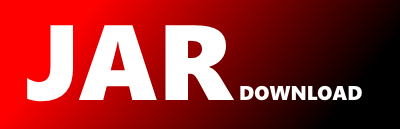
xworker.swt.xwidgets.QuickMenu Maven / Gradle / Ivy
package xworker.swt.xwidgets;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.events.SelectionListener;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.widgets.Control;
import org.eclipse.swt.widgets.Menu;
import org.eclipse.swt.widgets.MenuItem;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.TreeItem;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xmeta.ActionContext;
import org.xmeta.Thing;
import xworker.swt.ActionContainer;
import xworker.swt.util.ResourceManager;
import xworker.swt.util.SwtUtils;
public class QuickMenu {
private static Logger logger = LoggerFactory.getLogger(QuickMenu.class);
private static SelectionListener listener = new SelectionListener(){
@Override
public void widgetDefaultSelected(SelectionEvent event) {
}
@Override
public void widgetSelected(SelectionEvent event) {
Thing thing = (Thing) event.widget.getData();
ActionContext actionContext = (ActionContext) event.widget.getData("actionContext");
try{
String actionContainer = thing.getStringBlankAsNull("actionContainer");
String actionName = thing.getStringBlankAsNull("actionName");
if(actionName != null && actionContainer != null){
ActionContainer ac = (ActionContainer) actionContext.get(actionContainer);
if(ac != null){
ac.doAction(actionName, actionContext);
}
}else{
thing.doAction("run", actionContext);
}
}catch(Exception e){
logger.warn("QuickMenu selection error, path=" + thing.getMetadata().getPath(), e);
}
}
};
Map items = new HashMap();
public TreeItem getItem(String name){
return items.get(name);
}
public void putItem(String name, TreeItem item){
items.put(name, item);
}
public static void createQuickMenu(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
Object parent = actionContext.get("parent");
if(parent instanceof Shell){
Shell shell = (Shell) parent;
Menu menu = new Menu(shell, SWT.BAR);
shell.setMenuBar(menu);
actionContext.peek().put("menu", menu);
}else{
Menu menu = new Menu((Control) parent);
((Control) parent).setMenu(menu);
actionContext.peek().put("menu", menu);
}
for(Thing child : self.getChilds("Menu")){
child.doAction("create", actionContext);
}
}
public static void createMenuItem(ActionContext actionContext){
Thing self = (Thing) actionContext.get("self");
Menu menu = (Menu) actionContext.get("menu");
MenuItem item = null;
List childs = self.getChilds("Menu");
if(childs.size() > 0){
item = new MenuItem(menu, SWT.CASCADE);
}else if(self.getBoolean("seperator")){
item = new MenuItem(menu, SWT.SEPARATOR);
}else{
item = new MenuItem(menu, SWT.PUSH);
}
item.setText(self.getMetadata().getLabel());
item.setData(self);
item.setData("actionContext", actionContext);
item.addSelectionListener(listener);
String icon = self.getStringBlankAsNull("icon");
if(icon != null){
Image image = (Image) ResourceManager.createIamge(icon, actionContext);
if(image != null){
item.setImage(image);
}
}
String accelerator = self.getStringBlankAsNull("accelerator");
if(accelerator != null){
item.setAccelerator(SwtUtils.getAccelerator(accelerator));
}
if(childs.size() > 0){
Menu childMenu = new Menu(item);
item.setMenu(childMenu);
actionContext.peek().put("menu", childMenu);
for(Thing child : childs){
child.doAction("create", actionContext);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy