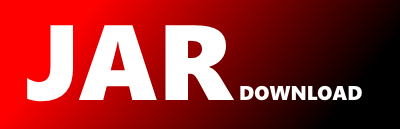
yakworks.spring.hazelcast.HazelCache.groovy Maven / Gradle / Ivy
/*
* Copyright 2008-2023 Yak.Works - Licensed under the Apache License, Version 2.0 (the "License")
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
*/
package yakworks.spring.hazelcast
import java.util.concurrent.Callable
import java.util.concurrent.TimeUnit
import javax.persistence.LockTimeoutException
import groovy.transform.CompileDynamic
import groovy.transform.CompileStatic
import groovy.util.logging.Slf4j
import org.springframework.cache.Cache
import com.hazelcast.map.IMap
import com.hazelcast.spring.cache.HazelcastCache
/**
* Spring related {@link Cache} implementation for Hazelcast.
*/
@SuppressWarnings(['UnnecessaryOverridingMethod'])
@Slf4j
@CompileStatic
public class HazelCache extends HazelcastCache {
/**
* Lock timeout for cache value retrieval operations.
*/
long lockTimeout
public HazelCache(IMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy