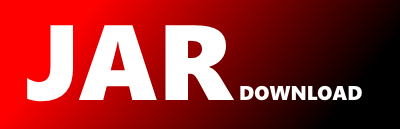
xworker.swt.util.UtilBrowser Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2007-2013 See AUTHORS file.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package xworker.swt.util;
import java.io.File;
import java.net.URLDecoder;
import java.util.HashMap;
import java.util.Map;
import org.eclipse.swt.SWT;
import org.eclipse.swt.browser.Browser;
import org.eclipse.swt.browser.StatusTextEvent;
import org.eclipse.swt.browser.StatusTextListener;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xmeta.ActionContext;
import org.xmeta.Thing;
import org.xmeta.World;
import xworker.swt.ActionContainer;
import xworker.swt.design.Designer;
/**
* 浏览器工具类,为浏览器中的网页提供一些交互的能力,比如点击一个连接打开编辑的事物等。
*
* 具体文档可以参看事物xworker.swt.util.UtilBrowser。
*
* 如果绑定了一个浏览器,且浏览器的页面引入了/js/xworker/InnerBrowserUtil.js脚本,
* 那么就可以使用invoke('aaa:bbb?ccc=ddd&eee=fff')或xw_invoke(('aaa:bbb?ccc=ddd&eee=fff')的方式调用UtilBrowser了。
*其中aaa是方法名,bbb是主参数,ccc和eee等是附加参数,ddd和fff等是对应的附加参数的值。
* 如:thing:xworker.core.project.Project意思是打开并且编辑xworker.core.project.Project这个事物。
*
* @author zyx
*
*/
public class UtilBrowser implements StatusTextListener{
private static Logger logger = LoggerFactory.getLogger(UtilBrowser.class);
ActionContext actionContext;
ActionContainer actions;
Display display;
Browser browser;
Thing utilBrowserThing;
/** -1 表示还未判断,0 表示不是, 1 表示是 */
private static byte isWebKit = -1;
public static void attach(Browser browser, ActionContainer actions, Display display){
UtilBrowser ub = new UtilBrowser(display, actions);
ub.attach(browser);
}
public static void attach(Browser browser, ActionContext actionContext){
UtilBrowser ub = new UtilBrowser(browser.getDisplay(), actionContext);
ub.attach(browser);
}
public static synchronized boolean isWebKit(){
if(isWebKit == -1){
new Thread(new Runnable(){
public void run(){
Display display = new Display();
Shell shell = new Shell(display);
Browser browser = new Browser(shell, SWT.NONE);
try{
browser.setUrl(new File(World.getInstance().getPath() + "/webroot/js/xworker/InnerBrowserUtil.js").toURI().toString());
Object value = browser.evaluate("var UA = navigator.userAgent.toLowerCase();return /webkit/i.test(UA);");
if(value != null && "true".equals(value)){
isWebKit = 1;
}else{
isWebKit = 0;
}
}catch(Exception e){
}finally{
display.dispose();
}
}
}).start();
int count = 0;
while(isWebKit == -1){
try {
Thread.sleep(100);
} catch (InterruptedException e) {
}
count++;
if(count > 20){
break;
}
}
}
return isWebKit == 1;
}
/**
* 一般是编辑器(IDE)的构造方法,传入处理openThing,thing,url,tab等编辑相关的默认方法。
*
* @param display
* @param actions
*/
public UtilBrowser(Display display, ActionContainer actions){
this.actions = actions;
this.display = display;
}
/**
* 一般的构造方法,用于非编辑器的场合。
*
* @param display
* @param actionContext
*/
public UtilBrowser(Display display, ActionContext actionContext){
this.actions = null;
this.actionContext = actionContext;
this.display = display;
}
/**
* 一般用于SWT创建时绑定到browser的构造方法,用于UtilBrowser事物的create方法中。
*
* @param utilBrowserThing
* @param display
* @param actionContext
*/
public UtilBrowser(Thing utilBrowserThing, Display display, ActionContext actionContext){
this.actions = null;
this.actionContext = actionContext;
this.display = display;
this.utilBrowserThing = utilBrowserThing;
}
public void attach(Browser browser){
browser.addStatusTextListener(this);
this.browser = browser;
try{
//swt 3.5
UtilBrowserJavaScriptFunction.attach(this);
}catch(Throwable t){
}
}
public void handle(String message){
//通过?号后可以附加参数,同url的规则
Map params = new HashMap();
int index = message.indexOf("?");
if(index != -1){
String pstr = message.substring(index + 1, message.length());
message = message.substring(0, index);
for(String str : pstr.split("[&]")){
String[] ss = str.split("[=]");
try {
if(ss.length > 1){
params.put(URLDecoder.decode(ss[0], "UTF-8"), URLDecoder.decode(ss[1], "UTF-8"));
}
} catch (Exception e) {
}
}
}
//params.put("browser", browser);
params.put("utilBrowser", this);
if(message != null && !"".equals(message)){
if(message.startsWith("openThing:")){
//开打事物一个事物编辑
String thingName = message.substring(10, message.length());
Thing thing = World.getInstance().getThing(thingName);
params.put("thing", thing);
runExplorerAction("openThing", params);
}else if(message.startsWith("thing:")){
//打开一个事物编辑,同openThing
String thingName = message.substring(6, message.length());
Thing thing = World.getInstance().getThing(thingName);
params.put("thing", thing);
runExplorerAction("openThing", params);
}else if(message.startsWith("tab:")){
//在编辑器中打开一个tab
String tabUrl = message.substring(4, message.length());
String compositeThingPath = getBaseUrl(tabUrl);
Thing compositeThing = World.getInstance().getThing(compositeThingPath);
params.put("compositeThing", compositeThing);
params.put("path", compositeThingPath);
params.put("title", compositeThing.getMetadata().getLabel());
runExplorerAction("openTab", params);
}else if(message.startsWith("url:")){
//在编辑器中打开一个url
String url = message.substring(4, message.length());
params.put("url", url);
params.put("name", url);
runExplorerAction("openUrl", params);
}else if(message.startsWith("action:")){
//在编辑器的环境中执行一个指定的动作
String thingName = message.substring(7, message.length());
Thing thing = World.getInstance().getThing(thingName);
params.put("action", thing.getAction());
if(thing != null){
runExplorerAction("doAction", params);
}
}else if(message.startsWith("run:")){
//在UtilBrowser绑定的环境下执行一个指定的动作,和action的差别是执行的环境不同
String thingName = message.substring(4, message.length());
Thing thing = World.getInstance().getThing(thingName);
if(thing != null){
ActionContext ac = actionContext;
if(ac == null || actions != null){
ac = actions.getActionContext();
}
thing.getAction().run(ac, params);
}
}else if(message.startsWith("runThing:")){
//在UtilBrowser绑定的环境下执行一个指定的动作,和action的差别是执行的环境不同
String method = "run";
String thingName = message.substring(9, message.length());
String ss[] = thingName.split("[:]");
if(ss.length > 1){
thingName = ss[0];
method = ss[1];
}
Thing thing = World.getInstance().getThing(thingName);
if(thing != null){
ActionContext ac = actionContext;
if(ac == null || actions != null){
ac = actions.getActionContext();
}
thing.doAction(method, ac, params);
}else{
logger.warn("UtilBroser: thing is null, path=" + thingName);
}
}else if(message.startsWith("webkit:")){
String webkit = message.substring(7, message.length());
if("true".equals(webkit)){
isWebKit = 1;
}else{
isWebKit = 0;
}
}else{
//默认的情况使用xworker.swt.util.UtilBrowser负责执行
int index1 = message.indexOf(":");
if(index1 != -1){
String prefix = message.substring(0, index1);
String path = message.substring(index1 + 1, message.length());
Thing uthing = utilBrowserThing;
if(uthing == null){
uthing = World.getInstance().getThing("xworker.swt.util.UtilBrowser");
}
if(uthing != null){
ActionContext ac = actionContext;
if(ac == null && actions != null){
ac = actions.getActionContext();
}
if(ac == null){
ac = new ActionContext();
ac.put("utilBrowser", this);
ac.put("path", path);
}
params.put("path", path);
params.put("utilBrowser", this);
uthing.doAction(prefix, ac, params);
}
}else{
if(utilBrowserThing != null){
utilBrowserThing.doAction("message", actionContext);
}
}
}
}
}
public void changed(StatusTextEvent event) {
if(actions == null){
return;
}
String message = event.text;
//System.out.println(message);
handle(message);
}
public String getBaseUrl(String str){
int index = str.indexOf("?");
if(index != -1){
return str.substring(0, index);
}else{
return str;
}
}
public Map getParameters(String str){
Map params = new HashMap();
String str1 = str;
int index = str.indexOf("?");
if(index != -1){
str1 = str.substring(index + 1, str.length());
}
String[] ps = str1.split("[&]");
for(int i=0; i objsToMap(Object[] objs){
Map m = new HashMap();
for(int i=0; i parameters){
Display explorerDisplay = Designer.getExplorerDisplay();
ActionContainer explorerActions = Designer.getExplorerActions();
if(explorerDisplay != null && explorerActions != null){
explorerDisplay.asyncExec(new Run(name, parameters, explorerActions));
}else{
runAction(name, parameters);
}
}
public void runAction(String name, Map parameters){
if(parameters != null){
parameters.put("browser", browser);
}
if(display == null){
actions.doAction(name, parameters);
}else{
display.asyncExec(new Run(name, parameters, actions));
}
}
public static class Run implements Runnable{
ActionContainer actions;
String name;
Map parameters;
public Run(String name, Map parameters, ActionContainer actions){
this.name = name;
this.parameters = parameters;
this.actions = actions;
}
public void run(){
ActionContext ac = actions.getActionContext();
Shell shell = (Shell) ac.get("shell");
if(shell != null){
shell.forceActive();
}
actions.doAction(name, parameters);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy