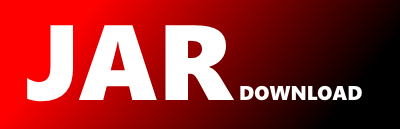
yakworks.etl.csv.CSVPathKeyMapReader.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gorm-etl Show documentation
Show all versions of gorm-etl Show documentation
gorm-tools CSV reader helpers
/*
* Copyright 2021 Yak.Works - Licensed under the Apache License, Version 2.0 (the "License")
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
*/
package yakworks.etl.csv
import groovy.transform.CompileStatic
import groovy.transform.builder.Builder
import groovy.transform.builder.SimpleStrategy
import com.opencsv.CSVReaderHeaderAware
import yakworks.commons.map.LazyPathKeyMap
import yakworks.commons.map.Maps
/**
* Overrides the CSVReaderHeaderAware to read csv rows into the LazyPathKeyMap
* which can then be used for the EntityMapBinder
*/
@Builder(builderStrategy = SimpleStrategy, prefix = "")
@CompileStatic
class CSVPathKeyMapReader extends CSVReaderHeaderAware {
/**
* the pathDelimiter is used when the headers are useing somthing like _ insted of dots
* for the nested paths
*/
String pathDelimiter = "."
/**
* true(default) to prune out any null or empty string fields from map,
*/
boolean prune = true
/**
* Constructor with supplied reader.
*
* @param reader The reader to an underlying CSV source
*/
CSVPathKeyMapReader(Reader reader) {
super(reader)
}
/**
* CSVPathKeyMapReader.of(reader).pathDelimiter('_')
*/
static CSVPathKeyMapReader of(Reader reader) {
new CSVPathKeyMapReader(reader)
}
static CSVPathKeyMapReader of(File file) {
return of(new FileReader(file))
}
@Override
Map readMap() {
Map data = super.readMap()
data = prune && data ? Maps.prune(data) : data
// data = data as Map
return LazyPathKeyMap.of(data as Map, pathDelimiter)
}
/**
* Read all the rows in CSV, we can't override the readAll in CVSReader as is return list of string array
*
* @return the list of maps for entire file
*/
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy