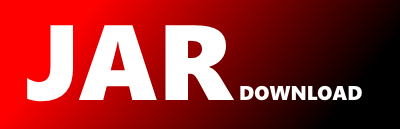
org.yamcs.protobuf.Table Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: yamcs/protobuf/table/table.proto
package org.yamcs.protobuf;
public final class Table {
private Table() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface RowOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.Row)
com.google.protobuf.MessageOrBuilder {
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
java.util.List
getColumnsList();
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
org.yamcs.protobuf.Table.Row.ColumnInfo getColumns(int index);
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
int getColumnsCount();
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
java.util.List extends org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder>
getColumnsOrBuilderList();
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder getColumnsOrBuilder(
int index);
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
java.util.List
getCellsList();
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
org.yamcs.protobuf.Table.Row.Cell getCells(int index);
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
int getCellsCount();
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
java.util.List extends org.yamcs.protobuf.Table.Row.CellOrBuilder>
getCellsOrBuilderList();
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
org.yamcs.protobuf.Table.Row.CellOrBuilder getCellsOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.table.Row}
*/
public static final class Row extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.Row)
RowOrBuilder {
private static final long serialVersionUID = 0L;
// Use Row.newBuilder() to construct.
private Row(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Row() {
columns_ = java.util.Collections.emptyList();
cells_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Row();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Row(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
columns_.add(
input.readMessage(org.yamcs.protobuf.Table.Row.ColumnInfo.PARSER, extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
cells_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
cells_.add(
input.readMessage(org.yamcs.protobuf.Table.Row.Cell.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
cells_ = java.util.Collections.unmodifiableList(cells_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.Row.class, org.yamcs.protobuf.Table.Row.Builder.class);
}
public interface ColumnInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.Row.ColumnInfo)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint32 id = 1;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* optional uint32 id = 1;
* @return The id.
*/
int getId();
/**
* optional string name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
* optional string name = 2;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional string type = 3;
* @return Whether the type field is set.
*/
boolean hasType();
/**
* optional string type = 3;
* @return The type.
*/
java.lang.String getType();
/**
* optional string type = 3;
* @return The bytes for type.
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return Whether the protoClass field is set.
*/
boolean hasProtoClass();
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return The protoClass.
*/
java.lang.String getProtoClass();
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return The bytes for protoClass.
*/
com.google.protobuf.ByteString
getProtoClassBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.Row.ColumnInfo}
*/
public static final class ColumnInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.Row.ColumnInfo)
ColumnInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ColumnInfo.newBuilder() to construct.
private ColumnInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ColumnInfo() {
name_ = "";
type_ = "";
protoClass_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ColumnInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ColumnInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readUInt32();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
type_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
protoClass_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_ColumnInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_ColumnInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.Row.ColumnInfo.class, org.yamcs.protobuf.Table.Row.ColumnInfo.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private int id_;
/**
* optional uint32 id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* optional string name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 3;
private volatile java.lang.Object type_;
/**
* optional string type = 3;
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string type = 3;
* @return The type.
*/
@java.lang.Override
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
}
}
/**
* optional string type = 3;
* @return The bytes for type.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROTOCLASS_FIELD_NUMBER = 4;
private volatile java.lang.Object protoClass_;
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return Whether the protoClass field is set.
*/
@java.lang.Override
public boolean hasProtoClass() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return The protoClass.
*/
@java.lang.Override
public java.lang.String getProtoClass() {
java.lang.Object ref = protoClass_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
protoClass_ = s;
}
return s;
}
}
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return The bytes for protoClass.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProtoClassBytes() {
java.lang.Object ref = protoClass_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protoClass_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeUInt32(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, type_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, protoClass_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, type_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, protoClass_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.Row.ColumnInfo)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.Row.ColumnInfo other = (org.yamcs.protobuf.Table.Row.ColumnInfo) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (!getType()
.equals(other.getType())) return false;
}
if (hasProtoClass() != other.hasProtoClass()) return false;
if (hasProtoClass()) {
if (!getProtoClass()
.equals(other.getProtoClass())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
if (hasProtoClass()) {
hash = (37 * hash) + PROTOCLASS_FIELD_NUMBER;
hash = (53 * hash) + getProtoClass().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.Row.ColumnInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.Row.ColumnInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.Row.ColumnInfo)
org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_ColumnInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_ColumnInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.Row.ColumnInfo.class, org.yamcs.protobuf.Table.Row.ColumnInfo.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.Row.ColumnInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
type_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
protoClass_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_ColumnInfo_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row.ColumnInfo getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.Row.ColumnInfo.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row.ColumnInfo build() {
org.yamcs.protobuf.Table.Row.ColumnInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row.ColumnInfo buildPartial() {
org.yamcs.protobuf.Table.Row.ColumnInfo result = new org.yamcs.protobuf.Table.Row.ColumnInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.protoClass_ = protoClass_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.Row.ColumnInfo) {
return mergeFrom((org.yamcs.protobuf.Table.Row.ColumnInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.Row.ColumnInfo other) {
if (other == org.yamcs.protobuf.Table.Row.ColumnInfo.getDefaultInstance()) return this;
if (other.hasId()) {
setId(other.getId());
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (other.hasType()) {
bitField0_ |= 0x00000004;
type_ = other.type_;
onChanged();
}
if (other.hasProtoClass()) {
bitField0_ |= 0x00000008;
protoClass_ = other.protoClass_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.Row.ColumnInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.Row.ColumnInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int id_ ;
/**
* optional uint32 id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
/**
* optional uint32 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(int value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional uint32 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 2;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
* optional string type = 3;
* @return Whether the type field is set.
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string type = 3;
* @return The type.
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string type = 3;
* @return The bytes for type.
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string type = 3;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
type_ = value;
onChanged();
return this;
}
/**
* optional string type = 3;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000004);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
* optional string type = 3;
* @param value The bytes for type to set.
* @return This builder for chaining.
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
type_ = value;
onChanged();
return this;
}
private java.lang.Object protoClass_ = "";
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return Whether the protoClass field is set.
*/
public boolean hasProtoClass() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return The protoClass.
*/
public java.lang.String getProtoClass() {
java.lang.Object ref = protoClass_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
protoClass_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return The bytes for protoClass.
*/
public com.google.protobuf.ByteString
getProtoClassBytes() {
java.lang.Object ref = protoClass_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protoClass_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @param value The protoClass to set.
* @return This builder for chaining.
*/
public Builder setProtoClass(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
protoClass_ = value;
onChanged();
return this;
}
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @return This builder for chaining.
*/
public Builder clearProtoClass() {
bitField0_ = (bitField0_ & ~0x00000008);
protoClass_ = getDefaultInstance().getProtoClass();
onChanged();
return this;
}
/**
*
* The name of the class implementing the proto object if dataType is PROTOBUF
*
*
* optional string protoClass = 4;
* @param value The bytes for protoClass to set.
* @return This builder for chaining.
*/
public Builder setProtoClassBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
protoClass_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.Row.ColumnInfo)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.Row.ColumnInfo)
private static final org.yamcs.protobuf.Table.Row.ColumnInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.Row.ColumnInfo();
}
public static org.yamcs.protobuf.Table.Row.ColumnInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ColumnInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ColumnInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row.ColumnInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CellOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.Row.Cell)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint32 columnId = 1;
* @return Whether the columnId field is set.
*/
boolean hasColumnId();
/**
* optional uint32 columnId = 1;
* @return The columnId.
*/
int getColumnId();
/**
* optional bytes data = 2;
* @return Whether the data field is set.
*/
boolean hasData();
/**
* optional bytes data = 2;
* @return The data.
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code yamcs.protobuf.table.Row.Cell}
*/
public static final class Cell extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.Row.Cell)
CellOrBuilder {
private static final long serialVersionUID = 0L;
// Use Cell.newBuilder() to construct.
private Cell(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Cell() {
data_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Cell();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Cell(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
columnId_ = input.readUInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
data_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_Cell_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_Cell_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.Row.Cell.class, org.yamcs.protobuf.Table.Row.Cell.Builder.class);
}
private int bitField0_;
public static final int COLUMNID_FIELD_NUMBER = 1;
private int columnId_;
/**
* optional uint32 columnId = 1;
* @return Whether the columnId field is set.
*/
@java.lang.Override
public boolean hasColumnId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional uint32 columnId = 1;
* @return The columnId.
*/
@java.lang.Override
public int getColumnId() {
return columnId_;
}
public static final int DATA_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString data_;
/**
* optional bytes data = 2;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes data = 2;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeUInt32(1, columnId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, data_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, columnId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, data_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.Row.Cell)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.Row.Cell other = (org.yamcs.protobuf.Table.Row.Cell) obj;
if (hasColumnId() != other.hasColumnId()) return false;
if (hasColumnId()) {
if (getColumnId()
!= other.getColumnId()) return false;
}
if (hasData() != other.hasData()) return false;
if (hasData()) {
if (!getData()
.equals(other.getData())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasColumnId()) {
hash = (37 * hash) + COLUMNID_FIELD_NUMBER;
hash = (53 * hash) + getColumnId();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.Cell parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.Row.Cell parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.Row.Cell parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.Row.Cell prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.Row.Cell}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.Row.Cell)
org.yamcs.protobuf.Table.Row.CellOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_Cell_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_Cell_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.Row.Cell.class, org.yamcs.protobuf.Table.Row.Cell.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.Row.Cell.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
columnId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_Cell_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row.Cell getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.Row.Cell.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row.Cell build() {
org.yamcs.protobuf.Table.Row.Cell result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row.Cell buildPartial() {
org.yamcs.protobuf.Table.Row.Cell result = new org.yamcs.protobuf.Table.Row.Cell(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.columnId_ = columnId_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.data_ = data_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.Row.Cell) {
return mergeFrom((org.yamcs.protobuf.Table.Row.Cell)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.Row.Cell other) {
if (other == org.yamcs.protobuf.Table.Row.Cell.getDefaultInstance()) return this;
if (other.hasColumnId()) {
setColumnId(other.getColumnId());
}
if (other.hasData()) {
setData(other.getData());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.Row.Cell parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.Row.Cell) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int columnId_ ;
/**
* optional uint32 columnId = 1;
* @return Whether the columnId field is set.
*/
@java.lang.Override
public boolean hasColumnId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional uint32 columnId = 1;
* @return The columnId.
*/
@java.lang.Override
public int getColumnId() {
return columnId_;
}
/**
* optional uint32 columnId = 1;
* @param value The columnId to set.
* @return This builder for chaining.
*/
public Builder setColumnId(int value) {
bitField0_ |= 0x00000001;
columnId_ = value;
onChanged();
return this;
}
/**
* optional uint32 columnId = 1;
* @return This builder for chaining.
*/
public Builder clearColumnId() {
bitField0_ = (bitField0_ & ~0x00000001);
columnId_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes data = 2;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes data = 2;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* optional bytes data = 2;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
data_ = value;
onChanged();
return this;
}
/**
* optional bytes data = 2;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000002);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.Row.Cell)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.Row.Cell)
private static final org.yamcs.protobuf.Table.Row.Cell DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.Row.Cell();
}
public static org.yamcs.protobuf.Table.Row.Cell getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Cell parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Cell(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row.Cell getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int COLUMNS_FIELD_NUMBER = 1;
private java.util.List columns_;
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
@java.lang.Override
public java.util.List getColumnsList() {
return columns_;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder>
getColumnsOrBuilderList() {
return columns_;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
@java.lang.Override
public int getColumnsCount() {
return columns_.size();
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.Row.ColumnInfo getColumns(int index) {
return columns_.get(index);
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder getColumnsOrBuilder(
int index) {
return columns_.get(index);
}
public static final int CELLS_FIELD_NUMBER = 2;
private java.util.List cells_;
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
@java.lang.Override
public java.util.List getCellsList() {
return cells_;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.Row.CellOrBuilder>
getCellsOrBuilderList() {
return cells_;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
@java.lang.Override
public int getCellsCount() {
return cells_.size();
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.Row.Cell getCells(int index) {
return cells_.get(index);
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.Row.CellOrBuilder getCellsOrBuilder(
int index) {
return cells_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < columns_.size(); i++) {
output.writeMessage(1, columns_.get(i));
}
for (int i = 0; i < cells_.size(); i++) {
output.writeMessage(2, cells_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < columns_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, columns_.get(i));
}
for (int i = 0; i < cells_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, cells_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.Row)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.Row other = (org.yamcs.protobuf.Table.Row) obj;
if (!getColumnsList()
.equals(other.getColumnsList())) return false;
if (!getCellsList()
.equals(other.getCellsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getColumnsCount() > 0) {
hash = (37 * hash) + COLUMNS_FIELD_NUMBER;
hash = (53 * hash) + getColumnsList().hashCode();
}
if (getCellsCount() > 0) {
hash = (37 * hash) + CELLS_FIELD_NUMBER;
hash = (53 * hash) + getCellsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.Row parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.Row parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.Row parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.Row parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.Row parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.Row parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.Row parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.Row parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.Row prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.Row}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.Row)
org.yamcs.protobuf.Table.RowOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.Row.class, org.yamcs.protobuf.Table.Row.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.Row.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getColumnsFieldBuilder();
getCellsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
columnsBuilder_.clear();
}
if (cellsBuilder_ == null) {
cells_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
cellsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_Row_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.Row.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row build() {
org.yamcs.protobuf.Table.Row result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row buildPartial() {
org.yamcs.protobuf.Table.Row result = new org.yamcs.protobuf.Table.Row(this);
int from_bitField0_ = bitField0_;
if (columnsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.columns_ = columns_;
} else {
result.columns_ = columnsBuilder_.build();
}
if (cellsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
cells_ = java.util.Collections.unmodifiableList(cells_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.cells_ = cells_;
} else {
result.cells_ = cellsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.Row) {
return mergeFrom((org.yamcs.protobuf.Table.Row)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.Row other) {
if (other == org.yamcs.protobuf.Table.Row.getDefaultInstance()) return this;
if (columnsBuilder_ == null) {
if (!other.columns_.isEmpty()) {
if (columns_.isEmpty()) {
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureColumnsIsMutable();
columns_.addAll(other.columns_);
}
onChanged();
}
} else {
if (!other.columns_.isEmpty()) {
if (columnsBuilder_.isEmpty()) {
columnsBuilder_.dispose();
columnsBuilder_ = null;
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
columnsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getColumnsFieldBuilder() : null;
} else {
columnsBuilder_.addAllMessages(other.columns_);
}
}
}
if (cellsBuilder_ == null) {
if (!other.cells_.isEmpty()) {
if (cells_.isEmpty()) {
cells_ = other.cells_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureCellsIsMutable();
cells_.addAll(other.cells_);
}
onChanged();
}
} else {
if (!other.cells_.isEmpty()) {
if (cellsBuilder_.isEmpty()) {
cellsBuilder_.dispose();
cellsBuilder_ = null;
cells_ = other.cells_;
bitField0_ = (bitField0_ & ~0x00000002);
cellsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCellsFieldBuilder() : null;
} else {
cellsBuilder_.addAllMessages(other.cells_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.Row parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.Row) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List columns_ =
java.util.Collections.emptyList();
private void ensureColumnsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList(columns_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.Row.ColumnInfo, org.yamcs.protobuf.Table.Row.ColumnInfo.Builder, org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder> columnsBuilder_;
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public java.util.List getColumnsList() {
if (columnsBuilder_ == null) {
return java.util.Collections.unmodifiableList(columns_);
} else {
return columnsBuilder_.getMessageList();
}
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public int getColumnsCount() {
if (columnsBuilder_ == null) {
return columns_.size();
} else {
return columnsBuilder_.getCount();
}
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.Row.ColumnInfo getColumns(int index) {
if (columnsBuilder_ == null) {
return columns_.get(index);
} else {
return columnsBuilder_.getMessage(index);
}
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public Builder setColumns(
int index, org.yamcs.protobuf.Table.Row.ColumnInfo value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.set(index, value);
onChanged();
} else {
columnsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public Builder setColumns(
int index, org.yamcs.protobuf.Table.Row.ColumnInfo.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.set(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public Builder addColumns(org.yamcs.protobuf.Table.Row.ColumnInfo value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(value);
onChanged();
} else {
columnsBuilder_.addMessage(value);
}
return this;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public Builder addColumns(
int index, org.yamcs.protobuf.Table.Row.ColumnInfo value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(index, value);
onChanged();
} else {
columnsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public Builder addColumns(
org.yamcs.protobuf.Table.Row.ColumnInfo.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public Builder addColumns(
int index, org.yamcs.protobuf.Table.Row.ColumnInfo.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public Builder addAllColumns(
java.lang.Iterable extends org.yamcs.protobuf.Table.Row.ColumnInfo> values) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, columns_);
onChanged();
} else {
columnsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public Builder clearColumns() {
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
columnsBuilder_.clear();
}
return this;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public Builder removeColumns(int index) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.remove(index);
onChanged();
} else {
columnsBuilder_.remove(index);
}
return this;
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.Row.ColumnInfo.Builder getColumnsBuilder(
int index) {
return getColumnsFieldBuilder().getBuilder(index);
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder getColumnsOrBuilder(
int index) {
if (columnsBuilder_ == null) {
return columns_.get(index); } else {
return columnsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public java.util.List extends org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder>
getColumnsOrBuilderList() {
if (columnsBuilder_ != null) {
return columnsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(columns_);
}
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.Row.ColumnInfo.Builder addColumnsBuilder() {
return getColumnsFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.Row.ColumnInfo.getDefaultInstance());
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.Row.ColumnInfo.Builder addColumnsBuilder(
int index) {
return getColumnsFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.Row.ColumnInfo.getDefaultInstance());
}
/**
*
*the column info is only present for new columns in a stream of Row messages
*
*
* repeated .yamcs.protobuf.table.Row.ColumnInfo columns = 1;
*/
public java.util.List
getColumnsBuilderList() {
return getColumnsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.Row.ColumnInfo, org.yamcs.protobuf.Table.Row.ColumnInfo.Builder, org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder>
getColumnsFieldBuilder() {
if (columnsBuilder_ == null) {
columnsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.Row.ColumnInfo, org.yamcs.protobuf.Table.Row.ColumnInfo.Builder, org.yamcs.protobuf.Table.Row.ColumnInfoOrBuilder>(
columns_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
columns_ = null;
}
return columnsBuilder_;
}
private java.util.List cells_ =
java.util.Collections.emptyList();
private void ensureCellsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
cells_ = new java.util.ArrayList(cells_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.Row.Cell, org.yamcs.protobuf.Table.Row.Cell.Builder, org.yamcs.protobuf.Table.Row.CellOrBuilder> cellsBuilder_;
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public java.util.List getCellsList() {
if (cellsBuilder_ == null) {
return java.util.Collections.unmodifiableList(cells_);
} else {
return cellsBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public int getCellsCount() {
if (cellsBuilder_ == null) {
return cells_.size();
} else {
return cellsBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public org.yamcs.protobuf.Table.Row.Cell getCells(int index) {
if (cellsBuilder_ == null) {
return cells_.get(index);
} else {
return cellsBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public Builder setCells(
int index, org.yamcs.protobuf.Table.Row.Cell value) {
if (cellsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCellsIsMutable();
cells_.set(index, value);
onChanged();
} else {
cellsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public Builder setCells(
int index, org.yamcs.protobuf.Table.Row.Cell.Builder builderForValue) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
cells_.set(index, builderForValue.build());
onChanged();
} else {
cellsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public Builder addCells(org.yamcs.protobuf.Table.Row.Cell value) {
if (cellsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCellsIsMutable();
cells_.add(value);
onChanged();
} else {
cellsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public Builder addCells(
int index, org.yamcs.protobuf.Table.Row.Cell value) {
if (cellsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCellsIsMutable();
cells_.add(index, value);
onChanged();
} else {
cellsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public Builder addCells(
org.yamcs.protobuf.Table.Row.Cell.Builder builderForValue) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
cells_.add(builderForValue.build());
onChanged();
} else {
cellsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public Builder addCells(
int index, org.yamcs.protobuf.Table.Row.Cell.Builder builderForValue) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
cells_.add(index, builderForValue.build());
onChanged();
} else {
cellsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public Builder addAllCells(
java.lang.Iterable extends org.yamcs.protobuf.Table.Row.Cell> values) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cells_);
onChanged();
} else {
cellsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public Builder clearCells() {
if (cellsBuilder_ == null) {
cells_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
cellsBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public Builder removeCells(int index) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
cells_.remove(index);
onChanged();
} else {
cellsBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public org.yamcs.protobuf.Table.Row.Cell.Builder getCellsBuilder(
int index) {
return getCellsFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public org.yamcs.protobuf.Table.Row.CellOrBuilder getCellsOrBuilder(
int index) {
if (cellsBuilder_ == null) {
return cells_.get(index); } else {
return cellsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public java.util.List extends org.yamcs.protobuf.Table.Row.CellOrBuilder>
getCellsOrBuilderList() {
if (cellsBuilder_ != null) {
return cellsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(cells_);
}
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public org.yamcs.protobuf.Table.Row.Cell.Builder addCellsBuilder() {
return getCellsFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.Row.Cell.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public org.yamcs.protobuf.Table.Row.Cell.Builder addCellsBuilder(
int index) {
return getCellsFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.Row.Cell.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.Row.Cell cells = 2;
*/
public java.util.List
getCellsBuilderList() {
return getCellsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.Row.Cell, org.yamcs.protobuf.Table.Row.Cell.Builder, org.yamcs.protobuf.Table.Row.CellOrBuilder>
getCellsFieldBuilder() {
if (cellsBuilder_ == null) {
cellsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.Row.Cell, org.yamcs.protobuf.Table.Row.Cell.Builder, org.yamcs.protobuf.Table.Row.CellOrBuilder>(
cells_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
cells_ = null;
}
return cellsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.Row)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.Row)
private static final org.yamcs.protobuf.Table.Row DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.Row();
}
public static org.yamcs.protobuf.Table.Row getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Row parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Row(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.Row getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReadRowsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ReadRowsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
*
* Table name.
*
*
* optional string table = 2;
* @return Whether the table field is set.
*/
boolean hasTable();
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The table.
*/
java.lang.String getTable();
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The bytes for table.
*/
com.google.protobuf.ByteString
getTableBytes();
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return A list containing the cols.
*/
java.util.List
getColsList();
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return The count of cols.
*/
int getColsCount();
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the element to return.
* @return The cols at the given index.
*/
java.lang.String getCols(int index);
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the value to return.
* @return The bytes of the cols at the given index.
*/
com.google.protobuf.ByteString
getColsBytes(int index);
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return Whether the query field is set.
*/
boolean hasQuery();
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return The query.
*/
java.lang.String getQuery();
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return The bytes for query.
*/
com.google.protobuf.ByteString
getQueryBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.ReadRowsRequest}
*/
public static final class ReadRowsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ReadRowsRequest)
ReadRowsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReadRowsRequest.newBuilder() to construct.
private ReadRowsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReadRowsRequest() {
instance_ = "";
table_ = "";
cols_ = com.google.protobuf.LazyStringArrayList.EMPTY;
query_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ReadRowsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ReadRowsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
table_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
cols_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000004;
}
cols_.add(bs);
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
query_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) != 0)) {
cols_ = cols_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ReadRowsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ReadRowsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ReadRowsRequest.class, org.yamcs.protobuf.Table.ReadRowsRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_FIELD_NUMBER = 2;
private volatile java.lang.Object table_;
/**
*
* Table name.
*
*
* optional string table = 2;
* @return Whether the table field is set.
*/
@java.lang.Override
public boolean hasTable() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The table.
*/
@java.lang.Override
public java.lang.String getTable() {
java.lang.Object ref = table_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
table_ = s;
}
return s;
}
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The bytes for table.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableBytes() {
java.lang.Object ref = table_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
table_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COLS_FIELD_NUMBER = 3;
private com.google.protobuf.LazyStringList cols_;
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return A list containing the cols.
*/
public com.google.protobuf.ProtocolStringList
getColsList() {
return cols_;
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return The count of cols.
*/
public int getColsCount() {
return cols_.size();
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the element to return.
* @return The cols at the given index.
*/
public java.lang.String getCols(int index) {
return cols_.get(index);
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the value to return.
* @return The bytes of the cols at the given index.
*/
public com.google.protobuf.ByteString
getColsBytes(int index) {
return cols_.getByteString(index);
}
public static final int QUERY_FIELD_NUMBER = 4;
private volatile java.lang.Object query_;
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return Whether the query field is set.
*/
@java.lang.Override
public boolean hasQuery() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return The query.
*/
@java.lang.Override
public java.lang.String getQuery() {
java.lang.Object ref = query_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
query_ = s;
}
return s;
}
}
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return The bytes for query.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getQueryBytes() {
java.lang.Object ref = query_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
query_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, table_);
}
for (int i = 0; i < cols_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, cols_.getRaw(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, query_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, table_);
}
{
int dataSize = 0;
for (int i = 0; i < cols_.size(); i++) {
dataSize += computeStringSizeNoTag(cols_.getRaw(i));
}
size += dataSize;
size += 1 * getColsList().size();
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, query_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ReadRowsRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ReadRowsRequest other = (org.yamcs.protobuf.Table.ReadRowsRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasTable() != other.hasTable()) return false;
if (hasTable()) {
if (!getTable()
.equals(other.getTable())) return false;
}
if (!getColsList()
.equals(other.getColsList())) return false;
if (hasQuery() != other.hasQuery()) return false;
if (hasQuery()) {
if (!getQuery()
.equals(other.getQuery())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasTable()) {
hash = (37 * hash) + TABLE_FIELD_NUMBER;
hash = (53 * hash) + getTable().hashCode();
}
if (getColsCount() > 0) {
hash = (37 * hash) + COLS_FIELD_NUMBER;
hash = (53 * hash) + getColsList().hashCode();
}
if (hasQuery()) {
hash = (37 * hash) + QUERY_FIELD_NUMBER;
hash = (53 * hash) + getQuery().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ReadRowsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ReadRowsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ReadRowsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ReadRowsRequest)
org.yamcs.protobuf.Table.ReadRowsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ReadRowsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ReadRowsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ReadRowsRequest.class, org.yamcs.protobuf.Table.ReadRowsRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ReadRowsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
table_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
cols_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
query_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ReadRowsRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ReadRowsRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ReadRowsRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ReadRowsRequest build() {
org.yamcs.protobuf.Table.ReadRowsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ReadRowsRequest buildPartial() {
org.yamcs.protobuf.Table.ReadRowsRequest result = new org.yamcs.protobuf.Table.ReadRowsRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.table_ = table_;
if (((bitField0_ & 0x00000004) != 0)) {
cols_ = cols_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.cols_ = cols_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.query_ = query_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ReadRowsRequest) {
return mergeFrom((org.yamcs.protobuf.Table.ReadRowsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ReadRowsRequest other) {
if (other == org.yamcs.protobuf.Table.ReadRowsRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
if (other.hasTable()) {
bitField0_ |= 0x00000002;
table_ = other.table_;
onChanged();
}
if (!other.cols_.isEmpty()) {
if (cols_.isEmpty()) {
cols_ = other.cols_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureColsIsMutable();
cols_.addAll(other.cols_);
}
onChanged();
}
if (other.hasQuery()) {
bitField0_ |= 0x00000008;
query_ = other.query_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ReadRowsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ReadRowsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
private java.lang.Object table_ = "";
/**
*
* Table name.
*
*
* optional string table = 2;
* @return Whether the table field is set.
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The table.
*/
public java.lang.String getTable() {
java.lang.Object ref = table_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
table_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The bytes for table.
*/
public com.google.protobuf.ByteString
getTableBytes() {
java.lang.Object ref = table_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
table_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @param value The table to set.
* @return This builder for chaining.
*/
public Builder setTable(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
table_ = value;
onChanged();
return this;
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return This builder for chaining.
*/
public Builder clearTable() {
bitField0_ = (bitField0_ & ~0x00000002);
table_ = getDefaultInstance().getTable();
onChanged();
return this;
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @param value The bytes for table to set.
* @return This builder for chaining.
*/
public Builder setTableBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
table_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList cols_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureColsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
cols_ = new com.google.protobuf.LazyStringArrayList(cols_);
bitField0_ |= 0x00000004;
}
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return A list containing the cols.
*/
public com.google.protobuf.ProtocolStringList
getColsList() {
return cols_.getUnmodifiableView();
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return The count of cols.
*/
public int getColsCount() {
return cols_.size();
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the element to return.
* @return The cols at the given index.
*/
public java.lang.String getCols(int index) {
return cols_.get(index);
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the value to return.
* @return The bytes of the cols at the given index.
*/
public com.google.protobuf.ByteString
getColsBytes(int index) {
return cols_.getByteString(index);
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index to set the value at.
* @param value The cols to set.
* @return This builder for chaining.
*/
public Builder setCols(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureColsIsMutable();
cols_.set(index, value);
onChanged();
return this;
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param value The cols to add.
* @return This builder for chaining.
*/
public Builder addCols(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureColsIsMutable();
cols_.add(value);
onChanged();
return this;
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param values The cols to add.
* @return This builder for chaining.
*/
public Builder addAllCols(
java.lang.Iterable values) {
ensureColsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cols_);
onChanged();
return this;
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return This builder for chaining.
*/
public Builder clearCols() {
cols_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* The columns to be included in the result. If unspecified, all
* table and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param value The bytes of the cols to add.
* @return This builder for chaining.
*/
public Builder addColsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureColsIsMutable();
cols_.add(value);
onChanged();
return this;
}
private java.lang.Object query_ = "";
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return Whether the query field is set.
*/
public boolean hasQuery() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return The query.
*/
public java.lang.String getQuery() {
java.lang.Object ref = query_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
query_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return The bytes for query.
*/
public com.google.protobuf.ByteString
getQueryBytes() {
java.lang.Object ref = query_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
query_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @param value The query to set.
* @return This builder for chaining.
*/
public Builder setQuery(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
query_ = value;
onChanged();
return this;
}
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @return This builder for chaining.
*/
public Builder clearQuery() {
bitField0_ = (bitField0_ & ~0x00000008);
query_ = getDefaultInstance().getQuery();
onChanged();
return this;
}
/**
*
* Limit the results by specifying a SQL WHERE clause.
* Examples:
* - pname = '/YSS/SIMULATOR/FlightData'
* - gentime > '2023-01-01T00:00:00.000Z'
* - pname = '/YSS/SIMULATOR/FlightData' and gentime > '2023-01-01T00:00:00.000Z'
*
*
* optional string query = 4;
* @param value The bytes for query to set.
* @return This builder for chaining.
*/
public Builder setQueryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
query_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ReadRowsRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ReadRowsRequest)
private static final org.yamcs.protobuf.Table.ReadRowsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ReadRowsRequest();
}
public static org.yamcs.protobuf.Table.ReadRowsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ReadRowsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ReadRowsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ReadRowsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WriteRowsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.WriteRowsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
*
* Table name.
*
*
* optional string table = 2;
* @return Whether the table field is set.
*/
boolean hasTable();
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The table.
*/
java.lang.String getTable();
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The bytes for table.
*/
com.google.protobuf.ByteString
getTableBytes();
/**
* optional .yamcs.protobuf.table.Row row = 3;
* @return Whether the row field is set.
*/
boolean hasRow();
/**
* optional .yamcs.protobuf.table.Row row = 3;
* @return The row.
*/
org.yamcs.protobuf.Table.Row getRow();
/**
* optional .yamcs.protobuf.table.Row row = 3;
*/
org.yamcs.protobuf.Table.RowOrBuilder getRowOrBuilder();
}
/**
* Protobuf type {@code yamcs.protobuf.table.WriteRowsRequest}
*/
public static final class WriteRowsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.WriteRowsRequest)
WriteRowsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use WriteRowsRequest.newBuilder() to construct.
private WriteRowsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WriteRowsRequest() {
instance_ = "";
table_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WriteRowsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WriteRowsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
table_ = bs;
break;
}
case 26: {
org.yamcs.protobuf.Table.Row.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) != 0)) {
subBuilder = row_.toBuilder();
}
row_ = input.readMessage(org.yamcs.protobuf.Table.Row.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(row_);
row_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.WriteRowsRequest.class, org.yamcs.protobuf.Table.WriteRowsRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_FIELD_NUMBER = 2;
private volatile java.lang.Object table_;
/**
*
* Table name.
*
*
* optional string table = 2;
* @return Whether the table field is set.
*/
@java.lang.Override
public boolean hasTable() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The table.
*/
@java.lang.Override
public java.lang.String getTable() {
java.lang.Object ref = table_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
table_ = s;
}
return s;
}
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The bytes for table.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableBytes() {
java.lang.Object ref = table_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
table_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROW_FIELD_NUMBER = 3;
private org.yamcs.protobuf.Table.Row row_;
/**
* optional .yamcs.protobuf.table.Row row = 3;
* @return Whether the row field is set.
*/
@java.lang.Override
public boolean hasRow() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
* @return The row.
*/
@java.lang.Override
public org.yamcs.protobuf.Table.Row getRow() {
return row_ == null ? org.yamcs.protobuf.Table.Row.getDefaultInstance() : row_;
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.RowOrBuilder getRowOrBuilder() {
return row_ == null ? org.yamcs.protobuf.Table.Row.getDefaultInstance() : row_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, table_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(3, getRow());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, table_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getRow());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.WriteRowsRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.WriteRowsRequest other = (org.yamcs.protobuf.Table.WriteRowsRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasTable() != other.hasTable()) return false;
if (hasTable()) {
if (!getTable()
.equals(other.getTable())) return false;
}
if (hasRow() != other.hasRow()) return false;
if (hasRow()) {
if (!getRow()
.equals(other.getRow())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasTable()) {
hash = (37 * hash) + TABLE_FIELD_NUMBER;
hash = (53 * hash) + getTable().hashCode();
}
if (hasRow()) {
hash = (37 * hash) + ROW_FIELD_NUMBER;
hash = (53 * hash) + getRow().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.WriteRowsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.WriteRowsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.WriteRowsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.WriteRowsRequest)
org.yamcs.protobuf.Table.WriteRowsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.WriteRowsRequest.class, org.yamcs.protobuf.Table.WriteRowsRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.WriteRowsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRowFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
table_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
if (rowBuilder_ == null) {
row_ = null;
} else {
rowBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.WriteRowsRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsRequest build() {
org.yamcs.protobuf.Table.WriteRowsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsRequest buildPartial() {
org.yamcs.protobuf.Table.WriteRowsRequest result = new org.yamcs.protobuf.Table.WriteRowsRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.table_ = table_;
if (((from_bitField0_ & 0x00000004) != 0)) {
if (rowBuilder_ == null) {
result.row_ = row_;
} else {
result.row_ = rowBuilder_.build();
}
to_bitField0_ |= 0x00000004;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.WriteRowsRequest) {
return mergeFrom((org.yamcs.protobuf.Table.WriteRowsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.WriteRowsRequest other) {
if (other == org.yamcs.protobuf.Table.WriteRowsRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
if (other.hasTable()) {
bitField0_ |= 0x00000002;
table_ = other.table_;
onChanged();
}
if (other.hasRow()) {
mergeRow(other.getRow());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.WriteRowsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.WriteRowsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
private java.lang.Object table_ = "";
/**
*
* Table name.
*
*
* optional string table = 2;
* @return Whether the table field is set.
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The table.
*/
public java.lang.String getTable() {
java.lang.Object ref = table_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
table_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return The bytes for table.
*/
public com.google.protobuf.ByteString
getTableBytes() {
java.lang.Object ref = table_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
table_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @param value The table to set.
* @return This builder for chaining.
*/
public Builder setTable(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
table_ = value;
onChanged();
return this;
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @return This builder for chaining.
*/
public Builder clearTable() {
bitField0_ = (bitField0_ & ~0x00000002);
table_ = getDefaultInstance().getTable();
onChanged();
return this;
}
/**
*
* Table name.
*
*
* optional string table = 2;
* @param value The bytes for table to set.
* @return This builder for chaining.
*/
public Builder setTableBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
table_ = value;
onChanged();
return this;
}
private org.yamcs.protobuf.Table.Row row_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Table.Row, org.yamcs.protobuf.Table.Row.Builder, org.yamcs.protobuf.Table.RowOrBuilder> rowBuilder_;
/**
* optional .yamcs.protobuf.table.Row row = 3;
* @return Whether the row field is set.
*/
public boolean hasRow() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
* @return The row.
*/
public org.yamcs.protobuf.Table.Row getRow() {
if (rowBuilder_ == null) {
return row_ == null ? org.yamcs.protobuf.Table.Row.getDefaultInstance() : row_;
} else {
return rowBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
*/
public Builder setRow(org.yamcs.protobuf.Table.Row value) {
if (rowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
row_ = value;
onChanged();
} else {
rowBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
*/
public Builder setRow(
org.yamcs.protobuf.Table.Row.Builder builderForValue) {
if (rowBuilder_ == null) {
row_ = builderForValue.build();
onChanged();
} else {
rowBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
*/
public Builder mergeRow(org.yamcs.protobuf.Table.Row value) {
if (rowBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
row_ != null &&
row_ != org.yamcs.protobuf.Table.Row.getDefaultInstance()) {
row_ =
org.yamcs.protobuf.Table.Row.newBuilder(row_).mergeFrom(value).buildPartial();
} else {
row_ = value;
}
onChanged();
} else {
rowBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
*/
public Builder clearRow() {
if (rowBuilder_ == null) {
row_ = null;
onChanged();
} else {
rowBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
*/
public org.yamcs.protobuf.Table.Row.Builder getRowBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getRowFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
*/
public org.yamcs.protobuf.Table.RowOrBuilder getRowOrBuilder() {
if (rowBuilder_ != null) {
return rowBuilder_.getMessageOrBuilder();
} else {
return row_ == null ?
org.yamcs.protobuf.Table.Row.getDefaultInstance() : row_;
}
}
/**
* optional .yamcs.protobuf.table.Row row = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Table.Row, org.yamcs.protobuf.Table.Row.Builder, org.yamcs.protobuf.Table.RowOrBuilder>
getRowFieldBuilder() {
if (rowBuilder_ == null) {
rowBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Table.Row, org.yamcs.protobuf.Table.Row.Builder, org.yamcs.protobuf.Table.RowOrBuilder>(
getRow(),
getParentForChildren(),
isClean());
row_ = null;
}
return rowBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.WriteRowsRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.WriteRowsRequest)
private static final org.yamcs.protobuf.Table.WriteRowsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.WriteRowsRequest();
}
public static org.yamcs.protobuf.Table.WriteRowsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WriteRowsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WriteRowsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WriteRowsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.WriteRowsResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* The number of rows that were written
*
*
* optional uint32 count = 1;
* @return Whether the count field is set.
*/
boolean hasCount();
/**
*
* The number of rows that were written
*
*
* optional uint32 count = 1;
* @return The count.
*/
int getCount();
}
/**
* Protobuf type {@code yamcs.protobuf.table.WriteRowsResponse}
*/
public static final class WriteRowsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.WriteRowsResponse)
WriteRowsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use WriteRowsResponse.newBuilder() to construct.
private WriteRowsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WriteRowsResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WriteRowsResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WriteRowsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
count_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.WriteRowsResponse.class, org.yamcs.protobuf.Table.WriteRowsResponse.Builder.class);
}
private int bitField0_;
public static final int COUNT_FIELD_NUMBER = 1;
private int count_;
/**
*
* The number of rows that were written
*
*
* optional uint32 count = 1;
* @return Whether the count field is set.
*/
@java.lang.Override
public boolean hasCount() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The number of rows that were written
*
*
* optional uint32 count = 1;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeUInt32(1, count_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, count_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.WriteRowsResponse)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.WriteRowsResponse other = (org.yamcs.protobuf.Table.WriteRowsResponse) obj;
if (hasCount() != other.hasCount()) return false;
if (hasCount()) {
if (getCount()
!= other.getCount()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCount()) {
hash = (37 * hash) + COUNT_FIELD_NUMBER;
hash = (53 * hash) + getCount();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.WriteRowsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.WriteRowsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.WriteRowsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.WriteRowsResponse)
org.yamcs.protobuf.Table.WriteRowsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.WriteRowsResponse.class, org.yamcs.protobuf.Table.WriteRowsResponse.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.WriteRowsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
count_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsResponse_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsResponse getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.WriteRowsResponse.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsResponse build() {
org.yamcs.protobuf.Table.WriteRowsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsResponse buildPartial() {
org.yamcs.protobuf.Table.WriteRowsResponse result = new org.yamcs.protobuf.Table.WriteRowsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.count_ = count_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.WriteRowsResponse) {
return mergeFrom((org.yamcs.protobuf.Table.WriteRowsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.WriteRowsResponse other) {
if (other == org.yamcs.protobuf.Table.WriteRowsResponse.getDefaultInstance()) return this;
if (other.hasCount()) {
setCount(other.getCount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.WriteRowsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.WriteRowsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int count_ ;
/**
*
* The number of rows that were written
*
*
* optional uint32 count = 1;
* @return Whether the count field is set.
*/
@java.lang.Override
public boolean hasCount() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The number of rows that were written
*
*
* optional uint32 count = 1;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
/**
*
* The number of rows that were written
*
*
* optional uint32 count = 1;
* @param value The count to set.
* @return This builder for chaining.
*/
public Builder setCount(int value) {
bitField0_ |= 0x00000001;
count_ = value;
onChanged();
return this;
}
/**
*
* The number of rows that were written
*
*
* optional uint32 count = 1;
* @return This builder for chaining.
*/
public Builder clearCount() {
bitField0_ = (bitField0_ & ~0x00000001);
count_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.WriteRowsResponse)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.WriteRowsResponse)
private static final org.yamcs.protobuf.Table.WriteRowsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.WriteRowsResponse();
}
public static org.yamcs.protobuf.Table.WriteRowsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WriteRowsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WriteRowsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WriteRowsExceptionDetailOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.WriteRowsExceptionDetail)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint32 count = 1;
* @return Whether the count field is set.
*/
boolean hasCount();
/**
* optional uint32 count = 1;
* @return The count.
*/
int getCount();
}
/**
* Protobuf type {@code yamcs.protobuf.table.WriteRowsExceptionDetail}
*/
public static final class WriteRowsExceptionDetail extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.WriteRowsExceptionDetail)
WriteRowsExceptionDetailOrBuilder {
private static final long serialVersionUID = 0L;
// Use WriteRowsExceptionDetail.newBuilder() to construct.
private WriteRowsExceptionDetail(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WriteRowsExceptionDetail() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WriteRowsExceptionDetail();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WriteRowsExceptionDetail(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
count_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsExceptionDetail_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsExceptionDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.WriteRowsExceptionDetail.class, org.yamcs.protobuf.Table.WriteRowsExceptionDetail.Builder.class);
}
private int bitField0_;
public static final int COUNT_FIELD_NUMBER = 1;
private int count_;
/**
* optional uint32 count = 1;
* @return Whether the count field is set.
*/
@java.lang.Override
public boolean hasCount() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional uint32 count = 1;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeUInt32(1, count_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, count_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.WriteRowsExceptionDetail)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.WriteRowsExceptionDetail other = (org.yamcs.protobuf.Table.WriteRowsExceptionDetail) obj;
if (hasCount() != other.hasCount()) return false;
if (hasCount()) {
if (getCount()
!= other.getCount()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCount()) {
hash = (37 * hash) + COUNT_FIELD_NUMBER;
hash = (53 * hash) + getCount();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.WriteRowsExceptionDetail prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.WriteRowsExceptionDetail}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.WriteRowsExceptionDetail)
org.yamcs.protobuf.Table.WriteRowsExceptionDetailOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsExceptionDetail_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsExceptionDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.WriteRowsExceptionDetail.class, org.yamcs.protobuf.Table.WriteRowsExceptionDetail.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.WriteRowsExceptionDetail.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
count_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_WriteRowsExceptionDetail_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsExceptionDetail getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.WriteRowsExceptionDetail.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsExceptionDetail build() {
org.yamcs.protobuf.Table.WriteRowsExceptionDetail result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsExceptionDetail buildPartial() {
org.yamcs.protobuf.Table.WriteRowsExceptionDetail result = new org.yamcs.protobuf.Table.WriteRowsExceptionDetail(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.count_ = count_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.WriteRowsExceptionDetail) {
return mergeFrom((org.yamcs.protobuf.Table.WriteRowsExceptionDetail)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.WriteRowsExceptionDetail other) {
if (other == org.yamcs.protobuf.Table.WriteRowsExceptionDetail.getDefaultInstance()) return this;
if (other.hasCount()) {
setCount(other.getCount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.WriteRowsExceptionDetail parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.WriteRowsExceptionDetail) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int count_ ;
/**
* optional uint32 count = 1;
* @return Whether the count field is set.
*/
@java.lang.Override
public boolean hasCount() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional uint32 count = 1;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
/**
* optional uint32 count = 1;
* @param value The count to set.
* @return This builder for chaining.
*/
public Builder setCount(int value) {
bitField0_ |= 0x00000001;
count_ = value;
onChanged();
return this;
}
/**
* optional uint32 count = 1;
* @return This builder for chaining.
*/
public Builder clearCount() {
bitField0_ = (bitField0_ & ~0x00000001);
count_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.WriteRowsExceptionDetail)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.WriteRowsExceptionDetail)
private static final org.yamcs.protobuf.Table.WriteRowsExceptionDetail DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.WriteRowsExceptionDetail();
}
public static org.yamcs.protobuf.Table.WriteRowsExceptionDetail getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WriteRowsExceptionDetail parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WriteRowsExceptionDetail(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.WriteRowsExceptionDetail getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ListValue)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
java.util.List
getValuesList();
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
org.yamcs.protobuf.Yamcs.Value getValues(int index);
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
int getValuesCount();
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
java.util.List extends org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValuesOrBuilderList();
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
org.yamcs.protobuf.Yamcs.ValueOrBuilder getValuesOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListValue}
*/
public static final class ListValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ListValue)
ListValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListValue.newBuilder() to construct.
private ListValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListValue() {
values_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ListValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
values_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
values_.add(
input.readMessage(org.yamcs.protobuf.Yamcs.Value.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
values_ = java.util.Collections.unmodifiableList(values_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListValue.class, org.yamcs.protobuf.Table.ListValue.Builder.class);
}
public static final int VALUES_FIELD_NUMBER = 1;
private java.util.List values_;
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
@java.lang.Override
public java.util.List getValuesList() {
return values_;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValuesOrBuilderList() {
return values_;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
@java.lang.Override
public int getValuesCount() {
return values_.size();
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value getValues(int index) {
return values_.get(index);
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValuesOrBuilder(
int index) {
return values_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getValuesCount(); i++) {
if (!getValues(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < values_.size(); i++) {
output.writeMessage(1, values_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < values_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, values_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ListValue)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ListValue other = (org.yamcs.protobuf.Table.ListValue) obj;
if (!getValuesList()
.equals(other.getValuesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getValuesCount() > 0) {
hash = (37 * hash) + VALUES_FIELD_NUMBER;
hash = (53 * hash) + getValuesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ListValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ListValue)
org.yamcs.protobuf.Table.ListValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListValue.class, org.yamcs.protobuf.Table.ListValue.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ListValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValuesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (valuesBuilder_ == null) {
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
valuesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListValue_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListValue getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ListValue.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListValue build() {
org.yamcs.protobuf.Table.ListValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListValue buildPartial() {
org.yamcs.protobuf.Table.ListValue result = new org.yamcs.protobuf.Table.ListValue(this);
int from_bitField0_ = bitField0_;
if (valuesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
values_ = java.util.Collections.unmodifiableList(values_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.values_ = values_;
} else {
result.values_ = valuesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ListValue) {
return mergeFrom((org.yamcs.protobuf.Table.ListValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ListValue other) {
if (other == org.yamcs.protobuf.Table.ListValue.getDefaultInstance()) return this;
if (valuesBuilder_ == null) {
if (!other.values_.isEmpty()) {
if (values_.isEmpty()) {
values_ = other.values_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureValuesIsMutable();
values_.addAll(other.values_);
}
onChanged();
}
} else {
if (!other.values_.isEmpty()) {
if (valuesBuilder_.isEmpty()) {
valuesBuilder_.dispose();
valuesBuilder_ = null;
values_ = other.values_;
bitField0_ = (bitField0_ & ~0x00000001);
valuesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getValuesFieldBuilder() : null;
} else {
valuesBuilder_.addAllMessages(other.values_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getValuesCount(); i++) {
if (!getValues(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ListValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ListValue) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List values_ =
java.util.Collections.emptyList();
private void ensureValuesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
values_ = new java.util.ArrayList(values_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder> valuesBuilder_;
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public java.util.List getValuesList() {
if (valuesBuilder_ == null) {
return java.util.Collections.unmodifiableList(values_);
} else {
return valuesBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public int getValuesCount() {
if (valuesBuilder_ == null) {
return values_.size();
} else {
return valuesBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public org.yamcs.protobuf.Yamcs.Value getValues(int index) {
if (valuesBuilder_ == null) {
return values_.get(index);
} else {
return valuesBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public Builder setValues(
int index, org.yamcs.protobuf.Yamcs.Value value) {
if (valuesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.set(index, value);
onChanged();
} else {
valuesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public Builder setValues(
int index, org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.set(index, builderForValue.build());
onChanged();
} else {
valuesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public Builder addValues(org.yamcs.protobuf.Yamcs.Value value) {
if (valuesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.add(value);
onChanged();
} else {
valuesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public Builder addValues(
int index, org.yamcs.protobuf.Yamcs.Value value) {
if (valuesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.add(index, value);
onChanged();
} else {
valuesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public Builder addValues(
org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.add(builderForValue.build());
onChanged();
} else {
valuesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public Builder addValues(
int index, org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.add(index, builderForValue.build());
onChanged();
} else {
valuesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public Builder addAllValues(
java.lang.Iterable extends org.yamcs.protobuf.Yamcs.Value> values) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, values_);
onChanged();
} else {
valuesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public Builder clearValues() {
if (valuesBuilder_ == null) {
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
valuesBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public Builder removeValues(int index) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.remove(index);
onChanged();
} else {
valuesBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder getValuesBuilder(
int index) {
return getValuesFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValuesOrBuilder(
int index) {
if (valuesBuilder_ == null) {
return values_.get(index); } else {
return valuesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public java.util.List extends org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValuesOrBuilderList() {
if (valuesBuilder_ != null) {
return valuesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(values_);
}
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder addValuesBuilder() {
return getValuesFieldBuilder().addBuilder(
org.yamcs.protobuf.Yamcs.Value.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder addValuesBuilder(
int index) {
return getValuesFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Yamcs.Value.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.Value values = 1;
*/
public java.util.List
getValuesBuilderList() {
return getValuesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValuesFieldBuilder() {
if (valuesBuilder_ == null) {
valuesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>(
values_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
values_ = null;
}
return valuesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ListValue)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ListValue)
private static final org.yamcs.protobuf.Table.ListValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ListValue();
}
public static org.yamcs.protobuf.Table.ListValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ListValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListValue(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ExecuteSqlRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ExecuteSqlRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return Whether the statement field is set.
*/
boolean hasStatement();
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return The statement.
*/
java.lang.String getStatement();
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return The bytes for statement.
*/
com.google.protobuf.ByteString
getStatementBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.ExecuteSqlRequest}
*/
public static final class ExecuteSqlRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ExecuteSqlRequest)
ExecuteSqlRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ExecuteSqlRequest.newBuilder() to construct.
private ExecuteSqlRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ExecuteSqlRequest() {
instance_ = "";
statement_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ExecuteSqlRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExecuteSqlRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
statement_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ExecuteSqlRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ExecuteSqlRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ExecuteSqlRequest.class, org.yamcs.protobuf.Table.ExecuteSqlRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATEMENT_FIELD_NUMBER = 2;
private volatile java.lang.Object statement_;
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return Whether the statement field is set.
*/
@java.lang.Override
public boolean hasStatement() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return The statement.
*/
@java.lang.Override
public java.lang.String getStatement() {
java.lang.Object ref = statement_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
statement_ = s;
}
return s;
}
}
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return The bytes for statement.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStatementBytes() {
java.lang.Object ref = statement_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
statement_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, statement_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, statement_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ExecuteSqlRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ExecuteSqlRequest other = (org.yamcs.protobuf.Table.ExecuteSqlRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasStatement() != other.hasStatement()) return false;
if (hasStatement()) {
if (!getStatement()
.equals(other.getStatement())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasStatement()) {
hash = (37 * hash) + STATEMENT_FIELD_NUMBER;
hash = (53 * hash) + getStatement().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ExecuteSqlRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ExecuteSqlRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ExecuteSqlRequest)
org.yamcs.protobuf.Table.ExecuteSqlRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ExecuteSqlRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ExecuteSqlRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ExecuteSqlRequest.class, org.yamcs.protobuf.Table.ExecuteSqlRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ExecuteSqlRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
statement_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ExecuteSqlRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ExecuteSqlRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ExecuteSqlRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ExecuteSqlRequest build() {
org.yamcs.protobuf.Table.ExecuteSqlRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ExecuteSqlRequest buildPartial() {
org.yamcs.protobuf.Table.ExecuteSqlRequest result = new org.yamcs.protobuf.Table.ExecuteSqlRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.statement_ = statement_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ExecuteSqlRequest) {
return mergeFrom((org.yamcs.protobuf.Table.ExecuteSqlRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ExecuteSqlRequest other) {
if (other == org.yamcs.protobuf.Table.ExecuteSqlRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
if (other.hasStatement()) {
bitField0_ |= 0x00000002;
statement_ = other.statement_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ExecuteSqlRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ExecuteSqlRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
private java.lang.Object statement_ = "";
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return Whether the statement field is set.
*/
public boolean hasStatement() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return The statement.
*/
public java.lang.String getStatement() {
java.lang.Object ref = statement_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
statement_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return The bytes for statement.
*/
public com.google.protobuf.ByteString
getStatementBytes() {
java.lang.Object ref = statement_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
statement_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @param value The statement to set.
* @return This builder for chaining.
*/
public Builder setStatement(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
statement_ = value;
onChanged();
return this;
}
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @return This builder for chaining.
*/
public Builder clearStatement() {
bitField0_ = (bitField0_ & ~0x00000002);
statement_ = getDefaultInstance().getStatement();
onChanged();
return this;
}
/**
*
* StreamSQL statement
*
*
* optional string statement = 2;
* @param value The bytes for statement to set.
* @return This builder for chaining.
*/
public Builder setStatementBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
statement_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ExecuteSqlRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ExecuteSqlRequest)
private static final org.yamcs.protobuf.Table.ExecuteSqlRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ExecuteSqlRequest();
}
public static org.yamcs.protobuf.Table.ExecuteSqlRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ExecuteSqlRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExecuteSqlRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ExecuteSqlRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ResultSetOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ResultSet)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
java.util.List
getColumnsList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
org.yamcs.protobuf.Table.ColumnInfo getColumns(int index);
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
int getColumnsCount();
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getColumnsOrBuilderList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
org.yamcs.protobuf.Table.ColumnInfoOrBuilder getColumnsOrBuilder(
int index);
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
java.util.List
getRowsList();
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
org.yamcs.protobuf.Table.ListValue getRows(int index);
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
int getRowsCount();
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
java.util.List extends org.yamcs.protobuf.Table.ListValueOrBuilder>
getRowsOrBuilderList();
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
org.yamcs.protobuf.Table.ListValueOrBuilder getRowsOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.table.ResultSet}
*/
public static final class ResultSet extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ResultSet)
ResultSetOrBuilder {
private static final long serialVersionUID = 0L;
// Use ResultSet.newBuilder() to construct.
private ResultSet(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ResultSet() {
columns_ = java.util.Collections.emptyList();
rows_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ResultSet();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ResultSet(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
columns_.add(
input.readMessage(org.yamcs.protobuf.Table.ColumnInfo.PARSER, extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
rows_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
rows_.add(
input.readMessage(org.yamcs.protobuf.Table.ListValue.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
rows_ = java.util.Collections.unmodifiableList(rows_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ResultSet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ResultSet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ResultSet.class, org.yamcs.protobuf.Table.ResultSet.Builder.class);
}
public static final int COLUMNS_FIELD_NUMBER = 1;
private java.util.List columns_;
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
@java.lang.Override
public java.util.List getColumnsList() {
return columns_;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getColumnsOrBuilderList() {
return columns_;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
@java.lang.Override
public int getColumnsCount() {
return columns_.size();
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfo getColumns(int index) {
return columns_.get(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfoOrBuilder getColumnsOrBuilder(
int index) {
return columns_.get(index);
}
public static final int ROWS_FIELD_NUMBER = 2;
private java.util.List rows_;
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
@java.lang.Override
public java.util.List getRowsList() {
return rows_;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.ListValueOrBuilder>
getRowsOrBuilderList() {
return rows_;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
@java.lang.Override
public int getRowsCount() {
return rows_.size();
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ListValue getRows(int index) {
return rows_.get(index);
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ListValueOrBuilder getRowsOrBuilder(
int index) {
return rows_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getRowsCount(); i++) {
if (!getRows(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < columns_.size(); i++) {
output.writeMessage(1, columns_.get(i));
}
for (int i = 0; i < rows_.size(); i++) {
output.writeMessage(2, rows_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < columns_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, columns_.get(i));
}
for (int i = 0; i < rows_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, rows_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ResultSet)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ResultSet other = (org.yamcs.protobuf.Table.ResultSet) obj;
if (!getColumnsList()
.equals(other.getColumnsList())) return false;
if (!getRowsList()
.equals(other.getRowsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getColumnsCount() > 0) {
hash = (37 * hash) + COLUMNS_FIELD_NUMBER;
hash = (53 * hash) + getColumnsList().hashCode();
}
if (getRowsCount() > 0) {
hash = (37 * hash) + ROWS_FIELD_NUMBER;
hash = (53 * hash) + getRowsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ResultSet parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ResultSet parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ResultSet parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ResultSet prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ResultSet}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ResultSet)
org.yamcs.protobuf.Table.ResultSetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ResultSet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ResultSet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ResultSet.class, org.yamcs.protobuf.Table.ResultSet.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ResultSet.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getColumnsFieldBuilder();
getRowsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
columnsBuilder_.clear();
}
if (rowsBuilder_ == null) {
rows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
rowsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ResultSet_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ResultSet getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ResultSet.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ResultSet build() {
org.yamcs.protobuf.Table.ResultSet result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ResultSet buildPartial() {
org.yamcs.protobuf.Table.ResultSet result = new org.yamcs.protobuf.Table.ResultSet(this);
int from_bitField0_ = bitField0_;
if (columnsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.columns_ = columns_;
} else {
result.columns_ = columnsBuilder_.build();
}
if (rowsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
rows_ = java.util.Collections.unmodifiableList(rows_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.rows_ = rows_;
} else {
result.rows_ = rowsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ResultSet) {
return mergeFrom((org.yamcs.protobuf.Table.ResultSet)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ResultSet other) {
if (other == org.yamcs.protobuf.Table.ResultSet.getDefaultInstance()) return this;
if (columnsBuilder_ == null) {
if (!other.columns_.isEmpty()) {
if (columns_.isEmpty()) {
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureColumnsIsMutable();
columns_.addAll(other.columns_);
}
onChanged();
}
} else {
if (!other.columns_.isEmpty()) {
if (columnsBuilder_.isEmpty()) {
columnsBuilder_.dispose();
columnsBuilder_ = null;
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
columnsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getColumnsFieldBuilder() : null;
} else {
columnsBuilder_.addAllMessages(other.columns_);
}
}
}
if (rowsBuilder_ == null) {
if (!other.rows_.isEmpty()) {
if (rows_.isEmpty()) {
rows_ = other.rows_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureRowsIsMutable();
rows_.addAll(other.rows_);
}
onChanged();
}
} else {
if (!other.rows_.isEmpty()) {
if (rowsBuilder_.isEmpty()) {
rowsBuilder_.dispose();
rowsBuilder_ = null;
rows_ = other.rows_;
bitField0_ = (bitField0_ & ~0x00000002);
rowsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRowsFieldBuilder() : null;
} else {
rowsBuilder_.addAllMessages(other.rows_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getRowsCount(); i++) {
if (!getRows(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ResultSet parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ResultSet) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List columns_ =
java.util.Collections.emptyList();
private void ensureColumnsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList(columns_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnInfo, org.yamcs.protobuf.Table.ColumnInfo.Builder, org.yamcs.protobuf.Table.ColumnInfoOrBuilder> columnsBuilder_;
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public java.util.List getColumnsList() {
if (columnsBuilder_ == null) {
return java.util.Collections.unmodifiableList(columns_);
} else {
return columnsBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public int getColumnsCount() {
if (columnsBuilder_ == null) {
return columns_.size();
} else {
return columnsBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.ColumnInfo getColumns(int index) {
if (columnsBuilder_ == null) {
return columns_.get(index);
} else {
return columnsBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public Builder setColumns(
int index, org.yamcs.protobuf.Table.ColumnInfo value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.set(index, value);
onChanged();
} else {
columnsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public Builder setColumns(
int index, org.yamcs.protobuf.Table.ColumnInfo.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.set(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public Builder addColumns(org.yamcs.protobuf.Table.ColumnInfo value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(value);
onChanged();
} else {
columnsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public Builder addColumns(
int index, org.yamcs.protobuf.Table.ColumnInfo value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(index, value);
onChanged();
} else {
columnsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public Builder addColumns(
org.yamcs.protobuf.Table.ColumnInfo.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public Builder addColumns(
int index, org.yamcs.protobuf.Table.ColumnInfo.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public Builder addAllColumns(
java.lang.Iterable extends org.yamcs.protobuf.Table.ColumnInfo> values) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, columns_);
onChanged();
} else {
columnsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public Builder clearColumns() {
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
columnsBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public Builder removeColumns(int index) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.remove(index);
onChanged();
} else {
columnsBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.ColumnInfo.Builder getColumnsBuilder(
int index) {
return getColumnsFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.ColumnInfoOrBuilder getColumnsOrBuilder(
int index) {
if (columnsBuilder_ == null) {
return columns_.get(index); } else {
return columnsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getColumnsOrBuilderList() {
if (columnsBuilder_ != null) {
return columnsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(columns_);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.ColumnInfo.Builder addColumnsBuilder() {
return getColumnsFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.ColumnInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public org.yamcs.protobuf.Table.ColumnInfo.Builder addColumnsBuilder(
int index) {
return getColumnsFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.ColumnInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 1;
*/
public java.util.List
getColumnsBuilderList() {
return getColumnsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnInfo, org.yamcs.protobuf.Table.ColumnInfo.Builder, org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getColumnsFieldBuilder() {
if (columnsBuilder_ == null) {
columnsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnInfo, org.yamcs.protobuf.Table.ColumnInfo.Builder, org.yamcs.protobuf.Table.ColumnInfoOrBuilder>(
columns_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
columns_ = null;
}
return columnsBuilder_;
}
private java.util.List rows_ =
java.util.Collections.emptyList();
private void ensureRowsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
rows_ = new java.util.ArrayList(rows_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ListValue, org.yamcs.protobuf.Table.ListValue.Builder, org.yamcs.protobuf.Table.ListValueOrBuilder> rowsBuilder_;
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public java.util.List getRowsList() {
if (rowsBuilder_ == null) {
return java.util.Collections.unmodifiableList(rows_);
} else {
return rowsBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public int getRowsCount() {
if (rowsBuilder_ == null) {
return rows_.size();
} else {
return rowsBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public org.yamcs.protobuf.Table.ListValue getRows(int index) {
if (rowsBuilder_ == null) {
return rows_.get(index);
} else {
return rowsBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public Builder setRows(
int index, org.yamcs.protobuf.Table.ListValue value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.set(index, value);
onChanged();
} else {
rowsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public Builder setRows(
int index, org.yamcs.protobuf.Table.ListValue.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.set(index, builderForValue.build());
onChanged();
} else {
rowsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public Builder addRows(org.yamcs.protobuf.Table.ListValue value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.add(value);
onChanged();
} else {
rowsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public Builder addRows(
int index, org.yamcs.protobuf.Table.ListValue value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.add(index, value);
onChanged();
} else {
rowsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public Builder addRows(
org.yamcs.protobuf.Table.ListValue.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.add(builderForValue.build());
onChanged();
} else {
rowsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public Builder addRows(
int index, org.yamcs.protobuf.Table.ListValue.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.add(index, builderForValue.build());
onChanged();
} else {
rowsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public Builder addAllRows(
java.lang.Iterable extends org.yamcs.protobuf.Table.ListValue> values) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rows_);
onChanged();
} else {
rowsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public Builder clearRows() {
if (rowsBuilder_ == null) {
rows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
rowsBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public Builder removeRows(int index) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.remove(index);
onChanged();
} else {
rowsBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public org.yamcs.protobuf.Table.ListValue.Builder getRowsBuilder(
int index) {
return getRowsFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public org.yamcs.protobuf.Table.ListValueOrBuilder getRowsOrBuilder(
int index) {
if (rowsBuilder_ == null) {
return rows_.get(index); } else {
return rowsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public java.util.List extends org.yamcs.protobuf.Table.ListValueOrBuilder>
getRowsOrBuilderList() {
if (rowsBuilder_ != null) {
return rowsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rows_);
}
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public org.yamcs.protobuf.Table.ListValue.Builder addRowsBuilder() {
return getRowsFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.ListValue.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public org.yamcs.protobuf.Table.ListValue.Builder addRowsBuilder(
int index) {
return getRowsFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.ListValue.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ListValue rows = 2;
*/
public java.util.List
getRowsBuilderList() {
return getRowsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ListValue, org.yamcs.protobuf.Table.ListValue.Builder, org.yamcs.protobuf.Table.ListValueOrBuilder>
getRowsFieldBuilder() {
if (rowsBuilder_ == null) {
rowsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ListValue, org.yamcs.protobuf.Table.ListValue.Builder, org.yamcs.protobuf.Table.ListValueOrBuilder>(
rows_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
rows_ = null;
}
return rowsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ResultSet)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ResultSet)
private static final org.yamcs.protobuf.Table.ResultSet DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ResultSet();
}
public static org.yamcs.protobuf.Table.ResultSet getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ResultSet parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ResultSet(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ResultSet getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListTablesRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ListTablesRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListTablesRequest}
*/
public static final class ListTablesRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ListTablesRequest)
ListTablesRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListTablesRequest.newBuilder() to construct.
private ListTablesRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListTablesRequest() {
instance_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ListTablesRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListTablesRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListTablesRequest.class, org.yamcs.protobuf.Table.ListTablesRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ListTablesRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ListTablesRequest other = (org.yamcs.protobuf.Table.ListTablesRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListTablesRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ListTablesRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListTablesRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ListTablesRequest)
org.yamcs.protobuf.Table.ListTablesRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListTablesRequest.class, org.yamcs.protobuf.Table.ListTablesRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ListTablesRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListTablesRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ListTablesRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListTablesRequest build() {
org.yamcs.protobuf.Table.ListTablesRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListTablesRequest buildPartial() {
org.yamcs.protobuf.Table.ListTablesRequest result = new org.yamcs.protobuf.Table.ListTablesRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ListTablesRequest) {
return mergeFrom((org.yamcs.protobuf.Table.ListTablesRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ListTablesRequest other) {
if (other == org.yamcs.protobuf.Table.ListTablesRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ListTablesRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ListTablesRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ListTablesRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ListTablesRequest)
private static final org.yamcs.protobuf.Table.ListTablesRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ListTablesRequest();
}
public static org.yamcs.protobuf.Table.ListTablesRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ListTablesRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListTablesRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListTablesRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListTablesResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ListTablesResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
java.util.List
getTablesList();
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
org.yamcs.protobuf.Table.TableInfo getTables(int index);
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
int getTablesCount();
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
java.util.List extends org.yamcs.protobuf.Table.TableInfoOrBuilder>
getTablesOrBuilderList();
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
org.yamcs.protobuf.Table.TableInfoOrBuilder getTablesOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListTablesResponse}
*/
public static final class ListTablesResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ListTablesResponse)
ListTablesResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListTablesResponse.newBuilder() to construct.
private ListTablesResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListTablesResponse() {
tables_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ListTablesResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListTablesResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tables_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
tables_.add(
input.readMessage(org.yamcs.protobuf.Table.TableInfo.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tables_ = java.util.Collections.unmodifiableList(tables_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListTablesResponse.class, org.yamcs.protobuf.Table.ListTablesResponse.Builder.class);
}
public static final int TABLES_FIELD_NUMBER = 1;
private java.util.List tables_;
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
@java.lang.Override
public java.util.List getTablesList() {
return tables_;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.TableInfoOrBuilder>
getTablesOrBuilderList() {
return tables_;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
@java.lang.Override
public int getTablesCount() {
return tables_.size();
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.TableInfo getTables(int index) {
return tables_.get(index);
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.TableInfoOrBuilder getTablesOrBuilder(
int index) {
return tables_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < tables_.size(); i++) {
output.writeMessage(1, tables_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < tables_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, tables_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ListTablesResponse)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ListTablesResponse other = (org.yamcs.protobuf.Table.ListTablesResponse) obj;
if (!getTablesList()
.equals(other.getTablesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTablesCount() > 0) {
hash = (37 * hash) + TABLES_FIELD_NUMBER;
hash = (53 * hash) + getTablesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListTablesResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ListTablesResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListTablesResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ListTablesResponse)
org.yamcs.protobuf.Table.ListTablesResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListTablesResponse.class, org.yamcs.protobuf.Table.ListTablesResponse.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ListTablesResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTablesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (tablesBuilder_ == null) {
tables_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
tablesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListTablesResponse_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListTablesResponse getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ListTablesResponse.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListTablesResponse build() {
org.yamcs.protobuf.Table.ListTablesResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListTablesResponse buildPartial() {
org.yamcs.protobuf.Table.ListTablesResponse result = new org.yamcs.protobuf.Table.ListTablesResponse(this);
int from_bitField0_ = bitField0_;
if (tablesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
tables_ = java.util.Collections.unmodifiableList(tables_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tables_ = tables_;
} else {
result.tables_ = tablesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ListTablesResponse) {
return mergeFrom((org.yamcs.protobuf.Table.ListTablesResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ListTablesResponse other) {
if (other == org.yamcs.protobuf.Table.ListTablesResponse.getDefaultInstance()) return this;
if (tablesBuilder_ == null) {
if (!other.tables_.isEmpty()) {
if (tables_.isEmpty()) {
tables_ = other.tables_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTablesIsMutable();
tables_.addAll(other.tables_);
}
onChanged();
}
} else {
if (!other.tables_.isEmpty()) {
if (tablesBuilder_.isEmpty()) {
tablesBuilder_.dispose();
tablesBuilder_ = null;
tables_ = other.tables_;
bitField0_ = (bitField0_ & ~0x00000001);
tablesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTablesFieldBuilder() : null;
} else {
tablesBuilder_.addAllMessages(other.tables_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ListTablesResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ListTablesResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List tables_ =
java.util.Collections.emptyList();
private void ensureTablesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tables_ = new java.util.ArrayList(tables_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.TableInfo, org.yamcs.protobuf.Table.TableInfo.Builder, org.yamcs.protobuf.Table.TableInfoOrBuilder> tablesBuilder_;
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public java.util.List getTablesList() {
if (tablesBuilder_ == null) {
return java.util.Collections.unmodifiableList(tables_);
} else {
return tablesBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public int getTablesCount() {
if (tablesBuilder_ == null) {
return tables_.size();
} else {
return tablesBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public org.yamcs.protobuf.Table.TableInfo getTables(int index) {
if (tablesBuilder_ == null) {
return tables_.get(index);
} else {
return tablesBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public Builder setTables(
int index, org.yamcs.protobuf.Table.TableInfo value) {
if (tablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTablesIsMutable();
tables_.set(index, value);
onChanged();
} else {
tablesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public Builder setTables(
int index, org.yamcs.protobuf.Table.TableInfo.Builder builderForValue) {
if (tablesBuilder_ == null) {
ensureTablesIsMutable();
tables_.set(index, builderForValue.build());
onChanged();
} else {
tablesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public Builder addTables(org.yamcs.protobuf.Table.TableInfo value) {
if (tablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTablesIsMutable();
tables_.add(value);
onChanged();
} else {
tablesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public Builder addTables(
int index, org.yamcs.protobuf.Table.TableInfo value) {
if (tablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTablesIsMutable();
tables_.add(index, value);
onChanged();
} else {
tablesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public Builder addTables(
org.yamcs.protobuf.Table.TableInfo.Builder builderForValue) {
if (tablesBuilder_ == null) {
ensureTablesIsMutable();
tables_.add(builderForValue.build());
onChanged();
} else {
tablesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public Builder addTables(
int index, org.yamcs.protobuf.Table.TableInfo.Builder builderForValue) {
if (tablesBuilder_ == null) {
ensureTablesIsMutable();
tables_.add(index, builderForValue.build());
onChanged();
} else {
tablesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public Builder addAllTables(
java.lang.Iterable extends org.yamcs.protobuf.Table.TableInfo> values) {
if (tablesBuilder_ == null) {
ensureTablesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tables_);
onChanged();
} else {
tablesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public Builder clearTables() {
if (tablesBuilder_ == null) {
tables_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
tablesBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public Builder removeTables(int index) {
if (tablesBuilder_ == null) {
ensureTablesIsMutable();
tables_.remove(index);
onChanged();
} else {
tablesBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public org.yamcs.protobuf.Table.TableInfo.Builder getTablesBuilder(
int index) {
return getTablesFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public org.yamcs.protobuf.Table.TableInfoOrBuilder getTablesOrBuilder(
int index) {
if (tablesBuilder_ == null) {
return tables_.get(index); } else {
return tablesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public java.util.List extends org.yamcs.protobuf.Table.TableInfoOrBuilder>
getTablesOrBuilderList() {
if (tablesBuilder_ != null) {
return tablesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(tables_);
}
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public org.yamcs.protobuf.Table.TableInfo.Builder addTablesBuilder() {
return getTablesFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.TableInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public org.yamcs.protobuf.Table.TableInfo.Builder addTablesBuilder(
int index) {
return getTablesFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.TableInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.TableInfo tables = 1;
*/
public java.util.List
getTablesBuilderList() {
return getTablesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.TableInfo, org.yamcs.protobuf.Table.TableInfo.Builder, org.yamcs.protobuf.Table.TableInfoOrBuilder>
getTablesFieldBuilder() {
if (tablesBuilder_ == null) {
tablesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.TableInfo, org.yamcs.protobuf.Table.TableInfo.Builder, org.yamcs.protobuf.Table.TableInfoOrBuilder>(
tables_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
tables_ = null;
}
return tablesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ListTablesResponse)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ListTablesResponse)
private static final org.yamcs.protobuf.Table.ListTablesResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ListTablesResponse();
}
public static org.yamcs.protobuf.Table.ListTablesResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ListTablesResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListTablesResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListTablesResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetTableRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.GetTableRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
*
* Table name.
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.GetTableRequest}
*/
public static final class GetTableRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.GetTableRequest)
GetTableRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetTableRequest.newBuilder() to construct.
private GetTableRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetTableRequest() {
instance_ = "";
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetTableRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetTableRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.GetTableRequest.class, org.yamcs.protobuf.Table.GetTableRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
*
* Table name.
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.GetTableRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.GetTableRequest other = (org.yamcs.protobuf.Table.GetTableRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.GetTableRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.GetTableRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.GetTableRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.GetTableRequest)
org.yamcs.protobuf.Table.GetTableRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.GetTableRequest.class, org.yamcs.protobuf.Table.GetTableRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.GetTableRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetTableRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.GetTableRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetTableRequest build() {
org.yamcs.protobuf.Table.GetTableRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetTableRequest buildPartial() {
org.yamcs.protobuf.Table.GetTableRequest result = new org.yamcs.protobuf.Table.GetTableRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.GetTableRequest) {
return mergeFrom((org.yamcs.protobuf.Table.GetTableRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.GetTableRequest other) {
if (other == org.yamcs.protobuf.Table.GetTableRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.GetTableRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.GetTableRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Table name.
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.GetTableRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.GetTableRequest)
private static final org.yamcs.protobuf.Table.GetTableRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.GetTableRequest();
}
public static org.yamcs.protobuf.Table.GetTableRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetTableRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetTableRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetTableRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetTableDataRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.GetTableDataRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
*
* Table name.
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return A list containing the cols.
*/
java.util.List
getColsList();
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return The count of cols.
*/
int getColsCount();
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the element to return.
* @return The cols at the given index.
*/
java.lang.String getCols(int index);
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the value to return.
* @return The bytes of the cols at the given index.
*/
com.google.protobuf.ByteString
getColsBytes(int index);
/**
*
* The zero-based row number at which to start outputting results. Default: ``0``
* Note that in the current rocksdb storage engine there is no way to jump to a row by its number.
* This is why such a request will do a table scan and can be slow for large values of pos.
*
*
* optional int64 pos = 4;
* @return Whether the pos field is set.
*/
boolean hasPos();
/**
*
* The zero-based row number at which to start outputting results. Default: ``0``
* Note that in the current rocksdb storage engine there is no way to jump to a row by its number.
* This is why such a request will do a table scan and can be slow for large values of pos.
*
*
* optional int64 pos = 4;
* @return The pos.
*/
long getPos();
/**
*
* The maximum number of returned records per page. Choose this value
* too high and you risk hitting the maximum response size limit
* enforced by the server. Default: ``100``
*
*
* optional int32 limit = 5;
* @return Whether the limit field is set.
*/
boolean hasLimit();
/**
*
* The maximum number of returned records per page. Choose this value
* too high and you risk hitting the maximum response size limit
* enforced by the server. Default: ``100``
*
*
* optional int32 limit = 5;
* @return The limit.
*/
int getLimit();
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return Whether the order field is set.
*/
boolean hasOrder();
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return The order.
*/
java.lang.String getOrder();
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return The bytes for order.
*/
com.google.protobuf.ByteString
getOrderBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.GetTableDataRequest}
*/
public static final class GetTableDataRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.GetTableDataRequest)
GetTableDataRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetTableDataRequest.newBuilder() to construct.
private GetTableDataRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetTableDataRequest() {
instance_ = "";
name_ = "";
cols_ = com.google.protobuf.LazyStringArrayList.EMPTY;
order_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetTableDataRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetTableDataRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
cols_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000004;
}
cols_.add(bs);
break;
}
case 32: {
bitField0_ |= 0x00000004;
pos_ = input.readInt64();
break;
}
case 40: {
bitField0_ |= 0x00000008;
limit_ = input.readInt32();
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
order_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) != 0)) {
cols_ = cols_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableDataRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableDataRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.GetTableDataRequest.class, org.yamcs.protobuf.Table.GetTableDataRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
*
* Table name.
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COLS_FIELD_NUMBER = 3;
private com.google.protobuf.LazyStringList cols_;
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return A list containing the cols.
*/
public com.google.protobuf.ProtocolStringList
getColsList() {
return cols_;
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return The count of cols.
*/
public int getColsCount() {
return cols_.size();
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the element to return.
* @return The cols at the given index.
*/
public java.lang.String getCols(int index) {
return cols_.get(index);
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the value to return.
* @return The bytes of the cols at the given index.
*/
public com.google.protobuf.ByteString
getColsBytes(int index) {
return cols_.getByteString(index);
}
public static final int POS_FIELD_NUMBER = 4;
private long pos_;
/**
*
* The zero-based row number at which to start outputting results. Default: ``0``
* Note that in the current rocksdb storage engine there is no way to jump to a row by its number.
* This is why such a request will do a table scan and can be slow for large values of pos.
*
*
* optional int64 pos = 4;
* @return Whether the pos field is set.
*/
@java.lang.Override
public boolean hasPos() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The zero-based row number at which to start outputting results. Default: ``0``
* Note that in the current rocksdb storage engine there is no way to jump to a row by its number.
* This is why such a request will do a table scan and can be slow for large values of pos.
*
*
* optional int64 pos = 4;
* @return The pos.
*/
@java.lang.Override
public long getPos() {
return pos_;
}
public static final int LIMIT_FIELD_NUMBER = 5;
private int limit_;
/**
*
* The maximum number of returned records per page. Choose this value
* too high and you risk hitting the maximum response size limit
* enforced by the server. Default: ``100``
*
*
* optional int32 limit = 5;
* @return Whether the limit field is set.
*/
@java.lang.Override
public boolean hasLimit() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The maximum number of returned records per page. Choose this value
* too high and you risk hitting the maximum response size limit
* enforced by the server. Default: ``100``
*
*
* optional int32 limit = 5;
* @return The limit.
*/
@java.lang.Override
public int getLimit() {
return limit_;
}
public static final int ORDER_FIELD_NUMBER = 6;
private volatile java.lang.Object order_;
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return Whether the order field is set.
*/
@java.lang.Override
public boolean hasOrder() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return The order.
*/
@java.lang.Override
public java.lang.String getOrder() {
java.lang.Object ref = order_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
order_ = s;
}
return s;
}
}
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return The bytes for order.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOrderBytes() {
java.lang.Object ref = order_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
order_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
for (int i = 0; i < cols_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, cols_.getRaw(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(4, pos_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(5, limit_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, order_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
{
int dataSize = 0;
for (int i = 0; i < cols_.size(); i++) {
dataSize += computeStringSizeNoTag(cols_.getRaw(i));
}
size += dataSize;
size += 1 * getColsList().size();
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, pos_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, limit_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, order_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.GetTableDataRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.GetTableDataRequest other = (org.yamcs.protobuf.Table.GetTableDataRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!getColsList()
.equals(other.getColsList())) return false;
if (hasPos() != other.hasPos()) return false;
if (hasPos()) {
if (getPos()
!= other.getPos()) return false;
}
if (hasLimit() != other.hasLimit()) return false;
if (hasLimit()) {
if (getLimit()
!= other.getLimit()) return false;
}
if (hasOrder() != other.hasOrder()) return false;
if (hasOrder()) {
if (!getOrder()
.equals(other.getOrder())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (getColsCount() > 0) {
hash = (37 * hash) + COLS_FIELD_NUMBER;
hash = (53 * hash) + getColsList().hashCode();
}
if (hasPos()) {
hash = (37 * hash) + POS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPos());
}
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit();
}
if (hasOrder()) {
hash = (37 * hash) + ORDER_FIELD_NUMBER;
hash = (53 * hash) + getOrder().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.GetTableDataRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.GetTableDataRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.GetTableDataRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.GetTableDataRequest)
org.yamcs.protobuf.Table.GetTableDataRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableDataRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableDataRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.GetTableDataRequest.class, org.yamcs.protobuf.Table.GetTableDataRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.GetTableDataRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
cols_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
pos_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
limit_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
order_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetTableDataRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetTableDataRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.GetTableDataRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetTableDataRequest build() {
org.yamcs.protobuf.Table.GetTableDataRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetTableDataRequest buildPartial() {
org.yamcs.protobuf.Table.GetTableDataRequest result = new org.yamcs.protobuf.Table.GetTableDataRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
if (((bitField0_ & 0x00000004) != 0)) {
cols_ = cols_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.cols_ = cols_;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.pos_ = pos_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.limit_ = limit_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.order_ = order_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.GetTableDataRequest) {
return mergeFrom((org.yamcs.protobuf.Table.GetTableDataRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.GetTableDataRequest other) {
if (other == org.yamcs.protobuf.Table.GetTableDataRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (!other.cols_.isEmpty()) {
if (cols_.isEmpty()) {
cols_ = other.cols_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureColsIsMutable();
cols_.addAll(other.cols_);
}
onChanged();
}
if (other.hasPos()) {
setPos(other.getPos());
}
if (other.hasLimit()) {
setLimit(other.getLimit());
}
if (other.hasOrder()) {
bitField0_ |= 0x00000020;
order_ = other.order_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.GetTableDataRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.GetTableDataRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Table name.
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Table name.
*
*
* optional string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList cols_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureColsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
cols_ = new com.google.protobuf.LazyStringArrayList(cols_);
bitField0_ |= 0x00000004;
}
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return A list containing the cols.
*/
public com.google.protobuf.ProtocolStringList
getColsList() {
return cols_.getUnmodifiableView();
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return The count of cols.
*/
public int getColsCount() {
return cols_.size();
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the element to return.
* @return The cols at the given index.
*/
public java.lang.String getCols(int index) {
return cols_.get(index);
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index of the value to return.
* @return The bytes of the cols at the given index.
*/
public com.google.protobuf.ByteString
getColsBytes(int index) {
return cols_.getByteString(index);
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param index The index to set the value at.
* @param value The cols to set.
* @return This builder for chaining.
*/
public Builder setCols(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureColsIsMutable();
cols_.set(index, value);
onChanged();
return this;
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param value The cols to add.
* @return This builder for chaining.
*/
public Builder addCols(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureColsIsMutable();
cols_.add(value);
onChanged();
return this;
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param values The cols to add.
* @return This builder for chaining.
*/
public Builder addAllCols(
java.lang.Iterable values) {
ensureColsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cols_);
onChanged();
return this;
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @return This builder for chaining.
*/
public Builder clearCols() {
cols_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* The columns to be included in the result. If unspecified, all table
* and/or additional tuple columns will be included.
*
*
* repeated string cols = 3;
* @param value The bytes of the cols to add.
* @return This builder for chaining.
*/
public Builder addColsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureColsIsMutable();
cols_.add(value);
onChanged();
return this;
}
private long pos_ ;
/**
*
* The zero-based row number at which to start outputting results. Default: ``0``
* Note that in the current rocksdb storage engine there is no way to jump to a row by its number.
* This is why such a request will do a table scan and can be slow for large values of pos.
*
*
* optional int64 pos = 4;
* @return Whether the pos field is set.
*/
@java.lang.Override
public boolean hasPos() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The zero-based row number at which to start outputting results. Default: ``0``
* Note that in the current rocksdb storage engine there is no way to jump to a row by its number.
* This is why such a request will do a table scan and can be slow for large values of pos.
*
*
* optional int64 pos = 4;
* @return The pos.
*/
@java.lang.Override
public long getPos() {
return pos_;
}
/**
*
* The zero-based row number at which to start outputting results. Default: ``0``
* Note that in the current rocksdb storage engine there is no way to jump to a row by its number.
* This is why such a request will do a table scan and can be slow for large values of pos.
*
*
* optional int64 pos = 4;
* @param value The pos to set.
* @return This builder for chaining.
*/
public Builder setPos(long value) {
bitField0_ |= 0x00000008;
pos_ = value;
onChanged();
return this;
}
/**
*
* The zero-based row number at which to start outputting results. Default: ``0``
* Note that in the current rocksdb storage engine there is no way to jump to a row by its number.
* This is why such a request will do a table scan and can be slow for large values of pos.
*
*
* optional int64 pos = 4;
* @return This builder for chaining.
*/
public Builder clearPos() {
bitField0_ = (bitField0_ & ~0x00000008);
pos_ = 0L;
onChanged();
return this;
}
private int limit_ ;
/**
*
* The maximum number of returned records per page. Choose this value
* too high and you risk hitting the maximum response size limit
* enforced by the server. Default: ``100``
*
*
* optional int32 limit = 5;
* @return Whether the limit field is set.
*/
@java.lang.Override
public boolean hasLimit() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The maximum number of returned records per page. Choose this value
* too high and you risk hitting the maximum response size limit
* enforced by the server. Default: ``100``
*
*
* optional int32 limit = 5;
* @return The limit.
*/
@java.lang.Override
public int getLimit() {
return limit_;
}
/**
*
* The maximum number of returned records per page. Choose this value
* too high and you risk hitting the maximum response size limit
* enforced by the server. Default: ``100``
*
*
* optional int32 limit = 5;
* @param value The limit to set.
* @return This builder for chaining.
*/
public Builder setLimit(int value) {
bitField0_ |= 0x00000010;
limit_ = value;
onChanged();
return this;
}
/**
*
* The maximum number of returned records per page. Choose this value
* too high and you risk hitting the maximum response size limit
* enforced by the server. Default: ``100``
*
*
* optional int32 limit = 5;
* @return This builder for chaining.
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000010);
limit_ = 0;
onChanged();
return this;
}
private java.lang.Object order_ = "";
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return Whether the order field is set.
*/
public boolean hasOrder() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return The order.
*/
public java.lang.String getOrder() {
java.lang.Object ref = order_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
order_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return The bytes for order.
*/
public com.google.protobuf.ByteString
getOrderBytes() {
java.lang.Object ref = order_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
order_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @param value The order to set.
* @return This builder for chaining.
*/
public Builder setOrder(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
order_ = value;
onChanged();
return this;
}
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @return This builder for chaining.
*/
public Builder clearOrder() {
bitField0_ = (bitField0_ & ~0x00000020);
order_ = getDefaultInstance().getOrder();
onChanged();
return this;
}
/**
*
* The direction of the sort. Sorting is always done on the key of the
* table. Can be either ``asc`` or ``desc``. Default: ``desc``
*
*
* optional string order = 6;
* @param value The bytes for order to set.
* @return This builder for chaining.
*/
public Builder setOrderBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
order_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.GetTableDataRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.GetTableDataRequest)
private static final org.yamcs.protobuf.Table.GetTableDataRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.GetTableDataRequest();
}
public static org.yamcs.protobuf.Table.GetTableDataRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetTableDataRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetTableDataRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetTableDataRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListStreamsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ListStreamsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListStreamsRequest}
*/
public static final class ListStreamsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ListStreamsRequest)
ListStreamsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListStreamsRequest.newBuilder() to construct.
private ListStreamsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListStreamsRequest() {
instance_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ListStreamsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListStreamsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListStreamsRequest.class, org.yamcs.protobuf.Table.ListStreamsRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ListStreamsRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ListStreamsRequest other = (org.yamcs.protobuf.Table.ListStreamsRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListStreamsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ListStreamsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListStreamsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ListStreamsRequest)
org.yamcs.protobuf.Table.ListStreamsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListStreamsRequest.class, org.yamcs.protobuf.Table.ListStreamsRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ListStreamsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListStreamsRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ListStreamsRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListStreamsRequest build() {
org.yamcs.protobuf.Table.ListStreamsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListStreamsRequest buildPartial() {
org.yamcs.protobuf.Table.ListStreamsRequest result = new org.yamcs.protobuf.Table.ListStreamsRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ListStreamsRequest) {
return mergeFrom((org.yamcs.protobuf.Table.ListStreamsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ListStreamsRequest other) {
if (other == org.yamcs.protobuf.Table.ListStreamsRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ListStreamsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ListStreamsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name.
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ListStreamsRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ListStreamsRequest)
private static final org.yamcs.protobuf.Table.ListStreamsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ListStreamsRequest();
}
public static org.yamcs.protobuf.Table.ListStreamsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ListStreamsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListStreamsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListStreamsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListStreamsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ListStreamsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
java.util.List
getStreamsList();
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
org.yamcs.protobuf.Table.StreamInfo getStreams(int index);
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
int getStreamsCount();
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
java.util.List extends org.yamcs.protobuf.Table.StreamInfoOrBuilder>
getStreamsOrBuilderList();
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
org.yamcs.protobuf.Table.StreamInfoOrBuilder getStreamsOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListStreamsResponse}
*/
public static final class ListStreamsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ListStreamsResponse)
ListStreamsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListStreamsResponse.newBuilder() to construct.
private ListStreamsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListStreamsResponse() {
streams_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ListStreamsResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListStreamsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
streams_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
streams_.add(
input.readMessage(org.yamcs.protobuf.Table.StreamInfo.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
streams_ = java.util.Collections.unmodifiableList(streams_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListStreamsResponse.class, org.yamcs.protobuf.Table.ListStreamsResponse.Builder.class);
}
public static final int STREAMS_FIELD_NUMBER = 1;
private java.util.List streams_;
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
@java.lang.Override
public java.util.List getStreamsList() {
return streams_;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.StreamInfoOrBuilder>
getStreamsOrBuilderList() {
return streams_;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
@java.lang.Override
public int getStreamsCount() {
return streams_.size();
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.StreamInfo getStreams(int index) {
return streams_.get(index);
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.StreamInfoOrBuilder getStreamsOrBuilder(
int index) {
return streams_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < streams_.size(); i++) {
output.writeMessage(1, streams_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < streams_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, streams_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ListStreamsResponse)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ListStreamsResponse other = (org.yamcs.protobuf.Table.ListStreamsResponse) obj;
if (!getStreamsList()
.equals(other.getStreamsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getStreamsCount() > 0) {
hash = (37 * hash) + STREAMS_FIELD_NUMBER;
hash = (53 * hash) + getStreamsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ListStreamsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ListStreamsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ListStreamsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ListStreamsResponse)
org.yamcs.protobuf.Table.ListStreamsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ListStreamsResponse.class, org.yamcs.protobuf.Table.ListStreamsResponse.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ListStreamsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStreamsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (streamsBuilder_ == null) {
streams_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
streamsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ListStreamsResponse_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListStreamsResponse getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ListStreamsResponse.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListStreamsResponse build() {
org.yamcs.protobuf.Table.ListStreamsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListStreamsResponse buildPartial() {
org.yamcs.protobuf.Table.ListStreamsResponse result = new org.yamcs.protobuf.Table.ListStreamsResponse(this);
int from_bitField0_ = bitField0_;
if (streamsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
streams_ = java.util.Collections.unmodifiableList(streams_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.streams_ = streams_;
} else {
result.streams_ = streamsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ListStreamsResponse) {
return mergeFrom((org.yamcs.protobuf.Table.ListStreamsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ListStreamsResponse other) {
if (other == org.yamcs.protobuf.Table.ListStreamsResponse.getDefaultInstance()) return this;
if (streamsBuilder_ == null) {
if (!other.streams_.isEmpty()) {
if (streams_.isEmpty()) {
streams_ = other.streams_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureStreamsIsMutable();
streams_.addAll(other.streams_);
}
onChanged();
}
} else {
if (!other.streams_.isEmpty()) {
if (streamsBuilder_.isEmpty()) {
streamsBuilder_.dispose();
streamsBuilder_ = null;
streams_ = other.streams_;
bitField0_ = (bitField0_ & ~0x00000001);
streamsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getStreamsFieldBuilder() : null;
} else {
streamsBuilder_.addAllMessages(other.streams_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ListStreamsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ListStreamsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List streams_ =
java.util.Collections.emptyList();
private void ensureStreamsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
streams_ = new java.util.ArrayList(streams_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.StreamInfo, org.yamcs.protobuf.Table.StreamInfo.Builder, org.yamcs.protobuf.Table.StreamInfoOrBuilder> streamsBuilder_;
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public java.util.List getStreamsList() {
if (streamsBuilder_ == null) {
return java.util.Collections.unmodifiableList(streams_);
} else {
return streamsBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public int getStreamsCount() {
if (streamsBuilder_ == null) {
return streams_.size();
} else {
return streamsBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public org.yamcs.protobuf.Table.StreamInfo getStreams(int index) {
if (streamsBuilder_ == null) {
return streams_.get(index);
} else {
return streamsBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public Builder setStreams(
int index, org.yamcs.protobuf.Table.StreamInfo value) {
if (streamsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStreamsIsMutable();
streams_.set(index, value);
onChanged();
} else {
streamsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public Builder setStreams(
int index, org.yamcs.protobuf.Table.StreamInfo.Builder builderForValue) {
if (streamsBuilder_ == null) {
ensureStreamsIsMutable();
streams_.set(index, builderForValue.build());
onChanged();
} else {
streamsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public Builder addStreams(org.yamcs.protobuf.Table.StreamInfo value) {
if (streamsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStreamsIsMutable();
streams_.add(value);
onChanged();
} else {
streamsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public Builder addStreams(
int index, org.yamcs.protobuf.Table.StreamInfo value) {
if (streamsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStreamsIsMutable();
streams_.add(index, value);
onChanged();
} else {
streamsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public Builder addStreams(
org.yamcs.protobuf.Table.StreamInfo.Builder builderForValue) {
if (streamsBuilder_ == null) {
ensureStreamsIsMutable();
streams_.add(builderForValue.build());
onChanged();
} else {
streamsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public Builder addStreams(
int index, org.yamcs.protobuf.Table.StreamInfo.Builder builderForValue) {
if (streamsBuilder_ == null) {
ensureStreamsIsMutable();
streams_.add(index, builderForValue.build());
onChanged();
} else {
streamsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public Builder addAllStreams(
java.lang.Iterable extends org.yamcs.protobuf.Table.StreamInfo> values) {
if (streamsBuilder_ == null) {
ensureStreamsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, streams_);
onChanged();
} else {
streamsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public Builder clearStreams() {
if (streamsBuilder_ == null) {
streams_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
streamsBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public Builder removeStreams(int index) {
if (streamsBuilder_ == null) {
ensureStreamsIsMutable();
streams_.remove(index);
onChanged();
} else {
streamsBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public org.yamcs.protobuf.Table.StreamInfo.Builder getStreamsBuilder(
int index) {
return getStreamsFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public org.yamcs.protobuf.Table.StreamInfoOrBuilder getStreamsOrBuilder(
int index) {
if (streamsBuilder_ == null) {
return streams_.get(index); } else {
return streamsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public java.util.List extends org.yamcs.protobuf.Table.StreamInfoOrBuilder>
getStreamsOrBuilderList() {
if (streamsBuilder_ != null) {
return streamsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(streams_);
}
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public org.yamcs.protobuf.Table.StreamInfo.Builder addStreamsBuilder() {
return getStreamsFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.StreamInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public org.yamcs.protobuf.Table.StreamInfo.Builder addStreamsBuilder(
int index) {
return getStreamsFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.StreamInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.StreamInfo streams = 1;
*/
public java.util.List
getStreamsBuilderList() {
return getStreamsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.StreamInfo, org.yamcs.protobuf.Table.StreamInfo.Builder, org.yamcs.protobuf.Table.StreamInfoOrBuilder>
getStreamsFieldBuilder() {
if (streamsBuilder_ == null) {
streamsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.StreamInfo, org.yamcs.protobuf.Table.StreamInfo.Builder, org.yamcs.protobuf.Table.StreamInfoOrBuilder>(
streams_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
streams_ = null;
}
return streamsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ListStreamsResponse)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ListStreamsResponse)
private static final org.yamcs.protobuf.Table.ListStreamsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ListStreamsResponse();
}
public static org.yamcs.protobuf.Table.ListStreamsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ListStreamsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListStreamsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ListStreamsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetStreamRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.GetStreamRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
*
* Stream name
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Stream name
*
*
* optional string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
*
* Stream name
*
*
* optional string name = 2;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.GetStreamRequest}
*/
public static final class GetStreamRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.GetStreamRequest)
GetStreamRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetStreamRequest.newBuilder() to construct.
private GetStreamRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetStreamRequest() {
instance_ = "";
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetStreamRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetStreamRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.GetStreamRequest.class, org.yamcs.protobuf.Table.GetStreamRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
*
* Stream name
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Stream name
*
*
* optional string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Stream name
*
*
* optional string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.GetStreamRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.GetStreamRequest other = (org.yamcs.protobuf.Table.GetStreamRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.GetStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.GetStreamRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.GetStreamRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.GetStreamRequest)
org.yamcs.protobuf.Table.GetStreamRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.GetStreamRequest.class, org.yamcs.protobuf.Table.GetStreamRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.GetStreamRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_GetStreamRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetStreamRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.GetStreamRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetStreamRequest build() {
org.yamcs.protobuf.Table.GetStreamRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetStreamRequest buildPartial() {
org.yamcs.protobuf.Table.GetStreamRequest result = new org.yamcs.protobuf.Table.GetStreamRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.GetStreamRequest) {
return mergeFrom((org.yamcs.protobuf.Table.GetStreamRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.GetStreamRequest other) {
if (other == org.yamcs.protobuf.Table.GetStreamRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.GetStreamRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.GetStreamRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Stream name
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Stream name
*
*
* optional string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Stream name
*
*
* optional string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Stream name
*
*
* optional string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
*
* Stream name
*
*
* optional string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Stream name
*
*
* optional string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.GetStreamRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.GetStreamRequest)
private static final org.yamcs.protobuf.Table.GetStreamRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.GetStreamRequest();
}
public static org.yamcs.protobuf.Table.GetStreamRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetStreamRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetStreamRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.GetStreamRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SubscribeStreamRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.SubscribeStreamRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return Whether the stream field is set.
*/
boolean hasStream();
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return The stream.
*/
java.lang.String getStream();
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return The bytes for stream.
*/
com.google.protobuf.ByteString
getStreamBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.SubscribeStreamRequest}
*/
public static final class SubscribeStreamRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.SubscribeStreamRequest)
SubscribeStreamRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use SubscribeStreamRequest.newBuilder() to construct.
private SubscribeStreamRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SubscribeStreamRequest() {
instance_ = "";
stream_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SubscribeStreamRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SubscribeStreamRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
stream_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.SubscribeStreamRequest.class, org.yamcs.protobuf.Table.SubscribeStreamRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STREAM_FIELD_NUMBER = 2;
private volatile java.lang.Object stream_;
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return Whether the stream field is set.
*/
@java.lang.Override
public boolean hasStream() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return The stream.
*/
@java.lang.Override
public java.lang.String getStream() {
java.lang.Object ref = stream_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stream_ = s;
}
return s;
}
}
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return The bytes for stream.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStreamBytes() {
java.lang.Object ref = stream_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stream_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, stream_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, stream_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.SubscribeStreamRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.SubscribeStreamRequest other = (org.yamcs.protobuf.Table.SubscribeStreamRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasStream() != other.hasStream()) return false;
if (hasStream()) {
if (!getStream()
.equals(other.getStream())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasStream()) {
hash = (37 * hash) + STREAM_FIELD_NUMBER;
hash = (53 * hash) + getStream().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.SubscribeStreamRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.SubscribeStreamRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.SubscribeStreamRequest)
org.yamcs.protobuf.Table.SubscribeStreamRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.SubscribeStreamRequest.class, org.yamcs.protobuf.Table.SubscribeStreamRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.SubscribeStreamRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
stream_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.SubscribeStreamRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.SubscribeStreamRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.SubscribeStreamRequest build() {
org.yamcs.protobuf.Table.SubscribeStreamRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.SubscribeStreamRequest buildPartial() {
org.yamcs.protobuf.Table.SubscribeStreamRequest result = new org.yamcs.protobuf.Table.SubscribeStreamRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.stream_ = stream_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.SubscribeStreamRequest) {
return mergeFrom((org.yamcs.protobuf.Table.SubscribeStreamRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.SubscribeStreamRequest other) {
if (other == org.yamcs.protobuf.Table.SubscribeStreamRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
if (other.hasStream()) {
bitField0_ |= 0x00000002;
stream_ = other.stream_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.SubscribeStreamRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.SubscribeStreamRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
*
* Yamcs instance name
*
*
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
private java.lang.Object stream_ = "";
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return Whether the stream field is set.
*/
public boolean hasStream() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return The stream.
*/
public java.lang.String getStream() {
java.lang.Object ref = stream_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stream_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return The bytes for stream.
*/
public com.google.protobuf.ByteString
getStreamBytes() {
java.lang.Object ref = stream_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stream_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Stream name
*
*
* optional string stream = 2;
* @param value The stream to set.
* @return This builder for chaining.
*/
public Builder setStream(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
stream_ = value;
onChanged();
return this;
}
/**
*
* Stream name
*
*
* optional string stream = 2;
* @return This builder for chaining.
*/
public Builder clearStream() {
bitField0_ = (bitField0_ & ~0x00000002);
stream_ = getDefaultInstance().getStream();
onChanged();
return this;
}
/**
*
* Stream name
*
*
* optional string stream = 2;
* @param value The bytes for stream to set.
* @return This builder for chaining.
*/
public Builder setStreamBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
stream_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.SubscribeStreamRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.SubscribeStreamRequest)
private static final org.yamcs.protobuf.Table.SubscribeStreamRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.SubscribeStreamRequest();
}
public static org.yamcs.protobuf.Table.SubscribeStreamRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SubscribeStreamRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SubscribeStreamRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.SubscribeStreamRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ColumnDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ColumnData)
com.google.protobuf.MessageOrBuilder {
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional .yamcs.protobuf.Value value = 2;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* optional .yamcs.protobuf.Value value = 2;
* @return The value.
*/
org.yamcs.protobuf.Yamcs.Value getValue();
/**
* optional .yamcs.protobuf.Value value = 2;
*/
org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder();
}
/**
* Protobuf type {@code yamcs.protobuf.table.ColumnData}
*/
public static final class ColumnData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ColumnData)
ColumnDataOrBuilder {
private static final long serialVersionUID = 0L;
// Use ColumnData.newBuilder() to construct.
private ColumnData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ColumnData() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ColumnData();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ColumnData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
case 18: {
org.yamcs.protobuf.Yamcs.Value.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = value_.toBuilder();
}
value_ = input.readMessage(org.yamcs.protobuf.Yamcs.Value.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(value_);
value_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ColumnData.class, org.yamcs.protobuf.Table.ColumnData.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private org.yamcs.protobuf.Yamcs.Value value_;
/**
* optional .yamcs.protobuf.Value value = 2;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.Value value = 2;
* @return The value.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value getValue() {
return value_ == null ? org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder() {
return value_ == null ? org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasValue()) {
if (!getValue().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getValue());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getValue());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ColumnData)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ColumnData other = (org.yamcs.protobuf.Table.ColumnData) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ColumnData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ColumnData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ColumnData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ColumnData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ColumnData)
org.yamcs.protobuf.Table.ColumnDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ColumnData.class, org.yamcs.protobuf.Table.ColumnData.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ColumnData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (valueBuilder_ == null) {
value_ = null;
} else {
valueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnData_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnData getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ColumnData.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnData build() {
org.yamcs.protobuf.Table.ColumnData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnData buildPartial() {
org.yamcs.protobuf.Table.ColumnData result = new org.yamcs.protobuf.Table.ColumnData(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) != 0)) {
if (valueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = valueBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ColumnData) {
return mergeFrom((org.yamcs.protobuf.Table.ColumnData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ColumnData other) {
if (other == org.yamcs.protobuf.Table.ColumnData.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasValue()) {
if (!getValue().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ColumnData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ColumnData) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private org.yamcs.protobuf.Yamcs.Value value_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder> valueBuilder_;
/**
* optional .yamcs.protobuf.Value value = 2;
* @return Whether the value field is set.
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.Value value = 2;
* @return The value.
*/
public org.yamcs.protobuf.Yamcs.Value getValue() {
if (valueBuilder_ == null) {
return value_ == null ? org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
} else {
return valueBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder setValue(org.yamcs.protobuf.Yamcs.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
valueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder setValue(
org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
valueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder mergeValue(org.yamcs.protobuf.Yamcs.Value value) {
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
value_ != null &&
value_ != org.yamcs.protobuf.Yamcs.Value.getDefaultInstance()) {
value_ =
org.yamcs.protobuf.Yamcs.Value.newBuilder(value_).mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
valueBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public Builder clearValue() {
if (valueBuilder_ == null) {
value_ = null;
onChanged();
} else {
valueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder getValueBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getValueFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilder();
} else {
return value_ == null ?
org.yamcs.protobuf.Yamcs.Value.getDefaultInstance() : value_;
}
}
/**
* optional .yamcs.protobuf.Value value = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>(
getValue(),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ColumnData)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ColumnData)
private static final org.yamcs.protobuf.Table.ColumnData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ColumnData();
}
public static org.yamcs.protobuf.Table.ColumnData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ColumnData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ColumnData(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StreamDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.StreamData)
com.google.protobuf.MessageOrBuilder {
/**
* optional string stream = 1;
* @return Whether the stream field is set.
*/
boolean hasStream();
/**
* optional string stream = 1;
* @return The stream.
*/
java.lang.String getStream();
/**
* optional string stream = 1;
* @return The bytes for stream.
*/
com.google.protobuf.ByteString
getStreamBytes();
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
java.util.List
getColumnList();
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
org.yamcs.protobuf.Table.ColumnData getColumn(int index);
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
int getColumnCount();
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
java.util.List extends org.yamcs.protobuf.Table.ColumnDataOrBuilder>
getColumnOrBuilderList();
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
org.yamcs.protobuf.Table.ColumnDataOrBuilder getColumnOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.table.StreamData}
*/
public static final class StreamData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.StreamData)
StreamDataOrBuilder {
private static final long serialVersionUID = 0L;
// Use StreamData.newBuilder() to construct.
private StreamData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StreamData() {
stream_ = "";
column_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StreamData();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StreamData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
stream_ = bs;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
column_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
column_.add(
input.readMessage(org.yamcs.protobuf.Table.ColumnData.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
column_ = java.util.Collections.unmodifiableList(column_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_StreamData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_StreamData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.StreamData.class, org.yamcs.protobuf.Table.StreamData.Builder.class);
}
private int bitField0_;
public static final int STREAM_FIELD_NUMBER = 1;
private volatile java.lang.Object stream_;
/**
* optional string stream = 1;
* @return Whether the stream field is set.
*/
@java.lang.Override
public boolean hasStream() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string stream = 1;
* @return The stream.
*/
@java.lang.Override
public java.lang.String getStream() {
java.lang.Object ref = stream_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stream_ = s;
}
return s;
}
}
/**
* optional string stream = 1;
* @return The bytes for stream.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStreamBytes() {
java.lang.Object ref = stream_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stream_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COLUMN_FIELD_NUMBER = 2;
private java.util.List column_;
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
@java.lang.Override
public java.util.List getColumnList() {
return column_;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.ColumnDataOrBuilder>
getColumnOrBuilderList() {
return column_;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
@java.lang.Override
public int getColumnCount() {
return column_.size();
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnData getColumn(int index) {
return column_.get(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnDataOrBuilder getColumnOrBuilder(
int index) {
return column_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getColumnCount(); i++) {
if (!getColumn(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, stream_);
}
for (int i = 0; i < column_.size(); i++) {
output.writeMessage(2, column_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, stream_);
}
for (int i = 0; i < column_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, column_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.StreamData)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.StreamData other = (org.yamcs.protobuf.Table.StreamData) obj;
if (hasStream() != other.hasStream()) return false;
if (hasStream()) {
if (!getStream()
.equals(other.getStream())) return false;
}
if (!getColumnList()
.equals(other.getColumnList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStream()) {
hash = (37 * hash) + STREAM_FIELD_NUMBER;
hash = (53 * hash) + getStream().hashCode();
}
if (getColumnCount() > 0) {
hash = (37 * hash) + COLUMN_FIELD_NUMBER;
hash = (53 * hash) + getColumnList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.StreamData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.StreamData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.StreamData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.StreamData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.StreamData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.StreamData)
org.yamcs.protobuf.Table.StreamDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_StreamData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_StreamData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.StreamData.class, org.yamcs.protobuf.Table.StreamData.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.StreamData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getColumnFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
stream_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (columnBuilder_ == null) {
column_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
columnBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_StreamData_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.StreamData getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.StreamData.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.StreamData build() {
org.yamcs.protobuf.Table.StreamData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.StreamData buildPartial() {
org.yamcs.protobuf.Table.StreamData result = new org.yamcs.protobuf.Table.StreamData(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.stream_ = stream_;
if (columnBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
column_ = java.util.Collections.unmodifiableList(column_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.column_ = column_;
} else {
result.column_ = columnBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.StreamData) {
return mergeFrom((org.yamcs.protobuf.Table.StreamData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.StreamData other) {
if (other == org.yamcs.protobuf.Table.StreamData.getDefaultInstance()) return this;
if (other.hasStream()) {
bitField0_ |= 0x00000001;
stream_ = other.stream_;
onChanged();
}
if (columnBuilder_ == null) {
if (!other.column_.isEmpty()) {
if (column_.isEmpty()) {
column_ = other.column_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureColumnIsMutable();
column_.addAll(other.column_);
}
onChanged();
}
} else {
if (!other.column_.isEmpty()) {
if (columnBuilder_.isEmpty()) {
columnBuilder_.dispose();
columnBuilder_ = null;
column_ = other.column_;
bitField0_ = (bitField0_ & ~0x00000002);
columnBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getColumnFieldBuilder() : null;
} else {
columnBuilder_.addAllMessages(other.column_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getColumnCount(); i++) {
if (!getColumn(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.StreamData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.StreamData) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object stream_ = "";
/**
* optional string stream = 1;
* @return Whether the stream field is set.
*/
public boolean hasStream() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string stream = 1;
* @return The stream.
*/
public java.lang.String getStream() {
java.lang.Object ref = stream_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stream_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string stream = 1;
* @return The bytes for stream.
*/
public com.google.protobuf.ByteString
getStreamBytes() {
java.lang.Object ref = stream_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stream_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string stream = 1;
* @param value The stream to set.
* @return This builder for chaining.
*/
public Builder setStream(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
stream_ = value;
onChanged();
return this;
}
/**
* optional string stream = 1;
* @return This builder for chaining.
*/
public Builder clearStream() {
bitField0_ = (bitField0_ & ~0x00000001);
stream_ = getDefaultInstance().getStream();
onChanged();
return this;
}
/**
* optional string stream = 1;
* @param value The bytes for stream to set.
* @return This builder for chaining.
*/
public Builder setStreamBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
stream_ = value;
onChanged();
return this;
}
private java.util.List column_ =
java.util.Collections.emptyList();
private void ensureColumnIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
column_ = new java.util.ArrayList(column_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnData, org.yamcs.protobuf.Table.ColumnData.Builder, org.yamcs.protobuf.Table.ColumnDataOrBuilder> columnBuilder_;
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public java.util.List getColumnList() {
if (columnBuilder_ == null) {
return java.util.Collections.unmodifiableList(column_);
} else {
return columnBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public int getColumnCount() {
if (columnBuilder_ == null) {
return column_.size();
} else {
return columnBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public org.yamcs.protobuf.Table.ColumnData getColumn(int index) {
if (columnBuilder_ == null) {
return column_.get(index);
} else {
return columnBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public Builder setColumn(
int index, org.yamcs.protobuf.Table.ColumnData value) {
if (columnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.set(index, value);
onChanged();
} else {
columnBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public Builder setColumn(
int index, org.yamcs.protobuf.Table.ColumnData.Builder builderForValue) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
column_.set(index, builderForValue.build());
onChanged();
} else {
columnBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public Builder addColumn(org.yamcs.protobuf.Table.ColumnData value) {
if (columnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.add(value);
onChanged();
} else {
columnBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public Builder addColumn(
int index, org.yamcs.protobuf.Table.ColumnData value) {
if (columnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.add(index, value);
onChanged();
} else {
columnBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public Builder addColumn(
org.yamcs.protobuf.Table.ColumnData.Builder builderForValue) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
column_.add(builderForValue.build());
onChanged();
} else {
columnBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public Builder addColumn(
int index, org.yamcs.protobuf.Table.ColumnData.Builder builderForValue) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
column_.add(index, builderForValue.build());
onChanged();
} else {
columnBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public Builder addAllColumn(
java.lang.Iterable extends org.yamcs.protobuf.Table.ColumnData> values) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, column_);
onChanged();
} else {
columnBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public Builder clearColumn() {
if (columnBuilder_ == null) {
column_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
columnBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public Builder removeColumn(int index) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
column_.remove(index);
onChanged();
} else {
columnBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public org.yamcs.protobuf.Table.ColumnData.Builder getColumnBuilder(
int index) {
return getColumnFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public org.yamcs.protobuf.Table.ColumnDataOrBuilder getColumnOrBuilder(
int index) {
if (columnBuilder_ == null) {
return column_.get(index); } else {
return columnBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public java.util.List extends org.yamcs.protobuf.Table.ColumnDataOrBuilder>
getColumnOrBuilderList() {
if (columnBuilder_ != null) {
return columnBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(column_);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public org.yamcs.protobuf.Table.ColumnData.Builder addColumnBuilder() {
return getColumnFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.ColumnData.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public org.yamcs.protobuf.Table.ColumnData.Builder addColumnBuilder(
int index) {
return getColumnFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.ColumnData.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 2;
*/
public java.util.List
getColumnBuilderList() {
return getColumnFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnData, org.yamcs.protobuf.Table.ColumnData.Builder, org.yamcs.protobuf.Table.ColumnDataOrBuilder>
getColumnFieldBuilder() {
if (columnBuilder_ == null) {
columnBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnData, org.yamcs.protobuf.Table.ColumnData.Builder, org.yamcs.protobuf.Table.ColumnDataOrBuilder>(
column_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
column_ = null;
}
return columnBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.StreamData)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.StreamData)
private static final org.yamcs.protobuf.Table.StreamData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.StreamData();
}
public static org.yamcs.protobuf.Table.StreamData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StreamData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StreamData(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.StreamData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SubscribeStreamStatisticsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.SubscribeStreamStatisticsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* optional string instance = 1;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
* optional string instance = 1;
* @return The instance.
*/
java.lang.String getInstance();
/**
* optional string instance = 1;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.SubscribeStreamStatisticsRequest}
*/
public static final class SubscribeStreamStatisticsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.SubscribeStreamStatisticsRequest)
SubscribeStreamStatisticsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use SubscribeStreamStatisticsRequest.newBuilder() to construct.
private SubscribeStreamStatisticsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SubscribeStreamStatisticsRequest() {
instance_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SubscribeStreamStatisticsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SubscribeStreamStatisticsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
instance_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamStatisticsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamStatisticsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest.class, org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest.Builder.class);
}
private int bitField0_;
public static final int INSTANCE_FIELD_NUMBER = 1;
private volatile java.lang.Object instance_;
/**
* optional string instance = 1;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string instance = 1;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
* optional string instance = 1;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, instance_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, instance_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest other = (org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest) obj;
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.SubscribeStreamStatisticsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.SubscribeStreamStatisticsRequest)
org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamStatisticsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamStatisticsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest.class, org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_SubscribeStreamStatisticsRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest build() {
org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest buildPartial() {
org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest result = new org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.instance_ = instance_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest) {
return mergeFrom((org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest other) {
if (other == org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest.getDefaultInstance()) return this;
if (other.hasInstance()) {
bitField0_ |= 0x00000001;
instance_ = other.instance_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object instance_ = "";
/**
* optional string instance = 1;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string instance = 1;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string instance = 1;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
/**
* optional string instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
bitField0_ = (bitField0_ & ~0x00000001);
instance_ = getDefaultInstance().getInstance();
onChanged();
return this;
}
/**
* optional string instance = 1;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
instance_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.SubscribeStreamStatisticsRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.SubscribeStreamStatisticsRequest)
private static final org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest();
}
public static org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SubscribeStreamStatisticsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SubscribeStreamStatisticsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.SubscribeStreamStatisticsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TableDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.TableData)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
java.util.List
getRecordList();
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
org.yamcs.protobuf.Table.TableData.TableRecord getRecord(int index);
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
int getRecordCount();
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
java.util.List extends org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder>
getRecordOrBuilderList();
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder getRecordOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.table.TableData}
*/
public static final class TableData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.TableData)
TableDataOrBuilder {
private static final long serialVersionUID = 0L;
// Use TableData.newBuilder() to construct.
private TableData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TableData() {
record_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TableData();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TableData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
record_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
record_.add(
input.readMessage(org.yamcs.protobuf.Table.TableData.TableRecord.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
record_ = java.util.Collections.unmodifiableList(record_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.TableData.class, org.yamcs.protobuf.Table.TableData.Builder.class);
}
public interface TableRecordOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.TableData.TableRecord)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
java.util.List
getColumnList();
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
org.yamcs.protobuf.Table.ColumnData getColumn(int index);
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
int getColumnCount();
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
java.util.List extends org.yamcs.protobuf.Table.ColumnDataOrBuilder>
getColumnOrBuilderList();
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
org.yamcs.protobuf.Table.ColumnDataOrBuilder getColumnOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.table.TableData.TableRecord}
*/
public static final class TableRecord extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.TableData.TableRecord)
TableRecordOrBuilder {
private static final long serialVersionUID = 0L;
// Use TableRecord.newBuilder() to construct.
private TableRecord(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TableRecord() {
column_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TableRecord();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TableRecord(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
column_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
column_.add(
input.readMessage(org.yamcs.protobuf.Table.ColumnData.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
column_ = java.util.Collections.unmodifiableList(column_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_TableRecord_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_TableRecord_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.TableData.TableRecord.class, org.yamcs.protobuf.Table.TableData.TableRecord.Builder.class);
}
public static final int COLUMN_FIELD_NUMBER = 1;
private java.util.List column_;
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
@java.lang.Override
public java.util.List getColumnList() {
return column_;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.ColumnDataOrBuilder>
getColumnOrBuilderList() {
return column_;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
@java.lang.Override
public int getColumnCount() {
return column_.size();
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnData getColumn(int index) {
return column_.get(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnDataOrBuilder getColumnOrBuilder(
int index) {
return column_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getColumnCount(); i++) {
if (!getColumn(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < column_.size(); i++) {
output.writeMessage(1, column_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < column_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, column_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.TableData.TableRecord)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.TableData.TableRecord other = (org.yamcs.protobuf.Table.TableData.TableRecord) obj;
if (!getColumnList()
.equals(other.getColumnList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getColumnCount() > 0) {
hash = (37 * hash) + COLUMN_FIELD_NUMBER;
hash = (53 * hash) + getColumnList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.TableData.TableRecord parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.TableData.TableRecord prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.TableData.TableRecord}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.TableData.TableRecord)
org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_TableRecord_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_TableRecord_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.TableData.TableRecord.class, org.yamcs.protobuf.Table.TableData.TableRecord.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.TableData.TableRecord.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getColumnFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (columnBuilder_ == null) {
column_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
columnBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_TableRecord_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableData.TableRecord getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.TableData.TableRecord.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableData.TableRecord build() {
org.yamcs.protobuf.Table.TableData.TableRecord result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableData.TableRecord buildPartial() {
org.yamcs.protobuf.Table.TableData.TableRecord result = new org.yamcs.protobuf.Table.TableData.TableRecord(this);
int from_bitField0_ = bitField0_;
if (columnBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
column_ = java.util.Collections.unmodifiableList(column_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.column_ = column_;
} else {
result.column_ = columnBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.TableData.TableRecord) {
return mergeFrom((org.yamcs.protobuf.Table.TableData.TableRecord)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.TableData.TableRecord other) {
if (other == org.yamcs.protobuf.Table.TableData.TableRecord.getDefaultInstance()) return this;
if (columnBuilder_ == null) {
if (!other.column_.isEmpty()) {
if (column_.isEmpty()) {
column_ = other.column_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureColumnIsMutable();
column_.addAll(other.column_);
}
onChanged();
}
} else {
if (!other.column_.isEmpty()) {
if (columnBuilder_.isEmpty()) {
columnBuilder_.dispose();
columnBuilder_ = null;
column_ = other.column_;
bitField0_ = (bitField0_ & ~0x00000001);
columnBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getColumnFieldBuilder() : null;
} else {
columnBuilder_.addAllMessages(other.column_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getColumnCount(); i++) {
if (!getColumn(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.TableData.TableRecord parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.TableData.TableRecord) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List column_ =
java.util.Collections.emptyList();
private void ensureColumnIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
column_ = new java.util.ArrayList(column_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnData, org.yamcs.protobuf.Table.ColumnData.Builder, org.yamcs.protobuf.Table.ColumnDataOrBuilder> columnBuilder_;
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public java.util.List getColumnList() {
if (columnBuilder_ == null) {
return java.util.Collections.unmodifiableList(column_);
} else {
return columnBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public int getColumnCount() {
if (columnBuilder_ == null) {
return column_.size();
} else {
return columnBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public org.yamcs.protobuf.Table.ColumnData getColumn(int index) {
if (columnBuilder_ == null) {
return column_.get(index);
} else {
return columnBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public Builder setColumn(
int index, org.yamcs.protobuf.Table.ColumnData value) {
if (columnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.set(index, value);
onChanged();
} else {
columnBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public Builder setColumn(
int index, org.yamcs.protobuf.Table.ColumnData.Builder builderForValue) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
column_.set(index, builderForValue.build());
onChanged();
} else {
columnBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public Builder addColumn(org.yamcs.protobuf.Table.ColumnData value) {
if (columnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.add(value);
onChanged();
} else {
columnBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public Builder addColumn(
int index, org.yamcs.protobuf.Table.ColumnData value) {
if (columnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.add(index, value);
onChanged();
} else {
columnBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public Builder addColumn(
org.yamcs.protobuf.Table.ColumnData.Builder builderForValue) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
column_.add(builderForValue.build());
onChanged();
} else {
columnBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public Builder addColumn(
int index, org.yamcs.protobuf.Table.ColumnData.Builder builderForValue) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
column_.add(index, builderForValue.build());
onChanged();
} else {
columnBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public Builder addAllColumn(
java.lang.Iterable extends org.yamcs.protobuf.Table.ColumnData> values) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, column_);
onChanged();
} else {
columnBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public Builder clearColumn() {
if (columnBuilder_ == null) {
column_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
columnBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public Builder removeColumn(int index) {
if (columnBuilder_ == null) {
ensureColumnIsMutable();
column_.remove(index);
onChanged();
} else {
columnBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public org.yamcs.protobuf.Table.ColumnData.Builder getColumnBuilder(
int index) {
return getColumnFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public org.yamcs.protobuf.Table.ColumnDataOrBuilder getColumnOrBuilder(
int index) {
if (columnBuilder_ == null) {
return column_.get(index); } else {
return columnBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public java.util.List extends org.yamcs.protobuf.Table.ColumnDataOrBuilder>
getColumnOrBuilderList() {
if (columnBuilder_ != null) {
return columnBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(column_);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public org.yamcs.protobuf.Table.ColumnData.Builder addColumnBuilder() {
return getColumnFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.ColumnData.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public org.yamcs.protobuf.Table.ColumnData.Builder addColumnBuilder(
int index) {
return getColumnFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.ColumnData.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnData column = 1;
*/
public java.util.List
getColumnBuilderList() {
return getColumnFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnData, org.yamcs.protobuf.Table.ColumnData.Builder, org.yamcs.protobuf.Table.ColumnDataOrBuilder>
getColumnFieldBuilder() {
if (columnBuilder_ == null) {
columnBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnData, org.yamcs.protobuf.Table.ColumnData.Builder, org.yamcs.protobuf.Table.ColumnDataOrBuilder>(
column_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
column_ = null;
}
return columnBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.TableData.TableRecord)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.TableData.TableRecord)
private static final org.yamcs.protobuf.Table.TableData.TableRecord DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.TableData.TableRecord();
}
public static org.yamcs.protobuf.Table.TableData.TableRecord getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TableRecord parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TableRecord(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableData.TableRecord getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int RECORD_FIELD_NUMBER = 1;
private java.util.List record_;
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
@java.lang.Override
public java.util.List getRecordList() {
return record_;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder>
getRecordOrBuilderList() {
return record_;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
@java.lang.Override
public int getRecordCount() {
return record_.size();
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.TableData.TableRecord getRecord(int index) {
return record_.get(index);
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder getRecordOrBuilder(
int index) {
return record_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getRecordCount(); i++) {
if (!getRecord(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < record_.size(); i++) {
output.writeMessage(1, record_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < record_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, record_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.TableData)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.TableData other = (org.yamcs.protobuf.Table.TableData) obj;
if (!getRecordList()
.equals(other.getRecordList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getRecordCount() > 0) {
hash = (37 * hash) + RECORD_FIELD_NUMBER;
hash = (53 * hash) + getRecordList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.TableData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.TableData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.TableData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.TableData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.TableData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.TableData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.TableData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.TableData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.TableData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.TableData)
org.yamcs.protobuf.Table.TableDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.TableData.class, org.yamcs.protobuf.Table.TableData.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.TableData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRecordFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (recordBuilder_ == null) {
record_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
recordBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableData_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableData getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.TableData.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableData build() {
org.yamcs.protobuf.Table.TableData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableData buildPartial() {
org.yamcs.protobuf.Table.TableData result = new org.yamcs.protobuf.Table.TableData(this);
int from_bitField0_ = bitField0_;
if (recordBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
record_ = java.util.Collections.unmodifiableList(record_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.record_ = record_;
} else {
result.record_ = recordBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.TableData) {
return mergeFrom((org.yamcs.protobuf.Table.TableData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.TableData other) {
if (other == org.yamcs.protobuf.Table.TableData.getDefaultInstance()) return this;
if (recordBuilder_ == null) {
if (!other.record_.isEmpty()) {
if (record_.isEmpty()) {
record_ = other.record_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRecordIsMutable();
record_.addAll(other.record_);
}
onChanged();
}
} else {
if (!other.record_.isEmpty()) {
if (recordBuilder_.isEmpty()) {
recordBuilder_.dispose();
recordBuilder_ = null;
record_ = other.record_;
bitField0_ = (bitField0_ & ~0x00000001);
recordBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRecordFieldBuilder() : null;
} else {
recordBuilder_.addAllMessages(other.record_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getRecordCount(); i++) {
if (!getRecord(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.TableData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.TableData) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List record_ =
java.util.Collections.emptyList();
private void ensureRecordIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
record_ = new java.util.ArrayList(record_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.TableData.TableRecord, org.yamcs.protobuf.Table.TableData.TableRecord.Builder, org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder> recordBuilder_;
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public java.util.List getRecordList() {
if (recordBuilder_ == null) {
return java.util.Collections.unmodifiableList(record_);
} else {
return recordBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public int getRecordCount() {
if (recordBuilder_ == null) {
return record_.size();
} else {
return recordBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public org.yamcs.protobuf.Table.TableData.TableRecord getRecord(int index) {
if (recordBuilder_ == null) {
return record_.get(index);
} else {
return recordBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public Builder setRecord(
int index, org.yamcs.protobuf.Table.TableData.TableRecord value) {
if (recordBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRecordIsMutable();
record_.set(index, value);
onChanged();
} else {
recordBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public Builder setRecord(
int index, org.yamcs.protobuf.Table.TableData.TableRecord.Builder builderForValue) {
if (recordBuilder_ == null) {
ensureRecordIsMutable();
record_.set(index, builderForValue.build());
onChanged();
} else {
recordBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public Builder addRecord(org.yamcs.protobuf.Table.TableData.TableRecord value) {
if (recordBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRecordIsMutable();
record_.add(value);
onChanged();
} else {
recordBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public Builder addRecord(
int index, org.yamcs.protobuf.Table.TableData.TableRecord value) {
if (recordBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRecordIsMutable();
record_.add(index, value);
onChanged();
} else {
recordBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public Builder addRecord(
org.yamcs.protobuf.Table.TableData.TableRecord.Builder builderForValue) {
if (recordBuilder_ == null) {
ensureRecordIsMutable();
record_.add(builderForValue.build());
onChanged();
} else {
recordBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public Builder addRecord(
int index, org.yamcs.protobuf.Table.TableData.TableRecord.Builder builderForValue) {
if (recordBuilder_ == null) {
ensureRecordIsMutable();
record_.add(index, builderForValue.build());
onChanged();
} else {
recordBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public Builder addAllRecord(
java.lang.Iterable extends org.yamcs.protobuf.Table.TableData.TableRecord> values) {
if (recordBuilder_ == null) {
ensureRecordIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, record_);
onChanged();
} else {
recordBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public Builder clearRecord() {
if (recordBuilder_ == null) {
record_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
recordBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public Builder removeRecord(int index) {
if (recordBuilder_ == null) {
ensureRecordIsMutable();
record_.remove(index);
onChanged();
} else {
recordBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public org.yamcs.protobuf.Table.TableData.TableRecord.Builder getRecordBuilder(
int index) {
return getRecordFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder getRecordOrBuilder(
int index) {
if (recordBuilder_ == null) {
return record_.get(index); } else {
return recordBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public java.util.List extends org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder>
getRecordOrBuilderList() {
if (recordBuilder_ != null) {
return recordBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(record_);
}
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public org.yamcs.protobuf.Table.TableData.TableRecord.Builder addRecordBuilder() {
return getRecordFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.TableData.TableRecord.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public org.yamcs.protobuf.Table.TableData.TableRecord.Builder addRecordBuilder(
int index) {
return getRecordFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.TableData.TableRecord.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.TableData.TableRecord record = 1;
*/
public java.util.List
getRecordBuilderList() {
return getRecordFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.TableData.TableRecord, org.yamcs.protobuf.Table.TableData.TableRecord.Builder, org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder>
getRecordFieldBuilder() {
if (recordBuilder_ == null) {
recordBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.TableData.TableRecord, org.yamcs.protobuf.Table.TableData.TableRecord.Builder, org.yamcs.protobuf.Table.TableData.TableRecordOrBuilder>(
record_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
record_ = null;
}
return recordBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.TableData)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.TableData)
private static final org.yamcs.protobuf.Table.TableData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.TableData();
}
public static org.yamcs.protobuf.Table.TableData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TableData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TableData(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ColumnInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.ColumnInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* Colum name
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Colum name
*
*
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
*
* Colum name
*
*
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Column type
*
*
* optional string type = 2;
* @return Whether the type field is set.
*/
boolean hasType();
/**
*
* Column type
*
*
* optional string type = 2;
* @return The type.
*/
java.lang.String getType();
/**
*
* Column type
*
*
* optional string type = 2;
* @return The bytes for type.
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
java.util.List
getEnumValueList();
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
org.yamcs.protobuf.Table.EnumValue getEnumValue(int index);
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
int getEnumValueCount();
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
java.util.List extends org.yamcs.protobuf.Table.EnumValueOrBuilder>
getEnumValueOrBuilderList();
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
org.yamcs.protobuf.Table.EnumValueOrBuilder getEnumValueOrBuilder(
int index);
/**
*
* Attribute indicating automatic auto increment upon
* record insertion. Only set for table column info.
*
*
* optional bool autoIncrement = 4;
* @return Whether the autoIncrement field is set.
*/
boolean hasAutoIncrement();
/**
*
* Attribute indicating automatic auto increment upon
* record insertion. Only set for table column info.
*
*
* optional bool autoIncrement = 4;
* @return The autoIncrement.
*/
boolean getAutoIncrement();
}
/**
* Protobuf type {@code yamcs.protobuf.table.ColumnInfo}
*/
public static final class ColumnInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.ColumnInfo)
ColumnInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ColumnInfo.newBuilder() to construct.
private ColumnInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ColumnInfo() {
name_ = "";
type_ = "";
enumValue_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ColumnInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ColumnInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
type_ = bs;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
enumValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
enumValue_.add(
input.readMessage(org.yamcs.protobuf.Table.EnumValue.PARSER, extensionRegistry));
break;
}
case 32: {
bitField0_ |= 0x00000004;
autoIncrement_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) != 0)) {
enumValue_ = java.util.Collections.unmodifiableList(enumValue_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ColumnInfo.class, org.yamcs.protobuf.Table.ColumnInfo.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
*
* Colum name
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Colum name
*
*
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Colum name
*
*
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 2;
private volatile java.lang.Object type_;
/**
*
* Column type
*
*
* optional string type = 2;
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Column type
*
*
* optional string type = 2;
* @return The type.
*/
@java.lang.Override
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
}
}
/**
*
* Column type
*
*
* optional string type = 2;
* @return The bytes for type.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ENUMVALUE_FIELD_NUMBER = 3;
private java.util.List enumValue_;
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
@java.lang.Override
public java.util.List getEnumValueList() {
return enumValue_;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.EnumValueOrBuilder>
getEnumValueOrBuilderList() {
return enumValue_;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
@java.lang.Override
public int getEnumValueCount() {
return enumValue_.size();
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.EnumValue getEnumValue(int index) {
return enumValue_.get(index);
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.EnumValueOrBuilder getEnumValueOrBuilder(
int index) {
return enumValue_.get(index);
}
public static final int AUTOINCREMENT_FIELD_NUMBER = 4;
private boolean autoIncrement_;
/**
*
* Attribute indicating automatic auto increment upon
* record insertion. Only set for table column info.
*
*
* optional bool autoIncrement = 4;
* @return Whether the autoIncrement field is set.
*/
@java.lang.Override
public boolean hasAutoIncrement() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Attribute indicating automatic auto increment upon
* record insertion. Only set for table column info.
*
*
* optional bool autoIncrement = 4;
* @return The autoIncrement.
*/
@java.lang.Override
public boolean getAutoIncrement() {
return autoIncrement_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, type_);
}
for (int i = 0; i < enumValue_.size(); i++) {
output.writeMessage(3, enumValue_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(4, autoIncrement_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, type_);
}
for (int i = 0; i < enumValue_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, enumValue_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, autoIncrement_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.ColumnInfo)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.ColumnInfo other = (org.yamcs.protobuf.Table.ColumnInfo) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (!getType()
.equals(other.getType())) return false;
}
if (!getEnumValueList()
.equals(other.getEnumValueList())) return false;
if (hasAutoIncrement() != other.hasAutoIncrement()) return false;
if (hasAutoIncrement()) {
if (getAutoIncrement()
!= other.getAutoIncrement()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
if (getEnumValueCount() > 0) {
hash = (37 * hash) + ENUMVALUE_FIELD_NUMBER;
hash = (53 * hash) + getEnumValueList().hashCode();
}
if (hasAutoIncrement()) {
hash = (37 * hash) + AUTOINCREMENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAutoIncrement());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.ColumnInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.ColumnInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.ColumnInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.ColumnInfo)
org.yamcs.protobuf.Table.ColumnInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.ColumnInfo.class, org.yamcs.protobuf.Table.ColumnInfo.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.ColumnInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEnumValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
type_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
if (enumValueBuilder_ == null) {
enumValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
enumValueBuilder_.clear();
}
autoIncrement_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_ColumnInfo_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfo getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.ColumnInfo.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfo build() {
org.yamcs.protobuf.Table.ColumnInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfo buildPartial() {
org.yamcs.protobuf.Table.ColumnInfo result = new org.yamcs.protobuf.Table.ColumnInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
if (enumValueBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
enumValue_ = java.util.Collections.unmodifiableList(enumValue_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.enumValue_ = enumValue_;
} else {
result.enumValue_ = enumValueBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.autoIncrement_ = autoIncrement_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.ColumnInfo) {
return mergeFrom((org.yamcs.protobuf.Table.ColumnInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.ColumnInfo other) {
if (other == org.yamcs.protobuf.Table.ColumnInfo.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasType()) {
bitField0_ |= 0x00000002;
type_ = other.type_;
onChanged();
}
if (enumValueBuilder_ == null) {
if (!other.enumValue_.isEmpty()) {
if (enumValue_.isEmpty()) {
enumValue_ = other.enumValue_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureEnumValueIsMutable();
enumValue_.addAll(other.enumValue_);
}
onChanged();
}
} else {
if (!other.enumValue_.isEmpty()) {
if (enumValueBuilder_.isEmpty()) {
enumValueBuilder_.dispose();
enumValueBuilder_ = null;
enumValue_ = other.enumValue_;
bitField0_ = (bitField0_ & ~0x00000004);
enumValueBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEnumValueFieldBuilder() : null;
} else {
enumValueBuilder_.addAllMessages(other.enumValue_);
}
}
}
if (other.hasAutoIncrement()) {
setAutoIncrement(other.getAutoIncrement());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.ColumnInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.ColumnInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* Colum name
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Colum name
*
*
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Colum name
*
*
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Colum name
*
*
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
*
* Colum name
*
*
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Colum name
*
*
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
*
* Column type
*
*
* optional string type = 2;
* @return Whether the type field is set.
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Column type
*
*
* optional string type = 2;
* @return The type.
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Column type
*
*
* optional string type = 2;
* @return The bytes for type.
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Column type
*
*
* optional string type = 2;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
*
* Column type
*
*
* optional string type = 2;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
*
* Column type
*
*
* optional string type = 2;
* @param value The bytes for type to set.
* @return This builder for chaining.
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
private java.util.List enumValue_ =
java.util.Collections.emptyList();
private void ensureEnumValueIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
enumValue_ = new java.util.ArrayList(enumValue_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.EnumValue, org.yamcs.protobuf.Table.EnumValue.Builder, org.yamcs.protobuf.Table.EnumValueOrBuilder> enumValueBuilder_;
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public java.util.List getEnumValueList() {
if (enumValueBuilder_ == null) {
return java.util.Collections.unmodifiableList(enumValue_);
} else {
return enumValueBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public int getEnumValueCount() {
if (enumValueBuilder_ == null) {
return enumValue_.size();
} else {
return enumValueBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public org.yamcs.protobuf.Table.EnumValue getEnumValue(int index) {
if (enumValueBuilder_ == null) {
return enumValue_.get(index);
} else {
return enumValueBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public Builder setEnumValue(
int index, org.yamcs.protobuf.Table.EnumValue value) {
if (enumValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEnumValueIsMutable();
enumValue_.set(index, value);
onChanged();
} else {
enumValueBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public Builder setEnumValue(
int index, org.yamcs.protobuf.Table.EnumValue.Builder builderForValue) {
if (enumValueBuilder_ == null) {
ensureEnumValueIsMutable();
enumValue_.set(index, builderForValue.build());
onChanged();
} else {
enumValueBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public Builder addEnumValue(org.yamcs.protobuf.Table.EnumValue value) {
if (enumValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEnumValueIsMutable();
enumValue_.add(value);
onChanged();
} else {
enumValueBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public Builder addEnumValue(
int index, org.yamcs.protobuf.Table.EnumValue value) {
if (enumValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEnumValueIsMutable();
enumValue_.add(index, value);
onChanged();
} else {
enumValueBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public Builder addEnumValue(
org.yamcs.protobuf.Table.EnumValue.Builder builderForValue) {
if (enumValueBuilder_ == null) {
ensureEnumValueIsMutable();
enumValue_.add(builderForValue.build());
onChanged();
} else {
enumValueBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public Builder addEnumValue(
int index, org.yamcs.protobuf.Table.EnumValue.Builder builderForValue) {
if (enumValueBuilder_ == null) {
ensureEnumValueIsMutable();
enumValue_.add(index, builderForValue.build());
onChanged();
} else {
enumValueBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public Builder addAllEnumValue(
java.lang.Iterable extends org.yamcs.protobuf.Table.EnumValue> values) {
if (enumValueBuilder_ == null) {
ensureEnumValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, enumValue_);
onChanged();
} else {
enumValueBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public Builder clearEnumValue() {
if (enumValueBuilder_ == null) {
enumValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
enumValueBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public Builder removeEnumValue(int index) {
if (enumValueBuilder_ == null) {
ensureEnumValueIsMutable();
enumValue_.remove(index);
onChanged();
} else {
enumValueBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public org.yamcs.protobuf.Table.EnumValue.Builder getEnumValueBuilder(
int index) {
return getEnumValueFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public org.yamcs.protobuf.Table.EnumValueOrBuilder getEnumValueOrBuilder(
int index) {
if (enumValueBuilder_ == null) {
return enumValue_.get(index); } else {
return enumValueBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public java.util.List extends org.yamcs.protobuf.Table.EnumValueOrBuilder>
getEnumValueOrBuilderList() {
if (enumValueBuilder_ != null) {
return enumValueBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(enumValue_);
}
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public org.yamcs.protobuf.Table.EnumValue.Builder addEnumValueBuilder() {
return getEnumValueFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.EnumValue.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public org.yamcs.protobuf.Table.EnumValue.Builder addEnumValueBuilder(
int index) {
return getEnumValueFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.EnumValue.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.EnumValue enumValue = 3;
*/
public java.util.List
getEnumValueBuilderList() {
return getEnumValueFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.EnumValue, org.yamcs.protobuf.Table.EnumValue.Builder, org.yamcs.protobuf.Table.EnumValueOrBuilder>
getEnumValueFieldBuilder() {
if (enumValueBuilder_ == null) {
enumValueBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.EnumValue, org.yamcs.protobuf.Table.EnumValue.Builder, org.yamcs.protobuf.Table.EnumValueOrBuilder>(
enumValue_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
enumValue_ = null;
}
return enumValueBuilder_;
}
private boolean autoIncrement_ ;
/**
*
* Attribute indicating automatic auto increment upon
* record insertion. Only set for table column info.
*
*
* optional bool autoIncrement = 4;
* @return Whether the autoIncrement field is set.
*/
@java.lang.Override
public boolean hasAutoIncrement() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Attribute indicating automatic auto increment upon
* record insertion. Only set for table column info.
*
*
* optional bool autoIncrement = 4;
* @return The autoIncrement.
*/
@java.lang.Override
public boolean getAutoIncrement() {
return autoIncrement_;
}
/**
*
* Attribute indicating automatic auto increment upon
* record insertion. Only set for table column info.
*
*
* optional bool autoIncrement = 4;
* @param value The autoIncrement to set.
* @return This builder for chaining.
*/
public Builder setAutoIncrement(boolean value) {
bitField0_ |= 0x00000008;
autoIncrement_ = value;
onChanged();
return this;
}
/**
*
* Attribute indicating automatic auto increment upon
* record insertion. Only set for table column info.
*
*
* optional bool autoIncrement = 4;
* @return This builder for chaining.
*/
public Builder clearAutoIncrement() {
bitField0_ = (bitField0_ & ~0x00000008);
autoIncrement_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.ColumnInfo)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.ColumnInfo)
private static final org.yamcs.protobuf.Table.ColumnInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.ColumnInfo();
}
public static org.yamcs.protobuf.Table.ColumnInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ColumnInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ColumnInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EnumValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.EnumValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 value = 1;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* optional int32 value = 1;
* @return The value.
*/
int getValue();
/**
* optional string label = 2;
* @return Whether the label field is set.
*/
boolean hasLabel();
/**
* optional string label = 2;
* @return The label.
*/
java.lang.String getLabel();
/**
* optional string label = 2;
* @return The bytes for label.
*/
com.google.protobuf.ByteString
getLabelBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.EnumValue}
*/
public static final class EnumValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.EnumValue)
EnumValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use EnumValue.newBuilder() to construct.
private EnumValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EnumValue() {
label_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new EnumValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EnumValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
value_ = input.readInt32();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
label_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_EnumValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_EnumValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.EnumValue.class, org.yamcs.protobuf.Table.EnumValue.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private int value_;
/**
* optional int32 value = 1;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 value = 1;
* @return The value.
*/
@java.lang.Override
public int getValue() {
return value_;
}
public static final int LABEL_FIELD_NUMBER = 2;
private volatile java.lang.Object label_;
/**
* optional string label = 2;
* @return Whether the label field is set.
*/
@java.lang.Override
public boolean hasLabel() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string label = 2;
* @return The label.
*/
@java.lang.Override
public java.lang.String getLabel() {
java.lang.Object ref = label_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
label_ = s;
}
return s;
}
}
/**
* optional string label = 2;
* @return The bytes for label.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLabelBytes() {
java.lang.Object ref = label_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
label_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, value_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, label_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, value_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, label_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.EnumValue)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.EnumValue other = (org.yamcs.protobuf.Table.EnumValue) obj;
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (getValue()
!= other.getValue()) return false;
}
if (hasLabel() != other.hasLabel()) return false;
if (hasLabel()) {
if (!getLabel()
.equals(other.getLabel())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue();
}
if (hasLabel()) {
hash = (37 * hash) + LABEL_FIELD_NUMBER;
hash = (53 * hash) + getLabel().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.EnumValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.EnumValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.EnumValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.EnumValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.EnumValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.EnumValue)
org.yamcs.protobuf.Table.EnumValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_EnumValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_EnumValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.EnumValue.class, org.yamcs.protobuf.Table.EnumValue.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.EnumValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
value_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
label_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_EnumValue_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.EnumValue getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.EnumValue.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.EnumValue build() {
org.yamcs.protobuf.Table.EnumValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.EnumValue buildPartial() {
org.yamcs.protobuf.Table.EnumValue result = new org.yamcs.protobuf.Table.EnumValue(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.value_ = value_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.label_ = label_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.EnumValue) {
return mergeFrom((org.yamcs.protobuf.Table.EnumValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.EnumValue other) {
if (other == org.yamcs.protobuf.Table.EnumValue.getDefaultInstance()) return this;
if (other.hasValue()) {
setValue(other.getValue());
}
if (other.hasLabel()) {
bitField0_ |= 0x00000002;
label_ = other.label_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.EnumValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.EnumValue) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int value_ ;
/**
* optional int32 value = 1;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int32 value = 1;
* @return The value.
*/
@java.lang.Override
public int getValue() {
return value_;
}
/**
* optional int32 value = 1;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(int value) {
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
* optional int32 value = 1;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = 0;
onChanged();
return this;
}
private java.lang.Object label_ = "";
/**
* optional string label = 2;
* @return Whether the label field is set.
*/
public boolean hasLabel() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string label = 2;
* @return The label.
*/
public java.lang.String getLabel() {
java.lang.Object ref = label_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
label_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string label = 2;
* @return The bytes for label.
*/
public com.google.protobuf.ByteString
getLabelBytes() {
java.lang.Object ref = label_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
label_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string label = 2;
* @param value The label to set.
* @return This builder for chaining.
*/
public Builder setLabel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
label_ = value;
onChanged();
return this;
}
/**
* optional string label = 2;
* @return This builder for chaining.
*/
public Builder clearLabel() {
bitField0_ = (bitField0_ & ~0x00000002);
label_ = getDefaultInstance().getLabel();
onChanged();
return this;
}
/**
* optional string label = 2;
* @param value The bytes for label to set.
* @return This builder for chaining.
*/
public Builder setLabelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
label_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.EnumValue)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.EnumValue)
private static final org.yamcs.protobuf.Table.EnumValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.EnumValue();
}
public static org.yamcs.protobuf.Table.EnumValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EnumValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EnumValue(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.EnumValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TableInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.TableInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* Table name
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Table name
*
*
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
*
* Table name
*
*
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
java.util.List
getKeyColumnList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
org.yamcs.protobuf.Table.ColumnInfo getKeyColumn(int index);
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
int getKeyColumnCount();
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getKeyColumnOrBuilderList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
org.yamcs.protobuf.Table.ColumnInfoOrBuilder getKeyColumnOrBuilder(
int index);
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
java.util.List
getValueColumnList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
org.yamcs.protobuf.Table.ColumnInfo getValueColumn(int index);
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
int getValueColumnCount();
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getValueColumnOrBuilderList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
org.yamcs.protobuf.Table.ColumnInfoOrBuilder getValueColumnOrBuilder(
int index);
/**
* optional string script = 4;
* @return Whether the script field is set.
*/
boolean hasScript();
/**
* optional string script = 4;
* @return The script.
*/
java.lang.String getScript();
/**
* optional string script = 4;
* @return The bytes for script.
*/
com.google.protobuf.ByteString
getScriptBytes();
/**
* repeated string histogramColumn = 5;
* @return A list containing the histogramColumn.
*/
java.util.List
getHistogramColumnList();
/**
* repeated string histogramColumn = 5;
* @return The count of histogramColumn.
*/
int getHistogramColumnCount();
/**
* repeated string histogramColumn = 5;
* @param index The index of the element to return.
* @return The histogramColumn at the given index.
*/
java.lang.String getHistogramColumn(int index);
/**
* repeated string histogramColumn = 5;
* @param index The index of the value to return.
* @return The bytes of the histogramColumn at the given index.
*/
com.google.protobuf.ByteString
getHistogramColumnBytes(int index);
/**
* optional string storageEngine = 6;
* @return Whether the storageEngine field is set.
*/
boolean hasStorageEngine();
/**
* optional string storageEngine = 6;
* @return The storageEngine.
*/
java.lang.String getStorageEngine();
/**
* optional string storageEngine = 6;
* @return The bytes for storageEngine.
*/
com.google.protobuf.ByteString
getStorageEngineBytes();
/**
* optional int32 formatVersion = 7;
* @return Whether the formatVersion field is set.
*/
boolean hasFormatVersion();
/**
* optional int32 formatVersion = 7;
* @return The formatVersion.
*/
int getFormatVersion();
/**
* optional string tablespace = 8;
* @return Whether the tablespace field is set.
*/
boolean hasTablespace();
/**
* optional string tablespace = 8;
* @return The tablespace.
*/
java.lang.String getTablespace();
/**
* optional string tablespace = 8;
* @return The bytes for tablespace.
*/
com.google.protobuf.ByteString
getTablespaceBytes();
/**
* optional bool compressed = 9;
* @return Whether the compressed field is set.
*/
boolean hasCompressed();
/**
* optional bool compressed = 9;
* @return The compressed.
*/
boolean getCompressed();
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
* @return Whether the partitioningInfo field is set.
*/
boolean hasPartitioningInfo();
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
* @return The partitioningInfo.
*/
org.yamcs.protobuf.Table.PartitioningInfo getPartitioningInfo();
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
*/
org.yamcs.protobuf.Table.PartitioningInfoOrBuilder getPartitioningInfoOrBuilder();
}
/**
* Protobuf type {@code yamcs.protobuf.table.TableInfo}
*/
public static final class TableInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.TableInfo)
TableInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use TableInfo.newBuilder() to construct.
private TableInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TableInfo() {
name_ = "";
keyColumn_ = java.util.Collections.emptyList();
valueColumn_ = java.util.Collections.emptyList();
script_ = "";
histogramColumn_ = com.google.protobuf.LazyStringArrayList.EMPTY;
storageEngine_ = "";
tablespace_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TableInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TableInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
keyColumn_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
keyColumn_.add(
input.readMessage(org.yamcs.protobuf.Table.ColumnInfo.PARSER, extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
valueColumn_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
valueColumn_.add(
input.readMessage(org.yamcs.protobuf.Table.ColumnInfo.PARSER, extensionRegistry));
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
script_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
histogramColumn_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000010;
}
histogramColumn_.add(bs);
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
storageEngine_ = bs;
break;
}
case 56: {
bitField0_ |= 0x00000008;
formatVersion_ = input.readInt32();
break;
}
case 66: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
tablespace_ = bs;
break;
}
case 72: {
bitField0_ |= 0x00000020;
compressed_ = input.readBool();
break;
}
case 82: {
org.yamcs.protobuf.Table.PartitioningInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) != 0)) {
subBuilder = partitioningInfo_.toBuilder();
}
partitioningInfo_ = input.readMessage(org.yamcs.protobuf.Table.PartitioningInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(partitioningInfo_);
partitioningInfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
keyColumn_ = java.util.Collections.unmodifiableList(keyColumn_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
valueColumn_ = java.util.Collections.unmodifiableList(valueColumn_);
}
if (((mutable_bitField0_ & 0x00000010) != 0)) {
histogramColumn_ = histogramColumn_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.TableInfo.class, org.yamcs.protobuf.Table.TableInfo.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
*
* Table name
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Table name
*
*
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Table name
*
*
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KEYCOLUMN_FIELD_NUMBER = 2;
private java.util.List keyColumn_;
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
@java.lang.Override
public java.util.List getKeyColumnList() {
return keyColumn_;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getKeyColumnOrBuilderList() {
return keyColumn_;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
@java.lang.Override
public int getKeyColumnCount() {
return keyColumn_.size();
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfo getKeyColumn(int index) {
return keyColumn_.get(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfoOrBuilder getKeyColumnOrBuilder(
int index) {
return keyColumn_.get(index);
}
public static final int VALUECOLUMN_FIELD_NUMBER = 3;
private java.util.List valueColumn_;
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
@java.lang.Override
public java.util.List getValueColumnList() {
return valueColumn_;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getValueColumnOrBuilderList() {
return valueColumn_;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
@java.lang.Override
public int getValueColumnCount() {
return valueColumn_.size();
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfo getValueColumn(int index) {
return valueColumn_.get(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.ColumnInfoOrBuilder getValueColumnOrBuilder(
int index) {
return valueColumn_.get(index);
}
public static final int SCRIPT_FIELD_NUMBER = 4;
private volatile java.lang.Object script_;
/**
* optional string script = 4;
* @return Whether the script field is set.
*/
@java.lang.Override
public boolean hasScript() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string script = 4;
* @return The script.
*/
@java.lang.Override
public java.lang.String getScript() {
java.lang.Object ref = script_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
script_ = s;
}
return s;
}
}
/**
* optional string script = 4;
* @return The bytes for script.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getScriptBytes() {
java.lang.Object ref = script_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
script_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HISTOGRAMCOLUMN_FIELD_NUMBER = 5;
private com.google.protobuf.LazyStringList histogramColumn_;
/**
* repeated string histogramColumn = 5;
* @return A list containing the histogramColumn.
*/
public com.google.protobuf.ProtocolStringList
getHistogramColumnList() {
return histogramColumn_;
}
/**
* repeated string histogramColumn = 5;
* @return The count of histogramColumn.
*/
public int getHistogramColumnCount() {
return histogramColumn_.size();
}
/**
* repeated string histogramColumn = 5;
* @param index The index of the element to return.
* @return The histogramColumn at the given index.
*/
public java.lang.String getHistogramColumn(int index) {
return histogramColumn_.get(index);
}
/**
* repeated string histogramColumn = 5;
* @param index The index of the value to return.
* @return The bytes of the histogramColumn at the given index.
*/
public com.google.protobuf.ByteString
getHistogramColumnBytes(int index) {
return histogramColumn_.getByteString(index);
}
public static final int STORAGEENGINE_FIELD_NUMBER = 6;
private volatile java.lang.Object storageEngine_;
/**
* optional string storageEngine = 6;
* @return Whether the storageEngine field is set.
*/
@java.lang.Override
public boolean hasStorageEngine() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string storageEngine = 6;
* @return The storageEngine.
*/
@java.lang.Override
public java.lang.String getStorageEngine() {
java.lang.Object ref = storageEngine_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
storageEngine_ = s;
}
return s;
}
}
/**
* optional string storageEngine = 6;
* @return The bytes for storageEngine.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStorageEngineBytes() {
java.lang.Object ref = storageEngine_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
storageEngine_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FORMATVERSION_FIELD_NUMBER = 7;
private int formatVersion_;
/**
* optional int32 formatVersion = 7;
* @return Whether the formatVersion field is set.
*/
@java.lang.Override
public boolean hasFormatVersion() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int32 formatVersion = 7;
* @return The formatVersion.
*/
@java.lang.Override
public int getFormatVersion() {
return formatVersion_;
}
public static final int TABLESPACE_FIELD_NUMBER = 8;
private volatile java.lang.Object tablespace_;
/**
* optional string tablespace = 8;
* @return Whether the tablespace field is set.
*/
@java.lang.Override
public boolean hasTablespace() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string tablespace = 8;
* @return The tablespace.
*/
@java.lang.Override
public java.lang.String getTablespace() {
java.lang.Object ref = tablespace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
tablespace_ = s;
}
return s;
}
}
/**
* optional string tablespace = 8;
* @return The bytes for tablespace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTablespaceBytes() {
java.lang.Object ref = tablespace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tablespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMPRESSED_FIELD_NUMBER = 9;
private boolean compressed_;
/**
* optional bool compressed = 9;
* @return Whether the compressed field is set.
*/
@java.lang.Override
public boolean hasCompressed() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional bool compressed = 9;
* @return The compressed.
*/
@java.lang.Override
public boolean getCompressed() {
return compressed_;
}
public static final int PARTITIONINGINFO_FIELD_NUMBER = 10;
private org.yamcs.protobuf.Table.PartitioningInfo partitioningInfo_;
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
* @return Whether the partitioningInfo field is set.
*/
@java.lang.Override
public boolean hasPartitioningInfo() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
* @return The partitioningInfo.
*/
@java.lang.Override
public org.yamcs.protobuf.Table.PartitioningInfo getPartitioningInfo() {
return partitioningInfo_ == null ? org.yamcs.protobuf.Table.PartitioningInfo.getDefaultInstance() : partitioningInfo_;
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
*/
@java.lang.Override
public org.yamcs.protobuf.Table.PartitioningInfoOrBuilder getPartitioningInfoOrBuilder() {
return partitioningInfo_ == null ? org.yamcs.protobuf.Table.PartitioningInfo.getDefaultInstance() : partitioningInfo_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
for (int i = 0; i < keyColumn_.size(); i++) {
output.writeMessage(2, keyColumn_.get(i));
}
for (int i = 0; i < valueColumn_.size(); i++) {
output.writeMessage(3, valueColumn_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, script_);
}
for (int i = 0; i < histogramColumn_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, histogramColumn_.getRaw(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, storageEngine_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(7, formatVersion_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, tablespace_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeBool(9, compressed_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(10, getPartitioningInfo());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
for (int i = 0; i < keyColumn_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, keyColumn_.get(i));
}
for (int i = 0; i < valueColumn_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, valueColumn_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, script_);
}
{
int dataSize = 0;
for (int i = 0; i < histogramColumn_.size(); i++) {
dataSize += computeStringSizeNoTag(histogramColumn_.getRaw(i));
}
size += dataSize;
size += 1 * getHistogramColumnList().size();
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, storageEngine_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(7, formatVersion_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, tablespace_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, compressed_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getPartitioningInfo());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.TableInfo)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.TableInfo other = (org.yamcs.protobuf.Table.TableInfo) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!getKeyColumnList()
.equals(other.getKeyColumnList())) return false;
if (!getValueColumnList()
.equals(other.getValueColumnList())) return false;
if (hasScript() != other.hasScript()) return false;
if (hasScript()) {
if (!getScript()
.equals(other.getScript())) return false;
}
if (!getHistogramColumnList()
.equals(other.getHistogramColumnList())) return false;
if (hasStorageEngine() != other.hasStorageEngine()) return false;
if (hasStorageEngine()) {
if (!getStorageEngine()
.equals(other.getStorageEngine())) return false;
}
if (hasFormatVersion() != other.hasFormatVersion()) return false;
if (hasFormatVersion()) {
if (getFormatVersion()
!= other.getFormatVersion()) return false;
}
if (hasTablespace() != other.hasTablespace()) return false;
if (hasTablespace()) {
if (!getTablespace()
.equals(other.getTablespace())) return false;
}
if (hasCompressed() != other.hasCompressed()) return false;
if (hasCompressed()) {
if (getCompressed()
!= other.getCompressed()) return false;
}
if (hasPartitioningInfo() != other.hasPartitioningInfo()) return false;
if (hasPartitioningInfo()) {
if (!getPartitioningInfo()
.equals(other.getPartitioningInfo())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (getKeyColumnCount() > 0) {
hash = (37 * hash) + KEYCOLUMN_FIELD_NUMBER;
hash = (53 * hash) + getKeyColumnList().hashCode();
}
if (getValueColumnCount() > 0) {
hash = (37 * hash) + VALUECOLUMN_FIELD_NUMBER;
hash = (53 * hash) + getValueColumnList().hashCode();
}
if (hasScript()) {
hash = (37 * hash) + SCRIPT_FIELD_NUMBER;
hash = (53 * hash) + getScript().hashCode();
}
if (getHistogramColumnCount() > 0) {
hash = (37 * hash) + HISTOGRAMCOLUMN_FIELD_NUMBER;
hash = (53 * hash) + getHistogramColumnList().hashCode();
}
if (hasStorageEngine()) {
hash = (37 * hash) + STORAGEENGINE_FIELD_NUMBER;
hash = (53 * hash) + getStorageEngine().hashCode();
}
if (hasFormatVersion()) {
hash = (37 * hash) + FORMATVERSION_FIELD_NUMBER;
hash = (53 * hash) + getFormatVersion();
}
if (hasTablespace()) {
hash = (37 * hash) + TABLESPACE_FIELD_NUMBER;
hash = (53 * hash) + getTablespace().hashCode();
}
if (hasCompressed()) {
hash = (37 * hash) + COMPRESSED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getCompressed());
}
if (hasPartitioningInfo()) {
hash = (37 * hash) + PARTITIONINGINFO_FIELD_NUMBER;
hash = (53 * hash) + getPartitioningInfo().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.TableInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.TableInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.TableInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.TableInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.TableInfo)
org.yamcs.protobuf.Table.TableInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.TableInfo.class, org.yamcs.protobuf.Table.TableInfo.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.TableInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getKeyColumnFieldBuilder();
getValueColumnFieldBuilder();
getPartitioningInfoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (keyColumnBuilder_ == null) {
keyColumn_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
keyColumnBuilder_.clear();
}
if (valueColumnBuilder_ == null) {
valueColumn_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
valueColumnBuilder_.clear();
}
script_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
histogramColumn_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
storageEngine_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
formatVersion_ = 0;
bitField0_ = (bitField0_ & ~0x00000040);
tablespace_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
compressed_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
if (partitioningInfoBuilder_ == null) {
partitioningInfo_ = null;
} else {
partitioningInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_TableInfo_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableInfo getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.TableInfo.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableInfo build() {
org.yamcs.protobuf.Table.TableInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableInfo buildPartial() {
org.yamcs.protobuf.Table.TableInfo result = new org.yamcs.protobuf.Table.TableInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (keyColumnBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
keyColumn_ = java.util.Collections.unmodifiableList(keyColumn_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.keyColumn_ = keyColumn_;
} else {
result.keyColumn_ = keyColumnBuilder_.build();
}
if (valueColumnBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
valueColumn_ = java.util.Collections.unmodifiableList(valueColumn_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.valueColumn_ = valueColumn_;
} else {
result.valueColumn_ = valueColumnBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.script_ = script_;
if (((bitField0_ & 0x00000010) != 0)) {
histogramColumn_ = histogramColumn_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000010);
}
result.histogramColumn_ = histogramColumn_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.storageEngine_ = storageEngine_;
if (((from_bitField0_ & 0x00000040) != 0)) {
result.formatVersion_ = formatVersion_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.tablespace_ = tablespace_;
if (((from_bitField0_ & 0x00000100) != 0)) {
result.compressed_ = compressed_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
if (partitioningInfoBuilder_ == null) {
result.partitioningInfo_ = partitioningInfo_;
} else {
result.partitioningInfo_ = partitioningInfoBuilder_.build();
}
to_bitField0_ |= 0x00000040;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.TableInfo) {
return mergeFrom((org.yamcs.protobuf.Table.TableInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.TableInfo other) {
if (other == org.yamcs.protobuf.Table.TableInfo.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (keyColumnBuilder_ == null) {
if (!other.keyColumn_.isEmpty()) {
if (keyColumn_.isEmpty()) {
keyColumn_ = other.keyColumn_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureKeyColumnIsMutable();
keyColumn_.addAll(other.keyColumn_);
}
onChanged();
}
} else {
if (!other.keyColumn_.isEmpty()) {
if (keyColumnBuilder_.isEmpty()) {
keyColumnBuilder_.dispose();
keyColumnBuilder_ = null;
keyColumn_ = other.keyColumn_;
bitField0_ = (bitField0_ & ~0x00000002);
keyColumnBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getKeyColumnFieldBuilder() : null;
} else {
keyColumnBuilder_.addAllMessages(other.keyColumn_);
}
}
}
if (valueColumnBuilder_ == null) {
if (!other.valueColumn_.isEmpty()) {
if (valueColumn_.isEmpty()) {
valueColumn_ = other.valueColumn_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureValueColumnIsMutable();
valueColumn_.addAll(other.valueColumn_);
}
onChanged();
}
} else {
if (!other.valueColumn_.isEmpty()) {
if (valueColumnBuilder_.isEmpty()) {
valueColumnBuilder_.dispose();
valueColumnBuilder_ = null;
valueColumn_ = other.valueColumn_;
bitField0_ = (bitField0_ & ~0x00000004);
valueColumnBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getValueColumnFieldBuilder() : null;
} else {
valueColumnBuilder_.addAllMessages(other.valueColumn_);
}
}
}
if (other.hasScript()) {
bitField0_ |= 0x00000008;
script_ = other.script_;
onChanged();
}
if (!other.histogramColumn_.isEmpty()) {
if (histogramColumn_.isEmpty()) {
histogramColumn_ = other.histogramColumn_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureHistogramColumnIsMutable();
histogramColumn_.addAll(other.histogramColumn_);
}
onChanged();
}
if (other.hasStorageEngine()) {
bitField0_ |= 0x00000020;
storageEngine_ = other.storageEngine_;
onChanged();
}
if (other.hasFormatVersion()) {
setFormatVersion(other.getFormatVersion());
}
if (other.hasTablespace()) {
bitField0_ |= 0x00000080;
tablespace_ = other.tablespace_;
onChanged();
}
if (other.hasCompressed()) {
setCompressed(other.getCompressed());
}
if (other.hasPartitioningInfo()) {
mergePartitioningInfo(other.getPartitioningInfo());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.TableInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.TableInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* Table name
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Table name
*
*
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Table name
*
*
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Table name
*
*
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
*
* Table name
*
*
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Table name
*
*
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private java.util.List keyColumn_ =
java.util.Collections.emptyList();
private void ensureKeyColumnIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
keyColumn_ = new java.util.ArrayList(keyColumn_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnInfo, org.yamcs.protobuf.Table.ColumnInfo.Builder, org.yamcs.protobuf.Table.ColumnInfoOrBuilder> keyColumnBuilder_;
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public java.util.List getKeyColumnList() {
if (keyColumnBuilder_ == null) {
return java.util.Collections.unmodifiableList(keyColumn_);
} else {
return keyColumnBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public int getKeyColumnCount() {
if (keyColumnBuilder_ == null) {
return keyColumn_.size();
} else {
return keyColumnBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public org.yamcs.protobuf.Table.ColumnInfo getKeyColumn(int index) {
if (keyColumnBuilder_ == null) {
return keyColumn_.get(index);
} else {
return keyColumnBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public Builder setKeyColumn(
int index, org.yamcs.protobuf.Table.ColumnInfo value) {
if (keyColumnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyColumnIsMutable();
keyColumn_.set(index, value);
onChanged();
} else {
keyColumnBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public Builder setKeyColumn(
int index, org.yamcs.protobuf.Table.ColumnInfo.Builder builderForValue) {
if (keyColumnBuilder_ == null) {
ensureKeyColumnIsMutable();
keyColumn_.set(index, builderForValue.build());
onChanged();
} else {
keyColumnBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public Builder addKeyColumn(org.yamcs.protobuf.Table.ColumnInfo value) {
if (keyColumnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyColumnIsMutable();
keyColumn_.add(value);
onChanged();
} else {
keyColumnBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public Builder addKeyColumn(
int index, org.yamcs.protobuf.Table.ColumnInfo value) {
if (keyColumnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyColumnIsMutable();
keyColumn_.add(index, value);
onChanged();
} else {
keyColumnBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public Builder addKeyColumn(
org.yamcs.protobuf.Table.ColumnInfo.Builder builderForValue) {
if (keyColumnBuilder_ == null) {
ensureKeyColumnIsMutable();
keyColumn_.add(builderForValue.build());
onChanged();
} else {
keyColumnBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public Builder addKeyColumn(
int index, org.yamcs.protobuf.Table.ColumnInfo.Builder builderForValue) {
if (keyColumnBuilder_ == null) {
ensureKeyColumnIsMutable();
keyColumn_.add(index, builderForValue.build());
onChanged();
} else {
keyColumnBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public Builder addAllKeyColumn(
java.lang.Iterable extends org.yamcs.protobuf.Table.ColumnInfo> values) {
if (keyColumnBuilder_ == null) {
ensureKeyColumnIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, keyColumn_);
onChanged();
} else {
keyColumnBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public Builder clearKeyColumn() {
if (keyColumnBuilder_ == null) {
keyColumn_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
keyColumnBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public Builder removeKeyColumn(int index) {
if (keyColumnBuilder_ == null) {
ensureKeyColumnIsMutable();
keyColumn_.remove(index);
onChanged();
} else {
keyColumnBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public org.yamcs.protobuf.Table.ColumnInfo.Builder getKeyColumnBuilder(
int index) {
return getKeyColumnFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public org.yamcs.protobuf.Table.ColumnInfoOrBuilder getKeyColumnOrBuilder(
int index) {
if (keyColumnBuilder_ == null) {
return keyColumn_.get(index); } else {
return keyColumnBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getKeyColumnOrBuilderList() {
if (keyColumnBuilder_ != null) {
return keyColumnBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(keyColumn_);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public org.yamcs.protobuf.Table.ColumnInfo.Builder addKeyColumnBuilder() {
return getKeyColumnFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.ColumnInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public org.yamcs.protobuf.Table.ColumnInfo.Builder addKeyColumnBuilder(
int index) {
return getKeyColumnFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.ColumnInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo keyColumn = 2;
*/
public java.util.List
getKeyColumnBuilderList() {
return getKeyColumnFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnInfo, org.yamcs.protobuf.Table.ColumnInfo.Builder, org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getKeyColumnFieldBuilder() {
if (keyColumnBuilder_ == null) {
keyColumnBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnInfo, org.yamcs.protobuf.Table.ColumnInfo.Builder, org.yamcs.protobuf.Table.ColumnInfoOrBuilder>(
keyColumn_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
keyColumn_ = null;
}
return keyColumnBuilder_;
}
private java.util.List valueColumn_ =
java.util.Collections.emptyList();
private void ensureValueColumnIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
valueColumn_ = new java.util.ArrayList(valueColumn_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnInfo, org.yamcs.protobuf.Table.ColumnInfo.Builder, org.yamcs.protobuf.Table.ColumnInfoOrBuilder> valueColumnBuilder_;
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public java.util.List getValueColumnList() {
if (valueColumnBuilder_ == null) {
return java.util.Collections.unmodifiableList(valueColumn_);
} else {
return valueColumnBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public int getValueColumnCount() {
if (valueColumnBuilder_ == null) {
return valueColumn_.size();
} else {
return valueColumnBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public org.yamcs.protobuf.Table.ColumnInfo getValueColumn(int index) {
if (valueColumnBuilder_ == null) {
return valueColumn_.get(index);
} else {
return valueColumnBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public Builder setValueColumn(
int index, org.yamcs.protobuf.Table.ColumnInfo value) {
if (valueColumnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueColumnIsMutable();
valueColumn_.set(index, value);
onChanged();
} else {
valueColumnBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public Builder setValueColumn(
int index, org.yamcs.protobuf.Table.ColumnInfo.Builder builderForValue) {
if (valueColumnBuilder_ == null) {
ensureValueColumnIsMutable();
valueColumn_.set(index, builderForValue.build());
onChanged();
} else {
valueColumnBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public Builder addValueColumn(org.yamcs.protobuf.Table.ColumnInfo value) {
if (valueColumnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueColumnIsMutable();
valueColumn_.add(value);
onChanged();
} else {
valueColumnBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public Builder addValueColumn(
int index, org.yamcs.protobuf.Table.ColumnInfo value) {
if (valueColumnBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueColumnIsMutable();
valueColumn_.add(index, value);
onChanged();
} else {
valueColumnBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public Builder addValueColumn(
org.yamcs.protobuf.Table.ColumnInfo.Builder builderForValue) {
if (valueColumnBuilder_ == null) {
ensureValueColumnIsMutable();
valueColumn_.add(builderForValue.build());
onChanged();
} else {
valueColumnBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public Builder addValueColumn(
int index, org.yamcs.protobuf.Table.ColumnInfo.Builder builderForValue) {
if (valueColumnBuilder_ == null) {
ensureValueColumnIsMutable();
valueColumn_.add(index, builderForValue.build());
onChanged();
} else {
valueColumnBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public Builder addAllValueColumn(
java.lang.Iterable extends org.yamcs.protobuf.Table.ColumnInfo> values) {
if (valueColumnBuilder_ == null) {
ensureValueColumnIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, valueColumn_);
onChanged();
} else {
valueColumnBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public Builder clearValueColumn() {
if (valueColumnBuilder_ == null) {
valueColumn_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
valueColumnBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public Builder removeValueColumn(int index) {
if (valueColumnBuilder_ == null) {
ensureValueColumnIsMutable();
valueColumn_.remove(index);
onChanged();
} else {
valueColumnBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public org.yamcs.protobuf.Table.ColumnInfo.Builder getValueColumnBuilder(
int index) {
return getValueColumnFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public org.yamcs.protobuf.Table.ColumnInfoOrBuilder getValueColumnOrBuilder(
int index) {
if (valueColumnBuilder_ == null) {
return valueColumn_.get(index); } else {
return valueColumnBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getValueColumnOrBuilderList() {
if (valueColumnBuilder_ != null) {
return valueColumnBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(valueColumn_);
}
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public org.yamcs.protobuf.Table.ColumnInfo.Builder addValueColumnBuilder() {
return getValueColumnFieldBuilder().addBuilder(
org.yamcs.protobuf.Table.ColumnInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public org.yamcs.protobuf.Table.ColumnInfo.Builder addValueColumnBuilder(
int index) {
return getValueColumnFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Table.ColumnInfo.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.table.ColumnInfo valueColumn = 3;
*/
public java.util.List
getValueColumnBuilderList() {
return getValueColumnFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnInfo, org.yamcs.protobuf.Table.ColumnInfo.Builder, org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getValueColumnFieldBuilder() {
if (valueColumnBuilder_ == null) {
valueColumnBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Table.ColumnInfo, org.yamcs.protobuf.Table.ColumnInfo.Builder, org.yamcs.protobuf.Table.ColumnInfoOrBuilder>(
valueColumn_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
valueColumn_ = null;
}
return valueColumnBuilder_;
}
private java.lang.Object script_ = "";
/**
* optional string script = 4;
* @return Whether the script field is set.
*/
public boolean hasScript() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string script = 4;
* @return The script.
*/
public java.lang.String getScript() {
java.lang.Object ref = script_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
script_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string script = 4;
* @return The bytes for script.
*/
public com.google.protobuf.ByteString
getScriptBytes() {
java.lang.Object ref = script_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
script_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string script = 4;
* @param value The script to set.
* @return This builder for chaining.
*/
public Builder setScript(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
script_ = value;
onChanged();
return this;
}
/**
* optional string script = 4;
* @return This builder for chaining.
*/
public Builder clearScript() {
bitField0_ = (bitField0_ & ~0x00000008);
script_ = getDefaultInstance().getScript();
onChanged();
return this;
}
/**
* optional string script = 4;
* @param value The bytes for script to set.
* @return This builder for chaining.
*/
public Builder setScriptBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
script_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList histogramColumn_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureHistogramColumnIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
histogramColumn_ = new com.google.protobuf.LazyStringArrayList(histogramColumn_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated string histogramColumn = 5;
* @return A list containing the histogramColumn.
*/
public com.google.protobuf.ProtocolStringList
getHistogramColumnList() {
return histogramColumn_.getUnmodifiableView();
}
/**
* repeated string histogramColumn = 5;
* @return The count of histogramColumn.
*/
public int getHistogramColumnCount() {
return histogramColumn_.size();
}
/**
* repeated string histogramColumn = 5;
* @param index The index of the element to return.
* @return The histogramColumn at the given index.
*/
public java.lang.String getHistogramColumn(int index) {
return histogramColumn_.get(index);
}
/**
* repeated string histogramColumn = 5;
* @param index The index of the value to return.
* @return The bytes of the histogramColumn at the given index.
*/
public com.google.protobuf.ByteString
getHistogramColumnBytes(int index) {
return histogramColumn_.getByteString(index);
}
/**
* repeated string histogramColumn = 5;
* @param index The index to set the value at.
* @param value The histogramColumn to set.
* @return This builder for chaining.
*/
public Builder setHistogramColumn(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureHistogramColumnIsMutable();
histogramColumn_.set(index, value);
onChanged();
return this;
}
/**
* repeated string histogramColumn = 5;
* @param value The histogramColumn to add.
* @return This builder for chaining.
*/
public Builder addHistogramColumn(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureHistogramColumnIsMutable();
histogramColumn_.add(value);
onChanged();
return this;
}
/**
* repeated string histogramColumn = 5;
* @param values The histogramColumn to add.
* @return This builder for chaining.
*/
public Builder addAllHistogramColumn(
java.lang.Iterable values) {
ensureHistogramColumnIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, histogramColumn_);
onChanged();
return this;
}
/**
* repeated string histogramColumn = 5;
* @return This builder for chaining.
*/
public Builder clearHistogramColumn() {
histogramColumn_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* repeated string histogramColumn = 5;
* @param value The bytes of the histogramColumn to add.
* @return This builder for chaining.
*/
public Builder addHistogramColumnBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureHistogramColumnIsMutable();
histogramColumn_.add(value);
onChanged();
return this;
}
private java.lang.Object storageEngine_ = "";
/**
* optional string storageEngine = 6;
* @return Whether the storageEngine field is set.
*/
public boolean hasStorageEngine() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional string storageEngine = 6;
* @return The storageEngine.
*/
public java.lang.String getStorageEngine() {
java.lang.Object ref = storageEngine_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
storageEngine_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string storageEngine = 6;
* @return The bytes for storageEngine.
*/
public com.google.protobuf.ByteString
getStorageEngineBytes() {
java.lang.Object ref = storageEngine_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
storageEngine_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string storageEngine = 6;
* @param value The storageEngine to set.
* @return This builder for chaining.
*/
public Builder setStorageEngine(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
storageEngine_ = value;
onChanged();
return this;
}
/**
* optional string storageEngine = 6;
* @return This builder for chaining.
*/
public Builder clearStorageEngine() {
bitField0_ = (bitField0_ & ~0x00000020);
storageEngine_ = getDefaultInstance().getStorageEngine();
onChanged();
return this;
}
/**
* optional string storageEngine = 6;
* @param value The bytes for storageEngine to set.
* @return This builder for chaining.
*/
public Builder setStorageEngineBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
storageEngine_ = value;
onChanged();
return this;
}
private int formatVersion_ ;
/**
* optional int32 formatVersion = 7;
* @return Whether the formatVersion field is set.
*/
@java.lang.Override
public boolean hasFormatVersion() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional int32 formatVersion = 7;
* @return The formatVersion.
*/
@java.lang.Override
public int getFormatVersion() {
return formatVersion_;
}
/**
* optional int32 formatVersion = 7;
* @param value The formatVersion to set.
* @return This builder for chaining.
*/
public Builder setFormatVersion(int value) {
bitField0_ |= 0x00000040;
formatVersion_ = value;
onChanged();
return this;
}
/**
* optional int32 formatVersion = 7;
* @return This builder for chaining.
*/
public Builder clearFormatVersion() {
bitField0_ = (bitField0_ & ~0x00000040);
formatVersion_ = 0;
onChanged();
return this;
}
private java.lang.Object tablespace_ = "";
/**
* optional string tablespace = 8;
* @return Whether the tablespace field is set.
*/
public boolean hasTablespace() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional string tablespace = 8;
* @return The tablespace.
*/
public java.lang.String getTablespace() {
java.lang.Object ref = tablespace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
tablespace_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string tablespace = 8;
* @return The bytes for tablespace.
*/
public com.google.protobuf.ByteString
getTablespaceBytes() {
java.lang.Object ref = tablespace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tablespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string tablespace = 8;
* @param value The tablespace to set.
* @return This builder for chaining.
*/
public Builder setTablespace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
tablespace_ = value;
onChanged();
return this;
}
/**
* optional string tablespace = 8;
* @return This builder for chaining.
*/
public Builder clearTablespace() {
bitField0_ = (bitField0_ & ~0x00000080);
tablespace_ = getDefaultInstance().getTablespace();
onChanged();
return this;
}
/**
* optional string tablespace = 8;
* @param value The bytes for tablespace to set.
* @return This builder for chaining.
*/
public Builder setTablespaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
tablespace_ = value;
onChanged();
return this;
}
private boolean compressed_ ;
/**
* optional bool compressed = 9;
* @return Whether the compressed field is set.
*/
@java.lang.Override
public boolean hasCompressed() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional bool compressed = 9;
* @return The compressed.
*/
@java.lang.Override
public boolean getCompressed() {
return compressed_;
}
/**
* optional bool compressed = 9;
* @param value The compressed to set.
* @return This builder for chaining.
*/
public Builder setCompressed(boolean value) {
bitField0_ |= 0x00000100;
compressed_ = value;
onChanged();
return this;
}
/**
* optional bool compressed = 9;
* @return This builder for chaining.
*/
public Builder clearCompressed() {
bitField0_ = (bitField0_ & ~0x00000100);
compressed_ = false;
onChanged();
return this;
}
private org.yamcs.protobuf.Table.PartitioningInfo partitioningInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Table.PartitioningInfo, org.yamcs.protobuf.Table.PartitioningInfo.Builder, org.yamcs.protobuf.Table.PartitioningInfoOrBuilder> partitioningInfoBuilder_;
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
* @return Whether the partitioningInfo field is set.
*/
public boolean hasPartitioningInfo() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
* @return The partitioningInfo.
*/
public org.yamcs.protobuf.Table.PartitioningInfo getPartitioningInfo() {
if (partitioningInfoBuilder_ == null) {
return partitioningInfo_ == null ? org.yamcs.protobuf.Table.PartitioningInfo.getDefaultInstance() : partitioningInfo_;
} else {
return partitioningInfoBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
*/
public Builder setPartitioningInfo(org.yamcs.protobuf.Table.PartitioningInfo value) {
if (partitioningInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
partitioningInfo_ = value;
onChanged();
} else {
partitioningInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
*/
public Builder setPartitioningInfo(
org.yamcs.protobuf.Table.PartitioningInfo.Builder builderForValue) {
if (partitioningInfoBuilder_ == null) {
partitioningInfo_ = builderForValue.build();
onChanged();
} else {
partitioningInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
*/
public Builder mergePartitioningInfo(org.yamcs.protobuf.Table.PartitioningInfo value) {
if (partitioningInfoBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0) &&
partitioningInfo_ != null &&
partitioningInfo_ != org.yamcs.protobuf.Table.PartitioningInfo.getDefaultInstance()) {
partitioningInfo_ =
org.yamcs.protobuf.Table.PartitioningInfo.newBuilder(partitioningInfo_).mergeFrom(value).buildPartial();
} else {
partitioningInfo_ = value;
}
onChanged();
} else {
partitioningInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
*/
public Builder clearPartitioningInfo() {
if (partitioningInfoBuilder_ == null) {
partitioningInfo_ = null;
onChanged();
} else {
partitioningInfoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
*/
public org.yamcs.protobuf.Table.PartitioningInfo.Builder getPartitioningInfoBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getPartitioningInfoFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
*/
public org.yamcs.protobuf.Table.PartitioningInfoOrBuilder getPartitioningInfoOrBuilder() {
if (partitioningInfoBuilder_ != null) {
return partitioningInfoBuilder_.getMessageOrBuilder();
} else {
return partitioningInfo_ == null ?
org.yamcs.protobuf.Table.PartitioningInfo.getDefaultInstance() : partitioningInfo_;
}
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo partitioningInfo = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Table.PartitioningInfo, org.yamcs.protobuf.Table.PartitioningInfo.Builder, org.yamcs.protobuf.Table.PartitioningInfoOrBuilder>
getPartitioningInfoFieldBuilder() {
if (partitioningInfoBuilder_ == null) {
partitioningInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Table.PartitioningInfo, org.yamcs.protobuf.Table.PartitioningInfo.Builder, org.yamcs.protobuf.Table.PartitioningInfoOrBuilder>(
getPartitioningInfo(),
getParentForChildren(),
isClean());
partitioningInfo_ = null;
}
return partitioningInfoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.TableInfo)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.TableInfo)
private static final org.yamcs.protobuf.Table.TableInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.TableInfo();
}
public static org.yamcs.protobuf.Table.TableInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TableInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TableInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.TableInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PartitioningInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.PartitioningInfo)
com.google.protobuf.MessageOrBuilder {
/**
* optional .yamcs.protobuf.table.PartitioningInfo.PartitioningType type = 1;
* @return Whether the type field is set.
*/
boolean hasType();
/**
* optional .yamcs.protobuf.table.PartitioningInfo.PartitioningType type = 1;
* @return The type.
*/
org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType getType();
/**
* optional string timeColumn = 2;
* @return Whether the timeColumn field is set.
*/
boolean hasTimeColumn();
/**
* optional string timeColumn = 2;
* @return The timeColumn.
*/
java.lang.String getTimeColumn();
/**
* optional string timeColumn = 2;
* @return The bytes for timeColumn.
*/
com.google.protobuf.ByteString
getTimeColumnBytes();
/**
* optional string timePartitionSchema = 3;
* @return Whether the timePartitionSchema field is set.
*/
boolean hasTimePartitionSchema();
/**
* optional string timePartitionSchema = 3;
* @return The timePartitionSchema.
*/
java.lang.String getTimePartitionSchema();
/**
* optional string timePartitionSchema = 3;
* @return The bytes for timePartitionSchema.
*/
com.google.protobuf.ByteString
getTimePartitionSchemaBytes();
/**
* optional string valueColumn = 4;
* @return Whether the valueColumn field is set.
*/
boolean hasValueColumn();
/**
* optional string valueColumn = 4;
* @return The valueColumn.
*/
java.lang.String getValueColumn();
/**
* optional string valueColumn = 4;
* @return The bytes for valueColumn.
*/
com.google.protobuf.ByteString
getValueColumnBytes();
/**
* optional string valueColumnType = 5;
* @return Whether the valueColumnType field is set.
*/
boolean hasValueColumnType();
/**
* optional string valueColumnType = 5;
* @return The valueColumnType.
*/
java.lang.String getValueColumnType();
/**
* optional string valueColumnType = 5;
* @return The bytes for valueColumnType.
*/
com.google.protobuf.ByteString
getValueColumnTypeBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.table.PartitioningInfo}
*/
public static final class PartitioningInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.table.PartitioningInfo)
PartitioningInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use PartitioningInfo.newBuilder() to construct.
private PartitioningInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PartitioningInfo() {
type_ = 1;
timeColumn_ = "";
timePartitionSchema_ = "";
valueColumn_ = "";
valueColumnType_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PartitioningInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PartitioningInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType value = org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = rawValue;
}
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
timeColumn_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
timePartitionSchema_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
valueColumn_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
valueColumnType_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_PartitioningInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_PartitioningInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.PartitioningInfo.class, org.yamcs.protobuf.Table.PartitioningInfo.Builder.class);
}
/**
* Protobuf enum {@code yamcs.protobuf.table.PartitioningInfo.PartitioningType}
*/
public enum PartitioningType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* TIME = 1;
*/
TIME(1),
/**
* VALUE = 2;
*/
VALUE(2),
/**
* TIME_AND_VALUE = 3;
*/
TIME_AND_VALUE(3),
;
/**
* TIME = 1;
*/
public static final int TIME_VALUE = 1;
/**
* VALUE = 2;
*/
public static final int VALUE_VALUE = 2;
/**
* TIME_AND_VALUE = 3;
*/
public static final int TIME_AND_VALUE_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PartitioningType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static PartitioningType forNumber(int value) {
switch (value) {
case 1: return TIME;
case 2: return VALUE;
case 3: return TIME_AND_VALUE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
PartitioningType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PartitioningType findValueByNumber(int number) {
return PartitioningType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.yamcs.protobuf.Table.PartitioningInfo.getDescriptor().getEnumTypes().get(0);
}
private static final PartitioningType[] VALUES = values();
public static PartitioningType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private PartitioningType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:yamcs.protobuf.table.PartitioningInfo.PartitioningType)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* optional .yamcs.protobuf.table.PartitioningInfo.PartitioningType type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo.PartitioningType type = 1;
* @return The type.
*/
@java.lang.Override public org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType getType() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType result = org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType.valueOf(type_);
return result == null ? org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType.TIME : result;
}
public static final int TIMECOLUMN_FIELD_NUMBER = 2;
private volatile java.lang.Object timeColumn_;
/**
* optional string timeColumn = 2;
* @return Whether the timeColumn field is set.
*/
@java.lang.Override
public boolean hasTimeColumn() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string timeColumn = 2;
* @return The timeColumn.
*/
@java.lang.Override
public java.lang.String getTimeColumn() {
java.lang.Object ref = timeColumn_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
timeColumn_ = s;
}
return s;
}
}
/**
* optional string timeColumn = 2;
* @return The bytes for timeColumn.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTimeColumnBytes() {
java.lang.Object ref = timeColumn_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timeColumn_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMEPARTITIONSCHEMA_FIELD_NUMBER = 3;
private volatile java.lang.Object timePartitionSchema_;
/**
* optional string timePartitionSchema = 3;
* @return Whether the timePartitionSchema field is set.
*/
@java.lang.Override
public boolean hasTimePartitionSchema() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string timePartitionSchema = 3;
* @return The timePartitionSchema.
*/
@java.lang.Override
public java.lang.String getTimePartitionSchema() {
java.lang.Object ref = timePartitionSchema_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
timePartitionSchema_ = s;
}
return s;
}
}
/**
* optional string timePartitionSchema = 3;
* @return The bytes for timePartitionSchema.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTimePartitionSchemaBytes() {
java.lang.Object ref = timePartitionSchema_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timePartitionSchema_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUECOLUMN_FIELD_NUMBER = 4;
private volatile java.lang.Object valueColumn_;
/**
* optional string valueColumn = 4;
* @return Whether the valueColumn field is set.
*/
@java.lang.Override
public boolean hasValueColumn() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string valueColumn = 4;
* @return The valueColumn.
*/
@java.lang.Override
public java.lang.String getValueColumn() {
java.lang.Object ref = valueColumn_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
valueColumn_ = s;
}
return s;
}
}
/**
* optional string valueColumn = 4;
* @return The bytes for valueColumn.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getValueColumnBytes() {
java.lang.Object ref = valueColumn_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
valueColumn_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUECOLUMNTYPE_FIELD_NUMBER = 5;
private volatile java.lang.Object valueColumnType_;
/**
* optional string valueColumnType = 5;
* @return Whether the valueColumnType field is set.
*/
@java.lang.Override
public boolean hasValueColumnType() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string valueColumnType = 5;
* @return The valueColumnType.
*/
@java.lang.Override
public java.lang.String getValueColumnType() {
java.lang.Object ref = valueColumnType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
valueColumnType_ = s;
}
return s;
}
}
/**
* optional string valueColumnType = 5;
* @return The bytes for valueColumnType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getValueColumnTypeBytes() {
java.lang.Object ref = valueColumnType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
valueColumnType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, timeColumn_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, timePartitionSchema_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, valueColumn_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, valueColumnType_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, timeColumn_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, timePartitionSchema_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, valueColumn_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, valueColumnType_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Table.PartitioningInfo)) {
return super.equals(obj);
}
org.yamcs.protobuf.Table.PartitioningInfo other = (org.yamcs.protobuf.Table.PartitioningInfo) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (hasTimeColumn() != other.hasTimeColumn()) return false;
if (hasTimeColumn()) {
if (!getTimeColumn()
.equals(other.getTimeColumn())) return false;
}
if (hasTimePartitionSchema() != other.hasTimePartitionSchema()) return false;
if (hasTimePartitionSchema()) {
if (!getTimePartitionSchema()
.equals(other.getTimePartitionSchema())) return false;
}
if (hasValueColumn() != other.hasValueColumn()) return false;
if (hasValueColumn()) {
if (!getValueColumn()
.equals(other.getValueColumn())) return false;
}
if (hasValueColumnType() != other.hasValueColumnType()) return false;
if (hasValueColumnType()) {
if (!getValueColumnType()
.equals(other.getValueColumnType())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasTimeColumn()) {
hash = (37 * hash) + TIMECOLUMN_FIELD_NUMBER;
hash = (53 * hash) + getTimeColumn().hashCode();
}
if (hasTimePartitionSchema()) {
hash = (37 * hash) + TIMEPARTITIONSCHEMA_FIELD_NUMBER;
hash = (53 * hash) + getTimePartitionSchema().hashCode();
}
if (hasValueColumn()) {
hash = (37 * hash) + VALUECOLUMN_FIELD_NUMBER;
hash = (53 * hash) + getValueColumn().hashCode();
}
if (hasValueColumnType()) {
hash = (37 * hash) + VALUECOLUMNTYPE_FIELD_NUMBER;
hash = (53 * hash) + getValueColumnType().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Table.PartitioningInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Table.PartitioningInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.table.PartitioningInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.table.PartitioningInfo)
org.yamcs.protobuf.Table.PartitioningInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_PartitioningInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_PartitioningInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Table.PartitioningInfo.class, org.yamcs.protobuf.Table.PartitioningInfo.Builder.class);
}
// Construct using org.yamcs.protobuf.Table.PartitioningInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 1;
bitField0_ = (bitField0_ & ~0x00000001);
timeColumn_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
timePartitionSchema_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
valueColumn_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
valueColumnType_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Table.internal_static_yamcs_protobuf_table_PartitioningInfo_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Table.PartitioningInfo getDefaultInstanceForType() {
return org.yamcs.protobuf.Table.PartitioningInfo.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Table.PartitioningInfo build() {
org.yamcs.protobuf.Table.PartitioningInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Table.PartitioningInfo buildPartial() {
org.yamcs.protobuf.Table.PartitioningInfo result = new org.yamcs.protobuf.Table.PartitioningInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.timeColumn_ = timeColumn_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.timePartitionSchema_ = timePartitionSchema_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.valueColumn_ = valueColumn_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.valueColumnType_ = valueColumnType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Table.PartitioningInfo) {
return mergeFrom((org.yamcs.protobuf.Table.PartitioningInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Table.PartitioningInfo other) {
if (other == org.yamcs.protobuf.Table.PartitioningInfo.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasTimeColumn()) {
bitField0_ |= 0x00000002;
timeColumn_ = other.timeColumn_;
onChanged();
}
if (other.hasTimePartitionSchema()) {
bitField0_ |= 0x00000004;
timePartitionSchema_ = other.timePartitionSchema_;
onChanged();
}
if (other.hasValueColumn()) {
bitField0_ |= 0x00000008;
valueColumn_ = other.valueColumn_;
onChanged();
}
if (other.hasValueColumnType()) {
bitField0_ |= 0x00000010;
valueColumnType_ = other.valueColumnType_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Table.PartitioningInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Table.PartitioningInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 1;
/**
* optional .yamcs.protobuf.table.PartitioningInfo.PartitioningType type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo.PartitioningType type = 1;
* @return The type.
*/
@java.lang.Override
public org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType getType() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType result = org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType.valueOf(type_);
return result == null ? org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType.TIME : result;
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo.PartitioningType type = 1;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.yamcs.protobuf.Table.PartitioningInfo.PartitioningType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .yamcs.protobuf.table.PartitioningInfo.PartitioningType type = 1;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 1;
onChanged();
return this;
}
private java.lang.Object timeColumn_ = "";
/**
* optional string timeColumn = 2;
* @return Whether the timeColumn field is set.
*/
public boolean hasTimeColumn() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string timeColumn = 2;
* @return The timeColumn.
*/
public java.lang.String getTimeColumn() {
java.lang.Object ref = timeColumn_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
timeColumn_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string timeColumn = 2;
* @return The bytes for timeColumn.
*/
public com.google.protobuf.ByteString
getTimeColumnBytes() {
java.lang.Object ref = timeColumn_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timeColumn_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string timeColumn = 2;
* @param value The timeColumn to set.
* @return This builder for chaining.
*/
public Builder setTimeColumn(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
timeColumn_ = value;
onChanged();
return this;
}
/**
* optional string timeColumn = 2;
* @return This builder for chaining.
*/
public Builder clearTimeColumn() {
bitField0_ = (bitField0_ & ~0x00000002);
timeColumn_ = getDefaultInstance().getTimeColumn();
onChanged();
return this;
}
/**
* optional string timeColumn = 2;
* @param value The bytes for timeColumn to set.
* @return This builder for chaining.
*/
public Builder setTimeColumnBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
timeColumn_ = value;
onChanged();
return this;
}
private java.lang.Object timePartitionSchema_ = "";
/**
* optional string timePartitionSchema = 3;
* @return Whether the timePartitionSchema field is set.
*/
public boolean hasTimePartitionSchema() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string timePartitionSchema = 3;
* @return The timePartitionSchema.
*/
public java.lang.String getTimePartitionSchema() {
java.lang.Object ref = timePartitionSchema_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
timePartitionSchema_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string timePartitionSchema = 3;
* @return The bytes for timePartitionSchema.
*/
public com.google.protobuf.ByteString
getTimePartitionSchemaBytes() {
java.lang.Object ref = timePartitionSchema_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timePartitionSchema_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string timePartitionSchema = 3;
* @param value The timePartitionSchema to set.
* @return This builder for chaining.
*/
public Builder setTimePartitionSchema(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
timePartitionSchema_ = value;
onChanged();
return this;
}
/**
* optional string timePartitionSchema = 3;
* @return This builder for chaining.
*/
public Builder clearTimePartitionSchema() {
bitField0_ = (bitField0_ & ~0x00000004);
timePartitionSchema_ = getDefaultInstance().getTimePartitionSchema();
onChanged();
return this;
}
/**
* optional string timePartitionSchema = 3;
* @param value The bytes for timePartitionSchema to set.
* @return This builder for chaining.
*/
public Builder setTimePartitionSchemaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
timePartitionSchema_ = value;
onChanged();
return this;
}
private java.lang.Object valueColumn_ = "";
/**
* optional string valueColumn = 4;
* @return Whether the valueColumn field is set.
*/
public boolean hasValueColumn() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string valueColumn = 4;
* @return The valueColumn.
*/
public java.lang.String getValueColumn() {
java.lang.Object ref = valueColumn_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
valueColumn_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string valueColumn = 4;
* @return The bytes for valueColumn.
*/
public com.google.protobuf.ByteString
getValueColumnBytes() {
java.lang.Object ref = valueColumn_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
valueColumn_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string valueColumn = 4;
* @param value The valueColumn to set.
* @return This builder for chaining.
*/
public Builder setValueColumn(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
valueColumn_ = value;
onChanged();
return this;
}
/**
* optional string valueColumn = 4;
* @return This builder for chaining.
*/
public Builder clearValueColumn() {
bitField0_ = (bitField0_ & ~0x00000008);
valueColumn_ = getDefaultInstance().getValueColumn();
onChanged();
return this;
}
/**
* optional string valueColumn = 4;
* @param value The bytes for valueColumn to set.
* @return This builder for chaining.
*/
public Builder setValueColumnBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
valueColumn_ = value;
onChanged();
return this;
}
private java.lang.Object valueColumnType_ = "";
/**
* optional string valueColumnType = 5;
* @return Whether the valueColumnType field is set.
*/
public boolean hasValueColumnType() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string valueColumnType = 5;
* @return The valueColumnType.
*/
public java.lang.String getValueColumnType() {
java.lang.Object ref = valueColumnType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
valueColumnType_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string valueColumnType = 5;
* @return The bytes for valueColumnType.
*/
public com.google.protobuf.ByteString
getValueColumnTypeBytes() {
java.lang.Object ref = valueColumnType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
valueColumnType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string valueColumnType = 5;
* @param value The valueColumnType to set.
* @return This builder for chaining.
*/
public Builder setValueColumnType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
valueColumnType_ = value;
onChanged();
return this;
}
/**
* optional string valueColumnType = 5;
* @return This builder for chaining.
*/
public Builder clearValueColumnType() {
bitField0_ = (bitField0_ & ~0x00000010);
valueColumnType_ = getDefaultInstance().getValueColumnType();
onChanged();
return this;
}
/**
* optional string valueColumnType = 5;
* @param value The bytes for valueColumnType to set.
* @return This builder for chaining.
*/
public Builder setValueColumnTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
valueColumnType_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.table.PartitioningInfo)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.table.PartitioningInfo)
private static final org.yamcs.protobuf.Table.PartitioningInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Table.PartitioningInfo();
}
public static org.yamcs.protobuf.Table.PartitioningInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PartitioningInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PartitioningInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Table.PartitioningInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StreamInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.table.StreamInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* Stream name
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Stream name
*
*
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
*
* Stream name
*
*
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* repeated .yamcs.protobuf.table.ColumnInfo column = 2 [deprecated = true];
*/
@java.lang.Deprecated java.util.List
getColumnList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo column = 2 [deprecated = true];
*/
@java.lang.Deprecated org.yamcs.protobuf.Table.ColumnInfo getColumn(int index);
/**
* repeated .yamcs.protobuf.table.ColumnInfo column = 2 [deprecated = true];
*/
@java.lang.Deprecated int getColumnCount();
/**
* repeated .yamcs.protobuf.table.ColumnInfo column = 2 [deprecated = true];
*/
@java.lang.Deprecated java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getColumnOrBuilderList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo column = 2 [deprecated = true];
*/
@java.lang.Deprecated org.yamcs.protobuf.Table.ColumnInfoOrBuilder getColumnOrBuilder(
int index);
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 5;
*/
java.util.List
getColumnsList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 5;
*/
org.yamcs.protobuf.Table.ColumnInfo getColumns(int index);
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 5;
*/
int getColumnsCount();
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 5;
*/
java.util.List extends org.yamcs.protobuf.Table.ColumnInfoOrBuilder>
getColumnsOrBuilderList();
/**
* repeated .yamcs.protobuf.table.ColumnInfo columns = 5;
*/
org.yamcs.protobuf.Table.ColumnInfoOrBuilder getColumnsOrBuilder(
int index);
/**
* optional string script = 3;
* @return Whether the script field is set.
*/
boolean hasScript();
/**
* optional string script = 3;
* @return The script.
*/
java.lang.String getScript();
/**
* optional string script = 3;
* @return The bytes for script.
*/
com.google.protobuf.ByteString
getScriptBytes();
/**
* optional int64 dataCount = 4;
* @return Whether the dataCount field is set.
*/
boolean hasDataCount();
/**
* optional int64 dataCount = 4;
* @return The dataCount.
*/
long getDataCount();
/**
*
* Subscribers represented in the format ``className@hashCode``.
*
*
* repeated string subscribers = 6;
* @return A list containing the subscribers.
*/
java.util.List
getSubscribersList();
/**
* | | | |