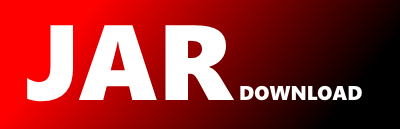
ccsds.sle.transfer.service.rocf.outgoing.pdus.RocfProviderToUserPdu Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsle Show documentation
Show all versions of jsle Show documentation
Java implementation for the SLE (Space Link Extension) protocol.
/**
* This class file was automatically generated by jASN1 v1.11.2 (http://www.beanit.com)
*/
package ccsds.sle.transfer.service.rocf.outgoing.pdus;
import java.io.IOException;
import java.io.EOFException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.ArrayList;
import java.util.Iterator;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.io.Serializable;
import com.beanit.jasn1.ber.*;
import com.beanit.jasn1.ber.types.*;
import com.beanit.jasn1.ber.types.string.*;
import ccsds.sle.transfer.service.bind.types.SleBindInvocation;
import ccsds.sle.transfer.service.bind.types.SleBindReturn;
import ccsds.sle.transfer.service.bind.types.SlePeerAbort;
import ccsds.sle.transfer.service.bind.types.SleUnbindInvocation;
import ccsds.sle.transfer.service.bind.types.SleUnbindReturn;
import ccsds.sle.transfer.service.common.pdus.SleAcknowledgement;
import ccsds.sle.transfer.service.common.pdus.SleScheduleStatusReportReturn;
import ccsds.sle.transfer.service.common.types.Credentials;
import ccsds.sle.transfer.service.common.types.IntUnsignedLong;
import ccsds.sle.transfer.service.common.types.InvokeId;
import ccsds.sle.transfer.service.common.types.SpaceLinkDataUnit;
import ccsds.sle.transfer.service.common.types.Time;
import ccsds.sle.transfer.service.rocf.structures.AntennaId;
import ccsds.sle.transfer.service.rocf.structures.CarrierLockStatus;
import ccsds.sle.transfer.service.rocf.structures.DiagnosticRocfGet;
import ccsds.sle.transfer.service.rocf.structures.DiagnosticRocfStart;
import ccsds.sle.transfer.service.rocf.structures.FrameSyncLockStatus;
import ccsds.sle.transfer.service.rocf.structures.LockStatus;
import ccsds.sle.transfer.service.rocf.structures.Notification;
import ccsds.sle.transfer.service.rocf.structures.RocfGetParameter;
import ccsds.sle.transfer.service.rocf.structures.RocfProductionStatus;
import ccsds.sle.transfer.service.rocf.structures.SymbolLockStatus;
public class RocfProviderToUserPdu implements BerType, Serializable {
private static final long serialVersionUID = 1L;
public byte[] code = null;
private SleBindInvocation rocfBindInvocation = null;
private SleBindReturn rocfBindReturn = null;
private SleUnbindInvocation rocfUnbindInvocation = null;
private SleUnbindReturn rocfUnbindReturn = null;
private RocfStartReturn rocfStartReturn = null;
private SleAcknowledgement rocfStopReturn = null;
private RocfTransferBuffer rocfTransferBuffer = null;
private SleScheduleStatusReportReturn rocfScheduleStatusReportReturn = null;
private RocfStatusReportInvocation rocfStatusReportInvocation = null;
private RocfGetParameterReturn rocfGetParameterReturn = null;
private SlePeerAbort rocfPeerAbortInvocation = null;
public RocfProviderToUserPdu() {
}
public RocfProviderToUserPdu(byte[] code) {
this.code = code;
}
public void setRocfBindInvocation(SleBindInvocation rocfBindInvocation) {
this.rocfBindInvocation = rocfBindInvocation;
}
public SleBindInvocation getRocfBindInvocation() {
return rocfBindInvocation;
}
public void setRocfBindReturn(SleBindReturn rocfBindReturn) {
this.rocfBindReturn = rocfBindReturn;
}
public SleBindReturn getRocfBindReturn() {
return rocfBindReturn;
}
public void setRocfUnbindInvocation(SleUnbindInvocation rocfUnbindInvocation) {
this.rocfUnbindInvocation = rocfUnbindInvocation;
}
public SleUnbindInvocation getRocfUnbindInvocation() {
return rocfUnbindInvocation;
}
public void setRocfUnbindReturn(SleUnbindReturn rocfUnbindReturn) {
this.rocfUnbindReturn = rocfUnbindReturn;
}
public SleUnbindReturn getRocfUnbindReturn() {
return rocfUnbindReturn;
}
public void setRocfStartReturn(RocfStartReturn rocfStartReturn) {
this.rocfStartReturn = rocfStartReturn;
}
public RocfStartReturn getRocfStartReturn() {
return rocfStartReturn;
}
public void setRocfStopReturn(SleAcknowledgement rocfStopReturn) {
this.rocfStopReturn = rocfStopReturn;
}
public SleAcknowledgement getRocfStopReturn() {
return rocfStopReturn;
}
public void setRocfTransferBuffer(RocfTransferBuffer rocfTransferBuffer) {
this.rocfTransferBuffer = rocfTransferBuffer;
}
public RocfTransferBuffer getRocfTransferBuffer() {
return rocfTransferBuffer;
}
public void setRocfScheduleStatusReportReturn(SleScheduleStatusReportReturn rocfScheduleStatusReportReturn) {
this.rocfScheduleStatusReportReturn = rocfScheduleStatusReportReturn;
}
public SleScheduleStatusReportReturn getRocfScheduleStatusReportReturn() {
return rocfScheduleStatusReportReturn;
}
public void setRocfStatusReportInvocation(RocfStatusReportInvocation rocfStatusReportInvocation) {
this.rocfStatusReportInvocation = rocfStatusReportInvocation;
}
public RocfStatusReportInvocation getRocfStatusReportInvocation() {
return rocfStatusReportInvocation;
}
public void setRocfGetParameterReturn(RocfGetParameterReturn rocfGetParameterReturn) {
this.rocfGetParameterReturn = rocfGetParameterReturn;
}
public RocfGetParameterReturn getRocfGetParameterReturn() {
return rocfGetParameterReturn;
}
public void setRocfPeerAbortInvocation(SlePeerAbort rocfPeerAbortInvocation) {
this.rocfPeerAbortInvocation = rocfPeerAbortInvocation;
}
public SlePeerAbort getRocfPeerAbortInvocation() {
return rocfPeerAbortInvocation;
}
public int encode(OutputStream reverseOS) throws IOException {
if (code != null) {
for (int i = code.length - 1; i >= 0; i--) {
reverseOS.write(code[i]);
}
return code.length;
}
int codeLength = 0;
if (rocfPeerAbortInvocation != null) {
codeLength += rocfPeerAbortInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, PRIMITIVE, 104
reverseOS.write(0x68);
reverseOS.write(0x9F);
codeLength += 2;
return codeLength;
}
if (rocfGetParameterReturn != null) {
codeLength += rocfGetParameterReturn.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 7
reverseOS.write(0xA7);
codeLength += 1;
return codeLength;
}
if (rocfStatusReportInvocation != null) {
codeLength += rocfStatusReportInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 9
reverseOS.write(0xA9);
codeLength += 1;
return codeLength;
}
if (rocfScheduleStatusReportReturn != null) {
codeLength += rocfScheduleStatusReportReturn.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 5
reverseOS.write(0xA5);
codeLength += 1;
return codeLength;
}
if (rocfTransferBuffer != null) {
codeLength += rocfTransferBuffer.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 8
reverseOS.write(0xA8);
codeLength += 1;
return codeLength;
}
if (rocfStopReturn != null) {
codeLength += rocfStopReturn.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 3
reverseOS.write(0xA3);
codeLength += 1;
return codeLength;
}
if (rocfStartReturn != null) {
codeLength += rocfStartReturn.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 1
reverseOS.write(0xA1);
codeLength += 1;
return codeLength;
}
if (rocfUnbindReturn != null) {
codeLength += rocfUnbindReturn.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 103
reverseOS.write(0x67);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
if (rocfUnbindInvocation != null) {
codeLength += rocfUnbindInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 102
reverseOS.write(0x66);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
if (rocfBindReturn != null) {
codeLength += rocfBindReturn.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 101
reverseOS.write(0x65);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
if (rocfBindInvocation != null) {
codeLength += rocfBindInvocation.encode(reverseOS, false);
// write tag: CONTEXT_CLASS, CONSTRUCTED, 100
reverseOS.write(0x64);
reverseOS.write(0xBF);
codeLength += 2;
return codeLength;
}
throw new IOException("Error encoding CHOICE: No element of CHOICE was selected.");
}
public int decode(InputStream is) throws IOException {
return decode(is, null);
}
public int decode(InputStream is, BerTag berTag) throws IOException {
int codeLength = 0;
BerTag passedTag = berTag;
if (berTag == null) {
berTag = new BerTag();
codeLength += berTag.decode(is);
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 100)) {
rocfBindInvocation = new SleBindInvocation();
codeLength += rocfBindInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 101)) {
rocfBindReturn = new SleBindReturn();
codeLength += rocfBindReturn.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 102)) {
rocfUnbindInvocation = new SleUnbindInvocation();
codeLength += rocfUnbindInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 103)) {
rocfUnbindReturn = new SleUnbindReturn();
codeLength += rocfUnbindReturn.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 1)) {
rocfStartReturn = new RocfStartReturn();
codeLength += rocfStartReturn.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 3)) {
rocfStopReturn = new SleAcknowledgement();
codeLength += rocfStopReturn.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 8)) {
rocfTransferBuffer = new RocfTransferBuffer();
codeLength += rocfTransferBuffer.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 5)) {
rocfScheduleStatusReportReturn = new SleScheduleStatusReportReturn();
codeLength += rocfScheduleStatusReportReturn.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 9)) {
rocfStatusReportInvocation = new RocfStatusReportInvocation();
codeLength += rocfStatusReportInvocation.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.CONSTRUCTED, 7)) {
rocfGetParameterReturn = new RocfGetParameterReturn();
codeLength += rocfGetParameterReturn.decode(is, false);
return codeLength;
}
if (berTag.equals(BerTag.CONTEXT_CLASS, BerTag.PRIMITIVE, 104)) {
rocfPeerAbortInvocation = new SlePeerAbort();
codeLength += rocfPeerAbortInvocation.decode(is, false);
return codeLength;
}
if (passedTag != null) {
return 0;
}
throw new IOException("Error decoding CHOICE: Tag " + berTag + " matched to no item.");
}
public void encodeAndSave(int encodingSizeGuess) throws IOException {
ReverseByteArrayOutputStream reverseOS = new ReverseByteArrayOutputStream(encodingSizeGuess);
encode(reverseOS);
code = reverseOS.getArray();
}
public String toString() {
StringBuilder sb = new StringBuilder();
appendAsString(sb, 0);
return sb.toString();
}
public void appendAsString(StringBuilder sb, int indentLevel) {
if (rocfBindInvocation != null) {
sb.append("rocfBindInvocation: ");
rocfBindInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfBindReturn != null) {
sb.append("rocfBindReturn: ");
rocfBindReturn.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfUnbindInvocation != null) {
sb.append("rocfUnbindInvocation: ");
rocfUnbindInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfUnbindReturn != null) {
sb.append("rocfUnbindReturn: ");
rocfUnbindReturn.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfStartReturn != null) {
sb.append("rocfStartReturn: ");
rocfStartReturn.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfStopReturn != null) {
sb.append("rocfStopReturn: ");
rocfStopReturn.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfTransferBuffer != null) {
sb.append("rocfTransferBuffer: ");
rocfTransferBuffer.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfScheduleStatusReportReturn != null) {
sb.append("rocfScheduleStatusReportReturn: ");
rocfScheduleStatusReportReturn.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfStatusReportInvocation != null) {
sb.append("rocfStatusReportInvocation: ");
rocfStatusReportInvocation.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfGetParameterReturn != null) {
sb.append("rocfGetParameterReturn: ");
rocfGetParameterReturn.appendAsString(sb, indentLevel + 1);
return;
}
if (rocfPeerAbortInvocation != null) {
sb.append("rocfPeerAbortInvocation: ").append(rocfPeerAbortInvocation);
return;
}
sb.append("");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy