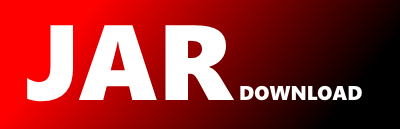
ccsds.sle.transfer.service.rcf.structures.RcfGetParameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsle Show documentation
Show all versions of jsle Show documentation
Java implementation for the SLE (Space Link Extension) protocol.
/**
* This class file was automatically generated by jASN1 v1.11.2 (http://www.beanit.com)
*/
package ccsds.sle.transfer.service.rcf.structures;
import java.io.IOException;
import java.io.EOFException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.ArrayList;
import java.util.Iterator;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.io.Serializable;
import com.beanit.jasn1.ber.*;
import com.beanit.jasn1.ber.types.*;
import com.beanit.jasn1.ber.types.string.*;
import ccsds.sle.transfer.service.common.pdus.ReportingCycle;
import ccsds.sle.transfer.service.common.types.DeliveryMode;
import ccsds.sle.transfer.service.common.types.Diagnostics;
import ccsds.sle.transfer.service.common.types.IntPosShort;
import ccsds.sle.transfer.service.common.types.ParameterName;
import ccsds.sle.transfer.service.common.types.Time;
public class RcfGetParameter implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public byte[] code = null;
public static class ParBufferSize implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private IntPosShort parameterValue = null;
public ParBufferSize() {
}
public ParBufferSize(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(IntPosShort parameterValue) {
this.parameterValue = parameterValue;
}
public IntPosShort getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(IntPosShort.tag)) {
parameterValue = new IntPosShort();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParDeliveryMode implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private RcfDeliveryMode parameterValue = null;
public ParDeliveryMode() {
}
public ParDeliveryMode(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(RcfDeliveryMode parameterValue) {
this.parameterValue = parameterValue;
}
public RcfDeliveryMode getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(RcfDeliveryMode.tag)) {
parameterValue = new RcfDeliveryMode();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParLatencyLimit implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static class ParameterValue implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public byte[] code = null;
private IntPosShort online = null;
private BerNull offline = null;
public ParameterValue() {
}
public ParameterValue(byte[] code) {
this.code = code;
}
public void setOnline(IntPosShort online) {
this.online = online;
}
public IntPosShort getOnline() {
return online;
}
public void setOffline(BerNull offline) {
this.offline = offline;
}
public BerNull getOffline() {
return offline;
}
public int encode(OutputStream reverseOS) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
return code.length;
}
int codeLength = 0;
if (offline != null) {
codeLength += offline.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 1
reverseOS.write(0x81);
codeLength += 1;
return codeLength;
}
if (online != null) {
codeLength += online.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 0
reverseOS.write(0x80);
codeLength += 1;
return codeLength;
}
throw new IOException("Error encoding CHOICE: No element of CHOICE was selected.");
}
public int decode(InputStream is) throws IOException {
return decode(is, null);
}
public int decode(InputStream is, BerTag berTag) throws IOException {
int codeLength = 0;
BerTag passedTag = berTag;
if (berTag == null) {
berTag = new BerTag();
codeLength += berTag.decode(is);
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 0)) {
online = new IntPosShort();
codeLength += online.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 1)) {
offline = new BerNull();
codeLength += offline.decode(is, false);
return codeLength;
}
if (passedTag != null) {
return 0;
}
throw new IOException("Error decoding CHOICE: Tag " + berTag + " matched to no item.");
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
if (online != null) {
sb.append("online: ").append(online);
return;
}
if (offline != null) {
sb.append("offline: ").append(offline);
return;
}
sb.append("");
}
}
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private ParameterValue parameterValue = null;
public ParLatencyLimit() {
}
public ParLatencyLimit(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(ParameterValue parameterValue) {
this.parameterValue = parameterValue;
}
public ParameterValue getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new ParameterValue();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParMinReportingCycle implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private IntPosShort parameterValue = null;
public ParMinReportingCycle() {
}
public ParMinReportingCycle(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(IntPosShort parameterValue) {
this.parameterValue = parameterValue;
}
public IntPosShort getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(IntPosShort.tag)) {
parameterValue = new IntPosShort();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParPermittedGvcidSet implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private GvcIdSet parameterValue = null;
public ParPermittedGvcidSet() {
}
public ParPermittedGvcidSet(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(GvcIdSet parameterValue) {
this.parameterValue = parameterValue;
}
public GvcIdSet getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(GvcIdSet.tag)) {
parameterValue = new GvcIdSet();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParReportingCycle implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private CurrentReportingCycle parameterValue = null;
public ParReportingCycle() {
}
public ParReportingCycle(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(CurrentReportingCycle parameterValue) {
this.parameterValue = parameterValue;
}
public CurrentReportingCycle getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new CurrentReportingCycle();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParReqGvcId implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private RequestedGvcId parameterValue = null;
public ParReqGvcId() {
}
public ParReqGvcId(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(RequestedGvcId parameterValue) {
this.parameterValue = parameterValue;
}
public RequestedGvcId getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
parameterValue = new RequestedGvcId();
subCodeLength += parameterValue.decode(is, berTag);
if (subCodeLength == totalLength) {
return codeLength;
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ");
parameterValue.appendAsString(sb, indentLevel + 1);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
public static class ParReturnTimeout implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public static final BerTag tag = new BerTag(BerTag.UNIVERSAL_CLASS, BerTag.CONSTRUCTED, 16);
public byte[] code = null;
private ParameterName parameterName = null;
private TimeoutPeriod parameterValue = null;
public ParReturnTimeout() {
}
public ParReturnTimeout(byte[] code) {
this.code = code;
}
public void setParameterName(ParameterName parameterName) {
this.parameterName = parameterName;
}
public ParameterName getParameterName() {
return parameterName;
}
public void setParameterValue(TimeoutPeriod parameterValue) {
this.parameterValue = parameterValue;
}
public TimeoutPeriod getParameterValue() {
return parameterValue;
}
public int encode(OutputStream reverseOS) throws IOException {
return encode(reverseOS, true);
}
public int encode(OutputStream reverseOS, boolean withTag) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
if (withTag) {
return tag.encode(reverseOS) + code.length;
}
return code.length;
}
int codeLength = 0;
codeLength += parameterValue.encode(reverseOS, true);
codeLength += parameterName.encode(reverseOS, true);
codeLength += BerLength.encodeLength(reverseOS, codeLength);
if (withTag) {
codeLength += tag.encode(reverseOS);
}
return codeLength;
}
public int decode(InputStream is) throws IOException {
return decode(is, true);
}
public int decode(InputStream is, boolean withTag) throws IOException {
int codeLength = 0;
int subCodeLength = 0;
BerTag berTag = new BerTag();
if (withTag) {
codeLength += tag.decodeAndCheck(is);
}
BerLength length = new BerLength();
codeLength += length.decode(is);
int totalLength = length.val;
codeLength += totalLength;
subCodeLength += berTag.decode(is);
if (berTag.equals(ParameterName.tag)) {
parameterName = new ParameterName();
subCodeLength += parameterName.decode(is, false);
subCodeLength += berTag.decode(is);
}
else {
throw new IOException("Tag does not match the mandatory sequence element tag.");
}
if (berTag.equals(TimeoutPeriod.tag)) {
parameterValue = new TimeoutPeriod();
subCodeLength += parameterValue.decode(is, false);
if (subCodeLength == totalLength) {
return codeLength;
}
}
throw new IOException("Unexpected end of sequence, length tag: " + totalLength + ", actual sequence length: " + subCodeLength);
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS, false);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
sb.append("{");
sb.append("\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterName != null) {
sb.append("parameterName: ").append(parameterName);
}
else {
sb.append("parameterName: ");
}
sb.append(",\n");
for (int i = 0; i < indentLevel + 1; i++) {
sb.append("\t");
}
if (parameterValue != null) {
sb.append("parameterValue: ").append(parameterValue);
}
else {
sb.append("parameterValue: ");
}
sb.append("\n");
for (int i = 0; i < indentLevel; i++) {
sb.append("\t");
}
sb.append("}");
}
}
private ParBufferSize parBufferSize = null;
private ParDeliveryMode parDeliveryMode = null;
private ParLatencyLimit parLatencyLimit = null;
private ParMinReportingCycle parMinReportingCycle = null;
private ParPermittedGvcidSet parPermittedGvcidSet = null;
private ParReportingCycle parReportingCycle = null;
private ParReqGvcId parReqGvcId = null;
private ParReturnTimeout parReturnTimeout = null;
public RcfGetParameter() {
}
public RcfGetParameter(byte[] code) {
this.code = code;
}
public void setParBufferSize(ParBufferSize parBufferSize) {
this.parBufferSize = parBufferSize;
}
public ParBufferSize getParBufferSize() {
return parBufferSize;
}
public void setParDeliveryMode(ParDeliveryMode parDeliveryMode) {
this.parDeliveryMode = parDeliveryMode;
}
public ParDeliveryMode getParDeliveryMode() {
return parDeliveryMode;
}
public void setParLatencyLimit(ParLatencyLimit parLatencyLimit) {
this.parLatencyLimit = parLatencyLimit;
}
public ParLatencyLimit getParLatencyLimit() {
return parLatencyLimit;
}
public void setParMinReportingCycle(ParMinReportingCycle parMinReportingCycle) {
this.parMinReportingCycle = parMinReportingCycle;
}
public ParMinReportingCycle getParMinReportingCycle() {
return parMinReportingCycle;
}
public void setParPermittedGvcidSet(ParPermittedGvcidSet parPermittedGvcidSet) {
this.parPermittedGvcidSet = parPermittedGvcidSet;
}
public ParPermittedGvcidSet getParPermittedGvcidSet() {
return parPermittedGvcidSet;
}
public void setParReportingCycle(ParReportingCycle parReportingCycle) {
this.parReportingCycle = parReportingCycle;
}
public ParReportingCycle getParReportingCycle() {
return parReportingCycle;
}
public void setParReqGvcId(ParReqGvcId parReqGvcId) {
this.parReqGvcId = parReqGvcId;
}
public ParReqGvcId getParReqGvcId() {
return parReqGvcId;
}
public void setParReturnTimeout(ParReturnTimeout parReturnTimeout) {
this.parReturnTimeout = parReturnTimeout;
}
public ParReturnTimeout getParReturnTimeout() {
return parReturnTimeout;
}
public int encode(OutputStream reverseOS) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
return code.length;
}
int codeLength = 0;
if (parReturnTimeout != null) {
codeLength += parReturnTimeout.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 6
reverseOS.write(0xA6);
codeLength += 1;
return codeLength;
}
if (parReqGvcId != null) {
codeLength += parReqGvcId.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 5
reverseOS.write(0xA5);
codeLength += 1;
return codeLength;
}
if (parReportingCycle != null) {
codeLength += parReportingCycle.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 4
reverseOS.write(0xA4);
codeLength += 1;
return codeLength;
}
if (parPermittedGvcidSet != null) {
codeLength += parPermittedGvcidSet.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 3
reverseOS.write(0xA3);
codeLength += 1;
return codeLength;
}
if (parMinReportingCycle != null) {
codeLength += parMinReportingCycle.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 7
reverseOS.write(0xA7);
codeLength += 1;
return codeLength;
}
if (parLatencyLimit != null) {
codeLength += parLatencyLimit.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 2
reverseOS.write(0xA2);
codeLength += 1;
return codeLength;
}
if (parDeliveryMode != null) {
codeLength += parDeliveryMode.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 1
reverseOS.write(0xA1);
codeLength += 1;
return codeLength;
}
if (parBufferSize != null) {
codeLength += parBufferSize.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 0
reverseOS.write(0xA0);
codeLength += 1;
return codeLength;
}
throw new IOException("Error encoding CHOICE: No element of CHOICE was selected.");
}
public int decode(InputStream is) throws IOException {
return decode(is, null);
}
public int decode(InputStream is, BerTag berTag) throws IOException {
int codeLength = 0;
BerTag passedTag = berTag;
if (berTag == null) {
berTag = new BerTag();
codeLength += berTag.decode(is);
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 0)) {
parBufferSize = new ParBufferSize();
codeLength += parBufferSize.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 1)) {
parDeliveryMode = new ParDeliveryMode();
codeLength += parDeliveryMode.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 2)) {
parLatencyLimit = new ParLatencyLimit();
codeLength += parLatencyLimit.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 7)) {
parMinReportingCycle = new ParMinReportingCycle();
codeLength += parMinReportingCycle.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 3)) {
parPermittedGvcidSet = new ParPermittedGvcidSet();
codeLength += parPermittedGvcidSet.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 4)) {
parReportingCycle = new ParReportingCycle();
codeLength += parReportingCycle.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 5)) {
parReqGvcId = new ParReqGvcId();
codeLength += parReqGvcId.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 6)) {
parReturnTimeout = new ParReturnTimeout();
codeLength += parReturnTimeout.decode(is, false);
return codeLength;
}
if (passedTag != null) {
return 0;
}
throw new IOException("Error decoding CHOICE: Tag " + berTag + " matched to no item.");
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
if (parBufferSize != null) {
sb.append("parBufferSize: ");
parBufferSize.appendAsString(sb, indentLevel + 1);
return;
}
if (parDeliveryMode != null) {
sb.append("parDeliveryMode: ");
parDeliveryMode.appendAsString(sb, indentLevel + 1);
return;
}
if (parLatencyLimit != null) {
sb.append("parLatencyLimit: ");
parLatencyLimit.appendAsString(sb, indentLevel + 1);
return;
}
if (parMinReportingCycle != null) {
sb.append("parMinReportingCycle: ");
parMinReportingCycle.appendAsString(sb, indentLevel + 1);
return;
}
if (parPermittedGvcidSet != null) {
sb.append("parPermittedGvcidSet: ");
parPermittedGvcidSet.appendAsString(sb, indentLevel + 1);
return;
}
if (parReportingCycle != null) {
sb.append("parReportingCycle: ");
parReportingCycle.appendAsString(sb, indentLevel + 1);
return;
}
if (parReqGvcId != null) {
sb.append("parReqGvcId: ");
parReqGvcId.appendAsString(sb, indentLevel + 1);
return;
}
if (parReturnTimeout != null) {
sb.append("parReturnTimeout: ");
parReturnTimeout.appendAsString(sb, indentLevel + 1);
return;
}
sb.append("");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy