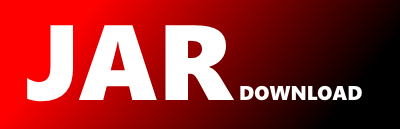
ccsds.sle.transfer.service.fsp.structures.FspNotification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsle Show documentation
Show all versions of jsle Show documentation
Java implementation for the SLE (Space Link Extension) protocol.
/**
* This class file was automatically generated by jASN1 v1.11.2 (http://www.beanit.com)
*/
package ccsds.sle.transfer.service.fsp.structures;
import java.io.IOException;
import java.io.EOFException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.ArrayList;
import java.util.Iterator;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.io.Serializable;
import com.beanit.jasn1.ber.*;
import com.beanit.jasn1.ber.types.*;
import com.beanit.jasn1.ber.types.string.*;
import ccsds.sle.transfer.service.common.pdus.ReportingCycle;
import ccsds.sle.transfer.service.common.types.DeliveryMode;
import ccsds.sle.transfer.service.common.types.Diagnostics;
import ccsds.sle.transfer.service.common.types.ForwardDuStatus;
import ccsds.sle.transfer.service.common.types.IntPosLong;
import ccsds.sle.transfer.service.common.types.IntPosShort;
import ccsds.sle.transfer.service.common.types.IntUnsignedLong;
import ccsds.sle.transfer.service.common.types.ParameterName;
import ccsds.sle.transfer.service.common.types.SpaceLinkDataUnit;
import ccsds.sle.transfer.service.common.types.Time;
public class FspNotification implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public byte[] code = null;
private PacketIdentificationList packetProcessingStarted = null;
private PacketRadiatedInfo packetRadiated = null;
private PacketIdentificationList packetAcknowledged = null;
private PacketIdentificationList slduExpired = null;
private PacketIdentificationList packetTransmissionModeMismatch = null;
private FopAlert transmissionModeCapabilityChange = null;
private BerNull bufferEmpty = null;
private BerNull noInvokeDirectiveCapabilityOnthisVC = null;
private BerNull invokeDirectiveCapabilityOnthisVC = null;
private DirectiveExecutedId positiveConfirmResponceToDirective = null;
private NegativeConfirmResponseToDirective negativeConfirmResponseToDirective = null;
private PacketIdentificationList vcAborted = null;
private PacketIdentificationList productionInterrupted = null;
private PacketIdentificationList productionHalted = null;
private BerNull productionOperational = null;
private EventInvocationId actionListCompleted = null;
private EventInvocationId actionListNotCompleted = null;
private EventInvocationId eventConditionEvaluatedToFalse = null;
public FspNotification() {
}
public FspNotification(byte[] code) {
this.code = code;
}
public void setPacketProcessingStarted(PacketIdentificationList packetProcessingStarted) {
this.packetProcessingStarted = packetProcessingStarted;
}
public PacketIdentificationList getPacketProcessingStarted() {
return packetProcessingStarted;
}
public void setPacketRadiated(PacketRadiatedInfo packetRadiated) {
this.packetRadiated = packetRadiated;
}
public PacketRadiatedInfo getPacketRadiated() {
return packetRadiated;
}
public void setPacketAcknowledged(PacketIdentificationList packetAcknowledged) {
this.packetAcknowledged = packetAcknowledged;
}
public PacketIdentificationList getPacketAcknowledged() {
return packetAcknowledged;
}
public void setSlduExpired(PacketIdentificationList slduExpired) {
this.slduExpired = slduExpired;
}
public PacketIdentificationList getSlduExpired() {
return slduExpired;
}
public void setPacketTransmissionModeMismatch(PacketIdentificationList packetTransmissionModeMismatch) {
this.packetTransmissionModeMismatch = packetTransmissionModeMismatch;
}
public PacketIdentificationList getPacketTransmissionModeMismatch() {
return packetTransmissionModeMismatch;
}
public void setTransmissionModeCapabilityChange(FopAlert transmissionModeCapabilityChange) {
this.transmissionModeCapabilityChange = transmissionModeCapabilityChange;
}
public FopAlert getTransmissionModeCapabilityChange() {
return transmissionModeCapabilityChange;
}
public void setBufferEmpty(BerNull bufferEmpty) {
this.bufferEmpty = bufferEmpty;
}
public BerNull getBufferEmpty() {
return bufferEmpty;
}
public void setNoInvokeDirectiveCapabilityOnthisVC(BerNull noInvokeDirectiveCapabilityOnthisVC) {
this.noInvokeDirectiveCapabilityOnthisVC = noInvokeDirectiveCapabilityOnthisVC;
}
public BerNull getNoInvokeDirectiveCapabilityOnthisVC() {
return noInvokeDirectiveCapabilityOnthisVC;
}
public void setInvokeDirectiveCapabilityOnthisVC(BerNull invokeDirectiveCapabilityOnthisVC) {
this.invokeDirectiveCapabilityOnthisVC = invokeDirectiveCapabilityOnthisVC;
}
public BerNull getInvokeDirectiveCapabilityOnthisVC() {
return invokeDirectiveCapabilityOnthisVC;
}
public void setPositiveConfirmResponceToDirective(DirectiveExecutedId positiveConfirmResponceToDirective) {
this.positiveConfirmResponceToDirective = positiveConfirmResponceToDirective;
}
public DirectiveExecutedId getPositiveConfirmResponceToDirective() {
return positiveConfirmResponceToDirective;
}
public void setNegativeConfirmResponseToDirective(NegativeConfirmResponseToDirective negativeConfirmResponseToDirective) {
this.negativeConfirmResponseToDirective = negativeConfirmResponseToDirective;
}
public NegativeConfirmResponseToDirective getNegativeConfirmResponseToDirective() {
return negativeConfirmResponseToDirective;
}
public void setVcAborted(PacketIdentificationList vcAborted) {
this.vcAborted = vcAborted;
}
public PacketIdentificationList getVcAborted() {
return vcAborted;
}
public void setProductionInterrupted(PacketIdentificationList productionInterrupted) {
this.productionInterrupted = productionInterrupted;
}
public PacketIdentificationList getProductionInterrupted() {
return productionInterrupted;
}
public void setProductionHalted(PacketIdentificationList productionHalted) {
this.productionHalted = productionHalted;
}
public PacketIdentificationList getProductionHalted() {
return productionHalted;
}
public void setProductionOperational(BerNull productionOperational) {
this.productionOperational = productionOperational;
}
public BerNull getProductionOperational() {
return productionOperational;
}
public void setActionListCompleted(EventInvocationId actionListCompleted) {
this.actionListCompleted = actionListCompleted;
}
public EventInvocationId getActionListCompleted() {
return actionListCompleted;
}
public void setActionListNotCompleted(EventInvocationId actionListNotCompleted) {
this.actionListNotCompleted = actionListNotCompleted;
}
public EventInvocationId getActionListNotCompleted() {
return actionListNotCompleted;
}
public void setEventConditionEvaluatedToFalse(EventInvocationId eventConditionEvaluatedToFalse) {
this.eventConditionEvaluatedToFalse = eventConditionEvaluatedToFalse;
}
public EventInvocationId getEventConditionEvaluatedToFalse() {
return eventConditionEvaluatedToFalse;
}
public int encode(OutputStream reverseOS) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
return code.length;
}
int codeLength = 0;
if (eventConditionEvaluatedToFalse != null) {
codeLength += eventConditionEvaluatedToFalse.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 16
reverseOS.write(0x90);
codeLength += 1;
return codeLength;
}
if (actionListNotCompleted != null) {
codeLength += actionListNotCompleted.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 15
reverseOS.write(0x8F);
codeLength += 1;
return codeLength;
}
if (actionListCompleted != null) {
codeLength += actionListCompleted.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 14
reverseOS.write(0x8E);
codeLength += 1;
return codeLength;
}
if (productionOperational != null) {
codeLength += productionOperational.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 13
reverseOS.write(0x8D);
codeLength += 1;
return codeLength;
}
if (productionHalted != null) {
codeLength += productionHalted.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 12
reverseOS.write(0xAC);
codeLength += 1;
return codeLength;
}
if (productionInterrupted != null) {
codeLength += productionInterrupted.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 11
reverseOS.write(0xAB);
codeLength += 1;
return codeLength;
}
if (vcAborted != null) {
codeLength += vcAborted.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 10
reverseOS.write(0xAA);
codeLength += 1;
return codeLength;
}
if (negativeConfirmResponseToDirective != null) {
codeLength += negativeConfirmResponseToDirective.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 9
reverseOS.write(0xA9);
codeLength += 1;
return codeLength;
}
if (positiveConfirmResponceToDirective != null) {
codeLength += positiveConfirmResponceToDirective.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 8
reverseOS.write(0x88);
codeLength += 1;
return codeLength;
}
if (invokeDirectiveCapabilityOnthisVC != null) {
codeLength += invokeDirectiveCapabilityOnthisVC.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 17
reverseOS.write(0x91);
codeLength += 1;
return codeLength;
}
if (noInvokeDirectiveCapabilityOnthisVC != null) {
codeLength += noInvokeDirectiveCapabilityOnthisVC.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 7
reverseOS.write(0x87);
codeLength += 1;
return codeLength;
}
if (bufferEmpty != null) {
codeLength += bufferEmpty.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 6
reverseOS.write(0x86);
codeLength += 1;
return codeLength;
}
if (transmissionModeCapabilityChange != null) {
codeLength += transmissionModeCapabilityChange.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 5
reverseOS.write(0x85);
codeLength += 1;
return codeLength;
}
if (packetTransmissionModeMismatch != null) {
codeLength += packetTransmissionModeMismatch.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 4
reverseOS.write(0xA4);
codeLength += 1;
return codeLength;
}
if (slduExpired != null) {
codeLength += slduExpired.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 3
reverseOS.write(0xA3);
codeLength += 1;
return codeLength;
}
if (packetAcknowledged != null) {
codeLength += packetAcknowledged.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 2
reverseOS.write(0xA2);
codeLength += 1;
return codeLength;
}
if (packetRadiated != null) {
codeLength += packetRadiated.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 1
reverseOS.write(0xA1);
codeLength += 1;
return codeLength;
}
if (packetProcessingStarted != null) {
codeLength += packetProcessingStarted.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 0
reverseOS.write(0xA0);
codeLength += 1;
return codeLength;
}
throw new IOException("Error encoding CHOICE: No element of CHOICE was selected.");
}
public int decode(InputStream is) throws IOException {
return decode(is, null);
}
public int decode(InputStream is, BerTag berTag) throws IOException {
int codeLength = 0;
BerTag passedTag = berTag;
if (berTag == null) {
berTag = new BerTag();
codeLength += berTag.decode(is);
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 0)) {
packetProcessingStarted = new PacketIdentificationList();
codeLength += packetProcessingStarted.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 1)) {
packetRadiated = new PacketRadiatedInfo();
codeLength += packetRadiated.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 2)) {
packetAcknowledged = new PacketIdentificationList();
codeLength += packetAcknowledged.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 3)) {
slduExpired = new PacketIdentificationList();
codeLength += slduExpired.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 4)) {
packetTransmissionModeMismatch = new PacketIdentificationList();
codeLength += packetTransmissionModeMismatch.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 5)) {
transmissionModeCapabilityChange = new FopAlert();
codeLength += transmissionModeCapabilityChange.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 6)) {
bufferEmpty = new BerNull();
codeLength += bufferEmpty.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 7)) {
noInvokeDirectiveCapabilityOnthisVC = new BerNull();
codeLength += noInvokeDirectiveCapabilityOnthisVC.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 17)) {
invokeDirectiveCapabilityOnthisVC = new BerNull();
codeLength += invokeDirectiveCapabilityOnthisVC.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 8)) {
positiveConfirmResponceToDirective = new DirectiveExecutedId();
codeLength += positiveConfirmResponceToDirective.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 9)) {
negativeConfirmResponseToDirective = new NegativeConfirmResponseToDirective();
codeLength += negativeConfirmResponseToDirective.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 10)) {
vcAborted = new PacketIdentificationList();
codeLength += vcAborted.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 11)) {
productionInterrupted = new PacketIdentificationList();
codeLength += productionInterrupted.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 12)) {
productionHalted = new PacketIdentificationList();
codeLength += productionHalted.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 13)) {
productionOperational = new BerNull();
codeLength += productionOperational.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 14)) {
actionListCompleted = new EventInvocationId();
codeLength += actionListCompleted.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 15)) {
actionListNotCompleted = new EventInvocationId();
codeLength += actionListNotCompleted.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 16)) {
eventConditionEvaluatedToFalse = new EventInvocationId();
codeLength += eventConditionEvaluatedToFalse.decode(is, false);
return codeLength;
}
if (passedTag != null) {
return 0;
}
throw new IOException("Error decoding CHOICE: Tag " + berTag + " matched to no item.");
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
if (packetProcessingStarted != null) {
sb.append("packetProcessingStarted: ");
packetProcessingStarted.appendAsString(sb, indentLevel + 1);
return;
}
if (packetRadiated != null) {
sb.append("packetRadiated: ");
packetRadiated.appendAsString(sb, indentLevel + 1);
return;
}
if (packetAcknowledged != null) {
sb.append("packetAcknowledged: ");
packetAcknowledged.appendAsString(sb, indentLevel + 1);
return;
}
if (slduExpired != null) {
sb.append("slduExpired: ");
slduExpired.appendAsString(sb, indentLevel + 1);
return;
}
if (packetTransmissionModeMismatch != null) {
sb.append("packetTransmissionModeMismatch: ");
packetTransmissionModeMismatch.appendAsString(sb, indentLevel + 1);
return;
}
if (transmissionModeCapabilityChange != null) {
sb.append("transmissionModeCapabilityChange: ").append(transmissionModeCapabilityChange);
return;
}
if (bufferEmpty != null) {
sb.append("bufferEmpty: ").append(bufferEmpty);
return;
}
if (noInvokeDirectiveCapabilityOnthisVC != null) {
sb.append("noInvokeDirectiveCapabilityOnthisVC: ").append(noInvokeDirectiveCapabilityOnthisVC);
return;
}
if (invokeDirectiveCapabilityOnthisVC != null) {
sb.append("invokeDirectiveCapabilityOnthisVC: ").append(invokeDirectiveCapabilityOnthisVC);
return;
}
if (positiveConfirmResponceToDirective != null) {
sb.append("positiveConfirmResponceToDirective: ").append(positiveConfirmResponceToDirective);
return;
}
if (negativeConfirmResponseToDirective != null) {
sb.append("negativeConfirmResponseToDirective: ");
negativeConfirmResponseToDirective.appendAsString(sb, indentLevel + 1);
return;
}
if (vcAborted != null) {
sb.append("vcAborted: ");
vcAborted.appendAsString(sb, indentLevel + 1);
return;
}
if (productionInterrupted != null) {
sb.append("productionInterrupted: ");
productionInterrupted.appendAsString(sb, indentLevel + 1);
return;
}
if (productionHalted != null) {
sb.append("productionHalted: ");
productionHalted.appendAsString(sb, indentLevel + 1);
return;
}
if (productionOperational != null) {
sb.append("productionOperational: ").append(productionOperational);
return;
}
if (actionListCompleted != null) {
sb.append("actionListCompleted: ").append(actionListCompleted);
return;
}
if (actionListNotCompleted != null) {
sb.append("actionListNotCompleted: ").append(actionListNotCompleted);
return;
}
if (eventConditionEvaluatedToFalse != null) {
sb.append("eventConditionEvaluatedToFalse: ").append(eventConditionEvaluatedToFalse);
return;
}
sb.append("");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy