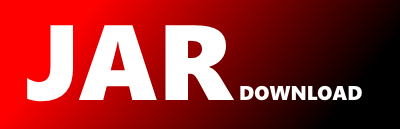
org.yamcs.protobuf.AbstractTimeCorrelationApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
package org.yamcs.protobuf;
import com.google.protobuf.Descriptors.MethodDescriptor;
import com.google.protobuf.Descriptors.ServiceDescriptor;
import com.google.protobuf.Empty;
import com.google.protobuf.Message;
import org.yamcs.api.Api;
import org.yamcs.api.Observer;
/**
*
* Methods related to the Time Correlation Service.
*
*/
@javax.annotation.processing.Generated(value = "org.yamcs.maven.ServiceGenerator", date = "2025-02-13T11:12:59.910553336Z")
@SuppressWarnings("unchecked")
public abstract class AbstractTimeCorrelationApi implements Api {
/**
*
* Get the TCO config
*
* Returns the TCO configuration comprising the accuracy, validity and the fixed delays.
*
*/
public abstract void getConfig(T ctx, GetTcoConfigRequest request, Observer observer);
/**
*
* Set the TCO config
*
* Set the TCO configuration. The configuration is not persisted on disk.
*
*/
public abstract void setConfig(T ctx, SetTcoConfigRequest request, Observer observer);
/**
*
* Get the TCO status
*
* Returns the TCO status comprising the currently used coefficients, the last computed deviation
* and the last received samples.
*
*/
public abstract void getStatus(T ctx, GetTcoStatusRequest request, Observer observer);
/**
*
* Set the TCO coefficients
*
* Manually set the coefficients to be used for time correlation. These will overwrite
* the automatic computed coefficients.
*
*/
public abstract void setCoefficients(T ctx, SetCoefficientsRequest request, Observer observer);
/**
*
* Reset the time correlation.
*
* The current used TCO coefficients are removed together with all collected samples.
*
*/
public abstract void reset(T ctx, TcoResetRequest request, Observer observer);
/**
*
* Add intervals for the time of flight calculation.
*
* Each ``[ertStart, ertStop)`` interval contains a polynomial function used to compute the time of flight for the given ``ert``.
* The intervals can overlap and are sorted in descending order of the start time.
* The first (highest start time) interval where the requested ert fits, will be used for the calculation.
*
* The formula used for calculating the time of flight for a frame/packet received
* at ``ert`` in the ``[ertStart, ertStop)`` interval is:
*
* ``delta = ert - ertStart``
*
* ``tof = polCoef[0] + polCoef[1] * delta + polCoef[2] * delta^2 + ...``
*
* The result of the polynomial is the ``tof`` expressed in seconds.
*
*
*/
public abstract void addTimeOfFlightIntervals(T ctx, AddTimeOfFlightIntervalsRequest request, Observer observer);
/**
*
* Delete intervals for the time of flight calculation.
*
* All the intervals with the start time
* falling in the requested [start, stop] interval will be removed.
*
*/
public abstract void deleteTimeOfFlightIntervals(T ctx, DeleteTimeOfFlightIntervalsRequest request, Observer observer);
@Override
public final ServiceDescriptor getDescriptorForType() {
return TcoProto.getDescriptor().getServices().get(0);
}
@Override
public final Message getRequestPrototype(MethodDescriptor method) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
return GetTcoConfigRequest.getDefaultInstance();
case 1:
return SetTcoConfigRequest.getDefaultInstance();
case 2:
return GetTcoStatusRequest.getDefaultInstance();
case 3:
return SetCoefficientsRequest.getDefaultInstance();
case 4:
return TcoResetRequest.getDefaultInstance();
case 5:
return AddTimeOfFlightIntervalsRequest.getDefaultInstance();
case 6:
return DeleteTimeOfFlightIntervalsRequest.getDefaultInstance();
default:
throw new IllegalStateException();
}
}
@Override
public final Message getResponsePrototype(MethodDescriptor method) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
return TcoConfig.getDefaultInstance();
case 1:
return Empty.getDefaultInstance();
case 2:
return TcoStatus.getDefaultInstance();
case 3:
return Empty.getDefaultInstance();
case 4:
return Empty.getDefaultInstance();
case 5:
return Empty.getDefaultInstance();
case 6:
return Empty.getDefaultInstance();
default:
throw new IllegalStateException();
}
}
@Override
public final void callMethod(MethodDescriptor method, T ctx, Message request, Observer future) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
getConfig(ctx, (GetTcoConfigRequest) request, (Observer)(Object) future);
return;
case 1:
setConfig(ctx, (SetTcoConfigRequest) request, (Observer)(Object) future);
return;
case 2:
getStatus(ctx, (GetTcoStatusRequest) request, (Observer)(Object) future);
return;
case 3:
setCoefficients(ctx, (SetCoefficientsRequest) request, (Observer)(Object) future);
return;
case 4:
reset(ctx, (TcoResetRequest) request, (Observer)(Object) future);
return;
case 5:
addTimeOfFlightIntervals(ctx, (AddTimeOfFlightIntervalsRequest) request, (Observer)(Object) future);
return;
case 6:
deleteTimeOfFlightIntervals(ctx, (DeleteTimeOfFlightIntervalsRequest) request, (Observer)(Object) future);
return;
default:
throw new IllegalStateException();
}
}
@Override
public final Observer callMethod(MethodDescriptor method, T ctx, Observer future) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
default:
throw new IllegalStateException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy