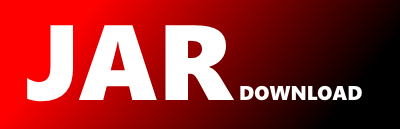
ccsds.sle.transfer.service.fsp.structures.FspGetParameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsle Show documentation
Show all versions of jsle Show documentation
Java implementation for the SLE (Space Link Extension) protocol.
/**
* This class file was automatically generated by jASN1 v1.11.2 (http://www.beanit.com)
*/
package ccsds.sle.transfer.service.fsp.structures;
import java.io.IOException;
import java.io.EOFException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.ArrayList;
import java.util.Iterator;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.io.Serializable;
import com.beanit.jasn1.ber.*;
import com.beanit.jasn1.ber.types.*;
import com.beanit.jasn1.ber.types.string.*;
import ccsds.sle.transfer.service.common.pdus.ReportingCycle;
import ccsds.sle.transfer.service.common.types.DeliveryMode;
import ccsds.sle.transfer.service.common.types.Diagnostics;
import ccsds.sle.transfer.service.common.types.ForwardDuStatus;
import ccsds.sle.transfer.service.common.types.IntPosLong;
import ccsds.sle.transfer.service.common.types.IntPosShort;
import ccsds.sle.transfer.service.common.types.IntUnsignedLong;
import ccsds.sle.transfer.service.common.types.ParameterName;
import ccsds.sle.transfer.service.common.types.SpaceLinkDataUnit;
import ccsds.sle.transfer.service.common.types.Time;
public class FspGetParameter implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public byte[] code = null;
public static class ParApidList implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private ApidList parameterValue = null;
public ParApidList() {
}
public ParApidList(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(ApidList parameterValue) {
this.parameterValue = parameterValue;
}
public ApidList getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new ApidList();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParBitLockRequired implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParBitLockRequired() {
}
public ParBitLockRequired(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParBlockingTimeout implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static class ParameterValue implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public byte[] code = null;
private BerNull blockingOff = null;
private BlockingTimeoutPeriod blockingOn = null;
public ParameterValue() {
}
public ParameterValue(byte[] code) {
this.code = code;
}
public void setBlockingOff(BerNull blockingOff) {
this.blockingOff = blockingOff;
}
public BerNull getBlockingOff() {
return blockingOff;
}
public void setBlockingOn(BlockingTimeoutPeriod blockingOn) {
this.blockingOn = blockingOn;
}
public BlockingTimeoutPeriod getBlockingOn() {
return blockingOn;
}
public int encode(OutputStream reverseOS) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
return code.length;
}
int codeLength = 0;
if (blockingOn != null) {
codeLength += blockingOn.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 1
reverseOS.write(0x81);
codeLength += 1;
return codeLength;
}
if (blockingOff != null) {
codeLength += blockingOff.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 0
reverseOS.write(0x80);
codeLength += 1;
return codeLength;
}
throw new IOException("Error encoding CHOICE: No element of CHOICE was selected.");
}
public int decode(InputStream is) throws IOException {
return decode(is, null);
}
public int decode(InputStream is, BerTag berTag) throws IOException {
int codeLength = 0;
BerTag passedTag = berTag;
if (berTag == null) {
berTag = new BerTag();
codeLength += berTag.decode(is);
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 0)) {
blockingOff = new BerNull();
codeLength += blockingOff.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 1)) {
blockingOn = new BlockingTimeoutPeriod();
codeLength += blockingOn.decode(is, false);
return codeLength;
}
if (passedTag != null) {
return 0;
}
throw new IOException("Error decoding CHOICE: Tag " + berTag + " matched to no item.");
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
if (blockingOff != null) {
sb.append("blockingOff: ").append(blockingOff);
return;
}
if (blockingOn != null) {
sb.append("blockingOn: ").append(blockingOn);
return;
}
sb.append("");
}
}
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private ParameterValue parameterValue = null;
public ParBlockingTimeout() {
}
public ParBlockingTimeout(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(ParameterValue parameterValue) {
this.parameterValue = parameterValue;
}
public ParameterValue getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new ParameterValue();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParBlockingUsage implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BlockingUsage parameterValue = null;
public ParBlockingUsage() {
}
public ParBlockingUsage(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BlockingUsage parameterValue) {
this.parameterValue = parameterValue;
}
public BlockingUsage getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BlockingUsage.tag)) {
parameterValue = new BlockingUsage();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParClcwGlobalVcId implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private ClcwGvcId parameterValue = null;
public ParClcwGlobalVcId() {
}
public ParClcwGlobalVcId(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(ClcwGvcId parameterValue) {
this.parameterValue = parameterValue;
}
public ClcwGvcId getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new ClcwGvcId();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParClcwPhysicalChannel implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private ClcwPhysicalChannel parameterValue = null;
public ParClcwPhysicalChannel() {
}
public ParClcwPhysicalChannel(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(ClcwPhysicalChannel parameterValue) {
this.parameterValue = parameterValue;
}
public ClcwPhysicalChannel getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new ClcwPhysicalChannel();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParCopCntrFramesRepetition implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private IntPosShort parameterValue = null;
public ParCopCntrFramesRepetition() {
}
public ParCopCntrFramesRepetition(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(IntPosShort parameterValue) {
this.parameterValue = parameterValue;
}
public IntPosShort getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(IntPosShort.tag)) {
parameterValue = new IntPosShort();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParDeliveryMode implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private FspDeliveryMode parameterValue = null;
public ParDeliveryMode() {
}
public ParDeliveryMode(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(FspDeliveryMode parameterValue) {
this.parameterValue = parameterValue;
}
public FspDeliveryMode getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(FspDeliveryMode.tag)) {
parameterValue = new FspDeliveryMode();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParDirectiveInvoc implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParDirectiveInvoc() {
}
public ParDirectiveInvoc(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParDirInvocOnl implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParDirInvocOnl() {
}
public ParDirInvocOnl(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParExpectDirectiveId implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private IntUnsignedLong parameterValue = null;
public ParExpectDirectiveId() {
}
public ParExpectDirectiveId(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(IntUnsignedLong parameterValue) {
this.parameterValue = parameterValue;
}
public IntUnsignedLong getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(IntUnsignedLong.tag)) {
parameterValue = new IntUnsignedLong();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParExpectEventInvId implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private IntUnsignedLong parameterValue = null;
public ParExpectEventInvId() {
}
public ParExpectEventInvId(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(IntUnsignedLong parameterValue) {
this.parameterValue = parameterValue;
}
public IntUnsignedLong getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(IntUnsignedLong.tag)) {
parameterValue = new IntUnsignedLong();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParExpectSlduId implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private PacketIdentification parameterValue = null;
public ParExpectSlduId() {
}
public ParExpectSlduId(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(PacketIdentification parameterValue) {
this.parameterValue = parameterValue;
}
public PacketIdentification getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(PacketIdentification.tag)) {
parameterValue = new PacketIdentification();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParFopSlidWindow implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParFopSlidWindow() {
}
public ParFopSlidWindow(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParFopState implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger fopState = null;
public ParFopState() {
}
public ParFopState(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setFopState(BerInteger fopState) {
this.fopState = fopState;
}
public BerInteger getFopState() {
return fopState;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += fopState.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
fopState = new BerInteger();
subCodeLength += fopState.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (fopState != null) {
sb.append("fopState: ").append(fopState);
}
else {
sb.append("fopState: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParMapList implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private MapList mapList = null;
public ParMapList() {
}
public ParMapList(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setMapList(MapList mapList) {
this.mapList = mapList;
}
public MapList getMapList() {
return mapList;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += mapList.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
mapList = new MapList();
subCodeLength += mapList.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (mapList != null) {
sb.append("mapList: ");
mapList.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("mapList: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParMapMuxControl implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private MuxControl parameterValue = null;
public ParMapMuxControl() {
}
public ParMapMuxControl(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(MuxControl parameterValue) {
this.parameterValue = parameterValue;
}
public MuxControl getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new MuxControl();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParMapMuxScheme implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private MuxScheme parameterValue = null;
public ParMapMuxScheme() {
}
public ParMapMuxScheme(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(MuxScheme parameterValue) {
this.parameterValue = parameterValue;
}
public MuxScheme getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(MuxScheme.tag)) {
parameterValue = new MuxScheme();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParMaxFrameLength implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParMaxFrameLength() {
}
public ParMaxFrameLength(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParMaxPacketLength implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParMaxPacketLength() {
}
public ParMaxPacketLength(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParMinReportingCycle implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private IntPosShort parameterValue = null;
public ParMinReportingCycle() {
}
public ParMinReportingCycle(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(IntPosShort parameterValue) {
this.parameterValue = parameterValue;
}
public IntPosShort getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(IntPosShort.tag)) {
parameterValue = new IntPosShort();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParPermTransMode implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private PermittedTransmissionMode parameterValue = null;
public ParPermTransMode() {
}
public ParPermTransMode(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(PermittedTransmissionMode parameterValue) {
this.parameterValue = parameterValue;
}
public PermittedTransmissionMode getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(PermittedTransmissionMode.tag)) {
parameterValue = new PermittedTransmissionMode();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParReportingCycle implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private CurrentReportingCycle parameterValue = null;
public ParReportingCycle() {
}
public ParReportingCycle(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(CurrentReportingCycle parameterValue) {
this.parameterValue = parameterValue;
}
public CurrentReportingCycle getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new CurrentReportingCycle();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParReturnTimeout implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private TimeoutPeriod parameterValue = null;
public ParReturnTimeout() {
}
public ParReturnTimeout(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(TimeoutPeriod parameterValue) {
this.parameterValue = parameterValue;
}
public TimeoutPeriod getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(TimeoutPeriod.tag)) {
parameterValue = new TimeoutPeriod();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParRfAvailableRequired implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParRfAvailableRequired() {
}
public ParRfAvailableRequired(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParSegmHeader implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParSegmHeader() {
}
public ParSegmHeader(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParSequCntrFramesRepetition implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private IntPosShort parameterValue = null;
public ParSequCntrFramesRepetition() {
}
public ParSequCntrFramesRepetition(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(IntPosShort parameterValue) {
this.parameterValue = parameterValue;
}
public IntPosShort getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(IntPosShort.tag)) {
parameterValue = new IntPosShort();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParThrowEventOperation implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParThrowEventOperation() {
}
public ParThrowEventOperation(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParTimeoutType implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParTimeoutType() {
}
public ParTimeoutType(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParTimerInitial implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private IntPosLong parameterValue = null;
public ParTimerInitial() {
}
public ParTimerInitial(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(IntPosLong parameterValue) {
this.parameterValue = parameterValue;
}
public IntPosLong getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(IntPosLong.tag)) {
parameterValue = new IntPosLong();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParTransmissLimit implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParTransmissLimit() {
}
public ParTransmissLimit(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParTrFrSeqNumber implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private BerInteger parameterValue = null;
public ParTrFrSeqNumber() {
}
public ParTrFrSeqNumber(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(BerInteger parameterValue) {
this.parameterValue = parameterValue;
}
public BerInteger getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(BerInteger.tag)) {
parameterValue = new BerInteger();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParVcMuxControl implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private MuxControl parameterValue = null;
public ParVcMuxControl() {
}
public ParVcMuxControl(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(MuxControl parameterValue) {
this.parameterValue = parameterValue;
}
public MuxControl getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new MuxControl();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParVcMuxScheme implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private MuxScheme parameterValue = null;
public ParVcMuxScheme() {
}
public ParVcMuxScheme(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(MuxScheme parameterValue) {
this.parameterValue = parameterValue;
}
public MuxScheme getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(MuxScheme.tag)) {
parameterValue = new MuxScheme();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParVirtualChannel implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private VcOrMapId parameterValue = null;
public ParVirtualChannel() {
}
public ParVirtualChannel(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(VcOrMapId parameterValue) {
this.parameterValue = parameterValue;
}
public VcOrMapId getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(VcOrMapId.tag)) {
parameterValue = new VcOrMapId();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
private ParApidList parApidList = null;
private ParBitLockRequired parBitLockRequired = null;
private ParBlockingTimeout parBlockingTimeout = null;
private ParBlockingUsage parBlockingUsage = null;
private ParClcwGlobalVcId parClcwGlobalVcId = null;
private ParClcwPhysicalChannel parClcwPhysicalChannel = null;
private ParCopCntrFramesRepetition parCopCntrFramesRepetition = null;
private ParDeliveryMode parDeliveryMode = null;
private ParDirectiveInvoc parDirectiveInvoc = null;
private ParDirInvocOnl parDirInvocOnl = null;
private ParExpectDirectiveId parExpectDirectiveId = null;
private ParExpectEventInvId parExpectEventInvId = null;
private ParExpectSlduId parExpectSlduId = null;
private ParFopSlidWindow parFopSlidWindow = null;
private ParFopState parFopState = null;
private ParMapList parMapList = null;
private ParMapMuxControl parMapMuxControl = null;
private ParMapMuxScheme parMapMuxScheme = null;
private ParMaxFrameLength parMaxFrameLength = null;
private ParMaxPacketLength parMaxPacketLength = null;
private ParMinReportingCycle parMinReportingCycle = null;
private ParPermTransMode parPermTransMode = null;
private ParReportingCycle parReportingCycle = null;
private ParReturnTimeout parReturnTimeout = null;
private ParRfAvailableRequired parRfAvailableRequired = null;
private ParSegmHeader parSegmHeader = null;
private ParSequCntrFramesRepetition parSequCntrFramesRepetition = null;
private ParThrowEventOperation parThrowEventOperation = null;
private ParTimeoutType parTimeoutType = null;
private ParTimerInitial parTimerInitial = null;
private ParTransmissLimit parTransmissLimit = null;
private ParTrFrSeqNumber parTrFrSeqNumber = null;
private ParVcMuxControl parVcMuxControl = null;
private ParVcMuxScheme parVcMuxScheme = null;
private ParVirtualChannel parVirtualChannel = null;
public FspGetParameter() {
}
public FspGetParameter(byte[] code) {
this.code = code;
}
public void setParApidList(ParApidList parApidList) {
this.parApidList = parApidList;
}
public ParApidList getParApidList() {
return parApidList;
}
public void setParBitLockRequired(ParBitLockRequired parBitLockRequired) {
this.parBitLockRequired = parBitLockRequired;
}
public ParBitLockRequired getParBitLockRequired() {
return parBitLockRequired;
}
public void setParBlockingTimeout(ParBlockingTimeout parBlockingTimeout) {
this.parBlockingTimeout = parBlockingTimeout;
}
public ParBlockingTimeout getParBlockingTimeout() {
return parBlockingTimeout;
}
public void setParBlockingUsage(ParBlockingUsage parBlockingUsage) {
this.parBlockingUsage = parBlockingUsage;
}
public ParBlockingUsage getParBlockingUsage() {
return parBlockingUsage;
}
public void setParClcwGlobalVcId(ParClcwGlobalVcId parClcwGlobalVcId) {
this.parClcwGlobalVcId = parClcwGlobalVcId;
}
public ParClcwGlobalVcId getParClcwGlobalVcId() {
return parClcwGlobalVcId;
}
public void setParClcwPhysicalChannel(ParClcwPhysicalChannel parClcwPhysicalChannel) {
this.parClcwPhysicalChannel = parClcwPhysicalChannel;
}
public ParClcwPhysicalChannel getParClcwPhysicalChannel() {
return parClcwPhysicalChannel;
}
public void setParCopCntrFramesRepetition(ParCopCntrFramesRepetition parCopCntrFramesRepetition) {
this.parCopCntrFramesRepetition = parCopCntrFramesRepetition;
}
public ParCopCntrFramesRepetition getParCopCntrFramesRepetition() {
return parCopCntrFramesRepetition;
}
public void setParDeliveryMode(ParDeliveryMode parDeliveryMode) {
this.parDeliveryMode = parDeliveryMode;
}
public ParDeliveryMode getParDeliveryMode() {
return parDeliveryMode;
}
public void setParDirectiveInvoc(ParDirectiveInvoc parDirectiveInvoc) {
this.parDirectiveInvoc = parDirectiveInvoc;
}
public ParDirectiveInvoc getParDirectiveInvoc() {
return parDirectiveInvoc;
}
public void setParDirInvocOnl(ParDirInvocOnl parDirInvocOnl) {
this.parDirInvocOnl = parDirInvocOnl;
}
public ParDirInvocOnl getParDirInvocOnl() {
return parDirInvocOnl;
}
public void setParExpectDirectiveId(ParExpectDirectiveId parExpectDirectiveId) {
this.parExpectDirectiveId = parExpectDirectiveId;
}
public ParExpectDirectiveId getParExpectDirectiveId() {
return parExpectDirectiveId;
}
public void setParExpectEventInvId(ParExpectEventInvId parExpectEventInvId) {
this.parExpectEventInvId = parExpectEventInvId;
}
public ParExpectEventInvId getParExpectEventInvId() {
return parExpectEventInvId;
}
public void setParExpectSlduId(ParExpectSlduId parExpectSlduId) {
this.parExpectSlduId = parExpectSlduId;
}
public ParExpectSlduId getParExpectSlduId() {
return parExpectSlduId;
}
public void setParFopSlidWindow(ParFopSlidWindow parFopSlidWindow) {
this.parFopSlidWindow = parFopSlidWindow;
}
public ParFopSlidWindow getParFopSlidWindow() {
return parFopSlidWindow;
}
public void setParFopState(ParFopState parFopState) {
this.parFopState = parFopState;
}
public ParFopState getParFopState() {
return parFopState;
}
public void setParMapList(ParMapList parMapList) {
this.parMapList = parMapList;
}
public ParMapList getParMapList() {
return parMapList;
}
public void setParMapMuxControl(ParMapMuxControl parMapMuxControl) {
this.parMapMuxControl = parMapMuxControl;
}
public ParMapMuxControl getParMapMuxControl() {
return parMapMuxControl;
}
public void setParMapMuxScheme(ParMapMuxScheme parMapMuxScheme) {
this.parMapMuxScheme = parMapMuxScheme;
}
public ParMapMuxScheme getParMapMuxScheme() {
return parMapMuxScheme;
}
public void setParMaxFrameLength(ParMaxFrameLength parMaxFrameLength) {
this.parMaxFrameLength = parMaxFrameLength;
}
public ParMaxFrameLength getParMaxFrameLength() {
return parMaxFrameLength;
}
public void setParMaxPacketLength(ParMaxPacketLength parMaxPacketLength) {
this.parMaxPacketLength = parMaxPacketLength;
}
public ParMaxPacketLength getParMaxPacketLength() {
return parMaxPacketLength;
}
public void setParMinReportingCycle(ParMinReportingCycle parMinReportingCycle) {
this.parMinReportingCycle = parMinReportingCycle;
}
public ParMinReportingCycle getParMinReportingCycle() {
return parMinReportingCycle;
}
public void setParPermTransMode(ParPermTransMode parPermTransMode) {
this.parPermTransMode = parPermTransMode;
}
public ParPermTransMode getParPermTransMode() {
return parPermTransMode;
}
public void setParReportingCycle(ParReportingCycle parReportingCycle) {
this.parReportingCycle = parReportingCycle;
}
public ParReportingCycle getParReportingCycle() {
return parReportingCycle;
}
public void setParReturnTimeout(ParReturnTimeout parReturnTimeout) {
this.parReturnTimeout = parReturnTimeout;
}
public ParReturnTimeout getParReturnTimeout() {
return parReturnTimeout;
}
public void setParRfAvailableRequired(ParRfAvailableRequired parRfAvailableRequired) {
this.parRfAvailableRequired = parRfAvailableRequired;
}
public ParRfAvailableRequired getParRfAvailableRequired() {
return parRfAvailableRequired;
}
public void setParSegmHeader(ParSegmHeader parSegmHeader) {
this.parSegmHeader = parSegmHeader;
}
public ParSegmHeader getParSegmHeader() {
return parSegmHeader;
}
public void setParSequCntrFramesRepetition(ParSequCntrFramesRepetition parSequCntrFramesRepetition) {
this.parSequCntrFramesRepetition = parSequCntrFramesRepetition;
}
public ParSequCntrFramesRepetition getParSequCntrFramesRepetition() {
return parSequCntrFramesRepetition;
}
public void setParThrowEventOperation(ParThrowEventOperation parThrowEventOperation) {
this.parThrowEventOperation = parThrowEventOperation;
}
public ParThrowEventOperation getParThrowEventOperation() {
return parThrowEventOperation;
}
public void setParTimeoutType(ParTimeoutType parTimeoutType) {
this.parTimeoutType = parTimeoutType;
}
public ParTimeoutType getParTimeoutType() {
return parTimeoutType;
}
public void setParTimerInitial(ParTimerInitial parTimerInitial) {
this.parTimerInitial = parTimerInitial;
}
public ParTimerInitial getParTimerInitial() {
return parTimerInitial;
}
public void setParTransmissLimit(ParTransmissLimit parTransmissLimit) {
this.parTransmissLimit = parTransmissLimit;
}
public ParTransmissLimit getParTransmissLimit() {
return parTransmissLimit;
}
public void setParTrFrSeqNumber(ParTrFrSeqNumber parTrFrSeqNumber) {
this.parTrFrSeqNumber = parTrFrSeqNumber;
}
public ParTrFrSeqNumber getParTrFrSeqNumber() {
return parTrFrSeqNumber;
}
public void setParVcMuxControl(ParVcMuxControl parVcMuxControl) {
this.parVcMuxControl = parVcMuxControl;
}
public ParVcMuxControl getParVcMuxControl() {
return parVcMuxControl;
}
public void setParVcMuxScheme(ParVcMuxScheme parVcMuxScheme) {
this.parVcMuxScheme = parVcMuxScheme;
}
public ParVcMuxScheme getParVcMuxScheme() {
return parVcMuxScheme;
}
public void setParVirtualChannel(ParVirtualChannel parVirtualChannel) {
this.parVirtualChannel = parVirtualChannel;
}
public ParVirtualChannel getParVirtualChannel() {
return parVirtualChannel;
}
public int encode(OutputStream reverseOS) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
return code.length;
}
int codeLength = 0;
if (parVirtualChannel != null) {
codeLength += parVirtualChannel.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 26
reverseOS.write(0xBA);
codeLength += 1;
return codeLength;
}
if (parVcMuxScheme != null) {
codeLength += parVcMuxScheme.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 25
reverseOS.write(0xB9);
codeLength += 1;
return codeLength;
}
if (parVcMuxControl != null) {
codeLength += parVcMuxControl.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 24
reverseOS.write(0xB8);
codeLength += 1;
return codeLength;
}
if (parTrFrSeqNumber != null) {
codeLength += parTrFrSeqNumber.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 23
reverseOS.write(0xB7);
codeLength += 1;
return codeLength;
}
if (parTransmissLimit != null) {
codeLength += parTransmissLimit.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 22
reverseOS.write(0xB6);
codeLength += 1;
return codeLength;
}
if (parTimerInitial != null) {
codeLength += parTimerInitial.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 21
reverseOS.write(0xB5);
codeLength += 1;
return codeLength;
}
if (parTimeoutType != null) {
codeLength += parTimeoutType.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 20
reverseOS.write(0xB4);
codeLength += 1;
return codeLength;
}
if (parThrowEventOperation != null) {
codeLength += parThrowEventOperation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 32
reverseOS.write(0x20);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
if (parSequCntrFramesRepetition != null) {
codeLength += parSequCntrFramesRepetition.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 31
reverseOS.write(0x1F);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
if (parSegmHeader != null) {
codeLength += parSegmHeader.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 19
reverseOS.write(0xB3);
codeLength += 1;
return codeLength;
}
if (parRfAvailableRequired != null) {
codeLength += parRfAvailableRequired.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 28
reverseOS.write(0xBC);
codeLength += 1;
return codeLength;
}
if (parReturnTimeout != null) {
codeLength += parReturnTimeout.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 18
reverseOS.write(0xB2);
codeLength += 1;
return codeLength;
}
if (parReportingCycle != null) {
codeLength += parReportingCycle.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 17
reverseOS.write(0xB1);
codeLength += 1;
return codeLength;
}
if (parPermTransMode != null) {
codeLength += parPermTransMode.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 16
reverseOS.write(0xB0);
codeLength += 1;
return codeLength;
}
if (parMinReportingCycle != null) {
codeLength += parMinReportingCycle.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 30
reverseOS.write(0xBE);
codeLength += 1;
return codeLength;
}
if (parMaxPacketLength != null) {
codeLength += parMaxPacketLength.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 15
reverseOS.write(0xAF);
codeLength += 1;
return codeLength;
}
if (parMaxFrameLength != null) {
codeLength += parMaxFrameLength.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 14
reverseOS.write(0xAE);
codeLength += 1;
return codeLength;
}
if (parMapMuxScheme != null) {
codeLength += parMapMuxScheme.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 13
reverseOS.write(0xAD);
codeLength += 1;
return codeLength;
}
if (parMapMuxControl != null) {
codeLength += parMapMuxControl.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 12
reverseOS.write(0xAC);
codeLength += 1;
return codeLength;
}
if (parMapList != null) {
codeLength += parMapList.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 11
reverseOS.write(0xAB);
codeLength += 1;
return codeLength;
}
if (parFopState != null) {
codeLength += parFopState.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 10
reverseOS.write(0xAA);
codeLength += 1;
return codeLength;
}
if (parFopSlidWindow != null) {
codeLength += parFopSlidWindow.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 9
reverseOS.write(0xA9);
codeLength += 1;
return codeLength;
}
if (parExpectSlduId != null) {
codeLength += parExpectSlduId.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 8
reverseOS.write(0xA8);
codeLength += 1;
return codeLength;
}
if (parExpectEventInvId != null) {
codeLength += parExpectEventInvId.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 7
reverseOS.write(0xA7);
codeLength += 1;
return codeLength;
}
if (parExpectDirectiveId != null) {
codeLength += parExpectDirectiveId.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 6
reverseOS.write(0xA6);
codeLength += 1;
return codeLength;
}
if (parDirInvocOnl != null) {
codeLength += parDirInvocOnl.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 5
reverseOS.write(0xA5);
codeLength += 1;
return codeLength;
}
if (parDirectiveInvoc != null) {
codeLength += parDirectiveInvoc.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 4
reverseOS.write(0xA4);
codeLength += 1;
return codeLength;
}
if (parDeliveryMode != null) {
codeLength += parDeliveryMode.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 3
reverseOS.write(0xA3);
codeLength += 1;
return codeLength;
}
if (parCopCntrFramesRepetition != null) {
codeLength += parCopCntrFramesRepetition.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 29
reverseOS.write(0xBD);
codeLength += 1;
return codeLength;
}
if (parClcwPhysicalChannel != null) {
codeLength += parClcwPhysicalChannel.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 28
reverseOS.write(0xBC);
codeLength += 1;
return codeLength;
}
if (parClcwGlobalVcId != null) {
codeLength += parClcwGlobalVcId.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 27
reverseOS.write(0xBB);
codeLength += 1;
return codeLength;
}
if (parBlockingUsage != null) {
codeLength += parBlockingUsage.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 2
reverseOS.write(0xA2);
codeLength += 1;
return codeLength;
}
if (parBlockingTimeout != null) {
codeLength += parBlockingTimeout.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 1
reverseOS.write(0xA1);
codeLength += 1;
return codeLength;
}
if (parBitLockRequired != null) {
codeLength += parBitLockRequired.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 27
reverseOS.write(0xBB);
codeLength += 1;
return codeLength;
}
if (parApidList != null) {
codeLength += parApidList.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 0
reverseOS.write(0xA0);
codeLength += 1;
return codeLength;
}
throw new IOException("Error encoding CHOICE: No element of CHOICE was selected.");
}
public int decode(InputStream is) throws IOException {
return decode(is, null);
}
public int decode(InputStream is, BerTag berTag) throws IOException {
int codeLength = 0;
BerTag passedTag = berTag;
if (berTag == null) {
berTag = new BerTag();
codeLength += berTag.decode(is);
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 0)) {
parApidList = new ParApidList();
codeLength += parApidList.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 27)) {
parBitLockRequired = new ParBitLockRequired();
codeLength += parBitLockRequired.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 1)) {
parBlockingTimeout = new ParBlockingTimeout();
codeLength += parBlockingTimeout.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 2)) {
parBlockingUsage = new ParBlockingUsage();
codeLength += parBlockingUsage.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 27)) {
parClcwGlobalVcId = new ParClcwGlobalVcId();
codeLength += parClcwGlobalVcId.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 28)) {
parClcwPhysicalChannel = new ParClcwPhysicalChannel();
codeLength += parClcwPhysicalChannel.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 29)) {
parCopCntrFramesRepetition = new ParCopCntrFramesRepetition();
codeLength += parCopCntrFramesRepetition.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 3)) {
parDeliveryMode = new ParDeliveryMode();
codeLength += parDeliveryMode.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 4)) {
parDirectiveInvoc = new ParDirectiveInvoc();
codeLength += parDirectiveInvoc.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 5)) {
parDirInvocOnl = new ParDirInvocOnl();
codeLength += parDirInvocOnl.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 6)) {
parExpectDirectiveId = new ParExpectDirectiveId();
codeLength += parExpectDirectiveId.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 7)) {
parExpectEventInvId = new ParExpectEventInvId();
codeLength += parExpectEventInvId.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 8)) {
parExpectSlduId = new ParExpectSlduId();
codeLength += parExpectSlduId.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 9)) {
parFopSlidWindow = new ParFopSlidWindow();
codeLength += parFopSlidWindow.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 10)) {
parFopState = new ParFopState();
codeLength += parFopState.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 11)) {
parMapList = new ParMapList();
codeLength += parMapList.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 12)) {
parMapMuxControl = new ParMapMuxControl();
codeLength += parMapMuxControl.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 13)) {
parMapMuxScheme = new ParMapMuxScheme();
codeLength += parMapMuxScheme.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 14)) {
parMaxFrameLength = new ParMaxFrameLength();
codeLength += parMaxFrameLength.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 15)) {
parMaxPacketLength = new ParMaxPacketLength();
codeLength += parMaxPacketLength.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 30)) {
parMinReportingCycle = new ParMinReportingCycle();
codeLength += parMinReportingCycle.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 16)) {
parPermTransMode = new ParPermTransMode();
codeLength += parPermTransMode.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 17)) {
parReportingCycle = new ParReportingCycle();
codeLength += parReportingCycle.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 18)) {
parReturnTimeout = new ParReturnTimeout();
codeLength += parReturnTimeout.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 28)) {
parRfAvailableRequired = new ParRfAvailableRequired();
codeLength += parRfAvailableRequired.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 19)) {
parSegmHeader = new ParSegmHeader();
codeLength += parSegmHeader.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 31)) {
parSequCntrFramesRepetition = new ParSequCntrFramesRepetition();
codeLength += parSequCntrFramesRepetition.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 32)) {
parThrowEventOperation = new ParThrowEventOperation();
codeLength += parThrowEventOperation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 20)) {
parTimeoutType = new ParTimeoutType();
codeLength += parTimeoutType.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 21)) {
parTimerInitial = new ParTimerInitial();
codeLength += parTimerInitial.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 22)) {
parTransmissLimit = new ParTransmissLimit();
codeLength += parTransmissLimit.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 23)) {
parTrFrSeqNumber = new ParTrFrSeqNumber();
codeLength += parTrFrSeqNumber.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 24)) {
parVcMuxControl = new ParVcMuxControl();
codeLength += parVcMuxControl.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 25)) {
parVcMuxScheme = new ParVcMuxScheme();
codeLength += parVcMuxScheme.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 26)) {
parVirtualChannel = new ParVirtualChannel();
codeLength += parVirtualChannel.decode(is, false);
return codeLength;
}
if (passedTag != null) {
return 0;
}
throw new IOException("Error decoding CHOICE: Tag " + berTag + " matched to no item.");
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
if (parApidList != null) {
sb.append("parApidList: ");
parApidList.appendAsString(sb, indentLevel + 1);
return;
}
if (parBitLockRequired != null) {
sb.append("parBitLockRequired: ");
parBitLockRequired.appendAsString(sb, indentLevel + 1);
return;
}
if (parBlockingTimeout != null) {
sb.append("parBlockingTimeout: ");
parBlockingTimeout.appendAsString(sb, indentLevel + 1);
return;
}
if (parBlockingUsage != null) {
sb.append("parBlockingUsage: ");
parBlockingUsage.appendAsString(sb, indentLevel + 1);
return;
}
if (parClcwGlobalVcId != null) {
sb.append("parClcwGlobalVcId: ");
parClcwGlobalVcId.appendAsString(sb, indentLevel + 1);
return;
}
if (parClcwPhysicalChannel != null) {
sb.append("parClcwPhysicalChannel: ");
parClcwPhysicalChannel.appendAsString(sb, indentLevel + 1);
return;
}
if (parCopCntrFramesRepetition != null) {
sb.append("parCopCntrFramesRepetition: ");
parCopCntrFramesRepetition.appendAsString(sb, indentLevel + 1);
return;
}
if (parDeliveryMode != null) {
sb.append("parDeliveryMode: ");
parDeliveryMode.appendAsString(sb, indentLevel + 1);
return;
}
if (parDirectiveInvoc != null) {
sb.append("parDirectiveInvoc: ");
parDirectiveInvoc.appendAsString(sb, indentLevel + 1);
return;
}
if (parDirInvocOnl != null) {
sb.append("parDirInvocOnl: ");
parDirInvocOnl.appendAsString(sb, indentLevel + 1);
return;
}
if (parExpectDirectiveId != null) {
sb.append("parExpectDirectiveId: ");
parExpectDirectiveId.appendAsString(sb, indentLevel + 1);
return;
}
if (parExpectEventInvId != null) {
sb.append("parExpectEventInvId: ");
parExpectEventInvId.appendAsString(sb, indentLevel + 1);
return;
}
if (parExpectSlduId != null) {
sb.append("parExpectSlduId: ");
parExpectSlduId.appendAsString(sb, indentLevel + 1);
return;
}
if (parFopSlidWindow != null) {
sb.append("parFopSlidWindow: ");
parFopSlidWindow.appendAsString(sb, indentLevel + 1);
return;
}
if (parFopState != null) {
sb.append("parFopState: ");
parFopState.appendAsString(sb, indentLevel + 1);
return;
}
if (parMapList != null) {
sb.append("parMapList: ");
parMapList.appendAsString(sb, indentLevel + 1);
return;
}
if (parMapMuxControl != null) {
sb.append("parMapMuxControl: ");
parMapMuxControl.appendAsString(sb, indentLevel + 1);
return;
}
if (parMapMuxScheme != null) {
sb.append("parMapMuxScheme: ");
parMapMuxScheme.appendAsString(sb, indentLevel + 1);
return;
}
if (parMaxFrameLength != null) {
sb.append("parMaxFrameLength: ");
parMaxFrameLength.appendAsString(sb, indentLevel + 1);
return;
}
if (parMaxPacketLength != null) {
sb.append("parMaxPacketLength: ");
parMaxPacketLength.appendAsString(sb, indentLevel + 1);
return;
}
if (parMinReportingCycle != null) {
sb.append("parMinReportingCycle: ");
parMinReportingCycle.appendAsString(sb, indentLevel + 1);
return;
}
if (parPermTransMode != null) {
sb.append("parPermTransMode: ");
parPermTransMode.appendAsString(sb, indentLevel + 1);
return;
}
if (parReportingCycle != null) {
sb.append("parReportingCycle: ");
parReportingCycle.appendAsString(sb, indentLevel + 1);
return;
}
if (parReturnTimeout != null) {
sb.append("parReturnTimeout: ");
parReturnTimeout.appendAsString(sb, indentLevel + 1);
return;
}
if (parRfAvailableRequired != null) {
sb.append("parRfAvailableRequired: ");
parRfAvailableRequired.appendAsString(sb, indentLevel + 1);
return;
}
if (parSegmHeader != null) {
sb.append("parSegmHeader: ");
parSegmHeader.appendAsString(sb, indentLevel + 1);
return;
}
if (parSequCntrFramesRepetition != null) {
sb.append("parSequCntrFramesRepetition: ");
parSequCntrFramesRepetition.appendAsString(sb, indentLevel + 1);
return;
}
if (parThrowEventOperation != null) {
sb.append("parThrowEventOperation: ");
parThrowEventOperation.appendAsString(sb, indentLevel + 1);
return;
}
if (parTimeoutType != null) {
sb.append("parTimeoutType: ");
parTimeoutType.appendAsString(sb, indentLevel + 1);
return;
}
if (parTimerInitial != null) {
sb.append("parTimerInitial: ");
parTimerInitial.appendAsString(sb, indentLevel + 1);
return;
}
if (parTransmissLimit != null) {
sb.append("parTransmissLimit: ");
parTransmissLimit.appendAsString(sb, indentLevel + 1);
return;
}
if (parTrFrSeqNumber != null) {
sb.append("parTrFrSeqNumber: ");
parTrFrSeqNumber.appendAsString(sb, indentLevel + 1);
return;
}
if (parVcMuxControl != null) {
sb.append("parVcMuxControl: ");
parVcMuxControl.appendAsString(sb, indentLevel + 1);
return;
}
if (parVcMuxScheme != null) {
sb.append("parVcMuxScheme: ");
parVcMuxScheme.appendAsString(sb, indentLevel + 1);
return;
}
if (parVirtualChannel != null) {
sb.append("parVirtualChannel: ");
parVirtualChannel.appendAsString(sb, indentLevel + 1);
return;
}
sb.append("");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy