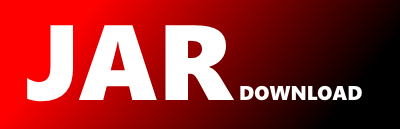
org.yamcs.api.HttpRouteOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: yamcs/api/annotations.proto
package org.yamcs.api;
public interface HttpRouteOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.api.HttpRoute)
com.google.protobuf.MessageOrBuilder {
/**
*
* Maps to HTTP GET. Used for listing and getting information about
* resources.
*
*
* string get = 1;
* @return Whether the get field is set.
*/
boolean hasGet();
/**
*
* Maps to HTTP GET. Used for listing and getting information about
* resources.
*
*
* string get = 1;
* @return The get.
*/
java.lang.String getGet();
/**
*
* Maps to HTTP GET. Used for listing and getting information about
* resources.
*
*
* string get = 1;
* @return The bytes for get.
*/
com.google.protobuf.ByteString
getGetBytes();
/**
*
* Maps to HTTP PUT. Used for replacing a resource.
*
*
* string put = 2;
* @return Whether the put field is set.
*/
boolean hasPut();
/**
*
* Maps to HTTP PUT. Used for replacing a resource.
*
*
* string put = 2;
* @return The put.
*/
java.lang.String getPut();
/**
*
* Maps to HTTP PUT. Used for replacing a resource.
*
*
* string put = 2;
* @return The bytes for put.
*/
com.google.protobuf.ByteString
getPutBytes();
/**
*
* Maps to HTTP POST. Used for creating a resource or performing an action.
*
*
* string post = 3;
* @return Whether the post field is set.
*/
boolean hasPost();
/**
*
* Maps to HTTP POST. Used for creating a resource or performing an action.
*
*
* string post = 3;
* @return The post.
*/
java.lang.String getPost();
/**
*
* Maps to HTTP POST. Used for creating a resource or performing an action.
*
*
* string post = 3;
* @return The bytes for post.
*/
com.google.protobuf.ByteString
getPostBytes();
/**
*
* Maps to HTTP DELETE. Used for deleting a resource.
*
*
* string delete = 4;
* @return Whether the delete field is set.
*/
boolean hasDelete();
/**
*
* Maps to HTTP DELETE. Used for deleting a resource.
*
*
* string delete = 4;
* @return The delete.
*/
java.lang.String getDelete();
/**
*
* Maps to HTTP DELETE. Used for deleting a resource.
*
*
* string delete = 4;
* @return The bytes for delete.
*/
com.google.protobuf.ByteString
getDeleteBytes();
/**
*
* Maps to HTTP PATCH. Used for updating a resource.
*
*
* string patch = 5;
* @return Whether the patch field is set.
*/
boolean hasPatch();
/**
*
* Maps to HTTP PATCH. Used for updating a resource.
*
*
* string patch = 5;
* @return The patch.
*/
java.lang.String getPatch();
/**
*
* Maps to HTTP PATCH. Used for updating a resource.
*
*
* string patch = 5;
* @return The bytes for patch.
*/
com.google.protobuf.ByteString
getPatchBytes();
/**
* optional bool deprecated = 6;
* @return Whether the deprecated field is set.
*/
boolean hasDeprecated();
/**
* optional bool deprecated = 6;
* @return The deprecated.
*/
boolean getDeprecated();
/**
*
* Name of the field in the request message that maps to the request body
* The special value "*" indicates that the request message as a whole
* represents the body (excepting route params).
*
*
* optional string body = 7;
* @return Whether the body field is set.
*/
boolean hasBody();
/**
*
* Name of the field in the request message that maps to the request body
* The special value "*" indicates that the request message as a whole
* represents the body (excepting route params).
*
*
* optional string body = 7;
* @return The body.
*/
java.lang.String getBody();
/**
*
* Name of the field in the request message that maps to the request body
* The special value "*" indicates that the request message as a whole
* represents the body (excepting route params).
*
*
* optional string body = 7;
* @return The bytes for body.
*/
com.google.protobuf.ByteString
getBodyBytes();
/**
* optional int32 max_body_size = 8;
* @return Whether the maxBodySize field is set.
*/
boolean hasMaxBodySize();
/**
* optional int32 max_body_size = 8;
* @return The maxBodySize.
*/
int getMaxBodySize();
/**
*
* Set true if the execution of the route is expected to take a long time
* (more than 0.5 seconds). It will be offloaded to a worker thread.
* Leave false if the route uses its own threading mechanism (most of the
* routes should do that).
*
*
* optional bool offloaded = 9;
* @return Whether the offloaded field is set.
*/
boolean hasOffloaded();
/**
*
* Set true if the execution of the route is expected to take a long time
* (more than 0.5 seconds). It will be offloaded to a worker thread.
* Leave false if the route uses its own threading mechanism (most of the
* routes should do that).
*
*
* optional bool offloaded = 9;
* @return The offloaded.
*/
boolean getOffloaded();
/**
*
* Name of the field in the response message where a user-specified field
* mask is applied to. If this indicates a repeated field, the field mask
* is applied to each of those messages.
* If unspecified, Yamcs will try to derive this itself.
*
*
* optional string field_mask_root = 10;
* @return Whether the fieldMaskRoot field is set.
*/
boolean hasFieldMaskRoot();
/**
*
* Name of the field in the response message where a user-specified field
* mask is applied to. If this indicates a repeated field, the field mask
* is applied to each of those messages.
* If unspecified, Yamcs will try to derive this itself.
*
*
* optional string field_mask_root = 10;
* @return The fieldMaskRoot.
*/
java.lang.String getFieldMaskRoot();
/**
*
* Name of the field in the response message where a user-specified field
* mask is applied to. If this indicates a repeated field, the field mask
* is applied to each of those messages.
* If unspecified, Yamcs will try to derive this itself.
*
*
* optional string field_mask_root = 10;
* @return The bytes for fieldMaskRoot.
*/
com.google.protobuf.ByteString
getFieldMaskRootBytes();
/**
* repeated .yamcs.api.HttpRoute additional_bindings = 11;
*/
java.util.List
getAdditionalBindingsList();
/**
* repeated .yamcs.api.HttpRoute additional_bindings = 11;
*/
org.yamcs.api.HttpRoute getAdditionalBindings(int index);
/**
* repeated .yamcs.api.HttpRoute additional_bindings = 11;
*/
int getAdditionalBindingsCount();
/**
* repeated .yamcs.api.HttpRoute additional_bindings = 11;
*/
java.util.List extends org.yamcs.api.HttpRouteOrBuilder>
getAdditionalBindingsOrBuilderList();
/**
* repeated .yamcs.api.HttpRoute additional_bindings = 11;
*/
org.yamcs.api.HttpRouteOrBuilder getAdditionalBindingsOrBuilder(
int index);
/**
*
* Human-friendly log message format.
*
*
* optional string log = 12;
* @return Whether the log field is set.
*/
boolean hasLog();
/**
*
* Human-friendly log message format.
*
*
* optional string log = 12;
* @return The log.
*/
java.lang.String getLog();
/**
*
* Human-friendly log message format.
*
*
* optional string log = 12;
* @return The bytes for log.
*/
com.google.protobuf.ByteString
getLogBytes();
/**
*
* Optional method label. This is a hint to programs that process
* proto definitions (example: document generation), intended for
* when the camel-case method name does not give a good result.
*
*
* optional string label = 13;
* @return Whether the label field is set.
*/
boolean hasLabel();
/**
*
* Optional method label. This is a hint to programs that process
* proto definitions (example: document generation), intended for
* when the camel-case method name does not give a good result.
*
*
* optional string label = 13;
* @return The label.
*/
java.lang.String getLabel();
/**
*
* Optional method label. This is a hint to programs that process
* proto definitions (example: document generation), intended for
* when the camel-case method name does not give a good result.
*
*
* optional string label = 13;
* @return The bytes for label.
*/
com.google.protobuf.ByteString
getLabelBytes();
public org.yamcs.api.HttpRoute.PatternCase getPatternCase();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy