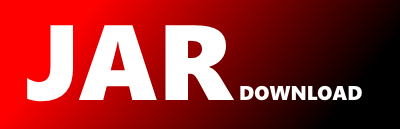
yakworks.etl.excel.ExcelBuilder.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gorm-etl Show documentation
Show all versions of gorm-etl Show documentation
gorm-tools CSV reader helpers
/*
* Copyright 2021 Yak.Works - Licensed under the Apache License, Version 2.0 (the "License")
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
*/
package yakworks.etl.excel
import java.time.LocalDate
import java.time.LocalDateTime
import java.time.temporal.ChronoUnit
import java.util.regex.Matcher
import java.util.regex.Pattern
import groovy.transform.CompileStatic
import groovy.transform.builder.Builder
import groovy.transform.builder.SimpleStrategy
import groovy.util.logging.Slf4j
import org.apache.poi.ss.usermodel.BorderStyle
import org.apache.poi.ss.usermodel.BuiltinFormats
import org.apache.poi.ss.usermodel.Cell
import org.apache.poi.ss.usermodel.CreationHelper
import org.apache.poi.ss.usermodel.DataFormat
import org.apache.poi.ss.usermodel.FillPatternType
import org.apache.poi.ss.usermodel.HorizontalAlignment
import org.apache.poi.ss.usermodel.Row
import org.apache.poi.xssf.usermodel.DefaultIndexedColorMap
import org.apache.poi.xssf.usermodel.XSSFCellStyle
import org.apache.poi.xssf.usermodel.XSSFColor
import org.apache.poi.xssf.usermodel.XSSFFont
import org.apache.poi.xssf.usermodel.XSSFRow
import org.apache.poi.xssf.usermodel.XSSFSheet
import org.apache.poi.xssf.usermodel.XSSFWorkbook
import yakworks.commons.beans.PropertyTools
import yakworks.commons.map.MapFlattener
import yakworks.meta.MetaMapList
import yakworks.meta.MetaProp
/**
* Builder to wrap POI and use our intelligent defaults.
* Has support for the MetaEntity and MetaListMap for building and getting headers and types.
* NOT THREAD SAFE, should have one instance of this for a thread.
*/
@Builder(builderStrategy= SimpleStrategy, prefix="", includes=['includes', 'headerType', 'headers', 'outputStream'])
@SuppressWarnings(['NestedBlockDepth'])
@CompileStatic
@Slf4j
class ExcelBuilder {
public static final String SHEET_NAME = "data"
public static final DefaultIndexedColorMap INDEXED_COLOR_MAP = new DefaultIndexedColorMap()
/** the property keys for the data, can be dot notations, such as 'id', 'num', 'org.name' etc.. */
List includes
/**
* use the key specified to look in the restconfig, should be a pointer to a list/array of column configs with key and label
* will use the column config found to build the includes and headers
*/
String configKey
/**
* header options
* - null or false : means dont show header
* - includes : use the includes path keys for the header
* - labels : will build the labels from config or the nameUtils
* - columns : uses the key specified to look up in config for the entity. grid
*/
String headerType = 'labels'
/**
* header labels, index should match the includes. if specified will use it regardless of header setting
* if headerType=keys then this will match whats in includes
* if headerType=labels then will inteligently build this from
*/
List headers
XSSFWorkbook workbook
XSSFSheet sheet
CreationHelper createHelper
/** the stream to write to */
OutputStream outputStream
XSSFCellStyle styleHeader
XSSFCellStyle styleMoney
XSSFCellStyle styleLocalDate
XSSFCellStyle styleDateTime
/**
* builder helper to create with an outputStream
*/
static ExcelBuilder of(OutputStream outstream){
def eb = new ExcelBuilder()
eb.outputStream = outstream
return eb
}
/**
* creates the workbook and a sheet with default styles that can be used
*/
ExcelBuilder build(){
workbook = new XSSFWorkbook()
sheet = workbook.createSheet(SHEET_NAME)
createHelper = sheet.workbook.getCreationHelper()
initDefaultStyles()
return this
}
/**
* initializes the default styles
*/
void initDefaultStyles(){
//NUMBER MONEY
styleMoney = workbook.createCellStyle()
styleMoney.alignment = HorizontalAlignment.RIGHT
// cellStyle.setFont(font)
styleMoney.wrapText = true
DataFormat xformat = workbook.createDataFormat()
styleMoney.setDataFormat(xformat.getFormat("#,##0.00"))
//DATE
styleLocalDate = workbook.createCellStyle()
// DataFormat ldformat = sheet.workbook.createDataFormat()
// localDateStyle.setDataFormat(ldformat.getFormat("mm/dd/yyyy"))
styleLocalDate.setDataFormat((short)BuiltinFormats.getBuiltinFormat("m/d/yy"))
styleDateTime = workbook.createCellStyle()
styleDateTime.setDataFormat((short)BuiltinFormats.getBuiltinFormat("m/d/yy h:mm"))
//HEADERS Style
styleHeader = workbook.createCellStyle()
// headerStyle.setFillForegroundColor(IndexedColors.LIGHT_BLUE.getIndex())
styleHeader.setFillForegroundColor(parseColor("#F5F5F5"))
styleHeader.setFillPattern(FillPatternType.SOLID_FOREGROUND)
styleHeader.setBorderBottom(BorderStyle.MEDIUM)
styleHeader.dataFormatString
XSSFFont font = workbook.createFont()
// font.setFontName("Calibri")
font.setFontHeightInPoints((short) 12)
font.setBold(true)
styleHeader.setFont(font)
}
/**
* Writes the dataList a (collection of maps) to the active sheet.
* If dataList is a MetaMapList then it will use its metaEntity to get the headers.
* otherwise it creates the header using the the data in the first item of collection.
*
* @param dataList a List of Maps or a MetaMapList
*/
ExcelBuilder writeData(Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy