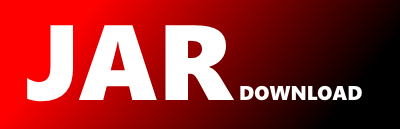
org.yamcs.protobuf.ParameterArchiveApiClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
package org.yamcs.protobuf;
import com.google.protobuf.Empty;
import org.yamcs.api.MethodHandler;
import org.yamcs.api.Observer;
import org.yamcs.protobuf.Archive.GetParameterSamplesRequest;
import org.yamcs.protobuf.Archive.ListParameterHistoryRequest;
import org.yamcs.protobuf.Archive.ListParameterHistoryResponse;
import org.yamcs.protobuf.Pvalue.Ranges;
import org.yamcs.protobuf.Pvalue.TimeSeries;
@javax.annotation.processing.Generated(value = "org.yamcs.maven.ServiceGenerator", date = "2025-02-13T11:12:59.911635194Z")
public class ParameterArchiveApiClient extends AbstractParameterArchiveApi {
private final MethodHandler handler;
public ParameterArchiveApiClient(MethodHandler handler) {
this.handler = handler;
}
/**
*
* Rebuild range
*
* The back filler has to be enabled for this purpose. The back filling process does not
* remove data but just overwrites it. That means that if the parameter replay returns
* less parameters than originally stored in the archive, the old parameters will still
* be found in the archive.
*
* It also means that if a replay returns the parameter of a different type than
* originally stored, the old ones will still be stored. This is because the parameter
* archive treats parameter with the same name but different type as different parameters.
* Each of them is given an id and the id is stored in the archive.
*
*/
@Override
public final void rebuildRange(Void ctx, RebuildRangeRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(0),
request,
Empty.getDefaultInstance(),
observer);
}
/**
*
* Get parameter samples
*
* This divides the query interval in a number of intervals and returns aggregated
* statistics (max, min, avg) about each interval.
*
* This operation is useful when making high-level overviews (such as plots) of a
* parameter's value over large time intervals without having to retrieve each
* and every individual parameter value.
*
* By default this operation fetches data from the parameter archive and/or
* parameter cache. If these services are not configured, you can still get
* correct results by specifying the option ``source=replay`` as detailed below.
*
*/
@Override
public final void getParameterSamples(Void ctx, GetParameterSamplesRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(1),
request,
TimeSeries.getDefaultInstance(),
observer);
}
/**
*
* Get parameter ranges
*
* A range is a tuple ``(start, stop, value, count)`` that represents the time
* interval for which the parameter has been steadily coming in with the same
* value. This request is useful for retrieving an overview for parameters that
* change unfrequently in a large time interval. For example an on/off status
* of a device, or some operational status. Two consecutive ranges containing
* the same value will be returned if there was a gap in the data. The gap is
* determined according to the parameter expiration time configured in the
* Mission Database.
*
*/
@Override
public final void getParameterRanges(Void ctx, GetParameterRangesRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(2),
request,
Ranges.getDefaultInstance(),
observer);
}
/**
*
* List parameter history
*
*/
@Override
public final void listParameterHistory(Void ctx, ListParameterHistoryRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(3),
request,
ListParameterHistoryResponse.getDefaultInstance(),
observer);
}
/**
*
* Get information about the archived parameters.
*
* Each combination of (parameter name, raw type, engineering type) is assigned a
* unique parameter id.
*
* The parameters are grouped such that the samples of all parameters from one group
* have the same timestamp. For example all parameters extracted from one TM packet
* have usually the same timestamp and are part of the same group.
*
* Each group is assigned a unique group id.
*
* A parameter can be part of multiple groups. For instance a parameter appearing
* in the header of a packet is part of all groups made by inherited containers
* (i.e. each packet with that header will compose another group).
*
* For each group, the parameter archive stores one common record for the timestamps
* and individual records for the raw and engineering values of each parameter. If a
* parameter appears in multiple groups, retrieving its value means combining
* (time-based merge operation). The records beloging to the groups in which the
* parameter appears.
*
* The response to this method contains the parameter id, name, engineering type,
* raw type and the groups of which this parameter is part of.
*
*/
@Override
public final void getArchivedParametersInfo(Void ctx, GetArchivedParametersInfoRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(4),
request,
ArchivedParametersInfoResponse.getDefaultInstance(),
observer);
}
/**
*
* For a given parameter id, get the list of segments available for that parameter.
* A segment contains multiple samples (maximum ~70 minutes) of the same parameter.
*
*/
@Override
public final void getArchivedParameterSegments(Void ctx, GetArchivedParameterSegmentsRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(5),
request,
ArchivedParameterSegmentsResponse.getDefaultInstance(),
observer);
}
/**
*
* For a given group id, get the list of parameters which are part of the group
*
*/
@Override
public final void getArchivedParameterGroup(Void ctx, GetArchivedParameterGroupRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(6),
request,
ArchivedParameterGroupResponse.getDefaultInstance(),
observer);
}
/**
*
* Removes all the parameter archive data and related metadata. All the filling operations are stopped before
* and started after the purge.
*
* The rebuild operation has to be called to rebuild the past segments of the archive.
*
* Starting with Yamcs 5.9.0 the Parameter Archive is stored into a different column family ``parameter_archive``.
* Storing data into a separate column family gives better performance and the Parameter Archive rebuild operations
* are less disturbing for the other data (TM, TC, Events...). If the archive is from a previous version of Yamcs,
* the purge/rebuild can be used to move the parameter archive from the default column family to the separate column family.
*
*/
@Override
public final void purge(Void ctx, PurgeRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(7),
request,
Empty.getDefaultInstance(),
observer);
}
/**
*
* Disables the automatic backfilling (rebuilding) of the parameter archive.
* See :manual:`services/instance/parameter-archive-service/#backfiller-options`
*
* If the backfilling is already disabled, this operation has no effect.
* If there is a backfilling running, this call will not stop it.
*
* Manual rebuild operations are still accepted.
*
*
*/
@Override
public final void disableBackfilling(Void ctx, DisableBackfillingRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(8),
request,
Empty.getDefaultInstance(),
observer);
}
/**
*
* Enables the automatic backfilling (rebuilding) of the parameter archive.
* If the backfilling is already enabled, this operation has no effect.
*
*
*/
@Override
public final void enableBackfilling(Void ctx, EnableBackfillingRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(9),
request,
Empty.getDefaultInstance(),
observer);
}
/**
*
* Receive backfill notifications
*
*/
@Override
public final void subscribeBackfilling(Void ctx, SubscribeBackfillingRequest request, Observer observer) {
handler.call(
getDescriptorForType().getMethods().get(10),
request,
SubscribeBackfillingData.getDefaultInstance(),
observer);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy