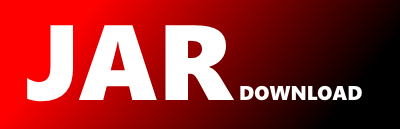
org.yamcs.protobuf.Commanding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: yamcs/protobuf/commanding/commanding.proto
package org.yamcs.protobuf;
public final class Commanding {
private Commanding() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code yamcs.protobuf.commanding.QueueState}
*/
public enum QueueState
implements com.google.protobuf.ProtocolMessageEnum {
/**
* BLOCKED = 1;
*/
BLOCKED(1),
/**
* DISABLED = 2;
*/
DISABLED(2),
/**
* ENABLED = 3;
*/
ENABLED(3),
;
/**
* BLOCKED = 1;
*/
public static final int BLOCKED_VALUE = 1;
/**
* DISABLED = 2;
*/
public static final int DISABLED_VALUE = 2;
/**
* ENABLED = 3;
*/
public static final int ENABLED_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static QueueState valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static QueueState forNumber(int value) {
switch (value) {
case 1: return BLOCKED;
case 2: return DISABLED;
case 3: return ENABLED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
QueueState> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public QueueState findValueByNumber(int number) {
return QueueState.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.getDescriptor().getEnumTypes().get(0);
}
private static final QueueState[] VALUES = values();
public static QueueState valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private QueueState(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:yamcs.protobuf.commanding.QueueState)
}
public interface CommandIdOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.CommandId)
com.google.protobuf.MessageOrBuilder {
/**
* required int64 generationTime = 1;
* @return Whether the generationTime field is set.
*/
boolean hasGenerationTime();
/**
* required int64 generationTime = 1;
* @return The generationTime.
*/
long getGenerationTime();
/**
* required string origin = 2;
* @return Whether the origin field is set.
*/
boolean hasOrigin();
/**
* required string origin = 2;
* @return The origin.
*/
java.lang.String getOrigin();
/**
* required string origin = 2;
* @return The bytes for origin.
*/
com.google.protobuf.ByteString
getOriginBytes();
/**
*
*unique in relation to generationTime and origin
*
*
* required int32 sequenceNumber = 3;
* @return Whether the sequenceNumber field is set.
*/
boolean hasSequenceNumber();
/**
*
*unique in relation to generationTime and origin
*
*
* required int32 sequenceNumber = 3;
* @return The sequenceNumber.
*/
int getSequenceNumber();
/**
* optional string commandName = 4;
* @return Whether the commandName field is set.
*/
boolean hasCommandName();
/**
* optional string commandName = 4;
* @return The commandName.
*/
java.lang.String getCommandName();
/**
* optional string commandName = 4;
* @return The bytes for commandName.
*/
com.google.protobuf.ByteString
getCommandNameBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandId}
*/
public static final class CommandId extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.CommandId)
CommandIdOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandId.newBuilder() to construct.
private CommandId(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandId() {
origin_ = "";
commandName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandId();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandId(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
generationTime_ = input.readInt64();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
origin_ = bs;
break;
}
case 24: {
bitField0_ |= 0x00000004;
sequenceNumber_ = input.readInt32();
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
commandName_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandId_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandId_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandId.class, org.yamcs.protobuf.Commanding.CommandId.Builder.class);
}
private int bitField0_;
public static final int GENERATIONTIME_FIELD_NUMBER = 1;
private long generationTime_;
/**
* required int64 generationTime = 1;
* @return Whether the generationTime field is set.
*/
@java.lang.Override
public boolean hasGenerationTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required int64 generationTime = 1;
* @return The generationTime.
*/
@java.lang.Override
public long getGenerationTime() {
return generationTime_;
}
public static final int ORIGIN_FIELD_NUMBER = 2;
private volatile java.lang.Object origin_;
/**
* required string origin = 2;
* @return Whether the origin field is set.
*/
@java.lang.Override
public boolean hasOrigin() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string origin = 2;
* @return The origin.
*/
@java.lang.Override
public java.lang.String getOrigin() {
java.lang.Object ref = origin_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
origin_ = s;
}
return s;
}
}
/**
* required string origin = 2;
* @return The bytes for origin.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOriginBytes() {
java.lang.Object ref = origin_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
origin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEQUENCENUMBER_FIELD_NUMBER = 3;
private int sequenceNumber_;
/**
*
*unique in relation to generationTime and origin
*
*
* required int32 sequenceNumber = 3;
* @return Whether the sequenceNumber field is set.
*/
@java.lang.Override
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*unique in relation to generationTime and origin
*
*
* required int32 sequenceNumber = 3;
* @return The sequenceNumber.
*/
@java.lang.Override
public int getSequenceNumber() {
return sequenceNumber_;
}
public static final int COMMANDNAME_FIELD_NUMBER = 4;
private volatile java.lang.Object commandName_;
/**
* optional string commandName = 4;
* @return Whether the commandName field is set.
*/
@java.lang.Override
public boolean hasCommandName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string commandName = 4;
* @return The commandName.
*/
@java.lang.Override
public java.lang.String getCommandName() {
java.lang.Object ref = commandName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
commandName_ = s;
}
return s;
}
}
/**
* optional string commandName = 4;
* @return The bytes for commandName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCommandNameBytes() {
java.lang.Object ref = commandName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commandName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasGenerationTime()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasOrigin()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasSequenceNumber()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, generationTime_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, origin_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(3, sequenceNumber_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, commandName_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, generationTime_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, origin_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, sequenceNumber_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, commandName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.CommandId)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.CommandId other = (org.yamcs.protobuf.Commanding.CommandId) obj;
if (hasGenerationTime() != other.hasGenerationTime()) return false;
if (hasGenerationTime()) {
if (getGenerationTime()
!= other.getGenerationTime()) return false;
}
if (hasOrigin() != other.hasOrigin()) return false;
if (hasOrigin()) {
if (!getOrigin()
.equals(other.getOrigin())) return false;
}
if (hasSequenceNumber() != other.hasSequenceNumber()) return false;
if (hasSequenceNumber()) {
if (getSequenceNumber()
!= other.getSequenceNumber()) return false;
}
if (hasCommandName() != other.hasCommandName()) return false;
if (hasCommandName()) {
if (!getCommandName()
.equals(other.getCommandName())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasGenerationTime()) {
hash = (37 * hash) + GENERATIONTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getGenerationTime());
}
if (hasOrigin()) {
hash = (37 * hash) + ORIGIN_FIELD_NUMBER;
hash = (53 * hash) + getOrigin().hashCode();
}
if (hasSequenceNumber()) {
hash = (37 * hash) + SEQUENCENUMBER_FIELD_NUMBER;
hash = (53 * hash) + getSequenceNumber();
}
if (hasCommandName()) {
hash = (37 * hash) + COMMANDNAME_FIELD_NUMBER;
hash = (53 * hash) + getCommandName().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandId parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandId parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandId parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.CommandId prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandId}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.CommandId)
org.yamcs.protobuf.Commanding.CommandIdOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandId_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandId_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandId.class, org.yamcs.protobuf.Commanding.CommandId.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.CommandId.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
generationTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
origin_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
sequenceNumber_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
commandName_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandId_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandId getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.CommandId.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandId build() {
org.yamcs.protobuf.Commanding.CommandId result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandId buildPartial() {
org.yamcs.protobuf.Commanding.CommandId result = new org.yamcs.protobuf.Commanding.CommandId(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.generationTime_ = generationTime_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.origin_ = origin_;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.sequenceNumber_ = sequenceNumber_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.commandName_ = commandName_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.CommandId) {
return mergeFrom((org.yamcs.protobuf.Commanding.CommandId)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.CommandId other) {
if (other == org.yamcs.protobuf.Commanding.CommandId.getDefaultInstance()) return this;
if (other.hasGenerationTime()) {
setGenerationTime(other.getGenerationTime());
}
if (other.hasOrigin()) {
bitField0_ |= 0x00000002;
origin_ = other.origin_;
onChanged();
}
if (other.hasSequenceNumber()) {
setSequenceNumber(other.getSequenceNumber());
}
if (other.hasCommandName()) {
bitField0_ |= 0x00000008;
commandName_ = other.commandName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasGenerationTime()) {
return false;
}
if (!hasOrigin()) {
return false;
}
if (!hasSequenceNumber()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.CommandId parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.CommandId) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long generationTime_ ;
/**
* required int64 generationTime = 1;
* @return Whether the generationTime field is set.
*/
@java.lang.Override
public boolean hasGenerationTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required int64 generationTime = 1;
* @return The generationTime.
*/
@java.lang.Override
public long getGenerationTime() {
return generationTime_;
}
/**
* required int64 generationTime = 1;
* @param value The generationTime to set.
* @return This builder for chaining.
*/
public Builder setGenerationTime(long value) {
bitField0_ |= 0x00000001;
generationTime_ = value;
onChanged();
return this;
}
/**
* required int64 generationTime = 1;
* @return This builder for chaining.
*/
public Builder clearGenerationTime() {
bitField0_ = (bitField0_ & ~0x00000001);
generationTime_ = 0L;
onChanged();
return this;
}
private java.lang.Object origin_ = "";
/**
* required string origin = 2;
* @return Whether the origin field is set.
*/
public boolean hasOrigin() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string origin = 2;
* @return The origin.
*/
public java.lang.String getOrigin() {
java.lang.Object ref = origin_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
origin_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string origin = 2;
* @return The bytes for origin.
*/
public com.google.protobuf.ByteString
getOriginBytes() {
java.lang.Object ref = origin_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
origin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string origin = 2;
* @param value The origin to set.
* @return This builder for chaining.
*/
public Builder setOrigin(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
origin_ = value;
onChanged();
return this;
}
/**
* required string origin = 2;
* @return This builder for chaining.
*/
public Builder clearOrigin() {
bitField0_ = (bitField0_ & ~0x00000002);
origin_ = getDefaultInstance().getOrigin();
onChanged();
return this;
}
/**
* required string origin = 2;
* @param value The bytes for origin to set.
* @return This builder for chaining.
*/
public Builder setOriginBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
origin_ = value;
onChanged();
return this;
}
private int sequenceNumber_ ;
/**
*
*unique in relation to generationTime and origin
*
*
* required int32 sequenceNumber = 3;
* @return Whether the sequenceNumber field is set.
*/
@java.lang.Override
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*unique in relation to generationTime and origin
*
*
* required int32 sequenceNumber = 3;
* @return The sequenceNumber.
*/
@java.lang.Override
public int getSequenceNumber() {
return sequenceNumber_;
}
/**
*
*unique in relation to generationTime and origin
*
*
* required int32 sequenceNumber = 3;
* @param value The sequenceNumber to set.
* @return This builder for chaining.
*/
public Builder setSequenceNumber(int value) {
bitField0_ |= 0x00000004;
sequenceNumber_ = value;
onChanged();
return this;
}
/**
*
*unique in relation to generationTime and origin
*
*
* required int32 sequenceNumber = 3;
* @return This builder for chaining.
*/
public Builder clearSequenceNumber() {
bitField0_ = (bitField0_ & ~0x00000004);
sequenceNumber_ = 0;
onChanged();
return this;
}
private java.lang.Object commandName_ = "";
/**
* optional string commandName = 4;
* @return Whether the commandName field is set.
*/
public boolean hasCommandName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string commandName = 4;
* @return The commandName.
*/
public java.lang.String getCommandName() {
java.lang.Object ref = commandName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
commandName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string commandName = 4;
* @return The bytes for commandName.
*/
public com.google.protobuf.ByteString
getCommandNameBytes() {
java.lang.Object ref = commandName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commandName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string commandName = 4;
* @param value The commandName to set.
* @return This builder for chaining.
*/
public Builder setCommandName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
commandName_ = value;
onChanged();
return this;
}
/**
* optional string commandName = 4;
* @return This builder for chaining.
*/
public Builder clearCommandName() {
bitField0_ = (bitField0_ & ~0x00000008);
commandName_ = getDefaultInstance().getCommandName();
onChanged();
return this;
}
/**
* optional string commandName = 4;
* @param value The bytes for commandName to set.
* @return This builder for chaining.
*/
public Builder setCommandNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
commandName_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.CommandId)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.CommandId)
private static final org.yamcs.protobuf.Commanding.CommandId DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.CommandId();
}
public static org.yamcs.protobuf.Commanding.CommandId getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandId parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandId(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandId getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandQueueInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.CommandQueueInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return Whether the processorName field is set.
*/
boolean hasProcessorName();
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return The processorName.
*/
java.lang.String getProcessorName();
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return The bytes for processorName.
*/
com.google.protobuf.ByteString
getProcessorNameBytes();
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return The name.
*/
java.lang.String getName();
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Current queue state
*
*
* optional .yamcs.protobuf.commanding.QueueState state = 4;
* @return Whether the state field is set.
*/
boolean hasState();
/**
*
* Current queue state
*
*
* optional .yamcs.protobuf.commanding.QueueState state = 4;
* @return The state.
*/
org.yamcs.protobuf.Commanding.QueueState getState();
/**
*
* Submitted commands are matches to the first queue that
* whose filter criteria (if any) match the command's
* features. Queues are considered in the order specified by
* this field, going from lowest to highest.
*
*
* optional int32 order = 9;
* @return Whether the order field is set.
*/
boolean hasOrder();
/**
*
* Submitted commands are matches to the first queue that
* whose filter criteria (if any) match the command's
* features. Queues are considered in the order specified by
* this field, going from lowest to highest.
*
*
* optional int32 order = 9;
* @return The order.
*/
int getOrder();
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @return A list containing the users.
*/
java.util.List
getUsersList();
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @return The count of users.
*/
int getUsersCount();
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param index The index of the element to return.
* @return The users at the given index.
*/
java.lang.String getUsers(int index);
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param index The index of the value to return.
* @return The bytes of the users at the given index.
*/
com.google.protobuf.ByteString
getUsersBytes(int index);
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @return A list containing the groups.
*/
java.util.List
getGroupsList();
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @return The count of groups.
*/
int getGroupsCount();
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param index The index of the element to return.
* @return The groups at the given index.
*/
java.lang.String getGroups(int index);
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param index The index of the value to return.
* @return The bytes of the groups at the given index.
*/
com.google.protobuf.ByteString
getGroupsBytes(int index);
/**
*
* This queue only considers commands that are at least
* as significant as this level.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType minLevel = 12;
* @return Whether the minLevel field is set.
*/
boolean hasMinLevel();
/**
*
* This queue only considers commands that are at least
* as significant as this level.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType minLevel = 12;
* @return The minLevel.
*/
org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType getMinLevel();
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @return A list containing the tcPatterns.
*/
java.util.List
getTcPatternsList();
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @return The count of tcPatterns.
*/
int getTcPatternsCount();
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param index The index of the element to return.
* @return The tcPatterns at the given index.
*/
java.lang.String getTcPatterns(int index);
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param index The index of the value to return.
* @return The bytes of the tcPatterns at the given index.
*/
com.google.protobuf.ByteString
getTcPatternsBytes(int index);
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
java.util.List
getEntriesList();
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
org.yamcs.protobuf.Commanding.CommandQueueEntry getEntries(int index);
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
int getEntriesCount();
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
java.util.List extends org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder>
getEntriesOrBuilderList();
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder getEntriesOrBuilder(
int index);
/**
*
* Number of commands that successfully passed through this queue.
*
*
* optional int32 acceptedCommandsCount = 15;
* @return Whether the acceptedCommandsCount field is set.
*/
boolean hasAcceptedCommandsCount();
/**
*
* Number of commands that successfully passed through this queue.
*
*
* optional int32 acceptedCommandsCount = 15;
* @return The acceptedCommandsCount.
*/
int getAcceptedCommandsCount();
/**
*
* Number of commands that were rejected by this queue.
*
*
* optional int32 rejectedCommandsCount = 16;
* @return Whether the rejectedCommandsCount field is set.
*/
boolean hasRejectedCommandsCount();
/**
*
* Number of commands that were rejected by this queue.
*
*
* optional int32 rejectedCommandsCount = 16;
* @return The rejectedCommandsCount.
*/
int getRejectedCommandsCount();
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandQueueInfo}
*/
public static final class CommandQueueInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.CommandQueueInfo)
CommandQueueInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandQueueInfo.newBuilder() to construct.
private CommandQueueInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandQueueInfo() {
instance_ = "";
processorName_ = "";
name_ = "";
state_ = 1;
users_ = com.google.protobuf.LazyStringArrayList.EMPTY;
groups_ = com.google.protobuf.LazyStringArrayList.EMPTY;
minLevel_ = 1;
tcPatterns_ = com.google.protobuf.LazyStringArrayList.EMPTY;
entries_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandQueueInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandQueueInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
processorName_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
name_ = bs;
break;
}
case 32: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Commanding.QueueState value = org.yamcs.protobuf.Commanding.QueueState.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(4, rawValue);
} else {
bitField0_ |= 0x00000008;
state_ = rawValue;
}
break;
}
case 72: {
bitField0_ |= 0x00000010;
order_ = input.readInt32();
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000020) != 0)) {
users_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000020;
}
users_.add(bs);
break;
}
case 90: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
groups_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000040;
}
groups_.add(bs);
break;
}
case 96: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType value = org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(12, rawValue);
} else {
bitField0_ |= 0x00000020;
minLevel_ = rawValue;
}
break;
}
case 106: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000100) != 0)) {
tcPatterns_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000100;
}
tcPatterns_.add(bs);
break;
}
case 114: {
if (!((mutable_bitField0_ & 0x00000200) != 0)) {
entries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000200;
}
entries_.add(
input.readMessage(org.yamcs.protobuf.Commanding.CommandQueueEntry.PARSER, extensionRegistry));
break;
}
case 120: {
bitField0_ |= 0x00000040;
acceptedCommandsCount_ = input.readInt32();
break;
}
case 128: {
bitField0_ |= 0x00000080;
rejectedCommandsCount_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000020) != 0)) {
users_ = users_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000040) != 0)) {
groups_ = groups_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000100) != 0)) {
tcPatterns_ = tcPatterns_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000200) != 0)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandQueueInfo.class, org.yamcs.protobuf.Commanding.CommandQueueInfo.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROCESSORNAME_FIELD_NUMBER = 2;
private volatile java.lang.Object processorName_;
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return Whether the processorName field is set.
*/
@java.lang.Override
public boolean hasProcessorName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return The processorName.
*/
@java.lang.Override
public java.lang.String getProcessorName() {
java.lang.Object ref = processorName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
processorName_ = s;
}
return s;
}
}
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return The bytes for processorName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProcessorNameBytes() {
java.lang.Object ref = processorName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
processorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object name_;
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATE_FIELD_NUMBER = 4;
private int state_;
/**
*
* Current queue state
*
*
* optional .yamcs.protobuf.commanding.QueueState state = 4;
* @return Whether the state field is set.
*/
@java.lang.Override public boolean hasState() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Current queue state
*
*
* optional .yamcs.protobuf.commanding.QueueState state = 4;
* @return The state.
*/
@java.lang.Override public org.yamcs.protobuf.Commanding.QueueState getState() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Commanding.QueueState result = org.yamcs.protobuf.Commanding.QueueState.valueOf(state_);
return result == null ? org.yamcs.protobuf.Commanding.QueueState.BLOCKED : result;
}
public static final int ORDER_FIELD_NUMBER = 9;
private int order_;
/**
*
* Submitted commands are matches to the first queue that
* whose filter criteria (if any) match the command's
* features. Queues are considered in the order specified by
* this field, going from lowest to highest.
*
*
* optional int32 order = 9;
* @return Whether the order field is set.
*/
@java.lang.Override
public boolean hasOrder() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Submitted commands are matches to the first queue that
* whose filter criteria (if any) match the command's
* features. Queues are considered in the order specified by
* this field, going from lowest to highest.
*
*
* optional int32 order = 9;
* @return The order.
*/
@java.lang.Override
public int getOrder() {
return order_;
}
public static final int USERS_FIELD_NUMBER = 10;
private com.google.protobuf.LazyStringList users_;
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @return A list containing the users.
*/
public com.google.protobuf.ProtocolStringList
getUsersList() {
return users_;
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @return The count of users.
*/
public int getUsersCount() {
return users_.size();
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param index The index of the element to return.
* @return The users at the given index.
*/
public java.lang.String getUsers(int index) {
return users_.get(index);
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param index The index of the value to return.
* @return The bytes of the users at the given index.
*/
public com.google.protobuf.ByteString
getUsersBytes(int index) {
return users_.getByteString(index);
}
public static final int GROUPS_FIELD_NUMBER = 11;
private com.google.protobuf.LazyStringList groups_;
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @return A list containing the groups.
*/
public com.google.protobuf.ProtocolStringList
getGroupsList() {
return groups_;
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @return The count of groups.
*/
public int getGroupsCount() {
return groups_.size();
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param index The index of the element to return.
* @return The groups at the given index.
*/
public java.lang.String getGroups(int index) {
return groups_.get(index);
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param index The index of the value to return.
* @return The bytes of the groups at the given index.
*/
public com.google.protobuf.ByteString
getGroupsBytes(int index) {
return groups_.getByteString(index);
}
public static final int MINLEVEL_FIELD_NUMBER = 12;
private int minLevel_;
/**
*
* This queue only considers commands that are at least
* as significant as this level.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType minLevel = 12;
* @return Whether the minLevel field is set.
*/
@java.lang.Override public boolean hasMinLevel() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* This queue only considers commands that are at least
* as significant as this level.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType minLevel = 12;
* @return The minLevel.
*/
@java.lang.Override public org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType getMinLevel() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType result = org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType.valueOf(minLevel_);
return result == null ? org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType.NONE : result;
}
public static final int TCPATTERNS_FIELD_NUMBER = 13;
private com.google.protobuf.LazyStringList tcPatterns_;
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @return A list containing the tcPatterns.
*/
public com.google.protobuf.ProtocolStringList
getTcPatternsList() {
return tcPatterns_;
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @return The count of tcPatterns.
*/
public int getTcPatternsCount() {
return tcPatterns_.size();
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param index The index of the element to return.
* @return The tcPatterns at the given index.
*/
public java.lang.String getTcPatterns(int index) {
return tcPatterns_.get(index);
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param index The index of the value to return.
* @return The bytes of the tcPatterns at the given index.
*/
public com.google.protobuf.ByteString
getTcPatternsBytes(int index) {
return tcPatterns_.getByteString(index);
}
public static final int ENTRIES_FIELD_NUMBER = 14;
private java.util.List entries_;
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
@java.lang.Override
public java.util.List getEntriesList() {
return entries_;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
@java.lang.Override
public int getEntriesCount() {
return entries_.size();
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntry getEntries(int index) {
return entries_.get(index);
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
public static final int ACCEPTEDCOMMANDSCOUNT_FIELD_NUMBER = 15;
private int acceptedCommandsCount_;
/**
*
* Number of commands that successfully passed through this queue.
*
*
* optional int32 acceptedCommandsCount = 15;
* @return Whether the acceptedCommandsCount field is set.
*/
@java.lang.Override
public boolean hasAcceptedCommandsCount() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Number of commands that successfully passed through this queue.
*
*
* optional int32 acceptedCommandsCount = 15;
* @return The acceptedCommandsCount.
*/
@java.lang.Override
public int getAcceptedCommandsCount() {
return acceptedCommandsCount_;
}
public static final int REJECTEDCOMMANDSCOUNT_FIELD_NUMBER = 16;
private int rejectedCommandsCount_;
/**
*
* Number of commands that were rejected by this queue.
*
*
* optional int32 rejectedCommandsCount = 16;
* @return Whether the rejectedCommandsCount field is set.
*/
@java.lang.Override
public boolean hasRejectedCommandsCount() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Number of commands that were rejected by this queue.
*
*
* optional int32 rejectedCommandsCount = 16;
* @return The rejectedCommandsCount.
*/
@java.lang.Override
public int getRejectedCommandsCount() {
return rejectedCommandsCount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getEntriesCount(); i++) {
if (!getEntries(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, processorName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeEnum(4, state_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(9, order_);
}
for (int i = 0; i < users_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, users_.getRaw(i));
}
for (int i = 0; i < groups_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, groups_.getRaw(i));
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeEnum(12, minLevel_);
}
for (int i = 0; i < tcPatterns_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, tcPatterns_.getRaw(i));
}
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(14, entries_.get(i));
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeInt32(15, acceptedCommandsCount_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeInt32(16, rejectedCommandsCount_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, processorName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, state_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(9, order_);
}
{
int dataSize = 0;
for (int i = 0; i < users_.size(); i++) {
dataSize += computeStringSizeNoTag(users_.getRaw(i));
}
size += dataSize;
size += 1 * getUsersList().size();
}
{
int dataSize = 0;
for (int i = 0; i < groups_.size(); i++) {
dataSize += computeStringSizeNoTag(groups_.getRaw(i));
}
size += dataSize;
size += 1 * getGroupsList().size();
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(12, minLevel_);
}
{
int dataSize = 0;
for (int i = 0; i < tcPatterns_.size(); i++) {
dataSize += computeStringSizeNoTag(tcPatterns_.getRaw(i));
}
size += dataSize;
size += 1 * getTcPatternsList().size();
}
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, entries_.get(i));
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(15, acceptedCommandsCount_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(16, rejectedCommandsCount_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.CommandQueueInfo)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.CommandQueueInfo other = (org.yamcs.protobuf.Commanding.CommandQueueInfo) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasProcessorName() != other.hasProcessorName()) return false;
if (hasProcessorName()) {
if (!getProcessorName()
.equals(other.getProcessorName())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasState() != other.hasState()) return false;
if (hasState()) {
if (state_ != other.state_) return false;
}
if (hasOrder() != other.hasOrder()) return false;
if (hasOrder()) {
if (getOrder()
!= other.getOrder()) return false;
}
if (!getUsersList()
.equals(other.getUsersList())) return false;
if (!getGroupsList()
.equals(other.getGroupsList())) return false;
if (hasMinLevel() != other.hasMinLevel()) return false;
if (hasMinLevel()) {
if (minLevel_ != other.minLevel_) return false;
}
if (!getTcPatternsList()
.equals(other.getTcPatternsList())) return false;
if (!getEntriesList()
.equals(other.getEntriesList())) return false;
if (hasAcceptedCommandsCount() != other.hasAcceptedCommandsCount()) return false;
if (hasAcceptedCommandsCount()) {
if (getAcceptedCommandsCount()
!= other.getAcceptedCommandsCount()) return false;
}
if (hasRejectedCommandsCount() != other.hasRejectedCommandsCount()) return false;
if (hasRejectedCommandsCount()) {
if (getRejectedCommandsCount()
!= other.getRejectedCommandsCount()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasProcessorName()) {
hash = (37 * hash) + PROCESSORNAME_FIELD_NUMBER;
hash = (53 * hash) + getProcessorName().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasState()) {
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + state_;
}
if (hasOrder()) {
hash = (37 * hash) + ORDER_FIELD_NUMBER;
hash = (53 * hash) + getOrder();
}
if (getUsersCount() > 0) {
hash = (37 * hash) + USERS_FIELD_NUMBER;
hash = (53 * hash) + getUsersList().hashCode();
}
if (getGroupsCount() > 0) {
hash = (37 * hash) + GROUPS_FIELD_NUMBER;
hash = (53 * hash) + getGroupsList().hashCode();
}
if (hasMinLevel()) {
hash = (37 * hash) + MINLEVEL_FIELD_NUMBER;
hash = (53 * hash) + minLevel_;
}
if (getTcPatternsCount() > 0) {
hash = (37 * hash) + TCPATTERNS_FIELD_NUMBER;
hash = (53 * hash) + getTcPatternsList().hashCode();
}
if (getEntriesCount() > 0) {
hash = (37 * hash) + ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getEntriesList().hashCode();
}
if (hasAcceptedCommandsCount()) {
hash = (37 * hash) + ACCEPTEDCOMMANDSCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAcceptedCommandsCount();
}
if (hasRejectedCommandsCount()) {
hash = (37 * hash) + REJECTEDCOMMANDSCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getRejectedCommandsCount();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.CommandQueueInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandQueueInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.CommandQueueInfo)
org.yamcs.protobuf.Commanding.CommandQueueInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandQueueInfo.class, org.yamcs.protobuf.Commanding.CommandQueueInfo.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.CommandQueueInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEntriesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
processorName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
state_ = 1;
bitField0_ = (bitField0_ & ~0x00000008);
order_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
users_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
groups_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
minLevel_ = 1;
bitField0_ = (bitField0_ & ~0x00000080);
tcPatterns_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
} else {
entriesBuilder_.clear();
}
acceptedCommandsCount_ = 0;
bitField0_ = (bitField0_ & ~0x00000400);
rejectedCommandsCount_ = 0;
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueInfo_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueInfo getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.CommandQueueInfo.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueInfo build() {
org.yamcs.protobuf.Commanding.CommandQueueInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueInfo buildPartial() {
org.yamcs.protobuf.Commanding.CommandQueueInfo result = new org.yamcs.protobuf.Commanding.CommandQueueInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.processorName_ = processorName_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.state_ = state_;
if (((from_bitField0_ & 0x00000010) != 0)) {
result.order_ = order_;
to_bitField0_ |= 0x00000010;
}
if (((bitField0_ & 0x00000020) != 0)) {
users_ = users_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000020);
}
result.users_ = users_;
if (((bitField0_ & 0x00000040) != 0)) {
groups_ = groups_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000040);
}
result.groups_ = groups_;
if (((from_bitField0_ & 0x00000080) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.minLevel_ = minLevel_;
if (((bitField0_ & 0x00000100) != 0)) {
tcPatterns_ = tcPatterns_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000100);
}
result.tcPatterns_ = tcPatterns_;
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.acceptedCommandsCount_ = acceptedCommandsCount_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.rejectedCommandsCount_ = rejectedCommandsCount_;
to_bitField0_ |= 0x00000080;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.CommandQueueInfo) {
return mergeFrom((org.yamcs.protobuf.Commanding.CommandQueueInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.CommandQueueInfo other) {
if (other == org.yamcs.protobuf.Commanding.CommandQueueInfo.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
if (other.hasProcessorName()) {
bitField0_ |= 0x00000002;
processorName_ = other.processorName_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000004;
name_ = other.name_;
onChanged();
}
if (other.hasState()) {
setState(other.getState());
}
if (other.hasOrder()) {
setOrder(other.getOrder());
}
if (!other.users_.isEmpty()) {
if (users_.isEmpty()) {
users_ = other.users_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureUsersIsMutable();
users_.addAll(other.users_);
}
onChanged();
}
if (!other.groups_.isEmpty()) {
if (groups_.isEmpty()) {
groups_ = other.groups_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureGroupsIsMutable();
groups_.addAll(other.groups_);
}
onChanged();
}
if (other.hasMinLevel()) {
setMinLevel(other.getMinLevel());
}
if (!other.tcPatterns_.isEmpty()) {
if (tcPatterns_.isEmpty()) {
tcPatterns_ = other.tcPatterns_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureTcPatternsIsMutable();
tcPatterns_.addAll(other.tcPatterns_);
}
onChanged();
}
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000200);
entriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
if (other.hasAcceptedCommandsCount()) {
setAcceptedCommandsCount(other.getAcceptedCommandsCount());
}
if (other.hasRejectedCommandsCount()) {
setRejectedCommandsCount(other.getRejectedCommandsCount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getEntriesCount(); i++) {
if (!getEntries(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.CommandQueueInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.CommandQueueInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
private java.lang.Object processorName_ = "";
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return Whether the processorName field is set.
*/
public boolean hasProcessorName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return The processorName.
*/
public java.lang.String getProcessorName() {
java.lang.Object ref = processorName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
processorName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return The bytes for processorName.
*/
public com.google.protobuf.ByteString
getProcessorNameBytes() {
java.lang.Object ref = processorName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
processorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @param value The processorName to set.
* @return This builder for chaining.
*/
public Builder setProcessorName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
processorName_ = value;
onChanged();
return this;
}
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @return This builder for chaining.
*/
public Builder clearProcessorName() {
bitField0_ = (bitField0_ & ~0x00000002);
processorName_ = getDefaultInstance().getProcessorName();
onChanged();
return this;
}
/**
*
* Processor name
*
*
* optional string processorName = 2;
* @param value The bytes for processorName to set.
* @return This builder for chaining.
*/
public Builder setProcessorNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
processorName_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Command queue name
*
*
* optional string name = 3;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
/**
*
* Command queue name
*
*
* optional string name = 3;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000004);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Command queue name
*
*
* optional string name = 3;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
private int state_ = 1;
/**
*
* Current queue state
*
*
* optional .yamcs.protobuf.commanding.QueueState state = 4;
* @return Whether the state field is set.
*/
@java.lang.Override public boolean hasState() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Current queue state
*
*
* optional .yamcs.protobuf.commanding.QueueState state = 4;
* @return The state.
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.QueueState getState() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Commanding.QueueState result = org.yamcs.protobuf.Commanding.QueueState.valueOf(state_);
return result == null ? org.yamcs.protobuf.Commanding.QueueState.BLOCKED : result;
}
/**
*
* Current queue state
*
*
* optional .yamcs.protobuf.commanding.QueueState state = 4;
* @param value The state to set.
* @return This builder for chaining.
*/
public Builder setState(org.yamcs.protobuf.Commanding.QueueState value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
state_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Current queue state
*
*
* optional .yamcs.protobuf.commanding.QueueState state = 4;
* @return This builder for chaining.
*/
public Builder clearState() {
bitField0_ = (bitField0_ & ~0x00000008);
state_ = 1;
onChanged();
return this;
}
private int order_ ;
/**
*
* Submitted commands are matches to the first queue that
* whose filter criteria (if any) match the command's
* features. Queues are considered in the order specified by
* this field, going from lowest to highest.
*
*
* optional int32 order = 9;
* @return Whether the order field is set.
*/
@java.lang.Override
public boolean hasOrder() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Submitted commands are matches to the first queue that
* whose filter criteria (if any) match the command's
* features. Queues are considered in the order specified by
* this field, going from lowest to highest.
*
*
* optional int32 order = 9;
* @return The order.
*/
@java.lang.Override
public int getOrder() {
return order_;
}
/**
*
* Submitted commands are matches to the first queue that
* whose filter criteria (if any) match the command's
* features. Queues are considered in the order specified by
* this field, going from lowest to highest.
*
*
* optional int32 order = 9;
* @param value The order to set.
* @return This builder for chaining.
*/
public Builder setOrder(int value) {
bitField0_ |= 0x00000010;
order_ = value;
onChanged();
return this;
}
/**
*
* Submitted commands are matches to the first queue that
* whose filter criteria (if any) match the command's
* features. Queues are considered in the order specified by
* this field, going from lowest to highest.
*
*
* optional int32 order = 9;
* @return This builder for chaining.
*/
public Builder clearOrder() {
bitField0_ = (bitField0_ & ~0x00000010);
order_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList users_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureUsersIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
users_ = new com.google.protobuf.LazyStringArrayList(users_);
bitField0_ |= 0x00000020;
}
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @return A list containing the users.
*/
public com.google.protobuf.ProtocolStringList
getUsersList() {
return users_.getUnmodifiableView();
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @return The count of users.
*/
public int getUsersCount() {
return users_.size();
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param index The index of the element to return.
* @return The users at the given index.
*/
public java.lang.String getUsers(int index) {
return users_.get(index);
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param index The index of the value to return.
* @return The bytes of the users at the given index.
*/
public com.google.protobuf.ByteString
getUsersBytes(int index) {
return users_.getByteString(index);
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param index The index to set the value at.
* @param value The users to set.
* @return This builder for chaining.
*/
public Builder setUsers(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureUsersIsMutable();
users_.set(index, value);
onChanged();
return this;
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param value The users to add.
* @return This builder for chaining.
*/
public Builder addUsers(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureUsersIsMutable();
users_.add(value);
onChanged();
return this;
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param values The users to add.
* @return This builder for chaining.
*/
public Builder addAllUsers(
java.lang.Iterable values) {
ensureUsersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, users_);
onChanged();
return this;
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @return This builder for chaining.
*/
public Builder clearUsers() {
users_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
* This queue only considers commands that are issued
* by one of the users in this list.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string users = 10;
* @param value The bytes of the users to add.
* @return This builder for chaining.
*/
public Builder addUsersBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureUsersIsMutable();
users_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList groups_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureGroupsIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
groups_ = new com.google.protobuf.LazyStringArrayList(groups_);
bitField0_ |= 0x00000040;
}
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @return A list containing the groups.
*/
public com.google.protobuf.ProtocolStringList
getGroupsList() {
return groups_.getUnmodifiableView();
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @return The count of groups.
*/
public int getGroupsCount() {
return groups_.size();
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param index The index of the element to return.
* @return The groups at the given index.
*/
public java.lang.String getGroups(int index) {
return groups_.get(index);
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param index The index of the value to return.
* @return The bytes of the groups at the given index.
*/
public com.google.protobuf.ByteString
getGroupsBytes(int index) {
return groups_.getByteString(index);
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param index The index to set the value at.
* @param value The groups to set.
* @return This builder for chaining.
*/
public Builder setGroups(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupsIsMutable();
groups_.set(index, value);
onChanged();
return this;
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param value The groups to add.
* @return This builder for chaining.
*/
public Builder addGroups(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupsIsMutable();
groups_.add(value);
onChanged();
return this;
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param values The groups to add.
* @return This builder for chaining.
*/
public Builder addAllGroups(
java.lang.Iterable values) {
ensureGroupsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, groups_);
onChanged();
return this;
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @return This builder for chaining.
*/
public Builder clearGroups() {
groups_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* This queue only considers commands that are issued
* by one of the users who belongs to any of these groups.
* If the list is empty, all commands are considered.
* Note that users/groups are considered at the same time
* (a match with any of the two is sufficient).
*
*
* repeated string groups = 11;
* @param value The bytes of the groups to add.
* @return This builder for chaining.
*/
public Builder addGroupsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupsIsMutable();
groups_.add(value);
onChanged();
return this;
}
private int minLevel_ = 1;
/**
*
* This queue only considers commands that are at least
* as significant as this level.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType minLevel = 12;
* @return Whether the minLevel field is set.
*/
@java.lang.Override public boolean hasMinLevel() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* This queue only considers commands that are at least
* as significant as this level.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType minLevel = 12;
* @return The minLevel.
*/
@java.lang.Override
public org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType getMinLevel() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType result = org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType.valueOf(minLevel_);
return result == null ? org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType.NONE : result;
}
/**
*
* This queue only considers commands that are at least
* as significant as this level.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType minLevel = 12;
* @param value The minLevel to set.
* @return This builder for chaining.
*/
public Builder setMinLevel(org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
minLevel_ = value.getNumber();
onChanged();
return this;
}
/**
*
* This queue only considers commands that are at least
* as significant as this level.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType minLevel = 12;
* @return This builder for chaining.
*/
public Builder clearMinLevel() {
bitField0_ = (bitField0_ & ~0x00000080);
minLevel_ = 1;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList tcPatterns_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTcPatternsIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
tcPatterns_ = new com.google.protobuf.LazyStringArrayList(tcPatterns_);
bitField0_ |= 0x00000100;
}
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @return A list containing the tcPatterns.
*/
public com.google.protobuf.ProtocolStringList
getTcPatternsList() {
return tcPatterns_.getUnmodifiableView();
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @return The count of tcPatterns.
*/
public int getTcPatternsCount() {
return tcPatterns_.size();
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param index The index of the element to return.
* @return The tcPatterns at the given index.
*/
public java.lang.String getTcPatterns(int index) {
return tcPatterns_.get(index);
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param index The index of the value to return.
* @return The bytes of the tcPatterns at the given index.
*/
public com.google.protobuf.ByteString
getTcPatternsBytes(int index) {
return tcPatterns_.getByteString(index);
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param index The index to set the value at.
* @param value The tcPatterns to set.
* @return This builder for chaining.
*/
public Builder setTcPatterns(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTcPatternsIsMutable();
tcPatterns_.set(index, value);
onChanged();
return this;
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param value The tcPatterns to add.
* @return This builder for chaining.
*/
public Builder addTcPatterns(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTcPatternsIsMutable();
tcPatterns_.add(value);
onChanged();
return this;
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param values The tcPatterns to add.
* @return This builder for chaining.
*/
public Builder addAllTcPatterns(
java.lang.Iterable values) {
ensureTcPatternsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tcPatterns_);
onChanged();
return this;
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @return This builder for chaining.
*/
public Builder clearTcPatterns() {
tcPatterns_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
*
* This queue only considers commands whose qualified name
* matches any of the regular expressions in this list.
* If the list is empty, all commands are considered.
*
*
* repeated string tcPatterns = 13;
* @param value The bytes of the tcPatterns to add.
* @return This builder for chaining.
*/
public Builder addTcPatternsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTcPatternsIsMutable();
tcPatterns_.add(value);
onChanged();
return this;
}
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000200) != 0)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000200;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueEntry, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder, org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder> entriesBuilder_;
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntry getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public Builder setEntries(
int index, org.yamcs.protobuf.Commanding.CommandQueueEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public Builder setEntries(
int index, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public Builder addEntries(org.yamcs.protobuf.Commanding.CommandQueueEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public Builder addEntries(
int index, org.yamcs.protobuf.Commanding.CommandQueueEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public Builder addEntries(
org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public Builder addEntries(
int index, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public Builder addAllEntries(
java.lang.Iterable extends org.yamcs.protobuf.Commanding.CommandQueueEntry> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public java.util.List extends org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance());
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance());
}
/**
*
* Currently pending (queued) commands
*
*
* repeated .yamcs.protobuf.commanding.CommandQueueEntry entries = 14;
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueEntry, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder, org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueEntry, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder, org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder>(
entries_,
((bitField0_ & 0x00000200) != 0),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
private int acceptedCommandsCount_ ;
/**
*
* Number of commands that successfully passed through this queue.
*
*
* optional int32 acceptedCommandsCount = 15;
* @return Whether the acceptedCommandsCount field is set.
*/
@java.lang.Override
public boolean hasAcceptedCommandsCount() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Number of commands that successfully passed through this queue.
*
*
* optional int32 acceptedCommandsCount = 15;
* @return The acceptedCommandsCount.
*/
@java.lang.Override
public int getAcceptedCommandsCount() {
return acceptedCommandsCount_;
}
/**
*
* Number of commands that successfully passed through this queue.
*
*
* optional int32 acceptedCommandsCount = 15;
* @param value The acceptedCommandsCount to set.
* @return This builder for chaining.
*/
public Builder setAcceptedCommandsCount(int value) {
bitField0_ |= 0x00000400;
acceptedCommandsCount_ = value;
onChanged();
return this;
}
/**
*
* Number of commands that successfully passed through this queue.
*
*
* optional int32 acceptedCommandsCount = 15;
* @return This builder for chaining.
*/
public Builder clearAcceptedCommandsCount() {
bitField0_ = (bitField0_ & ~0x00000400);
acceptedCommandsCount_ = 0;
onChanged();
return this;
}
private int rejectedCommandsCount_ ;
/**
*
* Number of commands that were rejected by this queue.
*
*
* optional int32 rejectedCommandsCount = 16;
* @return Whether the rejectedCommandsCount field is set.
*/
@java.lang.Override
public boolean hasRejectedCommandsCount() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* Number of commands that were rejected by this queue.
*
*
* optional int32 rejectedCommandsCount = 16;
* @return The rejectedCommandsCount.
*/
@java.lang.Override
public int getRejectedCommandsCount() {
return rejectedCommandsCount_;
}
/**
*
* Number of commands that were rejected by this queue.
*
*
* optional int32 rejectedCommandsCount = 16;
* @param value The rejectedCommandsCount to set.
* @return This builder for chaining.
*/
public Builder setRejectedCommandsCount(int value) {
bitField0_ |= 0x00000800;
rejectedCommandsCount_ = value;
onChanged();
return this;
}
/**
*
* Number of commands that were rejected by this queue.
*
*
* optional int32 rejectedCommandsCount = 16;
* @return This builder for chaining.
*/
public Builder clearRejectedCommandsCount() {
bitField0_ = (bitField0_ & ~0x00000800);
rejectedCommandsCount_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.CommandQueueInfo)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.CommandQueueInfo)
private static final org.yamcs.protobuf.Commanding.CommandQueueInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.CommandQueueInfo();
}
public static org.yamcs.protobuf.Commanding.CommandQueueInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandQueueInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandQueueInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandQueueEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.CommandQueueEntry)
com.google.protobuf.MessageOrBuilder {
/**
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
* optional string processorName = 2;
* @return Whether the processorName field is set.
*/
boolean hasProcessorName();
/**
* optional string processorName = 2;
* @return The processorName.
*/
java.lang.String getProcessorName();
/**
* optional string processorName = 2;
* @return The bytes for processorName.
*/
com.google.protobuf.ByteString
getProcessorNameBytes();
/**
* optional string queueName = 3;
* @return Whether the queueName field is set.
*/
boolean hasQueueName();
/**
* optional string queueName = 3;
* @return The queueName.
*/
java.lang.String getQueueName();
/**
* optional string queueName = 3;
* @return The bytes for queueName.
*/
com.google.protobuf.ByteString
getQueueNameBytes();
/**
* optional string id = 14;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* optional string id = 14;
* @return The id.
*/
java.lang.String getId();
/**
* optional string id = 14;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* optional string origin = 15;
* @return Whether the origin field is set.
*/
boolean hasOrigin();
/**
* optional string origin = 15;
* @return The origin.
*/
java.lang.String getOrigin();
/**
* optional string origin = 15;
* @return The bytes for origin.
*/
com.google.protobuf.ByteString
getOriginBytes();
/**
* optional int32 sequenceNumber = 16;
* @return Whether the sequenceNumber field is set.
*/
boolean hasSequenceNumber();
/**
* optional int32 sequenceNumber = 16;
* @return The sequenceNumber.
*/
int getSequenceNumber();
/**
* optional string commandName = 17;
* @return Whether the commandName field is set.
*/
boolean hasCommandName();
/**
* optional string commandName = 17;
* @return The commandName.
*/
java.lang.String getCommandName();
/**
* optional string commandName = 17;
* @return The bytes for commandName.
*/
com.google.protobuf.ByteString
getCommandNameBytes();
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
java.util.List
getAssignmentsList();
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
org.yamcs.protobuf.Commanding.CommandAssignment getAssignments(int index);
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
int getAssignmentsCount();
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
java.util.List extends org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>
getAssignmentsOrBuilderList();
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder getAssignmentsOrBuilder(
int index);
/**
* optional bytes binary = 6;
* @return Whether the binary field is set.
*/
boolean hasBinary();
/**
* optional bytes binary = 6;
* @return The binary.
*/
com.google.protobuf.ByteString getBinary();
/**
* optional string username = 7;
* @return Whether the username field is set.
*/
boolean hasUsername();
/**
* optional string username = 7;
* @return The username.
*/
java.lang.String getUsername();
/**
* optional string username = 7;
* @return The bytes for username.
*/
com.google.protobuf.ByteString
getUsernameBytes();
/**
* optional string comment = 11;
* @return Whether the comment field is set.
*/
boolean hasComment();
/**
* optional string comment = 11;
* @return The comment.
*/
java.lang.String getComment();
/**
* optional string comment = 11;
* @return The bytes for comment.
*/
com.google.protobuf.ByteString
getCommentBytes();
/**
* optional .google.protobuf.Timestamp generationTime = 12;
* @return Whether the generationTime field is set.
*/
boolean hasGenerationTime();
/**
* optional .google.protobuf.Timestamp generationTime = 12;
* @return The generationTime.
*/
com.google.protobuf.Timestamp getGenerationTime();
/**
* optional .google.protobuf.Timestamp generationTime = 12;
*/
com.google.protobuf.TimestampOrBuilder getGenerationTimeOrBuilder();
/**
*
* If true, the command has been accepted and is due for release
* as soon as transmission constraints are satisfied.
*
*
* optional bool pendingTransmissionConstraints = 13;
* @return Whether the pendingTransmissionConstraints field is set.
*/
boolean hasPendingTransmissionConstraints();
/**
*
* If true, the command has been accepted and is due for release
* as soon as transmission constraints are satisfied.
*
*
* optional bool pendingTransmissionConstraints = 13;
* @return The pendingTransmissionConstraints.
*/
boolean getPendingTransmissionConstraints();
}
/**
*
*One entry (command) in the command queue
*
*
* Protobuf type {@code yamcs.protobuf.commanding.CommandQueueEntry}
*/
public static final class CommandQueueEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.CommandQueueEntry)
CommandQueueEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandQueueEntry.newBuilder() to construct.
private CommandQueueEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandQueueEntry() {
instance_ = "";
processorName_ = "";
queueName_ = "";
id_ = "";
origin_ = "";
commandName_ = "";
assignments_ = java.util.Collections.emptyList();
binary_ = com.google.protobuf.ByteString.EMPTY;
username_ = "";
comment_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandQueueEntry();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandQueueEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
processorName_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
queueName_ = bs;
break;
}
case 50: {
bitField0_ |= 0x00000080;
binary_ = input.readBytes();
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000100;
username_ = bs;
break;
}
case 90: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000200;
comment_ = bs;
break;
}
case 98: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (((bitField0_ & 0x00000400) != 0)) {
subBuilder = generationTime_.toBuilder();
}
generationTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(generationTime_);
generationTime_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000400;
break;
}
case 104: {
bitField0_ |= 0x00000800;
pendingTransmissionConstraints_ = input.readBool();
break;
}
case 114: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
id_ = bs;
break;
}
case 122: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
origin_ = bs;
break;
}
case 128: {
bitField0_ |= 0x00000020;
sequenceNumber_ = input.readInt32();
break;
}
case 138: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
commandName_ = bs;
break;
}
case 146: {
if (!((mutable_bitField0_ & 0x00000080) != 0)) {
assignments_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
assignments_.add(
input.readMessage(org.yamcs.protobuf.Commanding.CommandAssignment.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000080) != 0)) {
assignments_ = java.util.Collections.unmodifiableList(assignments_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandQueueEntry.class, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROCESSORNAME_FIELD_NUMBER = 2;
private volatile java.lang.Object processorName_;
/**
* optional string processorName = 2;
* @return Whether the processorName field is set.
*/
@java.lang.Override
public boolean hasProcessorName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string processorName = 2;
* @return The processorName.
*/
@java.lang.Override
public java.lang.String getProcessorName() {
java.lang.Object ref = processorName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
processorName_ = s;
}
return s;
}
}
/**
* optional string processorName = 2;
* @return The bytes for processorName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProcessorNameBytes() {
java.lang.Object ref = processorName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
processorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int QUEUENAME_FIELD_NUMBER = 3;
private volatile java.lang.Object queueName_;
/**
* optional string queueName = 3;
* @return Whether the queueName field is set.
*/
@java.lang.Override
public boolean hasQueueName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string queueName = 3;
* @return The queueName.
*/
@java.lang.Override
public java.lang.String getQueueName() {
java.lang.Object ref = queueName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
queueName_ = s;
}
return s;
}
}
/**
* optional string queueName = 3;
* @return The bytes for queueName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getQueueNameBytes() {
java.lang.Object ref = queueName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queueName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 14;
private volatile java.lang.Object id_;
/**
* optional string id = 14;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string id = 14;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 14;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ORIGIN_FIELD_NUMBER = 15;
private volatile java.lang.Object origin_;
/**
* optional string origin = 15;
* @return Whether the origin field is set.
*/
@java.lang.Override
public boolean hasOrigin() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string origin = 15;
* @return The origin.
*/
@java.lang.Override
public java.lang.String getOrigin() {
java.lang.Object ref = origin_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
origin_ = s;
}
return s;
}
}
/**
* optional string origin = 15;
* @return The bytes for origin.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOriginBytes() {
java.lang.Object ref = origin_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
origin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEQUENCENUMBER_FIELD_NUMBER = 16;
private int sequenceNumber_;
/**
* optional int32 sequenceNumber = 16;
* @return Whether the sequenceNumber field is set.
*/
@java.lang.Override
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional int32 sequenceNumber = 16;
* @return The sequenceNumber.
*/
@java.lang.Override
public int getSequenceNumber() {
return sequenceNumber_;
}
public static final int COMMANDNAME_FIELD_NUMBER = 17;
private volatile java.lang.Object commandName_;
/**
* optional string commandName = 17;
* @return Whether the commandName field is set.
*/
@java.lang.Override
public boolean hasCommandName() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional string commandName = 17;
* @return The commandName.
*/
@java.lang.Override
public java.lang.String getCommandName() {
java.lang.Object ref = commandName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
commandName_ = s;
}
return s;
}
}
/**
* optional string commandName = 17;
* @return The bytes for commandName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCommandNameBytes() {
java.lang.Object ref = commandName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commandName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ASSIGNMENTS_FIELD_NUMBER = 18;
private java.util.List assignments_;
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
@java.lang.Override
public java.util.List getAssignmentsList() {
return assignments_;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>
getAssignmentsOrBuilderList() {
return assignments_;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
@java.lang.Override
public int getAssignmentsCount() {
return assignments_.size();
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandAssignment getAssignments(int index) {
return assignments_.get(index);
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder getAssignmentsOrBuilder(
int index) {
return assignments_.get(index);
}
public static final int BINARY_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString binary_;
/**
* optional bytes binary = 6;
* @return Whether the binary field is set.
*/
@java.lang.Override
public boolean hasBinary() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional bytes binary = 6;
* @return The binary.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBinary() {
return binary_;
}
public static final int USERNAME_FIELD_NUMBER = 7;
private volatile java.lang.Object username_;
/**
* optional string username = 7;
* @return Whether the username field is set.
*/
@java.lang.Override
public boolean hasUsername() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional string username = 7;
* @return The username.
*/
@java.lang.Override
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
username_ = s;
}
return s;
}
}
/**
* optional string username = 7;
* @return The bytes for username.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMMENT_FIELD_NUMBER = 11;
private volatile java.lang.Object comment_;
/**
* optional string comment = 11;
* @return Whether the comment field is set.
*/
@java.lang.Override
public boolean hasComment() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional string comment = 11;
* @return The comment.
*/
@java.lang.Override
public java.lang.String getComment() {
java.lang.Object ref = comment_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
comment_ = s;
}
return s;
}
}
/**
* optional string comment = 11;
* @return The bytes for comment.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCommentBytes() {
java.lang.Object ref = comment_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
comment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GENERATIONTIME_FIELD_NUMBER = 12;
private com.google.protobuf.Timestamp generationTime_;
/**
* optional .google.protobuf.Timestamp generationTime = 12;
* @return Whether the generationTime field is set.
*/
@java.lang.Override
public boolean hasGenerationTime() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
* @return The generationTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getGenerationTime() {
return generationTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : generationTime_;
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getGenerationTimeOrBuilder() {
return generationTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : generationTime_;
}
public static final int PENDINGTRANSMISSIONCONSTRAINTS_FIELD_NUMBER = 13;
private boolean pendingTransmissionConstraints_;
/**
*
* If true, the command has been accepted and is due for release
* as soon as transmission constraints are satisfied.
*
*
* optional bool pendingTransmissionConstraints = 13;
* @return Whether the pendingTransmissionConstraints field is set.
*/
@java.lang.Override
public boolean hasPendingTransmissionConstraints() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* If true, the command has been accepted and is due for release
* as soon as transmission constraints are satisfied.
*
*
* optional bool pendingTransmissionConstraints = 13;
* @return The pendingTransmissionConstraints.
*/
@java.lang.Override
public boolean getPendingTransmissionConstraints() {
return pendingTransmissionConstraints_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getAssignmentsCount(); i++) {
if (!getAssignments(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, processorName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, queueName_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeBytes(6, binary_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, username_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, comment_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeMessage(12, getGenerationTime());
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeBool(13, pendingTransmissionConstraints_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, id_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, origin_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt32(16, sequenceNumber_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, commandName_);
}
for (int i = 0; i < assignments_.size(); i++) {
output.writeMessage(18, assignments_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, processorName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, queueName_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, binary_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, username_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, comment_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getGenerationTime());
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, pendingTransmissionConstraints_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, id_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, origin_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(16, sequenceNumber_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, commandName_);
}
for (int i = 0; i < assignments_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, assignments_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.CommandQueueEntry)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.CommandQueueEntry other = (org.yamcs.protobuf.Commanding.CommandQueueEntry) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasProcessorName() != other.hasProcessorName()) return false;
if (hasProcessorName()) {
if (!getProcessorName()
.equals(other.getProcessorName())) return false;
}
if (hasQueueName() != other.hasQueueName()) return false;
if (hasQueueName()) {
if (!getQueueName()
.equals(other.getQueueName())) return false;
}
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (hasOrigin() != other.hasOrigin()) return false;
if (hasOrigin()) {
if (!getOrigin()
.equals(other.getOrigin())) return false;
}
if (hasSequenceNumber() != other.hasSequenceNumber()) return false;
if (hasSequenceNumber()) {
if (getSequenceNumber()
!= other.getSequenceNumber()) return false;
}
if (hasCommandName() != other.hasCommandName()) return false;
if (hasCommandName()) {
if (!getCommandName()
.equals(other.getCommandName())) return false;
}
if (!getAssignmentsList()
.equals(other.getAssignmentsList())) return false;
if (hasBinary() != other.hasBinary()) return false;
if (hasBinary()) {
if (!getBinary()
.equals(other.getBinary())) return false;
}
if (hasUsername() != other.hasUsername()) return false;
if (hasUsername()) {
if (!getUsername()
.equals(other.getUsername())) return false;
}
if (hasComment() != other.hasComment()) return false;
if (hasComment()) {
if (!getComment()
.equals(other.getComment())) return false;
}
if (hasGenerationTime() != other.hasGenerationTime()) return false;
if (hasGenerationTime()) {
if (!getGenerationTime()
.equals(other.getGenerationTime())) return false;
}
if (hasPendingTransmissionConstraints() != other.hasPendingTransmissionConstraints()) return false;
if (hasPendingTransmissionConstraints()) {
if (getPendingTransmissionConstraints()
!= other.getPendingTransmissionConstraints()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasProcessorName()) {
hash = (37 * hash) + PROCESSORNAME_FIELD_NUMBER;
hash = (53 * hash) + getProcessorName().hashCode();
}
if (hasQueueName()) {
hash = (37 * hash) + QUEUENAME_FIELD_NUMBER;
hash = (53 * hash) + getQueueName().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasOrigin()) {
hash = (37 * hash) + ORIGIN_FIELD_NUMBER;
hash = (53 * hash) + getOrigin().hashCode();
}
if (hasSequenceNumber()) {
hash = (37 * hash) + SEQUENCENUMBER_FIELD_NUMBER;
hash = (53 * hash) + getSequenceNumber();
}
if (hasCommandName()) {
hash = (37 * hash) + COMMANDNAME_FIELD_NUMBER;
hash = (53 * hash) + getCommandName().hashCode();
}
if (getAssignmentsCount() > 0) {
hash = (37 * hash) + ASSIGNMENTS_FIELD_NUMBER;
hash = (53 * hash) + getAssignmentsList().hashCode();
}
if (hasBinary()) {
hash = (37 * hash) + BINARY_FIELD_NUMBER;
hash = (53 * hash) + getBinary().hashCode();
}
if (hasUsername()) {
hash = (37 * hash) + USERNAME_FIELD_NUMBER;
hash = (53 * hash) + getUsername().hashCode();
}
if (hasComment()) {
hash = (37 * hash) + COMMENT_FIELD_NUMBER;
hash = (53 * hash) + getComment().hashCode();
}
if (hasGenerationTime()) {
hash = (37 * hash) + GENERATIONTIME_FIELD_NUMBER;
hash = (53 * hash) + getGenerationTime().hashCode();
}
if (hasPendingTransmissionConstraints()) {
hash = (37 * hash) + PENDINGTRANSMISSIONCONSTRAINTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPendingTransmissionConstraints());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.CommandQueueEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*One entry (command) in the command queue
*
*
* Protobuf type {@code yamcs.protobuf.commanding.CommandQueueEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.CommandQueueEntry)
org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandQueueEntry.class, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.CommandQueueEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAssignmentsFieldBuilder();
getGenerationTimeFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
processorName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
queueName_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
id_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
origin_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
sequenceNumber_ = 0;
bitField0_ = (bitField0_ & ~0x00000020);
commandName_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
if (assignmentsBuilder_ == null) {
assignments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
assignmentsBuilder_.clear();
}
binary_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
username_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
comment_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
if (generationTimeBuilder_ == null) {
generationTime_ = null;
} else {
generationTimeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
pendingTransmissionConstraints_ = false;
bitField0_ = (bitField0_ & ~0x00001000);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEntry_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntry getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntry build() {
org.yamcs.protobuf.Commanding.CommandQueueEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntry buildPartial() {
org.yamcs.protobuf.Commanding.CommandQueueEntry result = new org.yamcs.protobuf.Commanding.CommandQueueEntry(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.processorName_ = processorName_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.queueName_ = queueName_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.origin_ = origin_;
if (((from_bitField0_ & 0x00000020) != 0)) {
result.sequenceNumber_ = sequenceNumber_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.commandName_ = commandName_;
if (assignmentsBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
assignments_ = java.util.Collections.unmodifiableList(assignments_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.assignments_ = assignments_;
} else {
result.assignments_ = assignmentsBuilder_.build();
}
if (((from_bitField0_ & 0x00000100) != 0)) {
to_bitField0_ |= 0x00000080;
}
result.binary_ = binary_;
if (((from_bitField0_ & 0x00000200) != 0)) {
to_bitField0_ |= 0x00000100;
}
result.username_ = username_;
if (((from_bitField0_ & 0x00000400) != 0)) {
to_bitField0_ |= 0x00000200;
}
result.comment_ = comment_;
if (((from_bitField0_ & 0x00000800) != 0)) {
if (generationTimeBuilder_ == null) {
result.generationTime_ = generationTime_;
} else {
result.generationTime_ = generationTimeBuilder_.build();
}
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.pendingTransmissionConstraints_ = pendingTransmissionConstraints_;
to_bitField0_ |= 0x00000800;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.CommandQueueEntry) {
return mergeFrom((org.yamcs.protobuf.Commanding.CommandQueueEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.CommandQueueEntry other) {
if (other == org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
if (other.hasProcessorName()) {
bitField0_ |= 0x00000002;
processorName_ = other.processorName_;
onChanged();
}
if (other.hasQueueName()) {
bitField0_ |= 0x00000004;
queueName_ = other.queueName_;
onChanged();
}
if (other.hasId()) {
bitField0_ |= 0x00000008;
id_ = other.id_;
onChanged();
}
if (other.hasOrigin()) {
bitField0_ |= 0x00000010;
origin_ = other.origin_;
onChanged();
}
if (other.hasSequenceNumber()) {
setSequenceNumber(other.getSequenceNumber());
}
if (other.hasCommandName()) {
bitField0_ |= 0x00000040;
commandName_ = other.commandName_;
onChanged();
}
if (assignmentsBuilder_ == null) {
if (!other.assignments_.isEmpty()) {
if (assignments_.isEmpty()) {
assignments_ = other.assignments_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureAssignmentsIsMutable();
assignments_.addAll(other.assignments_);
}
onChanged();
}
} else {
if (!other.assignments_.isEmpty()) {
if (assignmentsBuilder_.isEmpty()) {
assignmentsBuilder_.dispose();
assignmentsBuilder_ = null;
assignments_ = other.assignments_;
bitField0_ = (bitField0_ & ~0x00000080);
assignmentsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAssignmentsFieldBuilder() : null;
} else {
assignmentsBuilder_.addAllMessages(other.assignments_);
}
}
}
if (other.hasBinary()) {
setBinary(other.getBinary());
}
if (other.hasUsername()) {
bitField0_ |= 0x00000200;
username_ = other.username_;
onChanged();
}
if (other.hasComment()) {
bitField0_ |= 0x00000400;
comment_ = other.comment_;
onChanged();
}
if (other.hasGenerationTime()) {
mergeGenerationTime(other.getGenerationTime());
}
if (other.hasPendingTransmissionConstraints()) {
setPendingTransmissionConstraints(other.getPendingTransmissionConstraints());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getAssignmentsCount(); i++) {
if (!getAssignments(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.CommandQueueEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.CommandQueueEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
private java.lang.Object processorName_ = "";
/**
* optional string processorName = 2;
* @return Whether the processorName field is set.
*/
public boolean hasProcessorName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string processorName = 2;
* @return The processorName.
*/
public java.lang.String getProcessorName() {
java.lang.Object ref = processorName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
processorName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string processorName = 2;
* @return The bytes for processorName.
*/
public com.google.protobuf.ByteString
getProcessorNameBytes() {
java.lang.Object ref = processorName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
processorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string processorName = 2;
* @param value The processorName to set.
* @return This builder for chaining.
*/
public Builder setProcessorName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
processorName_ = value;
onChanged();
return this;
}
/**
* optional string processorName = 2;
* @return This builder for chaining.
*/
public Builder clearProcessorName() {
bitField0_ = (bitField0_ & ~0x00000002);
processorName_ = getDefaultInstance().getProcessorName();
onChanged();
return this;
}
/**
* optional string processorName = 2;
* @param value The bytes for processorName to set.
* @return This builder for chaining.
*/
public Builder setProcessorNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
processorName_ = value;
onChanged();
return this;
}
private java.lang.Object queueName_ = "";
/**
* optional string queueName = 3;
* @return Whether the queueName field is set.
*/
public boolean hasQueueName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string queueName = 3;
* @return The queueName.
*/
public java.lang.String getQueueName() {
java.lang.Object ref = queueName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
queueName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string queueName = 3;
* @return The bytes for queueName.
*/
public com.google.protobuf.ByteString
getQueueNameBytes() {
java.lang.Object ref = queueName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queueName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string queueName = 3;
* @param value The queueName to set.
* @return This builder for chaining.
*/
public Builder setQueueName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
queueName_ = value;
onChanged();
return this;
}
/**
* optional string queueName = 3;
* @return This builder for chaining.
*/
public Builder clearQueueName() {
bitField0_ = (bitField0_ & ~0x00000004);
queueName_ = getDefaultInstance().getQueueName();
onChanged();
return this;
}
/**
* optional string queueName = 3;
* @param value The bytes for queueName to set.
* @return This builder for chaining.
*/
public Builder setQueueNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
queueName_ = value;
onChanged();
return this;
}
private java.lang.Object id_ = "";
/**
* optional string id = 14;
* @return Whether the id field is set.
*/
public boolean hasId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string id = 14;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 14;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 14;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 14;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000008);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 14;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
id_ = value;
onChanged();
return this;
}
private java.lang.Object origin_ = "";
/**
* optional string origin = 15;
* @return Whether the origin field is set.
*/
public boolean hasOrigin() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string origin = 15;
* @return The origin.
*/
public java.lang.String getOrigin() {
java.lang.Object ref = origin_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
origin_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string origin = 15;
* @return The bytes for origin.
*/
public com.google.protobuf.ByteString
getOriginBytes() {
java.lang.Object ref = origin_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
origin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string origin = 15;
* @param value The origin to set.
* @return This builder for chaining.
*/
public Builder setOrigin(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
origin_ = value;
onChanged();
return this;
}
/**
* optional string origin = 15;
* @return This builder for chaining.
*/
public Builder clearOrigin() {
bitField0_ = (bitField0_ & ~0x00000010);
origin_ = getDefaultInstance().getOrigin();
onChanged();
return this;
}
/**
* optional string origin = 15;
* @param value The bytes for origin to set.
* @return This builder for chaining.
*/
public Builder setOriginBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
origin_ = value;
onChanged();
return this;
}
private int sequenceNumber_ ;
/**
* optional int32 sequenceNumber = 16;
* @return Whether the sequenceNumber field is set.
*/
@java.lang.Override
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional int32 sequenceNumber = 16;
* @return The sequenceNumber.
*/
@java.lang.Override
public int getSequenceNumber() {
return sequenceNumber_;
}
/**
* optional int32 sequenceNumber = 16;
* @param value The sequenceNumber to set.
* @return This builder for chaining.
*/
public Builder setSequenceNumber(int value) {
bitField0_ |= 0x00000020;
sequenceNumber_ = value;
onChanged();
return this;
}
/**
* optional int32 sequenceNumber = 16;
* @return This builder for chaining.
*/
public Builder clearSequenceNumber() {
bitField0_ = (bitField0_ & ~0x00000020);
sequenceNumber_ = 0;
onChanged();
return this;
}
private java.lang.Object commandName_ = "";
/**
* optional string commandName = 17;
* @return Whether the commandName field is set.
*/
public boolean hasCommandName() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional string commandName = 17;
* @return The commandName.
*/
public java.lang.String getCommandName() {
java.lang.Object ref = commandName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
commandName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string commandName = 17;
* @return The bytes for commandName.
*/
public com.google.protobuf.ByteString
getCommandNameBytes() {
java.lang.Object ref = commandName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commandName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string commandName = 17;
* @param value The commandName to set.
* @return This builder for chaining.
*/
public Builder setCommandName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
commandName_ = value;
onChanged();
return this;
}
/**
* optional string commandName = 17;
* @return This builder for chaining.
*/
public Builder clearCommandName() {
bitField0_ = (bitField0_ & ~0x00000040);
commandName_ = getDefaultInstance().getCommandName();
onChanged();
return this;
}
/**
* optional string commandName = 17;
* @param value The bytes for commandName to set.
* @return This builder for chaining.
*/
public Builder setCommandNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
commandName_ = value;
onChanged();
return this;
}
private java.util.List assignments_ =
java.util.Collections.emptyList();
private void ensureAssignmentsIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
assignments_ = new java.util.ArrayList(assignments_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandAssignment, org.yamcs.protobuf.Commanding.CommandAssignment.Builder, org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder> assignmentsBuilder_;
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public java.util.List getAssignmentsList() {
if (assignmentsBuilder_ == null) {
return java.util.Collections.unmodifiableList(assignments_);
} else {
return assignmentsBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public int getAssignmentsCount() {
if (assignmentsBuilder_ == null) {
return assignments_.size();
} else {
return assignmentsBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public org.yamcs.protobuf.Commanding.CommandAssignment getAssignments(int index) {
if (assignmentsBuilder_ == null) {
return assignments_.get(index);
} else {
return assignmentsBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public Builder setAssignments(
int index, org.yamcs.protobuf.Commanding.CommandAssignment value) {
if (assignmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssignmentsIsMutable();
assignments_.set(index, value);
onChanged();
} else {
assignmentsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public Builder setAssignments(
int index, org.yamcs.protobuf.Commanding.CommandAssignment.Builder builderForValue) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
assignments_.set(index, builderForValue.build());
onChanged();
} else {
assignmentsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public Builder addAssignments(org.yamcs.protobuf.Commanding.CommandAssignment value) {
if (assignmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssignmentsIsMutable();
assignments_.add(value);
onChanged();
} else {
assignmentsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public Builder addAssignments(
int index, org.yamcs.protobuf.Commanding.CommandAssignment value) {
if (assignmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssignmentsIsMutable();
assignments_.add(index, value);
onChanged();
} else {
assignmentsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public Builder addAssignments(
org.yamcs.protobuf.Commanding.CommandAssignment.Builder builderForValue) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
assignments_.add(builderForValue.build());
onChanged();
} else {
assignmentsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public Builder addAssignments(
int index, org.yamcs.protobuf.Commanding.CommandAssignment.Builder builderForValue) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
assignments_.add(index, builderForValue.build());
onChanged();
} else {
assignmentsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public Builder addAllAssignments(
java.lang.Iterable extends org.yamcs.protobuf.Commanding.CommandAssignment> values) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, assignments_);
onChanged();
} else {
assignmentsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public Builder clearAssignments() {
if (assignmentsBuilder_ == null) {
assignments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
assignmentsBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public Builder removeAssignments(int index) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
assignments_.remove(index);
onChanged();
} else {
assignmentsBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public org.yamcs.protobuf.Commanding.CommandAssignment.Builder getAssignmentsBuilder(
int index) {
return getAssignmentsFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder getAssignmentsOrBuilder(
int index) {
if (assignmentsBuilder_ == null) {
return assignments_.get(index); } else {
return assignmentsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public java.util.List extends org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>
getAssignmentsOrBuilderList() {
if (assignmentsBuilder_ != null) {
return assignmentsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(assignments_);
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public org.yamcs.protobuf.Commanding.CommandAssignment.Builder addAssignmentsBuilder() {
return getAssignmentsFieldBuilder().addBuilder(
org.yamcs.protobuf.Commanding.CommandAssignment.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public org.yamcs.protobuf.Commanding.CommandAssignment.Builder addAssignmentsBuilder(
int index) {
return getAssignmentsFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Commanding.CommandAssignment.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 18;
*/
public java.util.List
getAssignmentsBuilderList() {
return getAssignmentsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandAssignment, org.yamcs.protobuf.Commanding.CommandAssignment.Builder, org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>
getAssignmentsFieldBuilder() {
if (assignmentsBuilder_ == null) {
assignmentsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandAssignment, org.yamcs.protobuf.Commanding.CommandAssignment.Builder, org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>(
assignments_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
assignments_ = null;
}
return assignmentsBuilder_;
}
private com.google.protobuf.ByteString binary_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes binary = 6;
* @return Whether the binary field is set.
*/
@java.lang.Override
public boolean hasBinary() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional bytes binary = 6;
* @return The binary.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBinary() {
return binary_;
}
/**
* optional bytes binary = 6;
* @param value The binary to set.
* @return This builder for chaining.
*/
public Builder setBinary(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
binary_ = value;
onChanged();
return this;
}
/**
* optional bytes binary = 6;
* @return This builder for chaining.
*/
public Builder clearBinary() {
bitField0_ = (bitField0_ & ~0x00000100);
binary_ = getDefaultInstance().getBinary();
onChanged();
return this;
}
private java.lang.Object username_ = "";
/**
* optional string username = 7;
* @return Whether the username field is set.
*/
public boolean hasUsername() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional string username = 7;
* @return The username.
*/
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
username_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string username = 7;
* @return The bytes for username.
*/
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string username = 7;
* @param value The username to set.
* @return This builder for chaining.
*/
public Builder setUsername(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
username_ = value;
onChanged();
return this;
}
/**
* optional string username = 7;
* @return This builder for chaining.
*/
public Builder clearUsername() {
bitField0_ = (bitField0_ & ~0x00000200);
username_ = getDefaultInstance().getUsername();
onChanged();
return this;
}
/**
* optional string username = 7;
* @param value The bytes for username to set.
* @return This builder for chaining.
*/
public Builder setUsernameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
username_ = value;
onChanged();
return this;
}
private java.lang.Object comment_ = "";
/**
* optional string comment = 11;
* @return Whether the comment field is set.
*/
public boolean hasComment() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional string comment = 11;
* @return The comment.
*/
public java.lang.String getComment() {
java.lang.Object ref = comment_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
comment_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string comment = 11;
* @return The bytes for comment.
*/
public com.google.protobuf.ByteString
getCommentBytes() {
java.lang.Object ref = comment_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
comment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string comment = 11;
* @param value The comment to set.
* @return This builder for chaining.
*/
public Builder setComment(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
comment_ = value;
onChanged();
return this;
}
/**
* optional string comment = 11;
* @return This builder for chaining.
*/
public Builder clearComment() {
bitField0_ = (bitField0_ & ~0x00000400);
comment_ = getDefaultInstance().getComment();
onChanged();
return this;
}
/**
* optional string comment = 11;
* @param value The bytes for comment to set.
* @return This builder for chaining.
*/
public Builder setCommentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
comment_ = value;
onChanged();
return this;
}
private com.google.protobuf.Timestamp generationTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> generationTimeBuilder_;
/**
* optional .google.protobuf.Timestamp generationTime = 12;
* @return Whether the generationTime field is set.
*/
public boolean hasGenerationTime() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
* @return The generationTime.
*/
public com.google.protobuf.Timestamp getGenerationTime() {
if (generationTimeBuilder_ == null) {
return generationTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : generationTime_;
} else {
return generationTimeBuilder_.getMessage();
}
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
*/
public Builder setGenerationTime(com.google.protobuf.Timestamp value) {
if (generationTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
generationTime_ = value;
onChanged();
} else {
generationTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
*/
public Builder setGenerationTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (generationTimeBuilder_ == null) {
generationTime_ = builderForValue.build();
onChanged();
} else {
generationTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
*/
public Builder mergeGenerationTime(com.google.protobuf.Timestamp value) {
if (generationTimeBuilder_ == null) {
if (((bitField0_ & 0x00000800) != 0) &&
generationTime_ != null &&
generationTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
generationTime_ =
com.google.protobuf.Timestamp.newBuilder(generationTime_).mergeFrom(value).buildPartial();
} else {
generationTime_ = value;
}
onChanged();
} else {
generationTimeBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
*/
public Builder clearGenerationTime() {
if (generationTimeBuilder_ == null) {
generationTime_ = null;
onChanged();
} else {
generationTimeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
*/
public com.google.protobuf.Timestamp.Builder getGenerationTimeBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getGenerationTimeFieldBuilder().getBuilder();
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
*/
public com.google.protobuf.TimestampOrBuilder getGenerationTimeOrBuilder() {
if (generationTimeBuilder_ != null) {
return generationTimeBuilder_.getMessageOrBuilder();
} else {
return generationTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : generationTime_;
}
}
/**
* optional .google.protobuf.Timestamp generationTime = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getGenerationTimeFieldBuilder() {
if (generationTimeBuilder_ == null) {
generationTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getGenerationTime(),
getParentForChildren(),
isClean());
generationTime_ = null;
}
return generationTimeBuilder_;
}
private boolean pendingTransmissionConstraints_ ;
/**
*
* If true, the command has been accepted and is due for release
* as soon as transmission constraints are satisfied.
*
*
* optional bool pendingTransmissionConstraints = 13;
* @return Whether the pendingTransmissionConstraints field is set.
*/
@java.lang.Override
public boolean hasPendingTransmissionConstraints() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* If true, the command has been accepted and is due for release
* as soon as transmission constraints are satisfied.
*
*
* optional bool pendingTransmissionConstraints = 13;
* @return The pendingTransmissionConstraints.
*/
@java.lang.Override
public boolean getPendingTransmissionConstraints() {
return pendingTransmissionConstraints_;
}
/**
*
* If true, the command has been accepted and is due for release
* as soon as transmission constraints are satisfied.
*
*
* optional bool pendingTransmissionConstraints = 13;
* @param value The pendingTransmissionConstraints to set.
* @return This builder for chaining.
*/
public Builder setPendingTransmissionConstraints(boolean value) {
bitField0_ |= 0x00001000;
pendingTransmissionConstraints_ = value;
onChanged();
return this;
}
/**
*
* If true, the command has been accepted and is due for release
* as soon as transmission constraints are satisfied.
*
*
* optional bool pendingTransmissionConstraints = 13;
* @return This builder for chaining.
*/
public Builder clearPendingTransmissionConstraints() {
bitField0_ = (bitField0_ & ~0x00001000);
pendingTransmissionConstraints_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.CommandQueueEntry)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.CommandQueueEntry)
private static final org.yamcs.protobuf.Commanding.CommandQueueEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.CommandQueueEntry();
}
public static org.yamcs.protobuf.Commanding.CommandQueueEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandQueueEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandQueueEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandQueueEventOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.CommandQueueEvent)
com.google.protobuf.MessageOrBuilder {
/**
* optional .yamcs.protobuf.commanding.CommandQueueEvent.Type type = 1;
* @return Whether the type field is set.
*/
boolean hasType();
/**
* optional .yamcs.protobuf.commanding.CommandQueueEvent.Type type = 1;
* @return The type.
*/
org.yamcs.protobuf.Commanding.CommandQueueEvent.Type getType();
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
* @return Whether the data field is set.
*/
boolean hasData();
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
* @return The data.
*/
org.yamcs.protobuf.Commanding.CommandQueueEntry getData();
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
*/
org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder getDataOrBuilder();
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandQueueEvent}
*/
public static final class CommandQueueEvent extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.CommandQueueEvent)
CommandQueueEventOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandQueueEvent.newBuilder() to construct.
private CommandQueueEvent(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandQueueEvent() {
type_ = 1;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandQueueEvent();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandQueueEvent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Commanding.CommandQueueEvent.Type value = org.yamcs.protobuf.Commanding.CommandQueueEvent.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = rawValue;
}
break;
}
case 18: {
org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = data_.toBuilder();
}
data_ = input.readMessage(org.yamcs.protobuf.Commanding.CommandQueueEntry.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(data_);
data_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandQueueEvent.class, org.yamcs.protobuf.Commanding.CommandQueueEvent.Builder.class);
}
/**
* Protobuf enum {@code yamcs.protobuf.commanding.CommandQueueEvent.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* COMMAND_ADDED = 1;
*/
COMMAND_ADDED(1),
/**
* COMMAND_REJECTED = 2;
*/
COMMAND_REJECTED(2),
/**
* COMMAND_SENT = 3;
*/
COMMAND_SENT(3),
/**
* COMMAND_UPDATED = 4;
*/
COMMAND_UPDATED(4),
;
/**
* COMMAND_ADDED = 1;
*/
public static final int COMMAND_ADDED_VALUE = 1;
/**
* COMMAND_REJECTED = 2;
*/
public static final int COMMAND_REJECTED_VALUE = 2;
/**
* COMMAND_SENT = 3;
*/
public static final int COMMAND_SENT_VALUE = 3;
/**
* COMMAND_UPDATED = 4;
*/
public static final int COMMAND_UPDATED_VALUE = 4;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Type forNumber(int value) {
switch (value) {
case 1: return COMMAND_ADDED;
case 2: return COMMAND_REJECTED;
case 3: return COMMAND_SENT;
case 4: return COMMAND_UPDATED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.CommandQueueEvent.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:yamcs.protobuf.commanding.CommandQueueEvent.Type)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* optional .yamcs.protobuf.commanding.CommandQueueEvent.Type type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEvent.Type type = 1;
* @return The type.
*/
@java.lang.Override public org.yamcs.protobuf.Commanding.CommandQueueEvent.Type getType() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Commanding.CommandQueueEvent.Type result = org.yamcs.protobuf.Commanding.CommandQueueEvent.Type.valueOf(type_);
return result == null ? org.yamcs.protobuf.Commanding.CommandQueueEvent.Type.COMMAND_ADDED : result;
}
public static final int DATA_FIELD_NUMBER = 2;
private org.yamcs.protobuf.Commanding.CommandQueueEntry data_;
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
* @return The data.
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntry getData() {
return data_ == null ? org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance() : data_;
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder getDataOrBuilder() {
return data_ == null ? org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance() : data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasData()) {
if (!getData().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getData());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getData());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.CommandQueueEvent)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.CommandQueueEvent other = (org.yamcs.protobuf.Commanding.CommandQueueEvent) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (hasData() != other.hasData()) return false;
if (hasData()) {
if (!getData()
.equals(other.getData())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.CommandQueueEvent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandQueueEvent}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.CommandQueueEvent)
org.yamcs.protobuf.Commanding.CommandQueueEventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandQueueEvent.class, org.yamcs.protobuf.Commanding.CommandQueueEvent.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.CommandQueueEvent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getDataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 1;
bitField0_ = (bitField0_ & ~0x00000001);
if (dataBuilder_ == null) {
data_ = null;
} else {
dataBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueEvent_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEvent getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.CommandQueueEvent.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEvent build() {
org.yamcs.protobuf.Commanding.CommandQueueEvent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEvent buildPartial() {
org.yamcs.protobuf.Commanding.CommandQueueEvent result = new org.yamcs.protobuf.Commanding.CommandQueueEvent(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) != 0)) {
if (dataBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = dataBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.CommandQueueEvent) {
return mergeFrom((org.yamcs.protobuf.Commanding.CommandQueueEvent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.CommandQueueEvent other) {
if (other == org.yamcs.protobuf.Commanding.CommandQueueEvent.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasData()) {
mergeData(other.getData());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasData()) {
if (!getData().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.CommandQueueEvent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.CommandQueueEvent) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 1;
/**
* optional .yamcs.protobuf.commanding.CommandQueueEvent.Type type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEvent.Type type = 1;
* @return The type.
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEvent.Type getType() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Commanding.CommandQueueEvent.Type result = org.yamcs.protobuf.Commanding.CommandQueueEvent.Type.valueOf(type_);
return result == null ? org.yamcs.protobuf.Commanding.CommandQueueEvent.Type.COMMAND_ADDED : result;
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEvent.Type type = 1;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.yamcs.protobuf.Commanding.CommandQueueEvent.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEvent.Type type = 1;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 1;
onChanged();
return this;
}
private org.yamcs.protobuf.Commanding.CommandQueueEntry data_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueEntry, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder, org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder> dataBuilder_;
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
* @return Whether the data field is set.
*/
public boolean hasData() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
* @return The data.
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntry getData() {
if (dataBuilder_ == null) {
return data_ == null ? org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance() : data_;
} else {
return dataBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
*/
public Builder setData(org.yamcs.protobuf.Commanding.CommandQueueEntry value) {
if (dataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
dataBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
*/
public Builder setData(
org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder builderForValue) {
if (dataBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
dataBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
*/
public Builder mergeData(org.yamcs.protobuf.Commanding.CommandQueueEntry value) {
if (dataBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
data_ != null &&
data_ != org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance()) {
data_ =
org.yamcs.protobuf.Commanding.CommandQueueEntry.newBuilder(data_).mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
dataBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
*/
public Builder clearData() {
if (dataBuilder_ == null) {
data_ = null;
onChanged();
} else {
dataBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder getDataBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getDataFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder getDataOrBuilder() {
if (dataBuilder_ != null) {
return dataBuilder_.getMessageOrBuilder();
} else {
return data_ == null ?
org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance() : data_;
}
}
/**
* optional .yamcs.protobuf.commanding.CommandQueueEntry data = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueEntry, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder, org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder>
getDataFieldBuilder() {
if (dataBuilder_ == null) {
dataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueEntry, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder, org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder>(
getData(),
getParentForChildren(),
isClean());
data_ = null;
}
return dataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.CommandQueueEvent)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.CommandQueueEvent)
private static final org.yamcs.protobuf.Commanding.CommandQueueEvent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.CommandQueueEvent();
}
public static org.yamcs.protobuf.Commanding.CommandQueueEvent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandQueueEvent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandQueueEvent(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEvent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandQueueRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.CommandQueueRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
* @return Whether the queueInfo field is set.
*/
boolean hasQueueInfo();
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
* @return The queueInfo.
*/
org.yamcs.protobuf.Commanding.CommandQueueInfo getQueueInfo();
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
*/
org.yamcs.protobuf.Commanding.CommandQueueInfoOrBuilder getQueueInfoOrBuilder();
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
* @return Whether the queueEntry field is set.
*/
boolean hasQueueEntry();
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
* @return The queueEntry.
*/
org.yamcs.protobuf.Commanding.CommandQueueEntry getQueueEntry();
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
*/
org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder getQueueEntryOrBuilder();
/**
*
*if rebuild is true, the binary packet will be recreated to include new time and sequence count
*
*
* optional bool rebuild = 3 [default = false];
* @return Whether the rebuild field is set.
*/
boolean hasRebuild();
/**
*
*if rebuild is true, the binary packet will be recreated to include new time and sequence count
*
*
* optional bool rebuild = 3 [default = false];
* @return The rebuild.
*/
boolean getRebuild();
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandQueueRequest}
*/
public static final class CommandQueueRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.CommandQueueRequest)
CommandQueueRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandQueueRequest.newBuilder() to construct.
private CommandQueueRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandQueueRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandQueueRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandQueueRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.yamcs.protobuf.Commanding.CommandQueueInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = queueInfo_.toBuilder();
}
queueInfo_ = input.readMessage(org.yamcs.protobuf.Commanding.CommandQueueInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(queueInfo_);
queueInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = queueEntry_.toBuilder();
}
queueEntry_ = input.readMessage(org.yamcs.protobuf.Commanding.CommandQueueEntry.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(queueEntry_);
queueEntry_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 24: {
bitField0_ |= 0x00000004;
rebuild_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandQueueRequest.class, org.yamcs.protobuf.Commanding.CommandQueueRequest.Builder.class);
}
private int bitField0_;
public static final int QUEUEINFO_FIELD_NUMBER = 1;
private org.yamcs.protobuf.Commanding.CommandQueueInfo queueInfo_;
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
* @return Whether the queueInfo field is set.
*/
@java.lang.Override
public boolean hasQueueInfo() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
* @return The queueInfo.
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueInfo getQueueInfo() {
return queueInfo_ == null ? org.yamcs.protobuf.Commanding.CommandQueueInfo.getDefaultInstance() : queueInfo_;
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueInfoOrBuilder getQueueInfoOrBuilder() {
return queueInfo_ == null ? org.yamcs.protobuf.Commanding.CommandQueueInfo.getDefaultInstance() : queueInfo_;
}
public static final int QUEUEENTRY_FIELD_NUMBER = 2;
private org.yamcs.protobuf.Commanding.CommandQueueEntry queueEntry_;
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
* @return Whether the queueEntry field is set.
*/
@java.lang.Override
public boolean hasQueueEntry() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
* @return The queueEntry.
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntry getQueueEntry() {
return queueEntry_ == null ? org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance() : queueEntry_;
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder getQueueEntryOrBuilder() {
return queueEntry_ == null ? org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance() : queueEntry_;
}
public static final int REBUILD_FIELD_NUMBER = 3;
private boolean rebuild_;
/**
*
*if rebuild is true, the binary packet will be recreated to include new time and sequence count
*
*
* optional bool rebuild = 3 [default = false];
* @return Whether the rebuild field is set.
*/
@java.lang.Override
public boolean hasRebuild() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*if rebuild is true, the binary packet will be recreated to include new time and sequence count
*
*
* optional bool rebuild = 3 [default = false];
* @return The rebuild.
*/
@java.lang.Override
public boolean getRebuild() {
return rebuild_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasQueueInfo()) {
if (!getQueueInfo().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasQueueEntry()) {
if (!getQueueEntry().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getQueueInfo());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getQueueEntry());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(3, rebuild_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getQueueInfo());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getQueueEntry());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, rebuild_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.CommandQueueRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.CommandQueueRequest other = (org.yamcs.protobuf.Commanding.CommandQueueRequest) obj;
if (hasQueueInfo() != other.hasQueueInfo()) return false;
if (hasQueueInfo()) {
if (!getQueueInfo()
.equals(other.getQueueInfo())) return false;
}
if (hasQueueEntry() != other.hasQueueEntry()) return false;
if (hasQueueEntry()) {
if (!getQueueEntry()
.equals(other.getQueueEntry())) return false;
}
if (hasRebuild() != other.hasRebuild()) return false;
if (hasRebuild()) {
if (getRebuild()
!= other.getRebuild()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasQueueInfo()) {
hash = (37 * hash) + QUEUEINFO_FIELD_NUMBER;
hash = (53 * hash) + getQueueInfo().hashCode();
}
if (hasQueueEntry()) {
hash = (37 * hash) + QUEUEENTRY_FIELD_NUMBER;
hash = (53 * hash) + getQueueEntry().hashCode();
}
if (hasRebuild()) {
hash = (37 * hash) + REBUILD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRebuild());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.CommandQueueRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandQueueRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.CommandQueueRequest)
org.yamcs.protobuf.Commanding.CommandQueueRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandQueueRequest.class, org.yamcs.protobuf.Commanding.CommandQueueRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.CommandQueueRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getQueueInfoFieldBuilder();
getQueueEntryFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (queueInfoBuilder_ == null) {
queueInfo_ = null;
} else {
queueInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (queueEntryBuilder_ == null) {
queueEntry_ = null;
} else {
queueEntryBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
rebuild_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandQueueRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.CommandQueueRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueRequest build() {
org.yamcs.protobuf.Commanding.CommandQueueRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueRequest buildPartial() {
org.yamcs.protobuf.Commanding.CommandQueueRequest result = new org.yamcs.protobuf.Commanding.CommandQueueRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
if (queueInfoBuilder_ == null) {
result.queueInfo_ = queueInfo_;
} else {
result.queueInfo_ = queueInfoBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
if (queueEntryBuilder_ == null) {
result.queueEntry_ = queueEntry_;
} else {
result.queueEntry_ = queueEntryBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.rebuild_ = rebuild_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.CommandQueueRequest) {
return mergeFrom((org.yamcs.protobuf.Commanding.CommandQueueRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.CommandQueueRequest other) {
if (other == org.yamcs.protobuf.Commanding.CommandQueueRequest.getDefaultInstance()) return this;
if (other.hasQueueInfo()) {
mergeQueueInfo(other.getQueueInfo());
}
if (other.hasQueueEntry()) {
mergeQueueEntry(other.getQueueEntry());
}
if (other.hasRebuild()) {
setRebuild(other.getRebuild());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasQueueInfo()) {
if (!getQueueInfo().isInitialized()) {
return false;
}
}
if (hasQueueEntry()) {
if (!getQueueEntry().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.CommandQueueRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.CommandQueueRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.yamcs.protobuf.Commanding.CommandQueueInfo queueInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueInfo, org.yamcs.protobuf.Commanding.CommandQueueInfo.Builder, org.yamcs.protobuf.Commanding.CommandQueueInfoOrBuilder> queueInfoBuilder_;
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
* @return Whether the queueInfo field is set.
*/
public boolean hasQueueInfo() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
* @return The queueInfo.
*/
public org.yamcs.protobuf.Commanding.CommandQueueInfo getQueueInfo() {
if (queueInfoBuilder_ == null) {
return queueInfo_ == null ? org.yamcs.protobuf.Commanding.CommandQueueInfo.getDefaultInstance() : queueInfo_;
} else {
return queueInfoBuilder_.getMessage();
}
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
*/
public Builder setQueueInfo(org.yamcs.protobuf.Commanding.CommandQueueInfo value) {
if (queueInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
queueInfo_ = value;
onChanged();
} else {
queueInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
*/
public Builder setQueueInfo(
org.yamcs.protobuf.Commanding.CommandQueueInfo.Builder builderForValue) {
if (queueInfoBuilder_ == null) {
queueInfo_ = builderForValue.build();
onChanged();
} else {
queueInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
*/
public Builder mergeQueueInfo(org.yamcs.protobuf.Commanding.CommandQueueInfo value) {
if (queueInfoBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
queueInfo_ != null &&
queueInfo_ != org.yamcs.protobuf.Commanding.CommandQueueInfo.getDefaultInstance()) {
queueInfo_ =
org.yamcs.protobuf.Commanding.CommandQueueInfo.newBuilder(queueInfo_).mergeFrom(value).buildPartial();
} else {
queueInfo_ = value;
}
onChanged();
} else {
queueInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
*/
public Builder clearQueueInfo() {
if (queueInfoBuilder_ == null) {
queueInfo_ = null;
onChanged();
} else {
queueInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
*/
public org.yamcs.protobuf.Commanding.CommandQueueInfo.Builder getQueueInfoBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getQueueInfoFieldBuilder().getBuilder();
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
*/
public org.yamcs.protobuf.Commanding.CommandQueueInfoOrBuilder getQueueInfoOrBuilder() {
if (queueInfoBuilder_ != null) {
return queueInfoBuilder_.getMessageOrBuilder();
} else {
return queueInfo_ == null ?
org.yamcs.protobuf.Commanding.CommandQueueInfo.getDefaultInstance() : queueInfo_;
}
}
/**
*
* for SetQueueState
*
*
* optional .yamcs.protobuf.commanding.CommandQueueInfo queueInfo = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueInfo, org.yamcs.protobuf.Commanding.CommandQueueInfo.Builder, org.yamcs.protobuf.Commanding.CommandQueueInfoOrBuilder>
getQueueInfoFieldBuilder() {
if (queueInfoBuilder_ == null) {
queueInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueInfo, org.yamcs.protobuf.Commanding.CommandQueueInfo.Builder, org.yamcs.protobuf.Commanding.CommandQueueInfoOrBuilder>(
getQueueInfo(),
getParentForChildren(),
isClean());
queueInfo_ = null;
}
return queueInfoBuilder_;
}
private org.yamcs.protobuf.Commanding.CommandQueueEntry queueEntry_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueEntry, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder, org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder> queueEntryBuilder_;
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
* @return Whether the queueEntry field is set.
*/
public boolean hasQueueEntry() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
* @return The queueEntry.
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntry getQueueEntry() {
if (queueEntryBuilder_ == null) {
return queueEntry_ == null ? org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance() : queueEntry_;
} else {
return queueEntryBuilder_.getMessage();
}
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
*/
public Builder setQueueEntry(org.yamcs.protobuf.Commanding.CommandQueueEntry value) {
if (queueEntryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
queueEntry_ = value;
onChanged();
} else {
queueEntryBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
*/
public Builder setQueueEntry(
org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder builderForValue) {
if (queueEntryBuilder_ == null) {
queueEntry_ = builderForValue.build();
onChanged();
} else {
queueEntryBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
*/
public Builder mergeQueueEntry(org.yamcs.protobuf.Commanding.CommandQueueEntry value) {
if (queueEntryBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
queueEntry_ != null &&
queueEntry_ != org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance()) {
queueEntry_ =
org.yamcs.protobuf.Commanding.CommandQueueEntry.newBuilder(queueEntry_).mergeFrom(value).buildPartial();
} else {
queueEntry_ = value;
}
onChanged();
} else {
queueEntryBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
*/
public Builder clearQueueEntry() {
if (queueEntryBuilder_ == null) {
queueEntry_ = null;
onChanged();
} else {
queueEntryBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder getQueueEntryBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getQueueEntryFieldBuilder().getBuilder();
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
*/
public org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder getQueueEntryOrBuilder() {
if (queueEntryBuilder_ != null) {
return queueEntryBuilder_.getMessageOrBuilder();
} else {
return queueEntry_ == null ?
org.yamcs.protobuf.Commanding.CommandQueueEntry.getDefaultInstance() : queueEntry_;
}
}
/**
*
*for SendCommand and RejectCommand
*
*
* optional .yamcs.protobuf.commanding.CommandQueueEntry queueEntry = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueEntry, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder, org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder>
getQueueEntryFieldBuilder() {
if (queueEntryBuilder_ == null) {
queueEntryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandQueueEntry, org.yamcs.protobuf.Commanding.CommandQueueEntry.Builder, org.yamcs.protobuf.Commanding.CommandQueueEntryOrBuilder>(
getQueueEntry(),
getParentForChildren(),
isClean());
queueEntry_ = null;
}
return queueEntryBuilder_;
}
private boolean rebuild_ ;
/**
*
*if rebuild is true, the binary packet will be recreated to include new time and sequence count
*
*
* optional bool rebuild = 3 [default = false];
* @return Whether the rebuild field is set.
*/
@java.lang.Override
public boolean hasRebuild() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*if rebuild is true, the binary packet will be recreated to include new time and sequence count
*
*
* optional bool rebuild = 3 [default = false];
* @return The rebuild.
*/
@java.lang.Override
public boolean getRebuild() {
return rebuild_;
}
/**
*
*if rebuild is true, the binary packet will be recreated to include new time and sequence count
*
*
* optional bool rebuild = 3 [default = false];
* @param value The rebuild to set.
* @return This builder for chaining.
*/
public Builder setRebuild(boolean value) {
bitField0_ |= 0x00000004;
rebuild_ = value;
onChanged();
return this;
}
/**
*
*if rebuild is true, the binary packet will be recreated to include new time and sequence count
*
*
* optional bool rebuild = 3 [default = false];
* @return This builder for chaining.
*/
public Builder clearRebuild() {
bitField0_ = (bitField0_ & ~0x00000004);
rebuild_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.CommandQueueRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.CommandQueueRequest)
private static final org.yamcs.protobuf.Commanding.CommandQueueRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.CommandQueueRequest();
}
public static org.yamcs.protobuf.Commanding.CommandQueueRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandQueueRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandQueueRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandQueueRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandSignificanceOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.CommandSignificance)
com.google.protobuf.MessageOrBuilder {
/**
*
*the sequence number of the command sent
*
*
* optional int32 sequenceNumber = 1;
* @return Whether the sequenceNumber field is set.
*/
boolean hasSequenceNumber();
/**
*
*the sequence number of the command sent
*
*
* optional int32 sequenceNumber = 1;
* @return The sequenceNumber.
*/
int getSequenceNumber();
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
* @return Whether the significance field is set.
*/
boolean hasSignificance();
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
* @return The significance.
*/
org.yamcs.protobuf.Mdb.SignificanceInfo getSignificance();
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
*/
org.yamcs.protobuf.Mdb.SignificanceInfoOrBuilder getSignificanceOrBuilder();
}
/**
*
* this message is sent as response to validate, in case the significance is defined for a commands
*
*
* Protobuf type {@code yamcs.protobuf.commanding.CommandSignificance}
*/
public static final class CommandSignificance extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.CommandSignificance)
CommandSignificanceOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandSignificance.newBuilder() to construct.
private CommandSignificance(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandSignificance() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandSignificance();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandSignificance(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
sequenceNumber_ = input.readInt32();
break;
}
case 18: {
org.yamcs.protobuf.Mdb.SignificanceInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = significance_.toBuilder();
}
significance_ = input.readMessage(org.yamcs.protobuf.Mdb.SignificanceInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(significance_);
significance_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandSignificance_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandSignificance_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandSignificance.class, org.yamcs.protobuf.Commanding.CommandSignificance.Builder.class);
}
private int bitField0_;
public static final int SEQUENCENUMBER_FIELD_NUMBER = 1;
private int sequenceNumber_;
/**
*
*the sequence number of the command sent
*
*
* optional int32 sequenceNumber = 1;
* @return Whether the sequenceNumber field is set.
*/
@java.lang.Override
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*the sequence number of the command sent
*
*
* optional int32 sequenceNumber = 1;
* @return The sequenceNumber.
*/
@java.lang.Override
public int getSequenceNumber() {
return sequenceNumber_;
}
public static final int SIGNIFICANCE_FIELD_NUMBER = 2;
private org.yamcs.protobuf.Mdb.SignificanceInfo significance_;
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
* @return Whether the significance field is set.
*/
@java.lang.Override
public boolean hasSignificance() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
* @return The significance.
*/
@java.lang.Override
public org.yamcs.protobuf.Mdb.SignificanceInfo getSignificance() {
return significance_ == null ? org.yamcs.protobuf.Mdb.SignificanceInfo.getDefaultInstance() : significance_;
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Mdb.SignificanceInfoOrBuilder getSignificanceOrBuilder() {
return significance_ == null ? org.yamcs.protobuf.Mdb.SignificanceInfo.getDefaultInstance() : significance_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, sequenceNumber_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getSignificance());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, sequenceNumber_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getSignificance());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.CommandSignificance)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.CommandSignificance other = (org.yamcs.protobuf.Commanding.CommandSignificance) obj;
if (hasSequenceNumber() != other.hasSequenceNumber()) return false;
if (hasSequenceNumber()) {
if (getSequenceNumber()
!= other.getSequenceNumber()) return false;
}
if (hasSignificance() != other.hasSignificance()) return false;
if (hasSignificance()) {
if (!getSignificance()
.equals(other.getSignificance())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSequenceNumber()) {
hash = (37 * hash) + SEQUENCENUMBER_FIELD_NUMBER;
hash = (53 * hash) + getSequenceNumber();
}
if (hasSignificance()) {
hash = (37 * hash) + SIGNIFICANCE_FIELD_NUMBER;
hash = (53 * hash) + getSignificance().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandSignificance parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.CommandSignificance prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* this message is sent as response to validate, in case the significance is defined for a commands
*
*
* Protobuf type {@code yamcs.protobuf.commanding.CommandSignificance}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.CommandSignificance)
org.yamcs.protobuf.Commanding.CommandSignificanceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandSignificance_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandSignificance_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandSignificance.class, org.yamcs.protobuf.Commanding.CommandSignificance.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.CommandSignificance.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getSignificanceFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
sequenceNumber_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
if (significanceBuilder_ == null) {
significance_ = null;
} else {
significanceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandSignificance_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandSignificance getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.CommandSignificance.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandSignificance build() {
org.yamcs.protobuf.Commanding.CommandSignificance result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandSignificance buildPartial() {
org.yamcs.protobuf.Commanding.CommandSignificance result = new org.yamcs.protobuf.Commanding.CommandSignificance(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.sequenceNumber_ = sequenceNumber_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
if (significanceBuilder_ == null) {
result.significance_ = significance_;
} else {
result.significance_ = significanceBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.CommandSignificance) {
return mergeFrom((org.yamcs.protobuf.Commanding.CommandSignificance)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.CommandSignificance other) {
if (other == org.yamcs.protobuf.Commanding.CommandSignificance.getDefaultInstance()) return this;
if (other.hasSequenceNumber()) {
setSequenceNumber(other.getSequenceNumber());
}
if (other.hasSignificance()) {
mergeSignificance(other.getSignificance());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.CommandSignificance parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.CommandSignificance) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int sequenceNumber_ ;
/**
*
*the sequence number of the command sent
*
*
* optional int32 sequenceNumber = 1;
* @return Whether the sequenceNumber field is set.
*/
@java.lang.Override
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*the sequence number of the command sent
*
*
* optional int32 sequenceNumber = 1;
* @return The sequenceNumber.
*/
@java.lang.Override
public int getSequenceNumber() {
return sequenceNumber_;
}
/**
*
*the sequence number of the command sent
*
*
* optional int32 sequenceNumber = 1;
* @param value The sequenceNumber to set.
* @return This builder for chaining.
*/
public Builder setSequenceNumber(int value) {
bitField0_ |= 0x00000001;
sequenceNumber_ = value;
onChanged();
return this;
}
/**
*
*the sequence number of the command sent
*
*
* optional int32 sequenceNumber = 1;
* @return This builder for chaining.
*/
public Builder clearSequenceNumber() {
bitField0_ = (bitField0_ & ~0x00000001);
sequenceNumber_ = 0;
onChanged();
return this;
}
private org.yamcs.protobuf.Mdb.SignificanceInfo significance_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Mdb.SignificanceInfo, org.yamcs.protobuf.Mdb.SignificanceInfo.Builder, org.yamcs.protobuf.Mdb.SignificanceInfoOrBuilder> significanceBuilder_;
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
* @return Whether the significance field is set.
*/
public boolean hasSignificance() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
* @return The significance.
*/
public org.yamcs.protobuf.Mdb.SignificanceInfo getSignificance() {
if (significanceBuilder_ == null) {
return significance_ == null ? org.yamcs.protobuf.Mdb.SignificanceInfo.getDefaultInstance() : significance_;
} else {
return significanceBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
*/
public Builder setSignificance(org.yamcs.protobuf.Mdb.SignificanceInfo value) {
if (significanceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
significance_ = value;
onChanged();
} else {
significanceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
*/
public Builder setSignificance(
org.yamcs.protobuf.Mdb.SignificanceInfo.Builder builderForValue) {
if (significanceBuilder_ == null) {
significance_ = builderForValue.build();
onChanged();
} else {
significanceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
*/
public Builder mergeSignificance(org.yamcs.protobuf.Mdb.SignificanceInfo value) {
if (significanceBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
significance_ != null &&
significance_ != org.yamcs.protobuf.Mdb.SignificanceInfo.getDefaultInstance()) {
significance_ =
org.yamcs.protobuf.Mdb.SignificanceInfo.newBuilder(significance_).mergeFrom(value).buildPartial();
} else {
significance_ = value;
}
onChanged();
} else {
significanceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
*/
public Builder clearSignificance() {
if (significanceBuilder_ == null) {
significance_ = null;
onChanged();
} else {
significanceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
*/
public org.yamcs.protobuf.Mdb.SignificanceInfo.Builder getSignificanceBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getSignificanceFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
*/
public org.yamcs.protobuf.Mdb.SignificanceInfoOrBuilder getSignificanceOrBuilder() {
if (significanceBuilder_ != null) {
return significanceBuilder_.getMessageOrBuilder();
} else {
return significance_ == null ?
org.yamcs.protobuf.Mdb.SignificanceInfo.getDefaultInstance() : significance_;
}
}
/**
* optional .yamcs.protobuf.mdb.SignificanceInfo significance = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Mdb.SignificanceInfo, org.yamcs.protobuf.Mdb.SignificanceInfo.Builder, org.yamcs.protobuf.Mdb.SignificanceInfoOrBuilder>
getSignificanceFieldBuilder() {
if (significanceBuilder_ == null) {
significanceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Mdb.SignificanceInfo, org.yamcs.protobuf.Mdb.SignificanceInfo.Builder, org.yamcs.protobuf.Mdb.SignificanceInfoOrBuilder>(
getSignificance(),
getParentForChildren(),
isClean());
significance_ = null;
}
return significanceBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.CommandSignificance)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.CommandSignificance)
private static final org.yamcs.protobuf.Commanding.CommandSignificance DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.CommandSignificance();
}
public static org.yamcs.protobuf.Commanding.CommandSignificance getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandSignificance parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandSignificance(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandSignificance getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VerifierConfigOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.VerifierConfig)
com.google.protobuf.MessageOrBuilder {
/**
*
*disable the verifier
*
*
* optional bool disable = 2;
* @return Whether the disable field is set.
*/
boolean hasDisable();
/**
*
*disable the verifier
*
*
* optional bool disable = 2;
* @return The disable.
*/
boolean getDisable();
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
* @return Whether the checkWindow field is set.
*/
boolean hasCheckWindow();
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
* @return The checkWindow.
*/
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow getCheckWindow();
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
*/
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindowOrBuilder getCheckWindowOrBuilder();
}
/**
*
*can be used when sending commands to affect the way post transmission verifiers are running
*
*
* Protobuf type {@code yamcs.protobuf.commanding.VerifierConfig}
*/
public static final class VerifierConfig extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.VerifierConfig)
VerifierConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use VerifierConfig.newBuilder() to construct.
private VerifierConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VerifierConfig() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new VerifierConfig();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private VerifierConfig(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 16: {
bitField0_ |= 0x00000001;
disable_ = input.readBool();
break;
}
case 26: {
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = checkWindow_.toBuilder();
}
checkWindow_ = input.readMessage(org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(checkWindow_);
checkWindow_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.VerifierConfig.class, org.yamcs.protobuf.Commanding.VerifierConfig.Builder.class);
}
public interface CheckWindowOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.VerifierConfig.CheckWindow)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 timeToStartChecking = 1;
* @return Whether the timeToStartChecking field is set.
*/
boolean hasTimeToStartChecking();
/**
* optional int64 timeToStartChecking = 1;
* @return The timeToStartChecking.
*/
long getTimeToStartChecking();
/**
* optional int64 timeToStopChecking = 2;
* @return Whether the timeToStopChecking field is set.
*/
boolean hasTimeToStopChecking();
/**
* optional int64 timeToStopChecking = 2;
* @return The timeToStopChecking.
*/
long getTimeToStopChecking();
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.VerifierConfig.CheckWindow}
*/
public static final class CheckWindow extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.VerifierConfig.CheckWindow)
CheckWindowOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckWindow.newBuilder() to construct.
private CheckWindow(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckWindow() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CheckWindow();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CheckWindow(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
timeToStartChecking_ = input.readInt64();
break;
}
case 16: {
bitField0_ |= 0x00000002;
timeToStopChecking_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.class, org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.Builder.class);
}
private int bitField0_;
public static final int TIMETOSTARTCHECKING_FIELD_NUMBER = 1;
private long timeToStartChecking_;
/**
* optional int64 timeToStartChecking = 1;
* @return Whether the timeToStartChecking field is set.
*/
@java.lang.Override
public boolean hasTimeToStartChecking() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int64 timeToStartChecking = 1;
* @return The timeToStartChecking.
*/
@java.lang.Override
public long getTimeToStartChecking() {
return timeToStartChecking_;
}
public static final int TIMETOSTOPCHECKING_FIELD_NUMBER = 2;
private long timeToStopChecking_;
/**
* optional int64 timeToStopChecking = 2;
* @return Whether the timeToStopChecking field is set.
*/
@java.lang.Override
public boolean hasTimeToStopChecking() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 timeToStopChecking = 2;
* @return The timeToStopChecking.
*/
@java.lang.Override
public long getTimeToStopChecking() {
return timeToStopChecking_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, timeToStartChecking_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, timeToStopChecking_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, timeToStartChecking_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, timeToStopChecking_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow other = (org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow) obj;
if (hasTimeToStartChecking() != other.hasTimeToStartChecking()) return false;
if (hasTimeToStartChecking()) {
if (getTimeToStartChecking()
!= other.getTimeToStartChecking()) return false;
}
if (hasTimeToStopChecking() != other.hasTimeToStopChecking()) return false;
if (hasTimeToStopChecking()) {
if (getTimeToStopChecking()
!= other.getTimeToStopChecking()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTimeToStartChecking()) {
hash = (37 * hash) + TIMETOSTARTCHECKING_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimeToStartChecking());
}
if (hasTimeToStopChecking()) {
hash = (37 * hash) + TIMETOSTOPCHECKING_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimeToStopChecking());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.VerifierConfig.CheckWindow}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.VerifierConfig.CheckWindow)
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindowOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.class, org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
timeToStartChecking_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
timeToStopChecking_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow build() {
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow buildPartial() {
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow result = new org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.timeToStartChecking_ = timeToStartChecking_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.timeToStopChecking_ = timeToStopChecking_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow) {
return mergeFrom((org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow other) {
if (other == org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.getDefaultInstance()) return this;
if (other.hasTimeToStartChecking()) {
setTimeToStartChecking(other.getTimeToStartChecking());
}
if (other.hasTimeToStopChecking()) {
setTimeToStopChecking(other.getTimeToStopChecking());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long timeToStartChecking_ ;
/**
* optional int64 timeToStartChecking = 1;
* @return Whether the timeToStartChecking field is set.
*/
@java.lang.Override
public boolean hasTimeToStartChecking() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int64 timeToStartChecking = 1;
* @return The timeToStartChecking.
*/
@java.lang.Override
public long getTimeToStartChecking() {
return timeToStartChecking_;
}
/**
* optional int64 timeToStartChecking = 1;
* @param value The timeToStartChecking to set.
* @return This builder for chaining.
*/
public Builder setTimeToStartChecking(long value) {
bitField0_ |= 0x00000001;
timeToStartChecking_ = value;
onChanged();
return this;
}
/**
* optional int64 timeToStartChecking = 1;
* @return This builder for chaining.
*/
public Builder clearTimeToStartChecking() {
bitField0_ = (bitField0_ & ~0x00000001);
timeToStartChecking_ = 0L;
onChanged();
return this;
}
private long timeToStopChecking_ ;
/**
* optional int64 timeToStopChecking = 2;
* @return Whether the timeToStopChecking field is set.
*/
@java.lang.Override
public boolean hasTimeToStopChecking() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 timeToStopChecking = 2;
* @return The timeToStopChecking.
*/
@java.lang.Override
public long getTimeToStopChecking() {
return timeToStopChecking_;
}
/**
* optional int64 timeToStopChecking = 2;
* @param value The timeToStopChecking to set.
* @return This builder for chaining.
*/
public Builder setTimeToStopChecking(long value) {
bitField0_ |= 0x00000002;
timeToStopChecking_ = value;
onChanged();
return this;
}
/**
* optional int64 timeToStopChecking = 2;
* @return This builder for chaining.
*/
public Builder clearTimeToStopChecking() {
bitField0_ = (bitField0_ & ~0x00000002);
timeToStopChecking_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.VerifierConfig.CheckWindow)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.VerifierConfig.CheckWindow)
private static final org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow();
}
public static org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CheckWindow parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CheckWindow(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int DISABLE_FIELD_NUMBER = 2;
private boolean disable_;
/**
*
*disable the verifier
*
*
* optional bool disable = 2;
* @return Whether the disable field is set.
*/
@java.lang.Override
public boolean hasDisable() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*disable the verifier
*
*
* optional bool disable = 2;
* @return The disable.
*/
@java.lang.Override
public boolean getDisable() {
return disable_;
}
public static final int CHECKWINDOW_FIELD_NUMBER = 3;
private org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow checkWindow_;
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
* @return Whether the checkWindow field is set.
*/
@java.lang.Override
public boolean hasCheckWindow() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
* @return The checkWindow.
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow getCheckWindow() {
return checkWindow_ == null ? org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.getDefaultInstance() : checkWindow_;
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindowOrBuilder getCheckWindowOrBuilder() {
return checkWindow_ == null ? org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.getDefaultInstance() : checkWindow_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBool(2, disable_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getCheckWindow());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, disable_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getCheckWindow());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.VerifierConfig)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.VerifierConfig other = (org.yamcs.protobuf.Commanding.VerifierConfig) obj;
if (hasDisable() != other.hasDisable()) return false;
if (hasDisable()) {
if (getDisable()
!= other.getDisable()) return false;
}
if (hasCheckWindow() != other.hasCheckWindow()) return false;
if (hasCheckWindow()) {
if (!getCheckWindow()
.equals(other.getCheckWindow())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDisable()) {
hash = (37 * hash) + DISABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDisable());
}
if (hasCheckWindow()) {
hash = (37 * hash) + CHECKWINDOW_FIELD_NUMBER;
hash = (53 * hash) + getCheckWindow().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.VerifierConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.VerifierConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*can be used when sending commands to affect the way post transmission verifiers are running
*
*
* Protobuf type {@code yamcs.protobuf.commanding.VerifierConfig}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.VerifierConfig)
org.yamcs.protobuf.Commanding.VerifierConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.VerifierConfig.class, org.yamcs.protobuf.Commanding.VerifierConfig.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.VerifierConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCheckWindowFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
disable_ = false;
bitField0_ = (bitField0_ & ~0x00000001);
if (checkWindowBuilder_ == null) {
checkWindow_ = null;
} else {
checkWindowBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_VerifierConfig_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.VerifierConfig.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig build() {
org.yamcs.protobuf.Commanding.VerifierConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig buildPartial() {
org.yamcs.protobuf.Commanding.VerifierConfig result = new org.yamcs.protobuf.Commanding.VerifierConfig(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.disable_ = disable_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
if (checkWindowBuilder_ == null) {
result.checkWindow_ = checkWindow_;
} else {
result.checkWindow_ = checkWindowBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.VerifierConfig) {
return mergeFrom((org.yamcs.protobuf.Commanding.VerifierConfig)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.VerifierConfig other) {
if (other == org.yamcs.protobuf.Commanding.VerifierConfig.getDefaultInstance()) return this;
if (other.hasDisable()) {
setDisable(other.getDisable());
}
if (other.hasCheckWindow()) {
mergeCheckWindow(other.getCheckWindow());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.VerifierConfig parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.VerifierConfig) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private boolean disable_ ;
/**
*
*disable the verifier
*
*
* optional bool disable = 2;
* @return Whether the disable field is set.
*/
@java.lang.Override
public boolean hasDisable() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*disable the verifier
*
*
* optional bool disable = 2;
* @return The disable.
*/
@java.lang.Override
public boolean getDisable() {
return disable_;
}
/**
*
*disable the verifier
*
*
* optional bool disable = 2;
* @param value The disable to set.
* @return This builder for chaining.
*/
public Builder setDisable(boolean value) {
bitField0_ |= 0x00000001;
disable_ = value;
onChanged();
return this;
}
/**
*
*disable the verifier
*
*
* optional bool disable = 2;
* @return This builder for chaining.
*/
public Builder clearDisable() {
bitField0_ = (bitField0_ & ~0x00000001);
disable_ = false;
onChanged();
return this;
}
private org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow checkWindow_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow, org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.Builder, org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindowOrBuilder> checkWindowBuilder_;
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
* @return Whether the checkWindow field is set.
*/
public boolean hasCheckWindow() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
* @return The checkWindow.
*/
public org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow getCheckWindow() {
if (checkWindowBuilder_ == null) {
return checkWindow_ == null ? org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.getDefaultInstance() : checkWindow_;
} else {
return checkWindowBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
*/
public Builder setCheckWindow(org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow value) {
if (checkWindowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
checkWindow_ = value;
onChanged();
} else {
checkWindowBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
*/
public Builder setCheckWindow(
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.Builder builderForValue) {
if (checkWindowBuilder_ == null) {
checkWindow_ = builderForValue.build();
onChanged();
} else {
checkWindowBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
*/
public Builder mergeCheckWindow(org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow value) {
if (checkWindowBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
checkWindow_ != null &&
checkWindow_ != org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.getDefaultInstance()) {
checkWindow_ =
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.newBuilder(checkWindow_).mergeFrom(value).buildPartial();
} else {
checkWindow_ = value;
}
onChanged();
} else {
checkWindowBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
*/
public Builder clearCheckWindow() {
if (checkWindowBuilder_ == null) {
checkWindow_ = null;
onChanged();
} else {
checkWindowBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
*/
public org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.Builder getCheckWindowBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getCheckWindowFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
*/
public org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindowOrBuilder getCheckWindowOrBuilder() {
if (checkWindowBuilder_ != null) {
return checkWindowBuilder_.getMessageOrBuilder();
} else {
return checkWindow_ == null ?
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.getDefaultInstance() : checkWindow_;
}
}
/**
* optional .yamcs.protobuf.commanding.VerifierConfig.CheckWindow checkWindow = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow, org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.Builder, org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindowOrBuilder>
getCheckWindowFieldBuilder() {
if (checkWindowBuilder_ == null) {
checkWindowBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow, org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindow.Builder, org.yamcs.protobuf.Commanding.VerifierConfig.CheckWindowOrBuilder>(
getCheckWindow(),
getParentForChildren(),
isClean());
checkWindow_ = null;
}
return checkWindowBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.VerifierConfig)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.VerifierConfig)
private static final org.yamcs.protobuf.Commanding.VerifierConfig DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.VerifierConfig();
}
public static org.yamcs.protobuf.Commanding.VerifierConfig getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VerifierConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new VerifierConfig(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.VerifierConfig getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandHistoryAttributeOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.CommandHistoryAttribute)
com.google.protobuf.MessageOrBuilder {
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional .yamcs.protobuf.Value value = 2;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* optional .yamcs.protobuf.Value value = 2;
* @return The value.
*/
org.yamcs.protobuf.Yamcs.Value getValue();
/**
* optional .yamcs.protobuf.Value value = 2;
*/
org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder();
/**
* optional int64 time = 3;
* @return Whether the time field is set.
*/
boolean hasTime();
/**
* optional int64 time = 3;
* @return The time.
*/
long getTime();
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandHistoryAttribute}
*/
public static final class CommandHistoryAttribute extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.CommandHistoryAttribute)
CommandHistoryAttributeOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandHistoryAttribute.newBuilder() to construct.
private CommandHistoryAttribute(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandHistoryAttribute() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandHistoryAttribute();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandHistoryAttribute(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
case 18: {
org.yamcs.protobuf.Yamcs.Value.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = value_.toBuilder();
}
value_ = input.readMessage(org.yamcs.protobuf.Yamcs.Value.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(value_);
value_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 24: {
bitField0_ |= 0x00000004;
time_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandHistoryAttribute.class, org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private org.yamcs.protobuf.Yamcs.Value value_;
/**
* optional .yamcs.protobuf.Value value = 2;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.Value value = 2;
* @return The value.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value getValue() {
return value_ == null ? org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder() {
return value_ == null ? org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
}
public static final int TIME_FIELD_NUMBER = 3;
private long time_;
/**
* optional int64 time = 3;
* @return Whether the time field is set.
*/
@java.lang.Override
public boolean hasTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int64 time = 3;
* @return The time.
*/
@java.lang.Override
public long getTime() {
return time_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasValue()) {
if (!getValue().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getValue());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(3, time_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getValue());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, time_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.CommandHistoryAttribute)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.CommandHistoryAttribute other = (org.yamcs.protobuf.Commanding.CommandHistoryAttribute) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (hasTime() != other.hasTime()) return false;
if (hasTime()) {
if (getTime()
!= other.getTime()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
if (hasTime()) {
hash = (37 * hash) + TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTime());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.CommandHistoryAttribute prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandHistoryAttribute}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.CommandHistoryAttribute)
org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandHistoryAttribute.class, org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.CommandHistoryAttribute.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (valueBuilder_ == null) {
value_ = null;
} else {
valueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
time_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryAttribute getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.CommandHistoryAttribute.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryAttribute build() {
org.yamcs.protobuf.Commanding.CommandHistoryAttribute result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryAttribute buildPartial() {
org.yamcs.protobuf.Commanding.CommandHistoryAttribute result = new org.yamcs.protobuf.Commanding.CommandHistoryAttribute(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) != 0)) {
if (valueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = valueBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.time_ = time_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.CommandHistoryAttribute) {
return mergeFrom((org.yamcs.protobuf.Commanding.CommandHistoryAttribute)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.CommandHistoryAttribute other) {
if (other == org.yamcs.protobuf.Commanding.CommandHistoryAttribute.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
if (other.hasTime()) {
setTime(other.getTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasValue()) {
if (!getValue().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.CommandHistoryAttribute parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.CommandHistoryAttribute) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private org.yamcs.protobuf.Yamcs.Value value_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder> valueBuilder_;
/**
* optional .yamcs.protobuf.Value value = 2;
* @return Whether the value field is set.
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.Value value = 2;
* @return The value.
*/
public org.yamcs.protobuf.Yamcs.Value getValue() {
if (valueBuilder_ == null) {
return value_ == null ? org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
} else {
return valueBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder setValue(org.yamcs.protobuf.Yamcs.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
valueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder setValue(
org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
valueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder mergeValue(org.yamcs.protobuf.Yamcs.Value value) {
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
value_ != null &&
value_ != org.yamcs.protobuf.Yamcs.Value.getDefaultInstance()) {
value_ =
org.yamcs.protobuf.Yamcs.Value.newBuilder(value_).mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
valueBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder clearValue() {
if (valueBuilder_ == null) {
value_ = null;
onChanged();
} else {
valueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder getValueBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getValueFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilder();
} else {
return value_ == null ?
org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
}
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>(
getValue(),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
private long time_ ;
/**
* optional int64 time = 3;
* @return Whether the time field is set.
*/
@java.lang.Override
public boolean hasTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int64 time = 3;
* @return The time.
*/
@java.lang.Override
public long getTime() {
return time_;
}
/**
* optional int64 time = 3;
* @param value The time to set.
* @return This builder for chaining.
*/
public Builder setTime(long value) {
bitField0_ |= 0x00000004;
time_ = value;
onChanged();
return this;
}
/**
* optional int64 time = 3;
* @return This builder for chaining.
*/
public Builder clearTime() {
bitField0_ = (bitField0_ & ~0x00000004);
time_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.CommandHistoryAttribute)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.CommandHistoryAttribute)
private static final org.yamcs.protobuf.Commanding.CommandHistoryAttribute DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.CommandHistoryAttribute();
}
public static org.yamcs.protobuf.Commanding.CommandHistoryAttribute getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandHistoryAttribute parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandHistoryAttribute(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryAttribute getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandAssignmentOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.CommandAssignment)
com.google.protobuf.MessageOrBuilder {
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional .yamcs.protobuf.Value value = 2;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* optional .yamcs.protobuf.Value value = 2;
* @return The value.
*/
org.yamcs.protobuf.Yamcs.Value getValue();
/**
* optional .yamcs.protobuf.Value value = 2;
*/
org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder();
/**
* optional bool userInput = 3;
* @return Whether the userInput field is set.
*/
boolean hasUserInput();
/**
* optional bool userInput = 3;
* @return The userInput.
*/
boolean getUserInput();
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandAssignment}
*/
public static final class CommandAssignment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.CommandAssignment)
CommandAssignmentOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandAssignment.newBuilder() to construct.
private CommandAssignment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandAssignment() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandAssignment();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandAssignment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
case 18: {
org.yamcs.protobuf.Yamcs.Value.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = value_.toBuilder();
}
value_ = input.readMessage(org.yamcs.protobuf.Yamcs.Value.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(value_);
value_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 24: {
bitField0_ |= 0x00000004;
userInput_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandAssignment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandAssignment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandAssignment.class, org.yamcs.protobuf.Commanding.CommandAssignment.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private org.yamcs.protobuf.Yamcs.Value value_;
/**
* optional .yamcs.protobuf.Value value = 2;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.Value value = 2;
* @return The value.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value getValue() {
return value_ == null ? org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder() {
return value_ == null ? org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
}
public static final int USERINPUT_FIELD_NUMBER = 3;
private boolean userInput_;
/**
* optional bool userInput = 3;
* @return Whether the userInput field is set.
*/
@java.lang.Override
public boolean hasUserInput() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bool userInput = 3;
* @return The userInput.
*/
@java.lang.Override
public boolean getUserInput() {
return userInput_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasValue()) {
if (!getValue().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getValue());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(3, userInput_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getValue());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, userInput_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.CommandAssignment)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.CommandAssignment other = (org.yamcs.protobuf.Commanding.CommandAssignment) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (hasUserInput() != other.hasUserInput()) return false;
if (hasUserInput()) {
if (getUserInput()
!= other.getUserInput()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
if (hasUserInput()) {
hash = (37 * hash) + USERINPUT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUserInput());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandAssignment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.CommandAssignment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandAssignment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.CommandAssignment)
org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandAssignment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandAssignment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandAssignment.class, org.yamcs.protobuf.Commanding.CommandAssignment.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.CommandAssignment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (valueBuilder_ == null) {
value_ = null;
} else {
valueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
userInput_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandAssignment_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandAssignment getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.CommandAssignment.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandAssignment build() {
org.yamcs.protobuf.Commanding.CommandAssignment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandAssignment buildPartial() {
org.yamcs.protobuf.Commanding.CommandAssignment result = new org.yamcs.protobuf.Commanding.CommandAssignment(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) != 0)) {
if (valueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = valueBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.userInput_ = userInput_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.CommandAssignment) {
return mergeFrom((org.yamcs.protobuf.Commanding.CommandAssignment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.CommandAssignment other) {
if (other == org.yamcs.protobuf.Commanding.CommandAssignment.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
if (other.hasUserInput()) {
setUserInput(other.getUserInput());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasValue()) {
if (!getValue().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.CommandAssignment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.CommandAssignment) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private org.yamcs.protobuf.Yamcs.Value value_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder> valueBuilder_;
/**
* optional .yamcs.protobuf.Value value = 2;
* @return Whether the value field is set.
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.Value value = 2;
* @return The value.
*/
public org.yamcs.protobuf.Yamcs.Value getValue() {
if (valueBuilder_ == null) {
return value_ == null ? org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
} else {
return valueBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder setValue(org.yamcs.protobuf.Yamcs.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
valueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder setValue(
org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
valueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder mergeValue(org.yamcs.protobuf.Yamcs.Value value) {
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
value_ != null &&
value_ != org.yamcs.protobuf.Yamcs.Value.getDefaultInstance()) {
value_ =
org.yamcs.protobuf.Yamcs.Value.newBuilder(value_).mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
valueBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder clearValue() {
if (valueBuilder_ == null) {
value_ = null;
onChanged();
} else {
valueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder getValueBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getValueFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilder();
} else {
return value_ == null ?
org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
}
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>(
getValue(),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
private boolean userInput_ ;
/**
* optional bool userInput = 3;
* @return Whether the userInput field is set.
*/
@java.lang.Override
public boolean hasUserInput() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bool userInput = 3;
* @return The userInput.
*/
@java.lang.Override
public boolean getUserInput() {
return userInput_;
}
/**
* optional bool userInput = 3;
* @param value The userInput to set.
* @return This builder for chaining.
*/
public Builder setUserInput(boolean value) {
bitField0_ |= 0x00000004;
userInput_ = value;
onChanged();
return this;
}
/**
* optional bool userInput = 3;
* @return This builder for chaining.
*/
public Builder clearUserInput() {
bitField0_ = (bitField0_ & ~0x00000004);
userInput_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.CommandAssignment)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.CommandAssignment)
private static final org.yamcs.protobuf.Commanding.CommandAssignment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.CommandAssignment();
}
public static org.yamcs.protobuf.Commanding.CommandAssignment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandAssignment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandAssignment(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandAssignment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandHistoryEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.CommandHistoryEntry)
com.google.protobuf.MessageOrBuilder {
/**
* optional string id = 7;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* optional string id = 7;
* @return The id.
*/
java.lang.String getId();
/**
* optional string id = 7;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return Whether the commandName field is set.
*/
boolean hasCommandName();
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return The commandName.
*/
java.lang.String getCommandName();
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return The bytes for commandName.
*/
com.google.protobuf.ByteString
getCommandNameBytes();
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
int getAliasesCount();
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
boolean containsAliases(
java.lang.String key);
/**
* Use {@link #getAliasesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getAliases();
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
java.util.Map
getAliasesMap();
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
java.lang.String getAliasesOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
java.lang.String getAliasesOrThrow(
java.lang.String key);
/**
* optional string origin = 9;
* @return Whether the origin field is set.
*/
boolean hasOrigin();
/**
* optional string origin = 9;
* @return The origin.
*/
java.lang.String getOrigin();
/**
* optional string origin = 9;
* @return The bytes for origin.
*/
com.google.protobuf.ByteString
getOriginBytes();
/**
* optional int32 sequenceNumber = 10;
* @return Whether the sequenceNumber field is set.
*/
boolean hasSequenceNumber();
/**
* optional int32 sequenceNumber = 10;
* @return The sequenceNumber.
*/
int getSequenceNumber();
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
* @return Whether the commandId field is set.
*/
boolean hasCommandId();
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
* @return The commandId.
*/
org.yamcs.protobuf.Commanding.CommandId getCommandId();
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
*/
org.yamcs.protobuf.Commanding.CommandIdOrBuilder getCommandIdOrBuilder();
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
java.util.List
getAttrList();
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
org.yamcs.protobuf.Commanding.CommandHistoryAttribute getAttr(int index);
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
int getAttrCount();
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
java.util.List extends org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder>
getAttrOrBuilderList();
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder getAttrOrBuilder(
int index);
/**
* optional .google.protobuf.Timestamp generationTime = 6;
* @return Whether the generationTime field is set.
*/
boolean hasGenerationTime();
/**
* optional .google.protobuf.Timestamp generationTime = 6;
* @return The generationTime.
*/
com.google.protobuf.Timestamp getGenerationTime();
/**
* optional .google.protobuf.Timestamp generationTime = 6;
*/
com.google.protobuf.TimestampOrBuilder getGenerationTimeOrBuilder();
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
java.util.List
getAssignmentsList();
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
org.yamcs.protobuf.Commanding.CommandAssignment getAssignments(int index);
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
int getAssignmentsCount();
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
java.util.List extends org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>
getAssignmentsOrBuilderList();
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder getAssignmentsOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandHistoryEntry}
*/
public static final class CommandHistoryEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.commanding.CommandHistoryEntry)
CommandHistoryEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandHistoryEntry.newBuilder() to construct.
private CommandHistoryEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandHistoryEntry() {
id_ = "";
commandName_ = "";
origin_ = "";
attr_ = java.util.Collections.emptyList();
assignments_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandHistoryEntry();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandHistoryEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.yamcs.protobuf.Commanding.CommandId.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) != 0)) {
subBuilder = commandId_.toBuilder();
}
commandId_ = input.readMessage(org.yamcs.protobuf.Commanding.CommandId.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(commandId_);
commandId_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
attr_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
attr_.add(
input.readMessage(org.yamcs.protobuf.Commanding.CommandHistoryAttribute.PARSER, extensionRegistry));
break;
}
case 50: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) != 0)) {
subBuilder = generationTime_.toBuilder();
}
generationTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(generationTime_);
generationTime_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
id_ = bs;
break;
}
case 66: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
commandName_ = bs;
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
origin_ = bs;
break;
}
case 80: {
bitField0_ |= 0x00000008;
sequenceNumber_ = input.readInt32();
break;
}
case 90: {
if (!((mutable_bitField0_ & 0x00000100) != 0)) {
assignments_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
assignments_.add(
input.readMessage(org.yamcs.protobuf.Commanding.CommandAssignment.PARSER, extensionRegistry));
break;
}
case 98: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
aliases_ = com.google.protobuf.MapField.newMapField(
AliasesDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000004;
}
com.google.protobuf.MapEntry
aliases__ = input.readMessage(
AliasesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
aliases_.getMutableMap().put(
aliases__.getKey(), aliases__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) != 0)) {
attr_ = java.util.Collections.unmodifiableList(attr_);
}
if (((mutable_bitField0_ & 0x00000100) != 0)) {
assignments_ = java.util.Collections.unmodifiableList(assignments_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 12:
return internalGetAliases();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandHistoryEntry.class, org.yamcs.protobuf.Commanding.CommandHistoryEntry.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 7;
private volatile java.lang.Object id_;
/**
* optional string id = 7;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string id = 7;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 7;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMMANDNAME_FIELD_NUMBER = 8;
private volatile java.lang.Object commandName_;
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return Whether the commandName field is set.
*/
@java.lang.Override
public boolean hasCommandName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return The commandName.
*/
@java.lang.Override
public java.lang.String getCommandName() {
java.lang.Object ref = commandName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
commandName_ = s;
}
return s;
}
}
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return The bytes for commandName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCommandNameBytes() {
java.lang.Object ref = commandName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commandName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ALIASES_FIELD_NUMBER = 12;
private static final class AliasesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_AliasesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> aliases_;
private com.google.protobuf.MapField
internalGetAliases() {
if (aliases_ == null) {
return com.google.protobuf.MapField.emptyMapField(
AliasesDefaultEntryHolder.defaultEntry);
}
return aliases_;
}
public int getAliasesCount() {
return internalGetAliases().getMap().size();
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
@java.lang.Override
public boolean containsAliases(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetAliases().getMap().containsKey(key);
}
/**
* Use {@link #getAliasesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getAliases() {
return getAliasesMap();
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
@java.lang.Override
public java.util.Map getAliasesMap() {
return internalGetAliases().getMap();
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
@java.lang.Override
public java.lang.String getAliasesOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetAliases().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
@java.lang.Override
public java.lang.String getAliasesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetAliases().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int ORIGIN_FIELD_NUMBER = 9;
private volatile java.lang.Object origin_;
/**
* optional string origin = 9;
* @return Whether the origin field is set.
*/
@java.lang.Override
public boolean hasOrigin() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string origin = 9;
* @return The origin.
*/
@java.lang.Override
public java.lang.String getOrigin() {
java.lang.Object ref = origin_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
origin_ = s;
}
return s;
}
}
/**
* optional string origin = 9;
* @return The bytes for origin.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOriginBytes() {
java.lang.Object ref = origin_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
origin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEQUENCENUMBER_FIELD_NUMBER = 10;
private int sequenceNumber_;
/**
* optional int32 sequenceNumber = 10;
* @return Whether the sequenceNumber field is set.
*/
@java.lang.Override
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int32 sequenceNumber = 10;
* @return The sequenceNumber.
*/
@java.lang.Override
public int getSequenceNumber() {
return sequenceNumber_;
}
public static final int COMMANDID_FIELD_NUMBER = 1;
private org.yamcs.protobuf.Commanding.CommandId commandId_;
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
* @return Whether the commandId field is set.
*/
@java.lang.Override
public boolean hasCommandId() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
* @return The commandId.
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandId getCommandId() {
return commandId_ == null ? org.yamcs.protobuf.Commanding.CommandId.getDefaultInstance() : commandId_;
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandIdOrBuilder getCommandIdOrBuilder() {
return commandId_ == null ? org.yamcs.protobuf.Commanding.CommandId.getDefaultInstance() : commandId_;
}
public static final int ATTR_FIELD_NUMBER = 3;
private java.util.List attr_;
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
@java.lang.Override
public java.util.List getAttrList() {
return attr_;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder>
getAttrOrBuilderList() {
return attr_;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
@java.lang.Override
public int getAttrCount() {
return attr_.size();
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryAttribute getAttr(int index) {
return attr_.get(index);
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder getAttrOrBuilder(
int index) {
return attr_.get(index);
}
public static final int GENERATIONTIME_FIELD_NUMBER = 6;
private com.google.protobuf.Timestamp generationTime_;
/**
* optional .google.protobuf.Timestamp generationTime = 6;
* @return Whether the generationTime field is set.
*/
@java.lang.Override
public boolean hasGenerationTime() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
* @return The generationTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getGenerationTime() {
return generationTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : generationTime_;
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getGenerationTimeOrBuilder() {
return generationTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : generationTime_;
}
public static final int ASSIGNMENTS_FIELD_NUMBER = 11;
private java.util.List assignments_;
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
@java.lang.Override
public java.util.List getAssignmentsList() {
return assignments_;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>
getAssignmentsOrBuilderList() {
return assignments_;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
@java.lang.Override
public int getAssignmentsCount() {
return assignments_.size();
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandAssignment getAssignments(int index) {
return assignments_.get(index);
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder getAssignmentsOrBuilder(
int index) {
return assignments_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasCommandId()) {
if (!getCommandId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getAttrCount(); i++) {
if (!getAttr(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getAssignmentsCount(); i++) {
if (!getAssignments(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(1, getCommandId());
}
for (int i = 0; i < attr_.size(); i++) {
output.writeMessage(3, attr_.get(i));
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(6, getGenerationTime());
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, commandName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, origin_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(10, sequenceNumber_);
}
for (int i = 0; i < assignments_.size(); i++) {
output.writeMessage(11, assignments_.get(i));
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetAliases(),
AliasesDefaultEntryHolder.defaultEntry,
12);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getCommandId());
}
for (int i = 0; i < attr_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, attr_.get(i));
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getGenerationTime());
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, commandName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, origin_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(10, sequenceNumber_);
}
for (int i = 0; i < assignments_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, assignments_.get(i));
}
for (java.util.Map.Entry entry
: internalGetAliases().getMap().entrySet()) {
com.google.protobuf.MapEntry
aliases__ = AliasesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, aliases__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Commanding.CommandHistoryEntry)) {
return super.equals(obj);
}
org.yamcs.protobuf.Commanding.CommandHistoryEntry other = (org.yamcs.protobuf.Commanding.CommandHistoryEntry) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (hasCommandName() != other.hasCommandName()) return false;
if (hasCommandName()) {
if (!getCommandName()
.equals(other.getCommandName())) return false;
}
if (!internalGetAliases().equals(
other.internalGetAliases())) return false;
if (hasOrigin() != other.hasOrigin()) return false;
if (hasOrigin()) {
if (!getOrigin()
.equals(other.getOrigin())) return false;
}
if (hasSequenceNumber() != other.hasSequenceNumber()) return false;
if (hasSequenceNumber()) {
if (getSequenceNumber()
!= other.getSequenceNumber()) return false;
}
if (hasCommandId() != other.hasCommandId()) return false;
if (hasCommandId()) {
if (!getCommandId()
.equals(other.getCommandId())) return false;
}
if (!getAttrList()
.equals(other.getAttrList())) return false;
if (hasGenerationTime() != other.hasGenerationTime()) return false;
if (hasGenerationTime()) {
if (!getGenerationTime()
.equals(other.getGenerationTime())) return false;
}
if (!getAssignmentsList()
.equals(other.getAssignmentsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasCommandName()) {
hash = (37 * hash) + COMMANDNAME_FIELD_NUMBER;
hash = (53 * hash) + getCommandName().hashCode();
}
if (!internalGetAliases().getMap().isEmpty()) {
hash = (37 * hash) + ALIASES_FIELD_NUMBER;
hash = (53 * hash) + internalGetAliases().hashCode();
}
if (hasOrigin()) {
hash = (37 * hash) + ORIGIN_FIELD_NUMBER;
hash = (53 * hash) + getOrigin().hashCode();
}
if (hasSequenceNumber()) {
hash = (37 * hash) + SEQUENCENUMBER_FIELD_NUMBER;
hash = (53 * hash) + getSequenceNumber();
}
if (hasCommandId()) {
hash = (37 * hash) + COMMANDID_FIELD_NUMBER;
hash = (53 * hash) + getCommandId().hashCode();
}
if (getAttrCount() > 0) {
hash = (37 * hash) + ATTR_FIELD_NUMBER;
hash = (53 * hash) + getAttrList().hashCode();
}
if (hasGenerationTime()) {
hash = (37 * hash) + GENERATIONTIME_FIELD_NUMBER;
hash = (53 * hash) + getGenerationTime().hashCode();
}
if (getAssignmentsCount() > 0) {
hash = (37 * hash) + ASSIGNMENTS_FIELD_NUMBER;
hash = (53 * hash) + getAssignmentsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Commanding.CommandHistoryEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.commanding.CommandHistoryEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.commanding.CommandHistoryEntry)
org.yamcs.protobuf.Commanding.CommandHistoryEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 12:
return internalGetAliases();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 12:
return internalGetMutableAliases();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Commanding.CommandHistoryEntry.class, org.yamcs.protobuf.Commanding.CommandHistoryEntry.Builder.class);
}
// Construct using org.yamcs.protobuf.Commanding.CommandHistoryEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCommandIdFieldBuilder();
getAttrFieldBuilder();
getGenerationTimeFieldBuilder();
getAssignmentsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
commandName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
internalGetMutableAliases().clear();
origin_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
sequenceNumber_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
if (commandIdBuilder_ == null) {
commandId_ = null;
} else {
commandIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (attrBuilder_ == null) {
attr_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
attrBuilder_.clear();
}
if (generationTimeBuilder_ == null) {
generationTime_ = null;
} else {
generationTimeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
if (assignmentsBuilder_ == null) {
assignments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
} else {
assignmentsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Commanding.internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryEntry getDefaultInstanceForType() {
return org.yamcs.protobuf.Commanding.CommandHistoryEntry.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryEntry build() {
org.yamcs.protobuf.Commanding.CommandHistoryEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryEntry buildPartial() {
org.yamcs.protobuf.Commanding.CommandHistoryEntry result = new org.yamcs.protobuf.Commanding.CommandHistoryEntry(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.commandName_ = commandName_;
result.aliases_ = internalGetAliases();
result.aliases_.makeImmutable();
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.origin_ = origin_;
if (((from_bitField0_ & 0x00000010) != 0)) {
result.sequenceNumber_ = sequenceNumber_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
if (commandIdBuilder_ == null) {
result.commandId_ = commandId_;
} else {
result.commandId_ = commandIdBuilder_.build();
}
to_bitField0_ |= 0x00000010;
}
if (attrBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
attr_ = java.util.Collections.unmodifiableList(attr_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.attr_ = attr_;
} else {
result.attr_ = attrBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) != 0)) {
if (generationTimeBuilder_ == null) {
result.generationTime_ = generationTime_;
} else {
result.generationTime_ = generationTimeBuilder_.build();
}
to_bitField0_ |= 0x00000020;
}
if (assignmentsBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
assignments_ = java.util.Collections.unmodifiableList(assignments_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.assignments_ = assignments_;
} else {
result.assignments_ = assignmentsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Commanding.CommandHistoryEntry) {
return mergeFrom((org.yamcs.protobuf.Commanding.CommandHistoryEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Commanding.CommandHistoryEntry other) {
if (other == org.yamcs.protobuf.Commanding.CommandHistoryEntry.getDefaultInstance()) return this;
if (other.hasId()) {
bitField0_ |= 0x00000001;
id_ = other.id_;
onChanged();
}
if (other.hasCommandName()) {
bitField0_ |= 0x00000002;
commandName_ = other.commandName_;
onChanged();
}
internalGetMutableAliases().mergeFrom(
other.internalGetAliases());
if (other.hasOrigin()) {
bitField0_ |= 0x00000008;
origin_ = other.origin_;
onChanged();
}
if (other.hasSequenceNumber()) {
setSequenceNumber(other.getSequenceNumber());
}
if (other.hasCommandId()) {
mergeCommandId(other.getCommandId());
}
if (attrBuilder_ == null) {
if (!other.attr_.isEmpty()) {
if (attr_.isEmpty()) {
attr_ = other.attr_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureAttrIsMutable();
attr_.addAll(other.attr_);
}
onChanged();
}
} else {
if (!other.attr_.isEmpty()) {
if (attrBuilder_.isEmpty()) {
attrBuilder_.dispose();
attrBuilder_ = null;
attr_ = other.attr_;
bitField0_ = (bitField0_ & ~0x00000040);
attrBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAttrFieldBuilder() : null;
} else {
attrBuilder_.addAllMessages(other.attr_);
}
}
}
if (other.hasGenerationTime()) {
mergeGenerationTime(other.getGenerationTime());
}
if (assignmentsBuilder_ == null) {
if (!other.assignments_.isEmpty()) {
if (assignments_.isEmpty()) {
assignments_ = other.assignments_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureAssignmentsIsMutable();
assignments_.addAll(other.assignments_);
}
onChanged();
}
} else {
if (!other.assignments_.isEmpty()) {
if (assignmentsBuilder_.isEmpty()) {
assignmentsBuilder_.dispose();
assignmentsBuilder_ = null;
assignments_ = other.assignments_;
bitField0_ = (bitField0_ & ~0x00000100);
assignmentsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAssignmentsFieldBuilder() : null;
} else {
assignmentsBuilder_.addAllMessages(other.assignments_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasCommandId()) {
if (!getCommandId().isInitialized()) {
return false;
}
}
for (int i = 0; i < getAttrCount(); i++) {
if (!getAttr(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getAssignmentsCount(); i++) {
if (!getAssignments(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Commanding.CommandHistoryEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Commanding.CommandHistoryEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
* optional string id = 7;
* @return Whether the id field is set.
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string id = 7;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 7;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 7;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 7;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 7;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
private java.lang.Object commandName_ = "";
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return Whether the commandName field is set.
*/
public boolean hasCommandName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return The commandName.
*/
public java.lang.String getCommandName() {
java.lang.Object ref = commandName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
commandName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return The bytes for commandName.
*/
public com.google.protobuf.ByteString
getCommandNameBytes() {
java.lang.Object ref = commandName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commandName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @param value The commandName to set.
* @return This builder for chaining.
*/
public Builder setCommandName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
commandName_ = value;
onChanged();
return this;
}
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @return This builder for chaining.
*/
public Builder clearCommandName() {
bitField0_ = (bitField0_ & ~0x00000002);
commandName_ = getDefaultInstance().getCommandName();
onChanged();
return this;
}
/**
*
* Qualified name
*
*
* optional string commandName = 8;
* @param value The bytes for commandName to set.
* @return This builder for chaining.
*/
public Builder setCommandNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
commandName_ = value;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> aliases_;
private com.google.protobuf.MapField
internalGetAliases() {
if (aliases_ == null) {
return com.google.protobuf.MapField.emptyMapField(
AliasesDefaultEntryHolder.defaultEntry);
}
return aliases_;
}
private com.google.protobuf.MapField
internalGetMutableAliases() {
onChanged();;
if (aliases_ == null) {
aliases_ = com.google.protobuf.MapField.newMapField(
AliasesDefaultEntryHolder.defaultEntry);
}
if (!aliases_.isMutable()) {
aliases_ = aliases_.copy();
}
return aliases_;
}
public int getAliasesCount() {
return internalGetAliases().getMap().size();
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
@java.lang.Override
public boolean containsAliases(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetAliases().getMap().containsKey(key);
}
/**
* Use {@link #getAliasesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getAliases() {
return getAliasesMap();
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
@java.lang.Override
public java.util.Map getAliasesMap() {
return internalGetAliases().getMap();
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
@java.lang.Override
public java.lang.String getAliasesOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetAliases().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
@java.lang.Override
public java.lang.String getAliasesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetAliases().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearAliases() {
internalGetMutableAliases().getMutableMap()
.clear();
return this;
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
public Builder removeAliases(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableAliases().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableAliases() {
return internalGetMutableAliases().getMutableMap();
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
public Builder putAliases(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableAliases().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 12;
*/
public Builder putAllAliases(
java.util.Map values) {
internalGetMutableAliases().getMutableMap()
.putAll(values);
return this;
}
private java.lang.Object origin_ = "";
/**
* optional string origin = 9;
* @return Whether the origin field is set.
*/
public boolean hasOrigin() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string origin = 9;
* @return The origin.
*/
public java.lang.String getOrigin() {
java.lang.Object ref = origin_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
origin_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string origin = 9;
* @return The bytes for origin.
*/
public com.google.protobuf.ByteString
getOriginBytes() {
java.lang.Object ref = origin_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
origin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string origin = 9;
* @param value The origin to set.
* @return This builder for chaining.
*/
public Builder setOrigin(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
origin_ = value;
onChanged();
return this;
}
/**
* optional string origin = 9;
* @return This builder for chaining.
*/
public Builder clearOrigin() {
bitField0_ = (bitField0_ & ~0x00000008);
origin_ = getDefaultInstance().getOrigin();
onChanged();
return this;
}
/**
* optional string origin = 9;
* @param value The bytes for origin to set.
* @return This builder for chaining.
*/
public Builder setOriginBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
origin_ = value;
onChanged();
return this;
}
private int sequenceNumber_ ;
/**
* optional int32 sequenceNumber = 10;
* @return Whether the sequenceNumber field is set.
*/
@java.lang.Override
public boolean hasSequenceNumber() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional int32 sequenceNumber = 10;
* @return The sequenceNumber.
*/
@java.lang.Override
public int getSequenceNumber() {
return sequenceNumber_;
}
/**
* optional int32 sequenceNumber = 10;
* @param value The sequenceNumber to set.
* @return This builder for chaining.
*/
public Builder setSequenceNumber(int value) {
bitField0_ |= 0x00000010;
sequenceNumber_ = value;
onChanged();
return this;
}
/**
* optional int32 sequenceNumber = 10;
* @return This builder for chaining.
*/
public Builder clearSequenceNumber() {
bitField0_ = (bitField0_ & ~0x00000010);
sequenceNumber_ = 0;
onChanged();
return this;
}
private org.yamcs.protobuf.Commanding.CommandId commandId_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandId, org.yamcs.protobuf.Commanding.CommandId.Builder, org.yamcs.protobuf.Commanding.CommandIdOrBuilder> commandIdBuilder_;
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
* @return Whether the commandId field is set.
*/
public boolean hasCommandId() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
* @return The commandId.
*/
public org.yamcs.protobuf.Commanding.CommandId getCommandId() {
if (commandIdBuilder_ == null) {
return commandId_ == null ? org.yamcs.protobuf.Commanding.CommandId.getDefaultInstance() : commandId_;
} else {
return commandIdBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
*/
public Builder setCommandId(org.yamcs.protobuf.Commanding.CommandId value) {
if (commandIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
commandId_ = value;
onChanged();
} else {
commandIdBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
*/
public Builder setCommandId(
org.yamcs.protobuf.Commanding.CommandId.Builder builderForValue) {
if (commandIdBuilder_ == null) {
commandId_ = builderForValue.build();
onChanged();
} else {
commandIdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
*/
public Builder mergeCommandId(org.yamcs.protobuf.Commanding.CommandId value) {
if (commandIdBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
commandId_ != null &&
commandId_ != org.yamcs.protobuf.Commanding.CommandId.getDefaultInstance()) {
commandId_ =
org.yamcs.protobuf.Commanding.CommandId.newBuilder(commandId_).mergeFrom(value).buildPartial();
} else {
commandId_ = value;
}
onChanged();
} else {
commandIdBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
*/
public Builder clearCommandId() {
if (commandIdBuilder_ == null) {
commandId_ = null;
onChanged();
} else {
commandIdBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
*/
public org.yamcs.protobuf.Commanding.CommandId.Builder getCommandIdBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getCommandIdFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
*/
public org.yamcs.protobuf.Commanding.CommandIdOrBuilder getCommandIdOrBuilder() {
if (commandIdBuilder_ != null) {
return commandIdBuilder_.getMessageOrBuilder();
} else {
return commandId_ == null ?
org.yamcs.protobuf.Commanding.CommandId.getDefaultInstance() : commandId_;
}
}
/**
* optional .yamcs.protobuf.commanding.CommandId commandId = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandId, org.yamcs.protobuf.Commanding.CommandId.Builder, org.yamcs.protobuf.Commanding.CommandIdOrBuilder>
getCommandIdFieldBuilder() {
if (commandIdBuilder_ == null) {
commandIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandId, org.yamcs.protobuf.Commanding.CommandId.Builder, org.yamcs.protobuf.Commanding.CommandIdOrBuilder>(
getCommandId(),
getParentForChildren(),
isClean());
commandId_ = null;
}
return commandIdBuilder_;
}
private java.util.List attr_ =
java.util.Collections.emptyList();
private void ensureAttrIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
attr_ = new java.util.ArrayList(attr_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandHistoryAttribute, org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder, org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder> attrBuilder_;
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public java.util.List getAttrList() {
if (attrBuilder_ == null) {
return java.util.Collections.unmodifiableList(attr_);
} else {
return attrBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public int getAttrCount() {
if (attrBuilder_ == null) {
return attr_.size();
} else {
return attrBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public org.yamcs.protobuf.Commanding.CommandHistoryAttribute getAttr(int index) {
if (attrBuilder_ == null) {
return attr_.get(index);
} else {
return attrBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public Builder setAttr(
int index, org.yamcs.protobuf.Commanding.CommandHistoryAttribute value) {
if (attrBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttrIsMutable();
attr_.set(index, value);
onChanged();
} else {
attrBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public Builder setAttr(
int index, org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder builderForValue) {
if (attrBuilder_ == null) {
ensureAttrIsMutable();
attr_.set(index, builderForValue.build());
onChanged();
} else {
attrBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public Builder addAttr(org.yamcs.protobuf.Commanding.CommandHistoryAttribute value) {
if (attrBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttrIsMutable();
attr_.add(value);
onChanged();
} else {
attrBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public Builder addAttr(
int index, org.yamcs.protobuf.Commanding.CommandHistoryAttribute value) {
if (attrBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttrIsMutable();
attr_.add(index, value);
onChanged();
} else {
attrBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public Builder addAttr(
org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder builderForValue) {
if (attrBuilder_ == null) {
ensureAttrIsMutable();
attr_.add(builderForValue.build());
onChanged();
} else {
attrBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public Builder addAttr(
int index, org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder builderForValue) {
if (attrBuilder_ == null) {
ensureAttrIsMutable();
attr_.add(index, builderForValue.build());
onChanged();
} else {
attrBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public Builder addAllAttr(
java.lang.Iterable extends org.yamcs.protobuf.Commanding.CommandHistoryAttribute> values) {
if (attrBuilder_ == null) {
ensureAttrIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, attr_);
onChanged();
} else {
attrBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public Builder clearAttr() {
if (attrBuilder_ == null) {
attr_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
attrBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public Builder removeAttr(int index) {
if (attrBuilder_ == null) {
ensureAttrIsMutable();
attr_.remove(index);
onChanged();
} else {
attrBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder getAttrBuilder(
int index) {
return getAttrFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder getAttrOrBuilder(
int index) {
if (attrBuilder_ == null) {
return attr_.get(index); } else {
return attrBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public java.util.List extends org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder>
getAttrOrBuilderList() {
if (attrBuilder_ != null) {
return attrBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(attr_);
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder addAttrBuilder() {
return getAttrFieldBuilder().addBuilder(
org.yamcs.protobuf.Commanding.CommandHistoryAttribute.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder addAttrBuilder(
int index) {
return getAttrFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Commanding.CommandHistoryAttribute.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.commanding.CommandHistoryAttribute attr = 3;
*/
public java.util.List
getAttrBuilderList() {
return getAttrFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandHistoryAttribute, org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder, org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder>
getAttrFieldBuilder() {
if (attrBuilder_ == null) {
attrBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandHistoryAttribute, org.yamcs.protobuf.Commanding.CommandHistoryAttribute.Builder, org.yamcs.protobuf.Commanding.CommandHistoryAttributeOrBuilder>(
attr_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
attr_ = null;
}
return attrBuilder_;
}
private com.google.protobuf.Timestamp generationTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> generationTimeBuilder_;
/**
* optional .google.protobuf.Timestamp generationTime = 6;
* @return Whether the generationTime field is set.
*/
public boolean hasGenerationTime() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
* @return The generationTime.
*/
public com.google.protobuf.Timestamp getGenerationTime() {
if (generationTimeBuilder_ == null) {
return generationTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : generationTime_;
} else {
return generationTimeBuilder_.getMessage();
}
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
*/
public Builder setGenerationTime(com.google.protobuf.Timestamp value) {
if (generationTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
generationTime_ = value;
onChanged();
} else {
generationTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
*/
public Builder setGenerationTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (generationTimeBuilder_ == null) {
generationTime_ = builderForValue.build();
onChanged();
} else {
generationTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
*/
public Builder mergeGenerationTime(com.google.protobuf.Timestamp value) {
if (generationTimeBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
generationTime_ != null &&
generationTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
generationTime_ =
com.google.protobuf.Timestamp.newBuilder(generationTime_).mergeFrom(value).buildPartial();
} else {
generationTime_ = value;
}
onChanged();
} else {
generationTimeBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
*/
public Builder clearGenerationTime() {
if (generationTimeBuilder_ == null) {
generationTime_ = null;
onChanged();
} else {
generationTimeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
*/
public com.google.protobuf.Timestamp.Builder getGenerationTimeBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getGenerationTimeFieldBuilder().getBuilder();
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
*/
public com.google.protobuf.TimestampOrBuilder getGenerationTimeOrBuilder() {
if (generationTimeBuilder_ != null) {
return generationTimeBuilder_.getMessageOrBuilder();
} else {
return generationTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : generationTime_;
}
}
/**
* optional .google.protobuf.Timestamp generationTime = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getGenerationTimeFieldBuilder() {
if (generationTimeBuilder_ == null) {
generationTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getGenerationTime(),
getParentForChildren(),
isClean());
generationTime_ = null;
}
return generationTimeBuilder_;
}
private java.util.List assignments_ =
java.util.Collections.emptyList();
private void ensureAssignmentsIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
assignments_ = new java.util.ArrayList(assignments_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandAssignment, org.yamcs.protobuf.Commanding.CommandAssignment.Builder, org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder> assignmentsBuilder_;
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public java.util.List getAssignmentsList() {
if (assignmentsBuilder_ == null) {
return java.util.Collections.unmodifiableList(assignments_);
} else {
return assignmentsBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public int getAssignmentsCount() {
if (assignmentsBuilder_ == null) {
return assignments_.size();
} else {
return assignmentsBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public org.yamcs.protobuf.Commanding.CommandAssignment getAssignments(int index) {
if (assignmentsBuilder_ == null) {
return assignments_.get(index);
} else {
return assignmentsBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public Builder setAssignments(
int index, org.yamcs.protobuf.Commanding.CommandAssignment value) {
if (assignmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssignmentsIsMutable();
assignments_.set(index, value);
onChanged();
} else {
assignmentsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public Builder setAssignments(
int index, org.yamcs.protobuf.Commanding.CommandAssignment.Builder builderForValue) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
assignments_.set(index, builderForValue.build());
onChanged();
} else {
assignmentsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public Builder addAssignments(org.yamcs.protobuf.Commanding.CommandAssignment value) {
if (assignmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssignmentsIsMutable();
assignments_.add(value);
onChanged();
} else {
assignmentsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public Builder addAssignments(
int index, org.yamcs.protobuf.Commanding.CommandAssignment value) {
if (assignmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssignmentsIsMutable();
assignments_.add(index, value);
onChanged();
} else {
assignmentsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public Builder addAssignments(
org.yamcs.protobuf.Commanding.CommandAssignment.Builder builderForValue) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
assignments_.add(builderForValue.build());
onChanged();
} else {
assignmentsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public Builder addAssignments(
int index, org.yamcs.protobuf.Commanding.CommandAssignment.Builder builderForValue) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
assignments_.add(index, builderForValue.build());
onChanged();
} else {
assignmentsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public Builder addAllAssignments(
java.lang.Iterable extends org.yamcs.protobuf.Commanding.CommandAssignment> values) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, assignments_);
onChanged();
} else {
assignmentsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public Builder clearAssignments() {
if (assignmentsBuilder_ == null) {
assignments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
assignmentsBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public Builder removeAssignments(int index) {
if (assignmentsBuilder_ == null) {
ensureAssignmentsIsMutable();
assignments_.remove(index);
onChanged();
} else {
assignmentsBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public org.yamcs.protobuf.Commanding.CommandAssignment.Builder getAssignmentsBuilder(
int index) {
return getAssignmentsFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder getAssignmentsOrBuilder(
int index) {
if (assignmentsBuilder_ == null) {
return assignments_.get(index); } else {
return assignmentsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public java.util.List extends org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>
getAssignmentsOrBuilderList() {
if (assignmentsBuilder_ != null) {
return assignmentsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(assignments_);
}
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public org.yamcs.protobuf.Commanding.CommandAssignment.Builder addAssignmentsBuilder() {
return getAssignmentsFieldBuilder().addBuilder(
org.yamcs.protobuf.Commanding.CommandAssignment.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public org.yamcs.protobuf.Commanding.CommandAssignment.Builder addAssignmentsBuilder(
int index) {
return getAssignmentsFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Commanding.CommandAssignment.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 11;
*/
public java.util.List
getAssignmentsBuilderList() {
return getAssignmentsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandAssignment, org.yamcs.protobuf.Commanding.CommandAssignment.Builder, org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>
getAssignmentsFieldBuilder() {
if (assignmentsBuilder_ == null) {
assignmentsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Commanding.CommandAssignment, org.yamcs.protobuf.Commanding.CommandAssignment.Builder, org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>(
assignments_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
assignments_ = null;
}
return assignmentsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.commanding.CommandHistoryEntry)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.commanding.CommandHistoryEntry)
private static final org.yamcs.protobuf.Commanding.CommandHistoryEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Commanding.CommandHistoryEntry();
}
public static org.yamcs.protobuf.Commanding.CommandHistoryEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandHistoryEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandHistoryEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Commanding.CommandHistoryEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandId_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandId_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandQueueInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandQueueInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandQueueEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandQueueEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandQueueEvent_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandQueueEvent_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandQueueRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandQueueRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandSignificance_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandSignificance_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_VerifierConfig_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_VerifierConfig_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandAssignment_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandAssignment_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_AliasesEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_AliasesEntry_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n*yamcs/protobuf/commanding/commanding.p" +
"roto\022\031yamcs.protobuf.commanding\032\032yamcs/p" +
"rotobuf/yamcs.proto\032\034yamcs/protobuf/mdb/" +
"mdb.proto\032\037google/protobuf/timestamp.pro" +
"to\"`\n\tCommandId\022\026\n\016generationTime\030\001 \002(\003\022" +
"\016\n\006origin\030\002 \002(\t\022\026\n\016sequenceNumber\030\003 \002(\005\022" +
"\023\n\013commandName\030\004 \001(\t\"\214\003\n\020CommandQueueInf" +
"o\022\020\n\010instance\030\001 \001(\t\022\025\n\rprocessorName\030\002 \001" +
"(\t\022\014\n\004name\030\003 \001(\t\0224\n\005state\030\004 \001(\0162%.yamcs." +
"protobuf.commanding.QueueState\022\r\n\005order\030" +
"\t \001(\005\022\r\n\005users\030\n \003(\t\022\016\n\006groups\030\013 \003(\t\022L\n\010" +
"minLevel\030\014 \001(\0162:.yamcs.protobuf.mdb.Sign" +
"ificanceInfo.SignificanceLevelType\022\022\n\ntc" +
"Patterns\030\r \003(\t\022=\n\007entries\030\016 \003(\0132,.yamcs." +
"protobuf.commanding.CommandQueueEntry\022\035\n" +
"\025acceptedCommandsCount\030\017 \001(\005\022\035\n\025rejected" +
"CommandsCount\030\020 \001(\005\"\352\002\n\021CommandQueueEntr" +
"y\022\020\n\010instance\030\001 \001(\t\022\025\n\rprocessorName\030\002 \001" +
"(\t\022\021\n\tqueueName\030\003 \001(\t\022\n\n\002id\030\016 \001(\t\022\016\n\006ori" +
"gin\030\017 \001(\t\022\026\n\016sequenceNumber\030\020 \001(\005\022\023\n\013com" +
"mandName\030\021 \001(\t\022A\n\013assignments\030\022 \003(\0132,.ya" +
"mcs.protobuf.commanding.CommandAssignmen" +
"t\022\016\n\006binary\030\006 \001(\014\022\020\n\010username\030\007 \001(\t\022\017\n\007c" +
"omment\030\013 \001(\t\0222\n\016generationTime\030\014 \001(\0132\032.g" +
"oogle.protobuf.Timestamp\022&\n\036pendingTrans" +
"missionConstraints\030\r \001(\010\"\350\001\n\021CommandQueu" +
"eEvent\022?\n\004type\030\001 \001(\01621.yamcs.protobuf.co" +
"mmanding.CommandQueueEvent.Type\022:\n\004data\030" +
"\002 \001(\0132,.yamcs.protobuf.commanding.Comman" +
"dQueueEntry\"V\n\004Type\022\021\n\rCOMMAND_ADDED\020\001\022\024" +
"\n\020COMMAND_REJECTED\020\002\022\020\n\014COMMAND_SENT\020\003\022\023" +
"\n\017COMMAND_UPDATED\020\004\"\257\001\n\023CommandQueueRequ" +
"est\022>\n\tqueueInfo\030\001 \001(\0132+.yamcs.protobuf." +
"commanding.CommandQueueInfo\022@\n\nqueueEntr" +
"y\030\002 \001(\0132,.yamcs.protobuf.commanding.Comm" +
"andQueueEntry\022\026\n\007rebuild\030\003 \001(\010:\005false\"i\n" +
"\023CommandSignificance\022\026\n\016sequenceNumber\030\001" +
" \001(\005\022:\n\014significance\030\002 \001(\0132$.yamcs.proto" +
"buf.mdb.SignificanceInfo\"\265\001\n\016VerifierCon" +
"fig\022\017\n\007disable\030\002 \001(\010\022J\n\013checkWindow\030\003 \001(" +
"\01325.yamcs.protobuf.commanding.VerifierCo" +
"nfig.CheckWindow\032F\n\013CheckWindow\022\033\n\023timeT" +
"oStartChecking\030\001 \001(\003\022\032\n\022timeToStopChecki" +
"ng\030\002 \001(\003\"[\n\027CommandHistoryAttribute\022\014\n\004n" +
"ame\030\001 \001(\t\022$\n\005value\030\002 \001(\0132\025.yamcs.protobu" +
"f.Value\022\014\n\004time\030\003 \001(\003\"Z\n\021CommandAssignme" +
"nt\022\014\n\004name\030\001 \001(\t\022$\n\005value\030\002 \001(\0132\025.yamcs." +
"protobuf.Value\022\021\n\tuserInput\030\003 \001(\010\"\316\003\n\023Co" +
"mmandHistoryEntry\022\n\n\002id\030\007 \001(\t\022\023\n\013command" +
"Name\030\010 \001(\t\022L\n\007aliases\030\014 \003(\0132;.yamcs.prot" +
"obuf.commanding.CommandHistoryEntry.Alia" +
"sesEntry\022\016\n\006origin\030\t \001(\t\022\026\n\016sequenceNumb" +
"er\030\n \001(\005\0227\n\tcommandId\030\001 \001(\0132$.yamcs.prot" +
"obuf.commanding.CommandId\022@\n\004attr\030\003 \003(\0132" +
"2.yamcs.protobuf.commanding.CommandHisto" +
"ryAttribute\0222\n\016generationTime\030\006 \001(\0132\032.go" +
"ogle.protobuf.Timestamp\022A\n\013assignments\030\013" +
" \003(\0132,.yamcs.protobuf.commanding.Command" +
"Assignment\032.\n\014AliasesEntry\022\013\n\003key\030\001 \001(\t\022" +
"\r\n\005value\030\002 \001(\t:\0028\001*4\n\nQueueState\022\013\n\007BLOC" +
"KED\020\001\022\014\n\010DISABLED\020\002\022\013\n\007ENABLED\020\003B\024\n\022org." +
"yamcs.protobuf"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.yamcs.protobuf.Yamcs.getDescriptor(),
org.yamcs.protobuf.Mdb.getDescriptor(),
com.google.protobuf.TimestampProto.getDescriptor(),
});
internal_static_yamcs_protobuf_commanding_CommandId_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_yamcs_protobuf_commanding_CommandId_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandId_descriptor,
new java.lang.String[] { "GenerationTime", "Origin", "SequenceNumber", "CommandName", });
internal_static_yamcs_protobuf_commanding_CommandQueueInfo_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_yamcs_protobuf_commanding_CommandQueueInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandQueueInfo_descriptor,
new java.lang.String[] { "Instance", "ProcessorName", "Name", "State", "Order", "Users", "Groups", "MinLevel", "TcPatterns", "Entries", "AcceptedCommandsCount", "RejectedCommandsCount", });
internal_static_yamcs_protobuf_commanding_CommandQueueEntry_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_yamcs_protobuf_commanding_CommandQueueEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandQueueEntry_descriptor,
new java.lang.String[] { "Instance", "ProcessorName", "QueueName", "Id", "Origin", "SequenceNumber", "CommandName", "Assignments", "Binary", "Username", "Comment", "GenerationTime", "PendingTransmissionConstraints", });
internal_static_yamcs_protobuf_commanding_CommandQueueEvent_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_yamcs_protobuf_commanding_CommandQueueEvent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandQueueEvent_descriptor,
new java.lang.String[] { "Type", "Data", });
internal_static_yamcs_protobuf_commanding_CommandQueueRequest_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_yamcs_protobuf_commanding_CommandQueueRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandQueueRequest_descriptor,
new java.lang.String[] { "QueueInfo", "QueueEntry", "Rebuild", });
internal_static_yamcs_protobuf_commanding_CommandSignificance_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_yamcs_protobuf_commanding_CommandSignificance_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandSignificance_descriptor,
new java.lang.String[] { "SequenceNumber", "Significance", });
internal_static_yamcs_protobuf_commanding_VerifierConfig_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_yamcs_protobuf_commanding_VerifierConfig_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_VerifierConfig_descriptor,
new java.lang.String[] { "Disable", "CheckWindow", });
internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_descriptor =
internal_static_yamcs_protobuf_commanding_VerifierConfig_descriptor.getNestedTypes().get(0);
internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_VerifierConfig_CheckWindow_descriptor,
new java.lang.String[] { "TimeToStartChecking", "TimeToStopChecking", });
internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandHistoryAttribute_descriptor,
new java.lang.String[] { "Name", "Value", "Time", });
internal_static_yamcs_protobuf_commanding_CommandAssignment_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_yamcs_protobuf_commanding_CommandAssignment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandAssignment_descriptor,
new java.lang.String[] { "Name", "Value", "UserInput", });
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_descriptor,
new java.lang.String[] { "Id", "CommandName", "Aliases", "Origin", "SequenceNumber", "CommandId", "Attr", "GenerationTime", "Assignments", });
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_AliasesEntry_descriptor =
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_descriptor.getNestedTypes().get(0);
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_AliasesEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_commanding_CommandHistoryEntry_AliasesEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
org.yamcs.protobuf.Yamcs.getDescriptor();
org.yamcs.protobuf.Mdb.getDescriptor();
com.google.protobuf.TimestampProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy