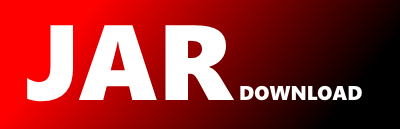
org.yamcs.protobuf.AbstractCop1Api Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
package org.yamcs.protobuf;
import com.google.protobuf.Descriptors.MethodDescriptor;
import com.google.protobuf.Descriptors.ServiceDescriptor;
import com.google.protobuf.Empty;
import com.google.protobuf.Message;
import org.yamcs.api.Api;
import org.yamcs.api.Observer;
/**
*
* Methods for virtual channel TC links that have ``useCop1: true``.
* This service contains methods for setting/getting the configuration and
* performing various operations.
* In addition, a websocket subscription is available that will allow receiving periodically the status.
*
*/
@javax.annotation.processing.Generated(value = "org.yamcs.maven.ServiceGenerator", date = "2025-02-13T11:12:59.906745953Z")
@SuppressWarnings("unchecked")
public abstract class AbstractCop1Api implements Api {
/**
*
* Initialize COP-1 in case state is UNITIALIZED
*
*/
public abstract void initialize(T ctx, InitializeRequest request, Observer observer);
/**
*
* Resume COP-1 operation in case state is SUSPENDED
*
*/
public abstract void resume(T ctx, ResumeRequest request, Observer observer);
/**
*
* Disable COP-1 operation
*
* This causes the sent queue to be purged.
* All TCs from the wait queue, as well as newly received TCs
* are sent immediately
*
*/
public abstract void disable(T ctx, DisableRequest request, Observer observer);
/**
*
* Update configuration settings
*
*/
public abstract void updateConfig(T ctx, UpdateConfigRequest request, Observer observer);
/**
*
* Get COP-1 configuration
*
*/
public abstract void getConfig(T ctx, GetConfigRequest request, Observer observer);
/**
*
* Get COP-1 status
*
*/
public abstract void getStatus(T ctx, GetStatusRequest request, Observer observer);
/**
*
* Receive COP-1 status updates
*
*/
public abstract void subscribeStatus(T ctx, SubscribeStatusRequest request, Observer observer);
@Override
public final ServiceDescriptor getDescriptorForType() {
return Cop1Proto.getDescriptor().getServices().get(0);
}
@Override
public final Message getRequestPrototype(MethodDescriptor method) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
return InitializeRequest.getDefaultInstance();
case 1:
return ResumeRequest.getDefaultInstance();
case 2:
return DisableRequest.getDefaultInstance();
case 3:
return UpdateConfigRequest.getDefaultInstance();
case 4:
return GetConfigRequest.getDefaultInstance();
case 5:
return GetStatusRequest.getDefaultInstance();
case 6:
return SubscribeStatusRequest.getDefaultInstance();
default:
throw new IllegalStateException();
}
}
@Override
public final Message getResponsePrototype(MethodDescriptor method) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
return Empty.getDefaultInstance();
case 1:
return Empty.getDefaultInstance();
case 2:
return Empty.getDefaultInstance();
case 3:
return Cop1Config.getDefaultInstance();
case 4:
return Cop1Config.getDefaultInstance();
case 5:
return Cop1Status.getDefaultInstance();
case 6:
return Cop1Status.getDefaultInstance();
default:
throw new IllegalStateException();
}
}
@Override
public final void callMethod(MethodDescriptor method, T ctx, Message request, Observer future) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
initialize(ctx, (InitializeRequest) request, (Observer)(Object) future);
return;
case 1:
resume(ctx, (ResumeRequest) request, (Observer)(Object) future);
return;
case 2:
disable(ctx, (DisableRequest) request, (Observer)(Object) future);
return;
case 3:
updateConfig(ctx, (UpdateConfigRequest) request, (Observer)(Object) future);
return;
case 4:
getConfig(ctx, (GetConfigRequest) request, (Observer)(Object) future);
return;
case 5:
getStatus(ctx, (GetStatusRequest) request, (Observer)(Object) future);
return;
case 6:
subscribeStatus(ctx, (SubscribeStatusRequest) request, (Observer)(Object) future);
return;
default:
throw new IllegalStateException();
}
}
@Override
public final Observer callMethod(MethodDescriptor method, T ctx, Observer future) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
default:
throw new IllegalStateException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy