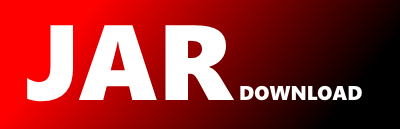
org.yamcs.protobuf.AbstractParameterArchiveApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
package org.yamcs.protobuf;
import com.google.protobuf.Descriptors.MethodDescriptor;
import com.google.protobuf.Descriptors.ServiceDescriptor;
import com.google.protobuf.Empty;
import com.google.protobuf.Message;
import org.yamcs.api.Api;
import org.yamcs.api.Observer;
import org.yamcs.protobuf.Archive.GetParameterSamplesRequest;
import org.yamcs.protobuf.Archive.ListParameterHistoryRequest;
import org.yamcs.protobuf.Archive.ListParameterHistoryResponse;
import org.yamcs.protobuf.Pvalue.Ranges;
import org.yamcs.protobuf.Pvalue.TimeSeries;
@javax.annotation.processing.Generated(value = "org.yamcs.maven.ServiceGenerator", date = "2025-02-13T11:12:59.911260781Z")
@SuppressWarnings("unchecked")
public abstract class AbstractParameterArchiveApi implements Api {
/**
*
* Rebuild range
*
* The back filler has to be enabled for this purpose. The back filling process does not
* remove data but just overwrites it. That means that if the parameter replay returns
* less parameters than originally stored in the archive, the old parameters will still
* be found in the archive.
*
* It also means that if a replay returns the parameter of a different type than
* originally stored, the old ones will still be stored. This is because the parameter
* archive treats parameter with the same name but different type as different parameters.
* Each of them is given an id and the id is stored in the archive.
*
*/
public abstract void rebuildRange(T ctx, RebuildRangeRequest request, Observer observer);
/**
*
* Get parameter samples
*
* This divides the query interval in a number of intervals and returns aggregated
* statistics (max, min, avg) about each interval.
*
* This operation is useful when making high-level overviews (such as plots) of a
* parameter's value over large time intervals without having to retrieve each
* and every individual parameter value.
*
* By default this operation fetches data from the parameter archive and/or
* parameter cache. If these services are not configured, you can still get
* correct results by specifying the option ``source=replay`` as detailed below.
*
*/
public abstract void getParameterSamples(T ctx, GetParameterSamplesRequest request, Observer observer);
/**
*
* Get parameter ranges
*
* A range is a tuple ``(start, stop, value, count)`` that represents the time
* interval for which the parameter has been steadily coming in with the same
* value. This request is useful for retrieving an overview for parameters that
* change unfrequently in a large time interval. For example an on/off status
* of a device, or some operational status. Two consecutive ranges containing
* the same value will be returned if there was a gap in the data. The gap is
* determined according to the parameter expiration time configured in the
* Mission Database.
*
*/
public abstract void getParameterRanges(T ctx, GetParameterRangesRequest request, Observer observer);
/**
*
* List parameter history
*
*/
public abstract void listParameterHistory(T ctx, ListParameterHistoryRequest request, Observer observer);
/**
*
* Get information about the archived parameters.
*
* Each combination of (parameter name, raw type, engineering type) is assigned a
* unique parameter id.
*
* The parameters are grouped such that the samples of all parameters from one group
* have the same timestamp. For example all parameters extracted from one TM packet
* have usually the same timestamp and are part of the same group.
*
* Each group is assigned a unique group id.
*
* A parameter can be part of multiple groups. For instance a parameter appearing
* in the header of a packet is part of all groups made by inherited containers
* (i.e. each packet with that header will compose another group).
*
* For each group, the parameter archive stores one common record for the timestamps
* and individual records for the raw and engineering values of each parameter. If a
* parameter appears in multiple groups, retrieving its value means combining
* (time-based merge operation). The records beloging to the groups in which the
* parameter appears.
*
* The response to this method contains the parameter id, name, engineering type,
* raw type and the groups of which this parameter is part of.
*
*/
public abstract void getArchivedParametersInfo(T ctx, GetArchivedParametersInfoRequest request, Observer observer);
/**
*
* For a given parameter id, get the list of segments available for that parameter.
* A segment contains multiple samples (maximum ~70 minutes) of the same parameter.
*
*/
public abstract void getArchivedParameterSegments(T ctx, GetArchivedParameterSegmentsRequest request, Observer observer);
/**
*
* For a given group id, get the list of parameters which are part of the group
*
*/
public abstract void getArchivedParameterGroup(T ctx, GetArchivedParameterGroupRequest request, Observer observer);
/**
*
* Removes all the parameter archive data and related metadata. All the filling operations are stopped before
* and started after the purge.
*
* The rebuild operation has to be called to rebuild the past segments of the archive.
*
* Starting with Yamcs 5.9.0 the Parameter Archive is stored into a different column family ``parameter_archive``.
* Storing data into a separate column family gives better performance and the Parameter Archive rebuild operations
* are less disturbing for the other data (TM, TC, Events...). If the archive is from a previous version of Yamcs,
* the purge/rebuild can be used to move the parameter archive from the default column family to the separate column family.
*
*/
public abstract void purge(T ctx, PurgeRequest request, Observer observer);
/**
*
* Disables the automatic backfilling (rebuilding) of the parameter archive.
* See :manual:`services/instance/parameter-archive-service/#backfiller-options`
*
* If the backfilling is already disabled, this operation has no effect.
* If there is a backfilling running, this call will not stop it.
*
* Manual rebuild operations are still accepted.
*
*
*/
public abstract void disableBackfilling(T ctx, DisableBackfillingRequest request, Observer observer);
/**
*
* Enables the automatic backfilling (rebuilding) of the parameter archive.
* If the backfilling is already enabled, this operation has no effect.
*
*
*/
public abstract void enableBackfilling(T ctx, EnableBackfillingRequest request, Observer observer);
/**
*
* Receive backfill notifications
*
*/
public abstract void subscribeBackfilling(T ctx, SubscribeBackfillingRequest request, Observer observer);
@Override
public final ServiceDescriptor getDescriptorForType() {
return ParameterArchiveServiceProto.getDescriptor().getServices().get(0);
}
@Override
public final Message getRequestPrototype(MethodDescriptor method) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
return RebuildRangeRequest.getDefaultInstance();
case 1:
return GetParameterSamplesRequest.getDefaultInstance();
case 2:
return GetParameterRangesRequest.getDefaultInstance();
case 3:
return ListParameterHistoryRequest.getDefaultInstance();
case 4:
return GetArchivedParametersInfoRequest.getDefaultInstance();
case 5:
return GetArchivedParameterSegmentsRequest.getDefaultInstance();
case 6:
return GetArchivedParameterGroupRequest.getDefaultInstance();
case 7:
return PurgeRequest.getDefaultInstance();
case 8:
return DisableBackfillingRequest.getDefaultInstance();
case 9:
return EnableBackfillingRequest.getDefaultInstance();
case 10:
return SubscribeBackfillingRequest.getDefaultInstance();
default:
throw new IllegalStateException();
}
}
@Override
public final Message getResponsePrototype(MethodDescriptor method) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
return Empty.getDefaultInstance();
case 1:
return TimeSeries.getDefaultInstance();
case 2:
return Ranges.getDefaultInstance();
case 3:
return ListParameterHistoryResponse.getDefaultInstance();
case 4:
return ArchivedParametersInfoResponse.getDefaultInstance();
case 5:
return ArchivedParameterSegmentsResponse.getDefaultInstance();
case 6:
return ArchivedParameterGroupResponse.getDefaultInstance();
case 7:
return Empty.getDefaultInstance();
case 8:
return Empty.getDefaultInstance();
case 9:
return Empty.getDefaultInstance();
case 10:
return SubscribeBackfillingData.getDefaultInstance();
default:
throw new IllegalStateException();
}
}
@Override
public final void callMethod(MethodDescriptor method, T ctx, Message request, Observer future) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
rebuildRange(ctx, (RebuildRangeRequest) request, (Observer)(Object) future);
return;
case 1:
getParameterSamples(ctx, (GetParameterSamplesRequest) request, (Observer)(Object) future);
return;
case 2:
getParameterRanges(ctx, (GetParameterRangesRequest) request, (Observer)(Object) future);
return;
case 3:
listParameterHistory(ctx, (ListParameterHistoryRequest) request, (Observer)(Object) future);
return;
case 4:
getArchivedParametersInfo(ctx, (GetArchivedParametersInfoRequest) request, (Observer)(Object) future);
return;
case 5:
getArchivedParameterSegments(ctx, (GetArchivedParameterSegmentsRequest) request, (Observer)(Object) future);
return;
case 6:
getArchivedParameterGroup(ctx, (GetArchivedParameterGroupRequest) request, (Observer)(Object) future);
return;
case 7:
purge(ctx, (PurgeRequest) request, (Observer)(Object) future);
return;
case 8:
disableBackfilling(ctx, (DisableBackfillingRequest) request, (Observer)(Object) future);
return;
case 9:
enableBackfilling(ctx, (EnableBackfillingRequest) request, (Observer)(Object) future);
return;
case 10:
subscribeBackfilling(ctx, (SubscribeBackfillingRequest) request, (Observer)(Object) future);
return;
default:
throw new IllegalStateException();
}
}
@Override
public final Observer callMethod(MethodDescriptor method, T ctx, Observer future) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
default:
throw new IllegalStateException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy