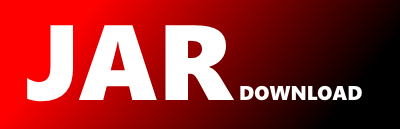
org.yamcs.protobuf.ClientConnectionInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: yamcs/protobuf/server/server_service.proto
package org.yamcs.protobuf;
/**
* Protobuf type {@code yamcs.protobuf.server.ClientConnectionInfo}
*/
public final class ClientConnectionInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.server.ClientConnectionInfo)
ClientConnectionInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ClientConnectionInfo.newBuilder() to construct.
private ClientConnectionInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ClientConnectionInfo() {
id_ = "";
remoteAddress_ = "";
username_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ClientConnectionInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ClientConnectionInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
id_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
open_ = input.readBool();
break;
}
case 24: {
bitField0_ |= 0x00000004;
active_ = input.readBool();
break;
}
case 32: {
bitField0_ |= 0x00000008;
writable_ = input.readBool();
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
remoteAddress_ = bs;
break;
}
case 48: {
bitField0_ |= 0x00000020;
readBytes_ = input.readUInt64();
break;
}
case 56: {
bitField0_ |= 0x00000040;
writtenBytes_ = input.readUInt64();
break;
}
case 64: {
bitField0_ |= 0x00000080;
readThroughput_ = input.readUInt64();
break;
}
case 72: {
bitField0_ |= 0x00000100;
writeThroughput_ = input.readUInt64();
break;
}
case 82: {
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000200) != 0)) {
subBuilder = httpRequest_.toBuilder();
}
httpRequest_ = input.readMessage(org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(httpRequest_);
httpRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000200;
break;
}
case 90: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
username_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.ClientConnectionInfo.class, org.yamcs.protobuf.ClientConnectionInfo.Builder.class);
}
public interface HttpRequestInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo)
com.google.protobuf.MessageOrBuilder {
/**
* optional string protocol = 1;
* @return Whether the protocol field is set.
*/
boolean hasProtocol();
/**
* optional string protocol = 1;
* @return The protocol.
*/
java.lang.String getProtocol();
/**
* optional string protocol = 1;
* @return The bytes for protocol.
*/
com.google.protobuf.ByteString
getProtocolBytes();
/**
* optional string method = 2;
* @return Whether the method field is set.
*/
boolean hasMethod();
/**
* optional string method = 2;
* @return The method.
*/
java.lang.String getMethod();
/**
* optional string method = 2;
* @return The bytes for method.
*/
com.google.protobuf.ByteString
getMethodBytes();
/**
* optional string uri = 3;
* @return Whether the uri field is set.
*/
boolean hasUri();
/**
* optional string uri = 3;
* @return The uri.
*/
java.lang.String getUri();
/**
* optional string uri = 3;
* @return The bytes for uri.
*/
com.google.protobuf.ByteString
getUriBytes();
/**
* optional bool keepAlive = 4;
* @return Whether the keepAlive field is set.
*/
boolean hasKeepAlive();
/**
* optional bool keepAlive = 4;
* @return The keepAlive.
*/
boolean getKeepAlive();
/**
* optional string userAgent = 5;
* @return Whether the userAgent field is set.
*/
boolean hasUserAgent();
/**
* optional string userAgent = 5;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* optional string userAgent = 5;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo}
*/
public static final class HttpRequestInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo)
HttpRequestInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use HttpRequestInfo.newBuilder() to construct.
private HttpRequestInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HttpRequestInfo() {
protocol_ = "";
method_ = "";
uri_ = "";
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HttpRequestInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HttpRequestInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
protocol_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
method_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
uri_ = bs;
break;
}
case 32: {
bitField0_ |= 0x00000008;
keepAlive_ = input.readBool();
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
userAgent_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_HttpRequestInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_HttpRequestInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.class, org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.Builder.class);
}
private int bitField0_;
public static final int PROTOCOL_FIELD_NUMBER = 1;
private volatile java.lang.Object protocol_;
/**
* optional string protocol = 1;
* @return Whether the protocol field is set.
*/
@java.lang.Override
public boolean hasProtocol() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string protocol = 1;
* @return The protocol.
*/
@java.lang.Override
public java.lang.String getProtocol() {
java.lang.Object ref = protocol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
protocol_ = s;
}
return s;
}
}
/**
* optional string protocol = 1;
* @return The bytes for protocol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProtocolBytes() {
java.lang.Object ref = protocol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int METHOD_FIELD_NUMBER = 2;
private volatile java.lang.Object method_;
/**
* optional string method = 2;
* @return Whether the method field is set.
*/
@java.lang.Override
public boolean hasMethod() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string method = 2;
* @return The method.
*/
@java.lang.Override
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
method_ = s;
}
return s;
}
}
/**
* optional string method = 2;
* @return The bytes for method.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int URI_FIELD_NUMBER = 3;
private volatile java.lang.Object uri_;
/**
* optional string uri = 3;
* @return Whether the uri field is set.
*/
@java.lang.Override
public boolean hasUri() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string uri = 3;
* @return The uri.
*/
@java.lang.Override
public java.lang.String getUri() {
java.lang.Object ref = uri_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uri_ = s;
}
return s;
}
}
/**
* optional string uri = 3;
* @return The bytes for uri.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUriBytes() {
java.lang.Object ref = uri_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KEEPALIVE_FIELD_NUMBER = 4;
private boolean keepAlive_;
/**
* optional bool keepAlive = 4;
* @return Whether the keepAlive field is set.
*/
@java.lang.Override
public boolean hasKeepAlive() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool keepAlive = 4;
* @return The keepAlive.
*/
@java.lang.Override
public boolean getKeepAlive() {
return keepAlive_;
}
public static final int USERAGENT_FIELD_NUMBER = 5;
private volatile java.lang.Object userAgent_;
/**
* optional string userAgent = 5;
* @return Whether the userAgent field is set.
*/
@java.lang.Override
public boolean hasUserAgent() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string userAgent = 5;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userAgent_ = s;
}
return s;
}
}
/**
* optional string userAgent = 5;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, protocol_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, method_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, uri_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBool(4, keepAlive_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, protocol_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, method_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, uri_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, keepAlive_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo)) {
return super.equals(obj);
}
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo other = (org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo) obj;
if (hasProtocol() != other.hasProtocol()) return false;
if (hasProtocol()) {
if (!getProtocol()
.equals(other.getProtocol())) return false;
}
if (hasMethod() != other.hasMethod()) return false;
if (hasMethod()) {
if (!getMethod()
.equals(other.getMethod())) return false;
}
if (hasUri() != other.hasUri()) return false;
if (hasUri()) {
if (!getUri()
.equals(other.getUri())) return false;
}
if (hasKeepAlive() != other.hasKeepAlive()) return false;
if (hasKeepAlive()) {
if (getKeepAlive()
!= other.getKeepAlive()) return false;
}
if (hasUserAgent() != other.hasUserAgent()) return false;
if (hasUserAgent()) {
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasProtocol()) {
hash = (37 * hash) + PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + getProtocol().hashCode();
}
if (hasMethod()) {
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + getMethod().hashCode();
}
if (hasUri()) {
hash = (37 * hash) + URI_FIELD_NUMBER;
hash = (53 * hash) + getUri().hashCode();
}
if (hasKeepAlive()) {
hash = (37 * hash) + KEEPALIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getKeepAlive());
}
if (hasUserAgent()) {
hash = (37 * hash) + USERAGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo)
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_HttpRequestInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_HttpRequestInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.class, org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.Builder.class);
}
// Construct using org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
protocol_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
method_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
uri_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
keepAlive_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
userAgent_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_HttpRequestInfo_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo getDefaultInstanceForType() {
return org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo build() {
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo buildPartial() {
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo result = new org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.protocol_ = protocol_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.method_ = method_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.uri_ = uri_;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.keepAlive_ = keepAlive_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.userAgent_ = userAgent_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo) {
return mergeFrom((org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo other) {
if (other == org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.getDefaultInstance()) return this;
if (other.hasProtocol()) {
bitField0_ |= 0x00000001;
protocol_ = other.protocol_;
onChanged();
}
if (other.hasMethod()) {
bitField0_ |= 0x00000002;
method_ = other.method_;
onChanged();
}
if (other.hasUri()) {
bitField0_ |= 0x00000004;
uri_ = other.uri_;
onChanged();
}
if (other.hasKeepAlive()) {
setKeepAlive(other.getKeepAlive());
}
if (other.hasUserAgent()) {
bitField0_ |= 0x00000010;
userAgent_ = other.userAgent_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object protocol_ = "";
/**
* optional string protocol = 1;
* @return Whether the protocol field is set.
*/
public boolean hasProtocol() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string protocol = 1;
* @return The protocol.
*/
public java.lang.String getProtocol() {
java.lang.Object ref = protocol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
protocol_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string protocol = 1;
* @return The bytes for protocol.
*/
public com.google.protobuf.ByteString
getProtocolBytes() {
java.lang.Object ref = protocol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string protocol = 1;
* @param value The protocol to set.
* @return This builder for chaining.
*/
public Builder setProtocol(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
protocol_ = value;
onChanged();
return this;
}
/**
* optional string protocol = 1;
* @return This builder for chaining.
*/
public Builder clearProtocol() {
bitField0_ = (bitField0_ & ~0x00000001);
protocol_ = getDefaultInstance().getProtocol();
onChanged();
return this;
}
/**
* optional string protocol = 1;
* @param value The bytes for protocol to set.
* @return This builder for chaining.
*/
public Builder setProtocolBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
protocol_ = value;
onChanged();
return this;
}
private java.lang.Object method_ = "";
/**
* optional string method = 2;
* @return Whether the method field is set.
*/
public boolean hasMethod() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string method = 2;
* @return The method.
*/
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
method_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string method = 2;
* @return The bytes for method.
*/
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string method = 2;
* @param value The method to set.
* @return This builder for chaining.
*/
public Builder setMethod(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
method_ = value;
onChanged();
return this;
}
/**
* optional string method = 2;
* @return This builder for chaining.
*/
public Builder clearMethod() {
bitField0_ = (bitField0_ & ~0x00000002);
method_ = getDefaultInstance().getMethod();
onChanged();
return this;
}
/**
* optional string method = 2;
* @param value The bytes for method to set.
* @return This builder for chaining.
*/
public Builder setMethodBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
method_ = value;
onChanged();
return this;
}
private java.lang.Object uri_ = "";
/**
* optional string uri = 3;
* @return Whether the uri field is set.
*/
public boolean hasUri() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string uri = 3;
* @return The uri.
*/
public java.lang.String getUri() {
java.lang.Object ref = uri_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uri_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string uri = 3;
* @return The bytes for uri.
*/
public com.google.protobuf.ByteString
getUriBytes() {
java.lang.Object ref = uri_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string uri = 3;
* @param value The uri to set.
* @return This builder for chaining.
*/
public Builder setUri(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
uri_ = value;
onChanged();
return this;
}
/**
* optional string uri = 3;
* @return This builder for chaining.
*/
public Builder clearUri() {
bitField0_ = (bitField0_ & ~0x00000004);
uri_ = getDefaultInstance().getUri();
onChanged();
return this;
}
/**
* optional string uri = 3;
* @param value The bytes for uri to set.
* @return This builder for chaining.
*/
public Builder setUriBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
uri_ = value;
onChanged();
return this;
}
private boolean keepAlive_ ;
/**
* optional bool keepAlive = 4;
* @return Whether the keepAlive field is set.
*/
@java.lang.Override
public boolean hasKeepAlive() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool keepAlive = 4;
* @return The keepAlive.
*/
@java.lang.Override
public boolean getKeepAlive() {
return keepAlive_;
}
/**
* optional bool keepAlive = 4;
* @param value The keepAlive to set.
* @return This builder for chaining.
*/
public Builder setKeepAlive(boolean value) {
bitField0_ |= 0x00000008;
keepAlive_ = value;
onChanged();
return this;
}
/**
* optional bool keepAlive = 4;
* @return This builder for chaining.
*/
public Builder clearKeepAlive() {
bitField0_ = (bitField0_ & ~0x00000008);
keepAlive_ = false;
onChanged();
return this;
}
private java.lang.Object userAgent_ = "";
/**
* optional string userAgent = 5;
* @return Whether the userAgent field is set.
*/
public boolean hasUserAgent() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string userAgent = 5;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userAgent_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string userAgent = 5;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string userAgent = 5;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
userAgent_ = value;
onChanged();
return this;
}
/**
* optional string userAgent = 5;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
bitField0_ = (bitField0_ & ~0x00000010);
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* optional string userAgent = 5;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo)
private static final org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo();
}
public static org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HttpRequestInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HttpRequestInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
* optional string id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPEN_FIELD_NUMBER = 2;
private boolean open_;
/**
* optional bool open = 2;
* @return Whether the open field is set.
*/
@java.lang.Override
public boolean hasOpen() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bool open = 2;
* @return The open.
*/
@java.lang.Override
public boolean getOpen() {
return open_;
}
public static final int ACTIVE_FIELD_NUMBER = 3;
private boolean active_;
/**
* optional bool active = 3;
* @return Whether the active field is set.
*/
@java.lang.Override
public boolean hasActive() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bool active = 3;
* @return The active.
*/
@java.lang.Override
public boolean getActive() {
return active_;
}
public static final int WRITABLE_FIELD_NUMBER = 4;
private boolean writable_;
/**
* optional bool writable = 4;
* @return Whether the writable field is set.
*/
@java.lang.Override
public boolean hasWritable() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool writable = 4;
* @return The writable.
*/
@java.lang.Override
public boolean getWritable() {
return writable_;
}
public static final int REMOTEADDRESS_FIELD_NUMBER = 5;
private volatile java.lang.Object remoteAddress_;
/**
* optional string remoteAddress = 5;
* @return Whether the remoteAddress field is set.
*/
@java.lang.Override
public boolean hasRemoteAddress() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string remoteAddress = 5;
* @return The remoteAddress.
*/
@java.lang.Override
public java.lang.String getRemoteAddress() {
java.lang.Object ref = remoteAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
remoteAddress_ = s;
}
return s;
}
}
/**
* optional string remoteAddress = 5;
* @return The bytes for remoteAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRemoteAddressBytes() {
java.lang.Object ref = remoteAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
remoteAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int READBYTES_FIELD_NUMBER = 6;
private long readBytes_;
/**
* optional uint64 readBytes = 6;
* @return Whether the readBytes field is set.
*/
@java.lang.Override
public boolean hasReadBytes() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional uint64 readBytes = 6;
* @return The readBytes.
*/
@java.lang.Override
public long getReadBytes() {
return readBytes_;
}
public static final int WRITTENBYTES_FIELD_NUMBER = 7;
private long writtenBytes_;
/**
* optional uint64 writtenBytes = 7;
* @return Whether the writtenBytes field is set.
*/
@java.lang.Override
public boolean hasWrittenBytes() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional uint64 writtenBytes = 7;
* @return The writtenBytes.
*/
@java.lang.Override
public long getWrittenBytes() {
return writtenBytes_;
}
public static final int READTHROUGHPUT_FIELD_NUMBER = 8;
private long readThroughput_;
/**
* optional uint64 readThroughput = 8;
* @return Whether the readThroughput field is set.
*/
@java.lang.Override
public boolean hasReadThroughput() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional uint64 readThroughput = 8;
* @return The readThroughput.
*/
@java.lang.Override
public long getReadThroughput() {
return readThroughput_;
}
public static final int WRITETHROUGHPUT_FIELD_NUMBER = 9;
private long writeThroughput_;
/**
* optional uint64 writeThroughput = 9;
* @return Whether the writeThroughput field is set.
*/
@java.lang.Override
public boolean hasWriteThroughput() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional uint64 writeThroughput = 9;
* @return The writeThroughput.
*/
@java.lang.Override
public long getWriteThroughput() {
return writeThroughput_;
}
public static final int HTTPREQUEST_FIELD_NUMBER = 10;
private org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo httpRequest_;
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
* @return Whether the httpRequest field is set.
*/
@java.lang.Override
public boolean hasHttpRequest() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
* @return The httpRequest.
*/
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo getHttpRequest() {
return httpRequest_ == null ? org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.getDefaultInstance() : httpRequest_;
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
*/
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfoOrBuilder getHttpRequestOrBuilder() {
return httpRequest_ == null ? org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.getDefaultInstance() : httpRequest_;
}
public static final int USERNAME_FIELD_NUMBER = 11;
private volatile java.lang.Object username_;
/**
* optional string username = 11;
* @return Whether the username field is set.
*/
@java.lang.Override
public boolean hasUsername() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional string username = 11;
* @return The username.
*/
@java.lang.Override
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
username_ = s;
}
return s;
}
}
/**
* optional string username = 11;
* @return The bytes for username.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(2, open_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(3, active_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBool(4, writable_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, remoteAddress_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeUInt64(6, readBytes_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeUInt64(7, writtenBytes_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeUInt64(8, readThroughput_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeUInt64(9, writeThroughput_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeMessage(10, getHttpRequest());
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, username_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, open_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, active_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, writable_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, remoteAddress_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, readBytes_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(7, writtenBytes_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(8, readThroughput_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(9, writeThroughput_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getHttpRequest());
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, username_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.ClientConnectionInfo)) {
return super.equals(obj);
}
org.yamcs.protobuf.ClientConnectionInfo other = (org.yamcs.protobuf.ClientConnectionInfo) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (hasOpen() != other.hasOpen()) return false;
if (hasOpen()) {
if (getOpen()
!= other.getOpen()) return false;
}
if (hasActive() != other.hasActive()) return false;
if (hasActive()) {
if (getActive()
!= other.getActive()) return false;
}
if (hasWritable() != other.hasWritable()) return false;
if (hasWritable()) {
if (getWritable()
!= other.getWritable()) return false;
}
if (hasRemoteAddress() != other.hasRemoteAddress()) return false;
if (hasRemoteAddress()) {
if (!getRemoteAddress()
.equals(other.getRemoteAddress())) return false;
}
if (hasReadBytes() != other.hasReadBytes()) return false;
if (hasReadBytes()) {
if (getReadBytes()
!= other.getReadBytes()) return false;
}
if (hasWrittenBytes() != other.hasWrittenBytes()) return false;
if (hasWrittenBytes()) {
if (getWrittenBytes()
!= other.getWrittenBytes()) return false;
}
if (hasReadThroughput() != other.hasReadThroughput()) return false;
if (hasReadThroughput()) {
if (getReadThroughput()
!= other.getReadThroughput()) return false;
}
if (hasWriteThroughput() != other.hasWriteThroughput()) return false;
if (hasWriteThroughput()) {
if (getWriteThroughput()
!= other.getWriteThroughput()) return false;
}
if (hasHttpRequest() != other.hasHttpRequest()) return false;
if (hasHttpRequest()) {
if (!getHttpRequest()
.equals(other.getHttpRequest())) return false;
}
if (hasUsername() != other.hasUsername()) return false;
if (hasUsername()) {
if (!getUsername()
.equals(other.getUsername())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasOpen()) {
hash = (37 * hash) + OPEN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getOpen());
}
if (hasActive()) {
hash = (37 * hash) + ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getActive());
}
if (hasWritable()) {
hash = (37 * hash) + WRITABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getWritable());
}
if (hasRemoteAddress()) {
hash = (37 * hash) + REMOTEADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getRemoteAddress().hashCode();
}
if (hasReadBytes()) {
hash = (37 * hash) + READBYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getReadBytes());
}
if (hasWrittenBytes()) {
hash = (37 * hash) + WRITTENBYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWrittenBytes());
}
if (hasReadThroughput()) {
hash = (37 * hash) + READTHROUGHPUT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getReadThroughput());
}
if (hasWriteThroughput()) {
hash = (37 * hash) + WRITETHROUGHPUT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWriteThroughput());
}
if (hasHttpRequest()) {
hash = (37 * hash) + HTTPREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getHttpRequest().hashCode();
}
if (hasUsername()) {
hash = (37 * hash) + USERNAME_FIELD_NUMBER;
hash = (53 * hash) + getUsername().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.ClientConnectionInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.ClientConnectionInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.server.ClientConnectionInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.server.ClientConnectionInfo)
org.yamcs.protobuf.ClientConnectionInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.ClientConnectionInfo.class, org.yamcs.protobuf.ClientConnectionInfo.Builder.class);
}
// Construct using org.yamcs.protobuf.ClientConnectionInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getHttpRequestFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
open_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
active_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
writable_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
remoteAddress_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
readBytes_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
writtenBytes_ = 0L;
bitField0_ = (bitField0_ & ~0x00000040);
readThroughput_ = 0L;
bitField0_ = (bitField0_ & ~0x00000080);
writeThroughput_ = 0L;
bitField0_ = (bitField0_ & ~0x00000100);
if (httpRequestBuilder_ == null) {
httpRequest_ = null;
} else {
httpRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
username_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.ServerServiceProto.internal_static_yamcs_protobuf_server_ClientConnectionInfo_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo getDefaultInstanceForType() {
return org.yamcs.protobuf.ClientConnectionInfo.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo build() {
org.yamcs.protobuf.ClientConnectionInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo buildPartial() {
org.yamcs.protobuf.ClientConnectionInfo result = new org.yamcs.protobuf.ClientConnectionInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.open_ = open_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.active_ = active_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.writable_ = writable_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.remoteAddress_ = remoteAddress_;
if (((from_bitField0_ & 0x00000020) != 0)) {
result.readBytes_ = readBytes_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.writtenBytes_ = writtenBytes_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.readThroughput_ = readThroughput_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.writeThroughput_ = writeThroughput_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
if (httpRequestBuilder_ == null) {
result.httpRequest_ = httpRequest_;
} else {
result.httpRequest_ = httpRequestBuilder_.build();
}
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
to_bitField0_ |= 0x00000400;
}
result.username_ = username_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.ClientConnectionInfo) {
return mergeFrom((org.yamcs.protobuf.ClientConnectionInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.ClientConnectionInfo other) {
if (other == org.yamcs.protobuf.ClientConnectionInfo.getDefaultInstance()) return this;
if (other.hasId()) {
bitField0_ |= 0x00000001;
id_ = other.id_;
onChanged();
}
if (other.hasOpen()) {
setOpen(other.getOpen());
}
if (other.hasActive()) {
setActive(other.getActive());
}
if (other.hasWritable()) {
setWritable(other.getWritable());
}
if (other.hasRemoteAddress()) {
bitField0_ |= 0x00000010;
remoteAddress_ = other.remoteAddress_;
onChanged();
}
if (other.hasReadBytes()) {
setReadBytes(other.getReadBytes());
}
if (other.hasWrittenBytes()) {
setWrittenBytes(other.getWrittenBytes());
}
if (other.hasReadThroughput()) {
setReadThroughput(other.getReadThroughput());
}
if (other.hasWriteThroughput()) {
setWriteThroughput(other.getWriteThroughput());
}
if (other.hasHttpRequest()) {
mergeHttpRequest(other.getHttpRequest());
}
if (other.hasUsername()) {
bitField0_ |= 0x00000400;
username_ = other.username_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.ClientConnectionInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.ClientConnectionInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
* optional string id = 1;
* @return Whether the id field is set.
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
private boolean open_ ;
/**
* optional bool open = 2;
* @return Whether the open field is set.
*/
@java.lang.Override
public boolean hasOpen() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bool open = 2;
* @return The open.
*/
@java.lang.Override
public boolean getOpen() {
return open_;
}
/**
* optional bool open = 2;
* @param value The open to set.
* @return This builder for chaining.
*/
public Builder setOpen(boolean value) {
bitField0_ |= 0x00000002;
open_ = value;
onChanged();
return this;
}
/**
* optional bool open = 2;
* @return This builder for chaining.
*/
public Builder clearOpen() {
bitField0_ = (bitField0_ & ~0x00000002);
open_ = false;
onChanged();
return this;
}
private boolean active_ ;
/**
* optional bool active = 3;
* @return Whether the active field is set.
*/
@java.lang.Override
public boolean hasActive() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bool active = 3;
* @return The active.
*/
@java.lang.Override
public boolean getActive() {
return active_;
}
/**
* optional bool active = 3;
* @param value The active to set.
* @return This builder for chaining.
*/
public Builder setActive(boolean value) {
bitField0_ |= 0x00000004;
active_ = value;
onChanged();
return this;
}
/**
* optional bool active = 3;
* @return This builder for chaining.
*/
public Builder clearActive() {
bitField0_ = (bitField0_ & ~0x00000004);
active_ = false;
onChanged();
return this;
}
private boolean writable_ ;
/**
* optional bool writable = 4;
* @return Whether the writable field is set.
*/
@java.lang.Override
public boolean hasWritable() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool writable = 4;
* @return The writable.
*/
@java.lang.Override
public boolean getWritable() {
return writable_;
}
/**
* optional bool writable = 4;
* @param value The writable to set.
* @return This builder for chaining.
*/
public Builder setWritable(boolean value) {
bitField0_ |= 0x00000008;
writable_ = value;
onChanged();
return this;
}
/**
* optional bool writable = 4;
* @return This builder for chaining.
*/
public Builder clearWritable() {
bitField0_ = (bitField0_ & ~0x00000008);
writable_ = false;
onChanged();
return this;
}
private java.lang.Object remoteAddress_ = "";
/**
* optional string remoteAddress = 5;
* @return Whether the remoteAddress field is set.
*/
public boolean hasRemoteAddress() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string remoteAddress = 5;
* @return The remoteAddress.
*/
public java.lang.String getRemoteAddress() {
java.lang.Object ref = remoteAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
remoteAddress_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string remoteAddress = 5;
* @return The bytes for remoteAddress.
*/
public com.google.protobuf.ByteString
getRemoteAddressBytes() {
java.lang.Object ref = remoteAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
remoteAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string remoteAddress = 5;
* @param value The remoteAddress to set.
* @return This builder for chaining.
*/
public Builder setRemoteAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
remoteAddress_ = value;
onChanged();
return this;
}
/**
* optional string remoteAddress = 5;
* @return This builder for chaining.
*/
public Builder clearRemoteAddress() {
bitField0_ = (bitField0_ & ~0x00000010);
remoteAddress_ = getDefaultInstance().getRemoteAddress();
onChanged();
return this;
}
/**
* optional string remoteAddress = 5;
* @param value The bytes for remoteAddress to set.
* @return This builder for chaining.
*/
public Builder setRemoteAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
remoteAddress_ = value;
onChanged();
return this;
}
private long readBytes_ ;
/**
* optional uint64 readBytes = 6;
* @return Whether the readBytes field is set.
*/
@java.lang.Override
public boolean hasReadBytes() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional uint64 readBytes = 6;
* @return The readBytes.
*/
@java.lang.Override
public long getReadBytes() {
return readBytes_;
}
/**
* optional uint64 readBytes = 6;
* @param value The readBytes to set.
* @return This builder for chaining.
*/
public Builder setReadBytes(long value) {
bitField0_ |= 0x00000020;
readBytes_ = value;
onChanged();
return this;
}
/**
* optional uint64 readBytes = 6;
* @return This builder for chaining.
*/
public Builder clearReadBytes() {
bitField0_ = (bitField0_ & ~0x00000020);
readBytes_ = 0L;
onChanged();
return this;
}
private long writtenBytes_ ;
/**
* optional uint64 writtenBytes = 7;
* @return Whether the writtenBytes field is set.
*/
@java.lang.Override
public boolean hasWrittenBytes() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional uint64 writtenBytes = 7;
* @return The writtenBytes.
*/
@java.lang.Override
public long getWrittenBytes() {
return writtenBytes_;
}
/**
* optional uint64 writtenBytes = 7;
* @param value The writtenBytes to set.
* @return This builder for chaining.
*/
public Builder setWrittenBytes(long value) {
bitField0_ |= 0x00000040;
writtenBytes_ = value;
onChanged();
return this;
}
/**
* optional uint64 writtenBytes = 7;
* @return This builder for chaining.
*/
public Builder clearWrittenBytes() {
bitField0_ = (bitField0_ & ~0x00000040);
writtenBytes_ = 0L;
onChanged();
return this;
}
private long readThroughput_ ;
/**
* optional uint64 readThroughput = 8;
* @return Whether the readThroughput field is set.
*/
@java.lang.Override
public boolean hasReadThroughput() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional uint64 readThroughput = 8;
* @return The readThroughput.
*/
@java.lang.Override
public long getReadThroughput() {
return readThroughput_;
}
/**
* optional uint64 readThroughput = 8;
* @param value The readThroughput to set.
* @return This builder for chaining.
*/
public Builder setReadThroughput(long value) {
bitField0_ |= 0x00000080;
readThroughput_ = value;
onChanged();
return this;
}
/**
* optional uint64 readThroughput = 8;
* @return This builder for chaining.
*/
public Builder clearReadThroughput() {
bitField0_ = (bitField0_ & ~0x00000080);
readThroughput_ = 0L;
onChanged();
return this;
}
private long writeThroughput_ ;
/**
* optional uint64 writeThroughput = 9;
* @return Whether the writeThroughput field is set.
*/
@java.lang.Override
public boolean hasWriteThroughput() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional uint64 writeThroughput = 9;
* @return The writeThroughput.
*/
@java.lang.Override
public long getWriteThroughput() {
return writeThroughput_;
}
/**
* optional uint64 writeThroughput = 9;
* @param value The writeThroughput to set.
* @return This builder for chaining.
*/
public Builder setWriteThroughput(long value) {
bitField0_ |= 0x00000100;
writeThroughput_ = value;
onChanged();
return this;
}
/**
* optional uint64 writeThroughput = 9;
* @return This builder for chaining.
*/
public Builder clearWriteThroughput() {
bitField0_ = (bitField0_ & ~0x00000100);
writeThroughput_ = 0L;
onChanged();
return this;
}
private org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo httpRequest_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo, org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.Builder, org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfoOrBuilder> httpRequestBuilder_;
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
* @return Whether the httpRequest field is set.
*/
public boolean hasHttpRequest() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
* @return The httpRequest.
*/
public org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo getHttpRequest() {
if (httpRequestBuilder_ == null) {
return httpRequest_ == null ? org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.getDefaultInstance() : httpRequest_;
} else {
return httpRequestBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
*/
public Builder setHttpRequest(org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo value) {
if (httpRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
httpRequest_ = value;
onChanged();
} else {
httpRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
*/
public Builder setHttpRequest(
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.Builder builderForValue) {
if (httpRequestBuilder_ == null) {
httpRequest_ = builderForValue.build();
onChanged();
} else {
httpRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
*/
public Builder mergeHttpRequest(org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo value) {
if (httpRequestBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0) &&
httpRequest_ != null &&
httpRequest_ != org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.getDefaultInstance()) {
httpRequest_ =
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.newBuilder(httpRequest_).mergeFrom(value).buildPartial();
} else {
httpRequest_ = value;
}
onChanged();
} else {
httpRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
*/
public Builder clearHttpRequest() {
if (httpRequestBuilder_ == null) {
httpRequest_ = null;
onChanged();
} else {
httpRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
*/
public org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.Builder getHttpRequestBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getHttpRequestFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
*/
public org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfoOrBuilder getHttpRequestOrBuilder() {
if (httpRequestBuilder_ != null) {
return httpRequestBuilder_.getMessageOrBuilder();
} else {
return httpRequest_ == null ?
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.getDefaultInstance() : httpRequest_;
}
}
/**
* optional .yamcs.protobuf.server.ClientConnectionInfo.HttpRequestInfo httpRequest = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo, org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.Builder, org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfoOrBuilder>
getHttpRequestFieldBuilder() {
if (httpRequestBuilder_ == null) {
httpRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo, org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfo.Builder, org.yamcs.protobuf.ClientConnectionInfo.HttpRequestInfoOrBuilder>(
getHttpRequest(),
getParentForChildren(),
isClean());
httpRequest_ = null;
}
return httpRequestBuilder_;
}
private java.lang.Object username_ = "";
/**
* optional string username = 11;
* @return Whether the username field is set.
*/
public boolean hasUsername() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional string username = 11;
* @return The username.
*/
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
username_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string username = 11;
* @return The bytes for username.
*/
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string username = 11;
* @param value The username to set.
* @return This builder for chaining.
*/
public Builder setUsername(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
username_ = value;
onChanged();
return this;
}
/**
* optional string username = 11;
* @return This builder for chaining.
*/
public Builder clearUsername() {
bitField0_ = (bitField0_ & ~0x00000400);
username_ = getDefaultInstance().getUsername();
onChanged();
return this;
}
/**
* optional string username = 11;
* @param value The bytes for username to set.
* @return This builder for chaining.
*/
public Builder setUsernameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
username_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.server.ClientConnectionInfo)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.server.ClientConnectionInfo)
private static final org.yamcs.protobuf.ClientConnectionInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.ClientConnectionInfo();
}
public static org.yamcs.protobuf.ClientConnectionInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ClientConnectionInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ClientConnectionInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.ClientConnectionInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy