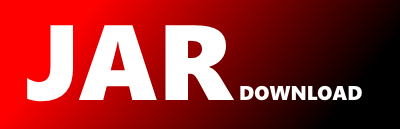
ccsds.sle.transfer.service.fsp.incoming.pdus.FspUserToProviderPdu Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsle Show documentation
Show all versions of jsle Show documentation
Java implementation for the SLE (Space Link Extension) protocol.
/**
* This class file was automatically generated by jASN1 v1.11.2 (http://www.beanit.com)
*/
package ccsds.sle.transfer.service.fsp.incoming.pdus;
import java.io.IOException;
import java.io.EOFException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.ArrayList;
import java.util.Iterator;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.io.Serializable;
import com.beanit.jasn1.ber.*;
import com.beanit.jasn1.ber.types.*;
import com.beanit.jasn1.ber.types.string.*;
import ccsds.sle.transfer.service.bind.types.SleBindInvocation;
import ccsds.sle.transfer.service.bind.types.SlePeerAbort;
import ccsds.sle.transfer.service.bind.types.SleUnbindInvocation;
import ccsds.sle.transfer.service.common.pdus.SleScheduleStatusReportInvocation;
import ccsds.sle.transfer.service.common.pdus.SleStopInvocation;
import ccsds.sle.transfer.service.common.types.Credentials;
import ccsds.sle.transfer.service.common.types.Duration;
import ccsds.sle.transfer.service.common.types.IntPosLong;
import ccsds.sle.transfer.service.common.types.IntPosShort;
import ccsds.sle.transfer.service.common.types.IntUnsignedLong;
import ccsds.sle.transfer.service.common.types.InvokeId;
import ccsds.sle.transfer.service.common.types.SlduStatusNotification;
import ccsds.sle.transfer.service.fsp.structures.BlockingUsage;
import ccsds.sle.transfer.service.fsp.structures.FspData;
import ccsds.sle.transfer.service.fsp.structures.FspParameterName;
import ccsds.sle.transfer.service.fsp.structures.Map;
import ccsds.sle.transfer.service.fsp.structures.MapMuxControl;
import ccsds.sle.transfer.service.fsp.structures.PacketIdentification;
import ccsds.sle.transfer.service.fsp.structures.ProductionTime;
import ccsds.sle.transfer.service.fsp.structures.TransmissionMode;
public class FspUserToProviderPdu implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public byte[] code = null;
private SleBindInvocation fspBindInvocation = null;
private SleUnbindInvocation fspUnbindInvocation = null;
private FspStartInvocation fspStartInvocation = null;
private SleStopInvocation fspStopInvocation = null;
private FspTransferDataInvocation fspTransferDataInvocation = null;
private SleScheduleStatusReportInvocation fspScheduleStatusReportInvocation = null;
private FspGetParameterInvocation fspGetParameterInvocation = null;
private FspThrowEventInvocation fspThrowEventInvocation = null;
private FspInvokeDirectiveInvocation fspInvokeDirectiveInvocation = null;
private SlePeerAbort fspPeerAbortInvocation = null;
public FspUserToProviderPdu() {
}
public FspUserToProviderPdu(byte[] code) {
this.code = code;
}
public void setFspBindInvocation(SleBindInvocation fspBindInvocation) {
this.fspBindInvocation = fspBindInvocation;
}
public SleBindInvocation getFspBindInvocation() {
return fspBindInvocation;
}
public void setFspUnbindInvocation(SleUnbindInvocation fspUnbindInvocation) {
this.fspUnbindInvocation = fspUnbindInvocation;
}
public SleUnbindInvocation getFspUnbindInvocation() {
return fspUnbindInvocation;
}
public void setFspStartInvocation(FspStartInvocation fspStartInvocation) {
this.fspStartInvocation = fspStartInvocation;
}
public FspStartInvocation getFspStartInvocation() {
return fspStartInvocation;
}
public void setFspStopInvocation(SleStopInvocation fspStopInvocation) {
this.fspStopInvocation = fspStopInvocation;
}
public SleStopInvocation getFspStopInvocation() {
return fspStopInvocation;
}
public void setFspTransferDataInvocation(FspTransferDataInvocation fspTransferDataInvocation) {
this.fspTransferDataInvocation = fspTransferDataInvocation;
}
public FspTransferDataInvocation getFspTransferDataInvocation() {
return fspTransferDataInvocation;
}
public void setFspScheduleStatusReportInvocation(SleScheduleStatusReportInvocation fspScheduleStatusReportInvocation) {
this.fspScheduleStatusReportInvocation = fspScheduleStatusReportInvocation;
}
public SleScheduleStatusReportInvocation getFspScheduleStatusReportInvocation() {
return fspScheduleStatusReportInvocation;
}
public void setFspGetParameterInvocation(FspGetParameterInvocation fspGetParameterInvocation) {
this.fspGetParameterInvocation = fspGetParameterInvocation;
}
public FspGetParameterInvocation getFspGetParameterInvocation() {
return fspGetParameterInvocation;
}
public void setFspThrowEventInvocation(FspThrowEventInvocation fspThrowEventInvocation) {
this.fspThrowEventInvocation = fspThrowEventInvocation;
}
public FspThrowEventInvocation getFspThrowEventInvocation() {
return fspThrowEventInvocation;
}
public void setFspInvokeDirectiveInvocation(FspInvokeDirectiveInvocation fspInvokeDirectiveInvocation) {
this.fspInvokeDirectiveInvocation = fspInvokeDirectiveInvocation;
}
public FspInvokeDirectiveInvocation getFspInvokeDirectiveInvocation() {
return fspInvokeDirectiveInvocation;
}
public void setFspPeerAbortInvocation(SlePeerAbort fspPeerAbortInvocation) {
this.fspPeerAbortInvocation = fspPeerAbortInvocation;
}
public SlePeerAbort getFspPeerAbortInvocation() {
return fspPeerAbortInvocation;
}
public int encode(OutputStream reverseOS) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
return code.length;
}
int codeLength = 0;
if (fspPeerAbortInvocation != null) {
codeLength += fspPeerAbortInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 104
reverseOS.write(0x68);
reverseOS.write(0x9F);
codeLength += 2;
return codeLength;
}
if (fspInvokeDirectiveInvocation != null) {
codeLength += fspInvokeDirectiveInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 14
reverseOS.write(0xAE);
codeLength += 1;
return codeLength;
}
if (fspThrowEventInvocation != null) {
codeLength += fspThrowEventInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 8
reverseOS.write(0xA8);
codeLength += 1;
return codeLength;
}
if (fspGetParameterInvocation != null) {
codeLength += fspGetParameterInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 6
reverseOS.write(0xA6);
codeLength += 1;
return codeLength;
}
if (fspScheduleStatusReportInvocation != null) {
codeLength += fspScheduleStatusReportInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 4
reverseOS.write(0xA4);
codeLength += 1;
return codeLength;
}
if (fspTransferDataInvocation != null) {
codeLength += fspTransferDataInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 10
reverseOS.write(0xAA);
codeLength += 1;
return codeLength;
}
if (fspStopInvocation != null) {
codeLength += fspStopInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 2
reverseOS.write(0xA2);
codeLength += 1;
return codeLength;
}
if (fspStartInvocation != null) {
codeLength += fspStartInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 0
reverseOS.write(0xA0);
codeLength += 1;
return codeLength;
}
if (fspUnbindInvocation != null) {
codeLength += fspUnbindInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 102
reverseOS.write(0x66);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
if (fspBindInvocation != null) {
codeLength += fspBindInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 100
reverseOS.write(0x64);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
throw new IOException("Error encoding CHOICE: No element of CHOICE was selected.");
}
public int decode(InputStream is) throws IOException {
return decode(is, null);
}
public int decode(InputStream is, BerTag berTag) throws IOException {
int codeLength = 0;
BerTag passedTag = berTag;
if (berTag == null) {
berTag = new BerTag();
codeLength += berTag.decode(is);
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 100)) {
fspBindInvocation = new SleBindInvocation();
codeLength += fspBindInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 102)) {
fspUnbindInvocation = new SleUnbindInvocation();
codeLength += fspUnbindInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 0)) {
fspStartInvocation = new FspStartInvocation();
codeLength += fspStartInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 2)) {
fspStopInvocation = new SleStopInvocation();
codeLength += fspStopInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 10)) {
fspTransferDataInvocation = new FspTransferDataInvocation();
codeLength += fspTransferDataInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 4)) {
fspScheduleStatusReportInvocation = new SleScheduleStatusReportInvocation();
codeLength += fspScheduleStatusReportInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 6)) {
fspGetParameterInvocation = new FspGetParameterInvocation();
codeLength += fspGetParameterInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 8)) {
fspThrowEventInvocation = new FspThrowEventInvocation();
codeLength += fspThrowEventInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 14)) {
fspInvokeDirectiveInvocation = new FspInvokeDirectiveInvocation();
codeLength += fspInvokeDirectiveInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 104)) {
fspPeerAbortInvocation = new SlePeerAbort();
codeLength += fspPeerAbortInvocation.decode(is, false);
return codeLength;
}
if (passedTag != null) {
return 0;
}
throw new IOException("Error decoding CHOICE: Tag " + berTag + " matched to no item.");
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
if (fspBindInvocation != null) {
sb.append("fspBindInvocation: ");
fspBindInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (fspUnbindInvocation != null) {
sb.append("fspUnbindInvocation: ");
fspUnbindInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (fspStartInvocation != null) {
sb.append("fspStartInvocation: ");
fspStartInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (fspStopInvocation != null) {
sb.append("fspStopInvocation: ");
fspStopInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (fspTransferDataInvocation != null) {
sb.append("fspTransferDataInvocation: ");
fspTransferDataInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (fspScheduleStatusReportInvocation != null) {
sb.append("fspScheduleStatusReportInvocation: ");
fspScheduleStatusReportInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (fspGetParameterInvocation != null) {
sb.append("fspGetParameterInvocation: ");
fspGetParameterInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (fspThrowEventInvocation != null) {
sb.append("fspThrowEventInvocation: ");
fspThrowEventInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (fspInvokeDirectiveInvocation != null) {
sb.append("fspInvokeDirectiveInvocation: ");
fspInvokeDirectiveInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (fspPeerAbortInvocation != null) {
sb.append("fspPeerAbortInvocation: ").append(fspPeerAbortInvocation);
return;
}
sb.append("");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy