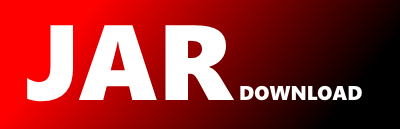
org.yamcs.protobuf.UserInfoOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: yamcs/protobuf/iam/iam.proto
package org.yamcs.protobuf;
public interface UserInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.iam.UserInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* Username
*
*
* optional string name = 17;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Username
*
*
* optional string name = 17;
* @return The name.
*/
java.lang.String getName();
/**
*
* Username
*
*
* optional string name = 17;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Displayed name
*
*
* optional string displayName = 18;
* @return Whether the displayName field is set.
*/
boolean hasDisplayName();
/**
*
* Displayed name
*
*
* optional string displayName = 18;
* @return The displayName.
*/
java.lang.String getDisplayName();
/**
*
* Displayed name
*
*
* optional string displayName = 18;
* @return The bytes for displayName.
*/
com.google.protobuf.ByteString
getDisplayNameBytes();
/**
*
* Email address
*
*
* optional string email = 19;
* @return Whether the email field is set.
*/
boolean hasEmail();
/**
*
* Email address
*
*
* optional string email = 19;
* @return The email.
*/
java.lang.String getEmail();
/**
*
* Email address
*
*
* optional string email = 19;
* @return The bytes for email.
*/
com.google.protobuf.ByteString
getEmailBytes();
/**
*
* Whether the user may login
*
*
* optional bool active = 16;
* @return Whether the active field is set.
*/
boolean hasActive();
/**
*
* Whether the user may login
*
*
* optional bool active = 16;
* @return The active.
*/
boolean getActive();
/**
*
* Whether the user has all privileges
*
*
* optional bool superuser = 13;
* @return Whether the superuser field is set.
*/
boolean hasSuperuser();
/**
*
* Whether the user has all privileges
*
*
* optional bool superuser = 13;
* @return The superuser.
*/
boolean getSuperuser();
/**
*
* User that created this user account
*
*
* optional .yamcs.protobuf.iam.UserInfo createdBy = 20;
* @return Whether the createdBy field is set.
*/
boolean hasCreatedBy();
/**
*
* User that created this user account
*
*
* optional .yamcs.protobuf.iam.UserInfo createdBy = 20;
* @return The createdBy.
*/
org.yamcs.protobuf.UserInfo getCreatedBy();
/**
*
* User that created this user account
*
*
* optional .yamcs.protobuf.iam.UserInfo createdBy = 20;
*/
org.yamcs.protobuf.UserInfoOrBuilder getCreatedByOrBuilder();
/**
*
* When this user was created
*
*
* optional .google.protobuf.Timestamp creationTime = 14;
* @return Whether the creationTime field is set.
*/
boolean hasCreationTime();
/**
*
* When this user was created
*
*
* optional .google.protobuf.Timestamp creationTime = 14;
* @return The creationTime.
*/
com.google.protobuf.Timestamp getCreationTime();
/**
*
* When this user was created
*
*
* optional .google.protobuf.Timestamp creationTime = 14;
*/
com.google.protobuf.TimestampOrBuilder getCreationTimeOrBuilder();
/**
*
* When this user was first activated
*
*
* optional .google.protobuf.Timestamp confirmationTime = 21;
* @return Whether the confirmationTime field is set.
*/
boolean hasConfirmationTime();
/**
*
* When this user was first activated
*
*
* optional .google.protobuf.Timestamp confirmationTime = 21;
* @return The confirmationTime.
*/
com.google.protobuf.Timestamp getConfirmationTime();
/**
*
* When this user was first activated
*
*
* optional .google.protobuf.Timestamp confirmationTime = 21;
*/
com.google.protobuf.TimestampOrBuilder getConfirmationTimeOrBuilder();
/**
*
* When this user last logged in
*
*
* optional .google.protobuf.Timestamp lastLoginTime = 15;
* @return Whether the lastLoginTime field is set.
*/
boolean hasLastLoginTime();
/**
*
* When this user last logged in
*
*
* optional .google.protobuf.Timestamp lastLoginTime = 15;
* @return The lastLoginTime.
*/
com.google.protobuf.Timestamp getLastLoginTime();
/**
*
* When this user last logged in
*
*
* optional .google.protobuf.Timestamp lastLoginTime = 15;
*/
com.google.protobuf.TimestampOrBuilder getLastLoginTimeOrBuilder();
/**
*
* System privileges
*
*
* repeated string systemPrivileges = 26;
* @return A list containing the systemPrivileges.
*/
java.util.List
getSystemPrivilegesList();
/**
*
* System privileges
*
*
* repeated string systemPrivileges = 26;
* @return The count of systemPrivileges.
*/
int getSystemPrivilegesCount();
/**
*
* System privileges
*
*
* repeated string systemPrivileges = 26;
* @param index The index of the element to return.
* @return The systemPrivileges at the given index.
*/
java.lang.String getSystemPrivileges(int index);
/**
*
* System privileges
*
*
* repeated string systemPrivileges = 26;
* @param index The index of the value to return.
* @return The bytes of the systemPrivileges at the given index.
*/
com.google.protobuf.ByteString
getSystemPrivilegesBytes(int index);
/**
*
* Object privileges
*
*
* repeated .yamcs.protobuf.iam.ObjectPrivilegeInfo objectPrivileges = 27;
*/
java.util.List
getObjectPrivilegesList();
/**
*
* Object privileges
*
*
* repeated .yamcs.protobuf.iam.ObjectPrivilegeInfo objectPrivileges = 27;
*/
org.yamcs.protobuf.ObjectPrivilegeInfo getObjectPrivileges(int index);
/**
*
* Object privileges
*
*
* repeated .yamcs.protobuf.iam.ObjectPrivilegeInfo objectPrivileges = 27;
*/
int getObjectPrivilegesCount();
/**
*
* Object privileges
*
*
* repeated .yamcs.protobuf.iam.ObjectPrivilegeInfo objectPrivileges = 27;
*/
java.util.List extends org.yamcs.protobuf.ObjectPrivilegeInfoOrBuilder>
getObjectPrivilegesOrBuilderList();
/**
*
* Object privileges
*
*
* repeated .yamcs.protobuf.iam.ObjectPrivilegeInfo objectPrivileges = 27;
*/
org.yamcs.protobuf.ObjectPrivilegeInfoOrBuilder getObjectPrivilegesOrBuilder(
int index);
/**
*
* Groups that this user is member of
*
*
* repeated .yamcs.protobuf.iam.GroupInfo groups = 22;
*/
java.util.List
getGroupsList();
/**
*
* Groups that this user is member of
*
*
* repeated .yamcs.protobuf.iam.GroupInfo groups = 22;
*/
org.yamcs.protobuf.GroupInfo getGroups(int index);
/**
*
* Groups that this user is member of
*
*
* repeated .yamcs.protobuf.iam.GroupInfo groups = 22;
*/
int getGroupsCount();
/**
*
* Groups that this user is member of
*
*
* repeated .yamcs.protobuf.iam.GroupInfo groups = 22;
*/
java.util.List extends org.yamcs.protobuf.GroupInfoOrBuilder>
getGroupsOrBuilderList();
/**
*
* Groups that this user is member of
*
*
* repeated .yamcs.protobuf.iam.GroupInfo groups = 22;
*/
org.yamcs.protobuf.GroupInfoOrBuilder getGroupsOrBuilder(
int index);
/**
*
* External identities
*
*
* repeated .yamcs.protobuf.iam.ExternalIdentityInfo identities = 23;
*/
java.util.List
getIdentitiesList();
/**
*
* External identities
*
*
* repeated .yamcs.protobuf.iam.ExternalIdentityInfo identities = 23;
*/
org.yamcs.protobuf.ExternalIdentityInfo getIdentities(int index);
/**
*
* External identities
*
*
* repeated .yamcs.protobuf.iam.ExternalIdentityInfo identities = 23;
*/
int getIdentitiesCount();
/**
*
* External identities
*
*
* repeated .yamcs.protobuf.iam.ExternalIdentityInfo identities = 23;
*/
java.util.List extends org.yamcs.protobuf.ExternalIdentityInfoOrBuilder>
getIdentitiesOrBuilderList();
/**
*
* External identities
*
*
* repeated .yamcs.protobuf.iam.ExternalIdentityInfo identities = 23;
*/
org.yamcs.protobuf.ExternalIdentityInfoOrBuilder getIdentitiesOrBuilder(
int index);
/**
*
* Assigned roles
*
*
* repeated .yamcs.protobuf.iam.RoleInfo roles = 24;
*/
java.util.List
getRolesList();
/**
*
* Assigned roles
*
*
* repeated .yamcs.protobuf.iam.RoleInfo roles = 24;
*/
org.yamcs.protobuf.RoleInfo getRoles(int index);
/**
*
* Assigned roles
*
*
* repeated .yamcs.protobuf.iam.RoleInfo roles = 24;
*/
int getRolesCount();
/**
*
* Assigned roles
*
*
* repeated .yamcs.protobuf.iam.RoleInfo roles = 24;
*/
java.util.List extends org.yamcs.protobuf.RoleInfoOrBuilder>
getRolesOrBuilderList();
/**
*
* Assigned roles
*
*
* repeated .yamcs.protobuf.iam.RoleInfo roles = 24;
*/
org.yamcs.protobuf.RoleInfoOrBuilder getRolesOrBuilder(
int index);
/**
*
* Clearance level. If the command clearance feature is enabled,
* then this user attribute is used as an additional check whether
* the user may send certain commands.
* The command clearance feature is disabled by default.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType clearance = 25;
* @return Whether the clearance field is set.
*/
boolean hasClearance();
/**
*
* Clearance level. If the command clearance feature is enabled,
* then this user attribute is used as an additional check whether
* the user may send certain commands.
* The command clearance feature is disabled by default.
*
*
* optional .yamcs.protobuf.mdb.SignificanceInfo.SignificanceLevelType clearance = 25;
* @return The clearance.
*/
org.yamcs.protobuf.Mdb.SignificanceInfo.SignificanceLevelType getClearance();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy