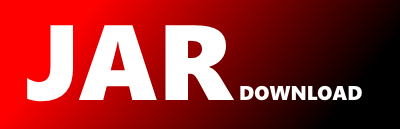
ccsds.sle.transfer.service.rcf.incoming.pdus.RcfUserToProviderPdu Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsle Show documentation
Show all versions of jsle Show documentation
Java implementation for the SLE (Space Link Extension) protocol.
/**
* This class file was automatically generated by jASN1 v1.11.2 (http://www.beanit.com)
*/
package ccsds.sle.transfer.service.rcf.incoming.pdus;
import java.io.IOException;
import java.io.EOFException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.ArrayList;
import java.util.Iterator;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.io.Serializable;
import com.beanit.jasn1.ber.*;
import com.beanit.jasn1.ber.types.*;
import com.beanit.jasn1.ber.types.string.*;
import ccsds.sle.transfer.service.bind.types.SleBindInvocation;
import ccsds.sle.transfer.service.bind.types.SleBindReturn;
import ccsds.sle.transfer.service.bind.types.SlePeerAbort;
import ccsds.sle.transfer.service.bind.types.SleUnbindInvocation;
import ccsds.sle.transfer.service.bind.types.SleUnbindReturn;
import ccsds.sle.transfer.service.common.pdus.SleScheduleStatusReportInvocation;
import ccsds.sle.transfer.service.common.pdus.SleStopInvocation;
import ccsds.sle.transfer.service.common.types.ConditionalTime;
import ccsds.sle.transfer.service.common.types.Credentials;
import ccsds.sle.transfer.service.common.types.InvokeId;
import ccsds.sle.transfer.service.rcf.structures.GvcId;
import ccsds.sle.transfer.service.rcf.structures.RcfParameterName;
public class RcfUserToProviderPdu implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public byte[] code = null;
private SleBindInvocation rcfBindInvocation = null;
private SleBindReturn rcfBindReturn = null;
private SleUnbindInvocation rcfUnbindInvocation = null;
private SleUnbindReturn rcfUnbindReturn = null;
private RcfStartInvocation rcfStartInvocation = null;
private SleStopInvocation rcfStopInvocation = null;
private SleScheduleStatusReportInvocation rcfScheduleStatusReportInvocation = null;
private RcfGetParameterInvocation rcfGetParameterInvocation = null;
private SlePeerAbort rcfPeerAbortInvocation = null;
public RcfUserToProviderPdu() {
}
public RcfUserToProviderPdu(byte[] code) {
this.code = code;
}
public void setRcfBindInvocation(SleBindInvocation rcfBindInvocation) {
this.rcfBindInvocation = rcfBindInvocation;
}
public SleBindInvocation getRcfBindInvocation() {
return rcfBindInvocation;
}
public void setRcfBindReturn(SleBindReturn rcfBindReturn) {
this.rcfBindReturn = rcfBindReturn;
}
public SleBindReturn getRcfBindReturn() {
return rcfBindReturn;
}
public void setRcfUnbindInvocation(SleUnbindInvocation rcfUnbindInvocation) {
this.rcfUnbindInvocation = rcfUnbindInvocation;
}
public SleUnbindInvocation getRcfUnbindInvocation() {
return rcfUnbindInvocation;
}
public void setRcfUnbindReturn(SleUnbindReturn rcfUnbindReturn) {
this.rcfUnbindReturn = rcfUnbindReturn;
}
public SleUnbindReturn getRcfUnbindReturn() {
return rcfUnbindReturn;
}
public void setRcfStartInvocation(RcfStartInvocation rcfStartInvocation) {
this.rcfStartInvocation = rcfStartInvocation;
}
public RcfStartInvocation getRcfStartInvocation() {
return rcfStartInvocation;
}
public void setRcfStopInvocation(SleStopInvocation rcfStopInvocation) {
this.rcfStopInvocation = rcfStopInvocation;
}
public SleStopInvocation getRcfStopInvocation() {
return rcfStopInvocation;
}
public void setRcfScheduleStatusReportInvocation(SleScheduleStatusReportInvocation rcfScheduleStatusReportInvocation) {
this.rcfScheduleStatusReportInvocation = rcfScheduleStatusReportInvocation;
}
public SleScheduleStatusReportInvocation getRcfScheduleStatusReportInvocation() {
return rcfScheduleStatusReportInvocation;
}
public void setRcfGetParameterInvocation(RcfGetParameterInvocation rcfGetParameterInvocation) {
this.rcfGetParameterInvocation = rcfGetParameterInvocation;
}
public RcfGetParameterInvocation getRcfGetParameterInvocation() {
return rcfGetParameterInvocation;
}
public void setRcfPeerAbortInvocation(SlePeerAbort rcfPeerAbortInvocation) {
this.rcfPeerAbortInvocation = rcfPeerAbortInvocation;
}
public SlePeerAbort getRcfPeerAbortInvocation() {
return rcfPeerAbortInvocation;
}
public int encode(OutputStream reverseOS) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
return code.length;
}
int codeLength = 0;
if (rcfPeerAbortInvocation != null) {
codeLength += rcfPeerAbortInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 104
reverseOS.write(0x68);
reverseOS.write(0x9F);
codeLength += 2;
return codeLength;
}
if (rcfGetParameterInvocation != null) {
codeLength += rcfGetParameterInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 6
reverseOS.write(0xA6);
codeLength += 1;
return codeLength;
}
if (rcfScheduleStatusReportInvocation != null) {
codeLength += rcfScheduleStatusReportInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 4
reverseOS.write(0xA4);
codeLength += 1;
return codeLength;
}
if (rcfStopInvocation != null) {
codeLength += rcfStopInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 2
reverseOS.write(0xA2);
codeLength += 1;
return codeLength;
}
if (rcfStartInvocation != null) {
codeLength += rcfStartInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 0
reverseOS.write(0xA0);
codeLength += 1;
return codeLength;
}
if (rcfUnbindReturn != null) {
codeLength += rcfUnbindReturn.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 103
reverseOS.write(0x67);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
if (rcfUnbindInvocation != null) {
codeLength += rcfUnbindInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 102
reverseOS.write(0x66);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
if (rcfBindReturn != null) {
codeLength += rcfBindReturn.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 101
reverseOS.write(0x65);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
if (rcfBindInvocation != null) {
codeLength += rcfBindInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 100
reverseOS.write(0x64);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
throw new IOException("Error encoding CHOICE: No element of CHOICE was selected.");
}
public int decode(InputStream is) throws IOException {
return decode(is, null);
}
public int decode(InputStream is, BerTag berTag) throws IOException {
int codeLength = 0;
BerTag passedTag = berTag;
if (berTag == null) {
berTag = new BerTag();
codeLength += berTag.decode(is);
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 100)) {
rcfBindInvocation = new SleBindInvocation();
codeLength += rcfBindInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 101)) {
rcfBindReturn = new SleBindReturn();
codeLength += rcfBindReturn.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 102)) {
rcfUnbindInvocation = new SleUnbindInvocation();
codeLength += rcfUnbindInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 103)) {
rcfUnbindReturn = new SleUnbindReturn();
codeLength += rcfUnbindReturn.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 0)) {
rcfStartInvocation = new RcfStartInvocation();
codeLength += rcfStartInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 2)) {
rcfStopInvocation = new SleStopInvocation();
codeLength += rcfStopInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 4)) {
rcfScheduleStatusReportInvocation = new SleScheduleStatusReportInvocation();
codeLength += rcfScheduleStatusReportInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 6)) {
rcfGetParameterInvocation = new RcfGetParameterInvocation();
codeLength += rcfGetParameterInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 104)) {
rcfPeerAbortInvocation = new SlePeerAbort();
codeLength += rcfPeerAbortInvocation.decode(is, false);
return codeLength;
}
if (passedTag != null) {
return 0;
}
throw new IOException("Error decoding CHOICE: Tag " + berTag + " matched to no item.");
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
if (rcfBindInvocation != null) {
sb.append("rcfBindInvocation: ");
rcfBindInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (rcfBindReturn != null) {
sb.append("rcfBindReturn: ");
rcfBindReturn.appendAsString(sb, indentLevel + 1);
return;
}
if (rcfUnbindInvocation != null) {
sb.append("rcfUnbindInvocation: ");
rcfUnbindInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (rcfUnbindReturn != null) {
sb.append("rcfUnbindReturn: ");
rcfUnbindReturn.appendAsString(sb, indentLevel + 1);
return;
}
if (rcfStartInvocation != null) {
sb.append("rcfStartInvocation: ");
rcfStartInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (rcfStopInvocation != null) {
sb.append("rcfStopInvocation: ");
rcfStopInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (rcfScheduleStatusReportInvocation != null) {
sb.append("rcfScheduleStatusReportInvocation: ");
rcfScheduleStatusReportInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (rcfGetParameterInvocation != null) {
sb.append("rcfGetParameterInvocation: ");
rcfGetParameterInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (rcfPeerAbortInvocation != null) {
sb.append("rcfPeerAbortInvocation: ").append(rcfPeerAbortInvocation);
return;
}
sb.append("");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy