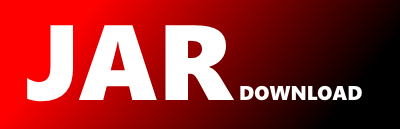
org.yamcs.protobuf.IssueCommandResponseOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: yamcs/protobuf/commanding/commands_service.proto
package org.yamcs.protobuf;
public interface IssueCommandResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.commanding.IssueCommandResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Command ID
*
*
* optional string id = 5;
* @return Whether the id field is set.
*/
boolean hasId();
/**
*
* Command ID
*
*
* optional string id = 5;
* @return The id.
*/
java.lang.String getId();
/**
*
* Command ID
*
*
* optional string id = 5;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Command generation time
*
*
* optional .google.protobuf.Timestamp generationTime = 6;
* @return Whether the generationTime field is set.
*/
boolean hasGenerationTime();
/**
*
* Command generation time
*
*
* optional .google.protobuf.Timestamp generationTime = 6;
* @return The generationTime.
*/
com.google.protobuf.Timestamp getGenerationTime();
/**
*
* Command generation time
*
*
* optional .google.protobuf.Timestamp generationTime = 6;
*/
com.google.protobuf.TimestampOrBuilder getGenerationTimeOrBuilder();
/**
*
* The origin of the command. Typically a hostname.
*
*
* optional string origin = 7;
* @return Whether the origin field is set.
*/
boolean hasOrigin();
/**
*
* The origin of the command. Typically a hostname.
*
*
* optional string origin = 7;
* @return The origin.
*/
java.lang.String getOrigin();
/**
*
* The origin of the command. Typically a hostname.
*
*
* optional string origin = 7;
* @return The bytes for origin.
*/
com.google.protobuf.ByteString
getOriginBytes();
/**
*
* The sequence number for the origin
*
*
* optional int32 sequenceNumber = 8;
* @return Whether the sequenceNumber field is set.
*/
boolean hasSequenceNumber();
/**
*
* The sequence number for the origin
*
*
* optional int32 sequenceNumber = 8;
* @return The sequenceNumber.
*/
int getSequenceNumber();
/**
*
* Qualified name
*
*
* optional string commandName = 9;
* @return Whether the commandName field is set.
*/
boolean hasCommandName();
/**
*
* Qualified name
*
*
* optional string commandName = 9;
* @return The commandName.
*/
java.lang.String getCommandName();
/**
*
* Qualified name
*
*
* optional string commandName = 9;
* @return The bytes for commandName.
*/
com.google.protobuf.ByteString
getCommandNameBytes();
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 14;
*/
int getAliasesCount();
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 14;
*/
boolean containsAliases(
java.lang.String key);
/**
* Use {@link #getAliasesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getAliases();
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 14;
*/
java.util.Map
getAliasesMap();
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 14;
*/
java.lang.String getAliasesOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Name aliases keyed by namespace.
* (as currently present in Mission Database)
*
*
* map<string, string> aliases = 14;
*/
java.lang.String getAliasesOrThrow(
java.lang.String key);
/**
*
* The name/value assignments for this command
*
*
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 12;
*/
java.util.List
getAssignmentsList();
/**
*
* The name/value assignments for this command
*
*
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 12;
*/
org.yamcs.protobuf.Commanding.CommandAssignment getAssignments(int index);
/**
*
* The name/value assignments for this command
*
*
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 12;
*/
int getAssignmentsCount();
/**
*
* The name/value assignments for this command
*
*
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 12;
*/
java.util.List extends org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder>
getAssignmentsOrBuilderList();
/**
*
* The name/value assignments for this command
*
*
* repeated .yamcs.protobuf.commanding.CommandAssignment assignments = 12;
*/
org.yamcs.protobuf.Commanding.CommandAssignmentOrBuilder getAssignmentsOrBuilder(
int index);
/**
*
* Generated binary, before any link post-processing
*
*
* optional bytes unprocessedBinary = 13;
* @return Whether the unprocessedBinary field is set.
*/
boolean hasUnprocessedBinary();
/**
*
* Generated binary, before any link post-processing
*
*
* optional bytes unprocessedBinary = 13;
* @return The unprocessedBinary.
*/
com.google.protobuf.ByteString getUnprocessedBinary();
/**
*
* Generated binary, after link post-processing.
* The differences compared to ``unprocessedBinary``,
* can be anything. Typical manipulations include
* sequence numbers or checksum calculations.
*
*
* optional bytes binary = 4;
* @return Whether the binary field is set.
*/
boolean hasBinary();
/**
*
* Generated binary, after link post-processing.
* The differences compared to ``unprocessedBinary``,
* can be anything. Typical manipulations include
* sequence numbers or checksum calculations.
*
*
* optional bytes binary = 4;
* @return The binary.
*/
com.google.protobuf.ByteString getBinary();
/**
*
* Command issuer
*
*
* optional string username = 11;
* @return Whether the username field is set.
*/
boolean hasUsername();
/**
*
* Command issuer
*
*
* optional string username = 11;
* @return The username.
*/
java.lang.String getUsername();
/**
*
* Command issuer
*
*
* optional string username = 11;
* @return The bytes for username.
*/
com.google.protobuf.ByteString
getUsernameBytes();
/**
*
* Queue that was selected for this command
*
*
* optional string queue = 10;
* @return Whether the queue field is set.
*/
boolean hasQueue();
/**
*
* Queue that was selected for this command
*
*
* optional string queue = 10;
* @return The queue.
*/
java.lang.String getQueue();
/**
*
* Queue that was selected for this command
*
*
* optional string queue = 10;
* @return The bytes for queue.
*/
com.google.protobuf.ByteString
getQueueBytes();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy