAVERSE_NONE);
swtKeys.put("TRAVERSE_PAGE_NEXT", SWT.TRAVERSE_PAGE_NEXT);
swtKeys.put("TRAVERSE_PAGE_PREVIOUS", SWT.TRAVERSE_PAGE_PREVIOUS);
swtKeys.put("TRAVERSE_RETURN", SWT.TRAVERSE_RETURN);
swtKeys.put("TRAVERSE_TAB_NEXT", SWT.TRAVERSE_TAB_NEXT);
swtKeys.put("TRAVERSE_TAB_PREVIOUS", SWT.TRAVERSE_TAB_PREVIOUS);
swtKeys.put("UNDERLINE_DOUBLE", SWT.UNDERLINE_DOUBLE);
swtKeys.put("UNDERLINE_ERROR", SWT.UNDERLINE_ERROR);
swtKeys.put("UNDERLINE_SINGLE", SWT.UNDERLINE_SINGLE);
swtKeys.put("UNDERLINE_SQUIGGLE", SWT.UNDERLINE_SQUIGGLE);
swtKeys.put("UP", SWT.UP);
swtKeys.put("V_SCROLL", SWT.V_SCROLL);
swtKeys.put("Verify", SWT.Verify);
swtKeys.put("VERTICAL", SWT.VERTICAL);
swtKeys.put("VIRTUAL", SWT.VIRTUAL);
swtKeys.put("WRAP", SWT.WRAP);
swtKeys.put("YES", SWT.YES);
}
public static int getSWT(String name){
Integer v = swtKeys.get(name);
if(v != null){
return v.intValue();
}else{
return 0;
}
}
public static Set getSWTKeys(){
return swtKeys.keySet();
}
public static void addDisposeViewer(Widget parent, final String name){
parent.addDisposeListener(new DisposeListener(){
public void widgetDisposed(DisposeEvent event) {
log.info(name + ":" + event.widget + " is disposed!");
}
});
}
public static void addDisposeData(Widget parent, String name, Widget widget){
ResourceDisposeListener listener = (ResourceDisposeListener) parent.getData("_disposer");
if(listener == null){
listener = new ResourceDisposeListener();
parent.setData("_disposer", listener);
}
listener.add(name, widget);
}
/**
* 根据一个控件的位置和大小设置弹出窗口的位置。
*
* @param shell 要弹出的窗口
* @param relateLocation 控件的location
* @param relateSize 控件的大小
*/
public static void setShellRelateLocation(Shell shell, Point relateLocation, Point relateSize){
Point point = relateLocation;
Rectangle shellRect = shell.getBounds();
Monitor monitor = shell.getMonitor();
int clientHeight = monitor.getClientArea().height;
int clientWidth = monitor.getClientArea().width;
int width = shellRect.width;
int height = shellRect.height;
Point x = getRelateWidth(width, clientWidth, point.x, point.x + relateSize.x);
Point y = getRelateHeight(height, clientHeight, point.y, point.y + relateSize.y);
shell.setLocation(x.y, y.y);
shell.setSize(x.x, y.x);
}
public static Point getRelateWidth(int width, int clientWidth, int x1, int x2){
int w1 = clientWidth - width - x1;
int w2 = x2 - width;
if(w1 >= 0){
return new Point(width, x1);
}else if(w2 >= 0){
return new Point(width, x2 - width);
}else if(w1 > w2){
return new Point(width + w1, x1);
}else{
return new Point(width + w2, 0);
}
}
public static Point getRelateHeight(int height, int clientHeight, int y1 , int y2){
int w1 = clientHeight - y2 - height;
int w2 = y1 - height;
if(w1 >= 0){
//下面可以显示
return new Point(height, y2);
}else if(w2 >= 0){
//上面可以显示
return new Point(height, y1 - height);
}else if(w1 > w2){
//下面优先显示
return new Point(height + w1, y2);
}else{
//上面优先显示
return new Point(height + w2, 0);
}
}
/**
* 居中一个Shell。
*
* @param shell
*/
public static void centerShell(Shell shell){
Display display = Display.getCurrent();
Rectangle rec = display.getClientArea();
Rectangle srec = shell.getBounds();
int x = rec.width / 2 - srec.width / 2;
int y = rec.height / 2 - srec.height / 2;
shell.setLocation (new Point(x, y));
}
public static Dialog createDialog(final Shell shell, final ActionContext actionContext){
return new Dialog(shell){
@SuppressWarnings("unused")
public Object open(){
shell.open();
final Display display = shell.getDisplay();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) display.sleep();
}
return actionContext.get("result");
}
};
}
/**
* 获得加速键,如Shift+X.
*
* @param acceleratorStr
* @return
*/
public static int getAccelerator(String acceleratorStr){
if(acceleratorStr == null || "".equals(acceleratorStr)) return 0;
String[] s = acceleratorStr.split("[+]");
int value = 0;
for(int i=0; i data = new HashMap();
data.put("value", value);
dataControl.doAction("setValue", new ActionContext(), data);
}else if(control instanceof Combo){
Combo combo = (Combo) control;
if(combo.getData() instanceof List){
List datas = (List) combo.getData();
for(int i=0; i params = new HashMap();
params.put("type", type);
params.put("pattern", pattern);
return ac.doAction("getValue", params);
}
if(!(control instanceof Widget)){
//如果control不是swt控件,那么可能就是数据本身,直接返回
return control;
}
if(control instanceof Text || control instanceof StyledText){
if(control instanceof Text){
Text t = (Text) control;
return UtilData.parse(t.getText().trim(), type, pattern);
}else if(control instanceof StyledText){
StyledText t = (StyledText) control;
return UtilData.parse(t.getText().trim(), type, pattern);
}
}else if(control instanceof Label){
Label l = (Label) control;
return UtilData.parse(l.getText().trim(), type, pattern);
}else if(control instanceof Button){
Button b = (Button) control;
if(b.getData("selected") != null && b.getSelection()){
return b.getData("selected");
}
if(b.getData("unSelected") != null && !b.getSelection()){
return b.getData("unSelected");
}
if("boolean".equals(type)){
if(b.getSelection()){
return new Boolean(true);
}else{
return new Boolean(false);
}
}else{
if(b.getSelection()){
return b.getData();
}else{
return null;
}
}
}else if(control instanceof DateTime){
DateTime d = (DateTime) control;
return new GregorianCalendar(d.getYear(), d.getMonth(), d.getDay()).getTime();
//String time = d.getYear() + "-" + (d.getMonth()+1) + "-" + d.getDay();
//return UtilData.parse(time, type, pattern);
}else if(control instanceof CCombo || control instanceof Combo){
//下拉选择
if(control instanceof CCombo){
CCombo combo = (CCombo) control;
/*
if((combo.getStyle() & SWT.READ_ONLY) == 0){
//如果是可以修改内容的,那么返回的应该是字符串
return combo.getText();
}else{
*/
int index = combo.getSelectionIndex();
if(index != -1){
Object data = combo.getData();
if(!(data instanceof List)){
return null;
}else{
List datas = (List) data;
if(index < datas.size()){
return datas.get(index);
}else{
return null;
}
}
}else{
return combo.getText();
}
}else if(control instanceof Combo){
Combo combo = (Combo) control;
if((combo.getStyle() & SWT.READ_ONLY) == 0){
//如果是可以修改内容的,那么返回的应该是字符串
return combo.getText();
}else{
int index = combo.getSelectionIndex();
if(index != -1){
Object data = combo.getData();
if(!(data instanceof List)){
return null;
}else{
List datas = (List) data;
if(index < datas.size()){
return datas.get(index);
}else{
return null;
}
}
}else{
return null;
}
}
}
}else if(control instanceof Composite){
//如果控件是Composite,那么可能是单项选择或者多项选择,此时composite下都应该是Button
Composite composite = (Composite) control;
Control[] controls = composite.getChildren();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy